The `strcpy` function in C++ is used to copy a C-style string (null-terminated character array) from a source to a destination.
#include <cstring>
#include <iostream>
int main() {
const char* source = "Hello, World!";
char destination[50]; // Ensure the destination has enough space
strcpy(destination, source);
std::cout << destination << std::endl; // Outputs: Hello, World!
return 0;
}
Understanding the Syntax of strcpy
Basic Syntax
The strcpy function in C++ has a straightforward syntax. It is declared as follows:
char* strcpy(char* destination, const char* source);
This means that it takes two parameters: the destination string where the source string will be copied, and the source string itself. The function will copy the content from the `source` string to the `destination`, including the null terminator.
Parameters Explained
-
destination: This is a pointer to the character array (string) where the copied content will be stored. It must be large enough to accommodate the content of the source string plus the null terminator.
-
source: This pointer refers to the string (array of characters) that needs to be copied. It is declared as `const` to indicate that it should not be modified during the copying process.
Return Value
The strcpy function in C++ returns a pointer to the destination string. Understanding the significance of the return value is crucial, especially when manipulating multiple strings or passing them as function arguments.
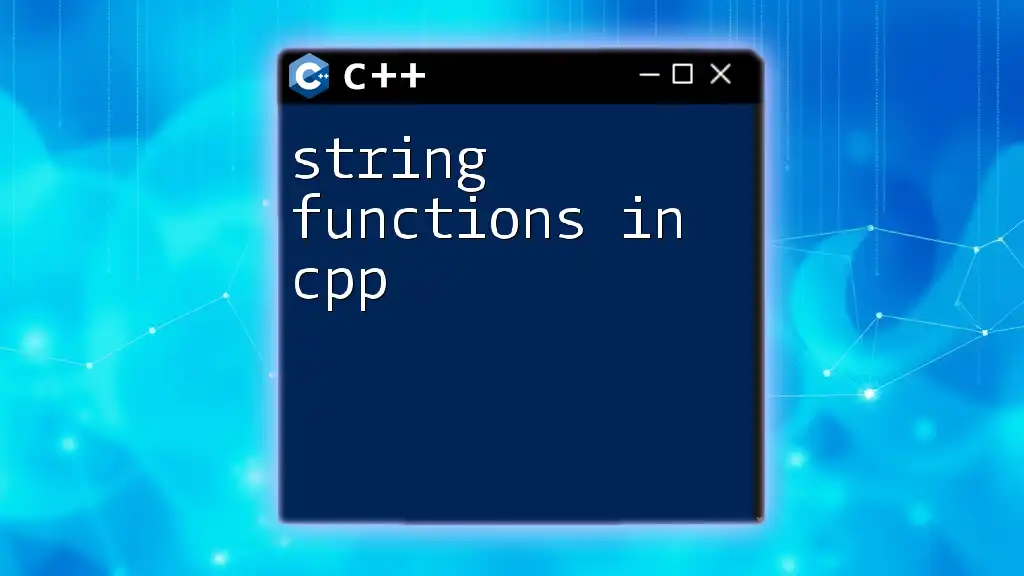
The Role of Header Files
Required Header
To use the `strcpy` function, you must include the appropriate header file:
#include <cstring>
Including this header provides access to all string manipulation functions available, including `strcpy`, `strcat`, and others, making it essential for effective string handling in C++.
Link to Other String Manipulation Functions
The `strcpy` function isn’t the only tool available for string manipulation. Functions like `strcat` for concatenating strings and `strncpy` for safer copying can be vital in different contexts. Understanding how to leverage these functions will allow for more robust string handling in your programs.
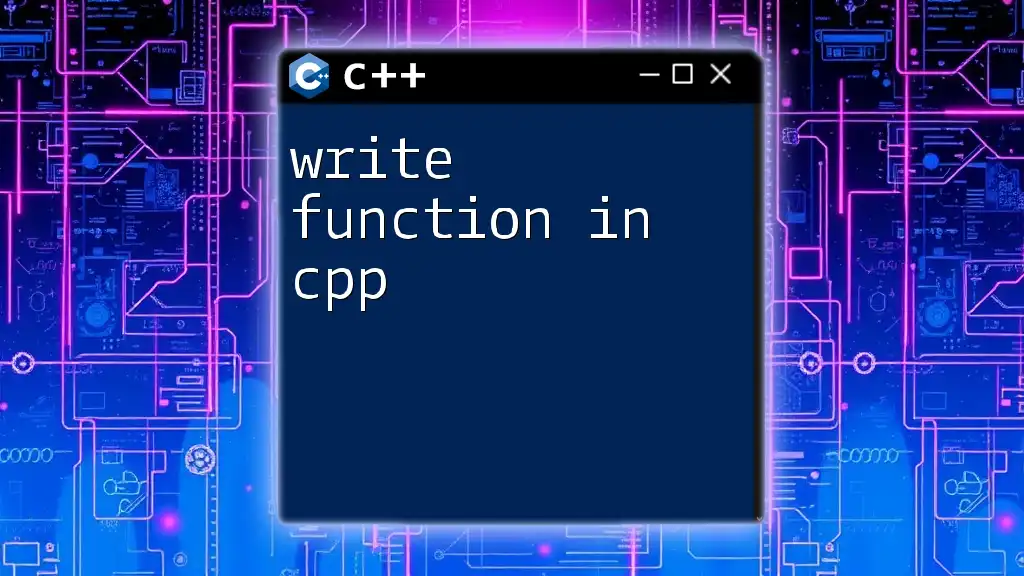
How to Use strcpy in C++
Basic Example of strcpy
To illustrate the use of the `strcpy` function, consider the following example:
#include <iostream>
#include <cstring>
int main() {
char source[] = "Hello, World!";
char destination[50];
strcpy(destination, source);
std::cout << "Source: " << source << std::endl;
std::cout << "Destination: " << destination << std::endl;
return 0;
}
In this example, a string “Hello, World!” is stored in `source`, and `strcpy` is used to copy it to `destination`. The output will confirm that both strings now contain the same content.
Copying String Literals
You can also use `strcpy` with string literals. This can be done as shown in the example below:
char* str_literal_dest = new char[20];
strcpy(str_literal_dest, "String literals");
Here, a dynamic array is allocated, and the string literal "String literals" is copied into `str_literal_dest`. However, ensure that enough memory is allocated to avoid overflow.
Copying User Input
Using `strcpy` to copy user input can be illustrated like this:
char user_input[100];
std::cout << "Enter a string: ";
std::cin.getline(user_input, 100);
char destination[100];
strcpy(destination, user_input);
std::cout << "You entered: " << destination << std::endl;
In this case, the user is prompted to enter a string, which is then copied into the `destination` array. Note that it is crucial to control the size of user input to prevent buffer overflow.
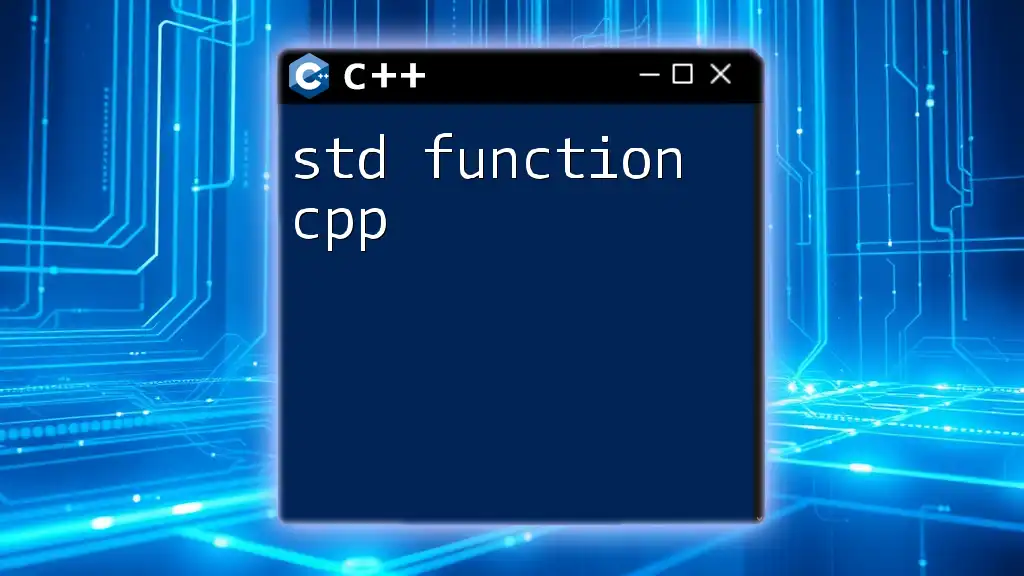
Important Considerations
Buffer Overflow Risks
One of the most critical considerations when using the strcpy function in C++ is the risk of buffer overflow. If the source string exceeds the allocated size of the destination buffer, it can overwrite adjacent memory. This can lead to unpredictable behavior, including crashes and security vulnerabilities. Being vigilant about string lengths is important to mitigate these risks.
Using strncpy as a Safer Alternative
To enhance safety, consider using `strncpy`, which allows specifying the maximum number of characters to copy:
strncpy(destination, source, sizeof(destination) - 1);
destination[sizeof(destination) - 1] = '\0'; // Ensuring null-termination
By controlling the number of characters copied, you can help prevent buffer overflow. However, it’s essential to remember to manually null-terminate the destination string if the source is longer than the maximum limit.
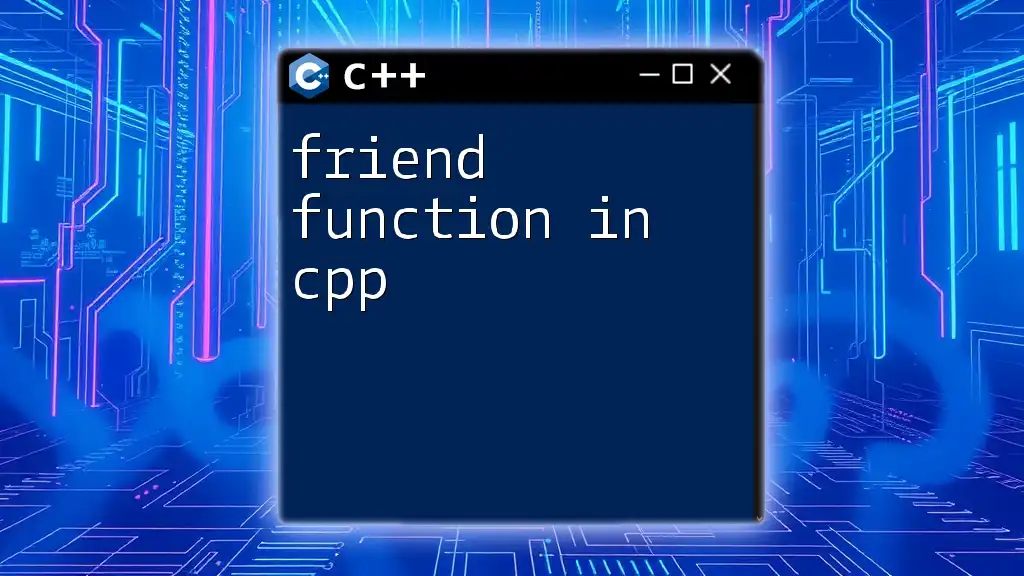
Best Practices with strcpy in C++
Choose the Right Data Type
When working with strings in C++, consider leveraging `std::string`, which automatically manages memory and eliminates many risks associated with character arrays. For example, using `std::string` allows you to write:
std::string source = "Hello";
std::string destination = source; // Direct copy
This approach is cleaner and inherently safer compared to using `strcpy`.
Always Consider String Length
It is important to always check the size of strings involved in copying operations. Functions like `strlen` can help determine the length of the source string:
size_t len = strlen(source);
Ensuring that the destination buffer is adequately sized is vital for safe string manipulation.
When to Avoid strcpy
When developing new applications, it is advisable to avoid using `strcpy` altogether unless absolutely necessary. Functions like `strncpy`, `std::string`, or other C++ Standard Library functions are generally safer and easier to work with.
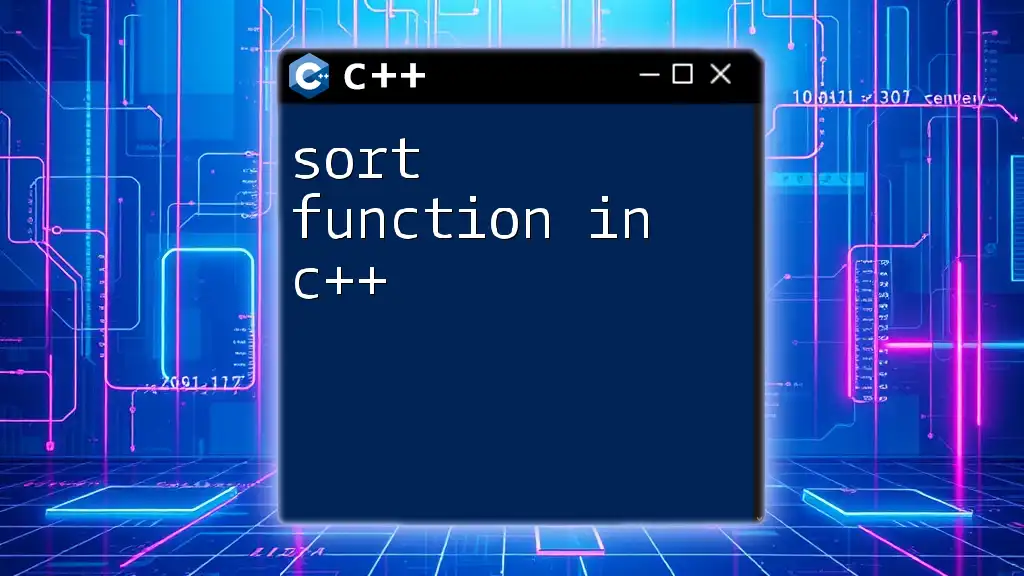
Conclusion
The strcpy function in C++ provides a fundamental capability for string manipulation but comes with significant risks if not used carefully. By keeping the best practices outlined in mind and considering alternative methods of string handling, you can write cleaner, safer, and more maintainable C++ code that handles strings effectively. As you continue your journey in learning C++, mastering string functions will enhance your overall programming prowess.