In C++, a function defined within a class is known as a member function, which can operate on the class's data and is declared within the class definition.
class MyClass {
public:
void displayMessage() {
std::cout << "Hello, C++ Functions in Classes!" << std::endl;
}
};
What is a C++ Class?
A class in C++ serves as a blueprint for creating objects, which encapsulates data and functions in a single unit. The structure of a class typically consists of two principal components:
- Data Members: These are variables that hold the state of the class.
- Member Functions: These functions define the behaviors or actions that the class can perform.
Here is an example of a simple class definition:
class MyClass {
public:
int number; // Data member
void showNumber() { // Member function
std::cout << "Number: " << number << std::endl;
}
};
In the example above, `MyClass` has one data member, `number`, and one member function, `showNumber`, which outputs the value of `number`.
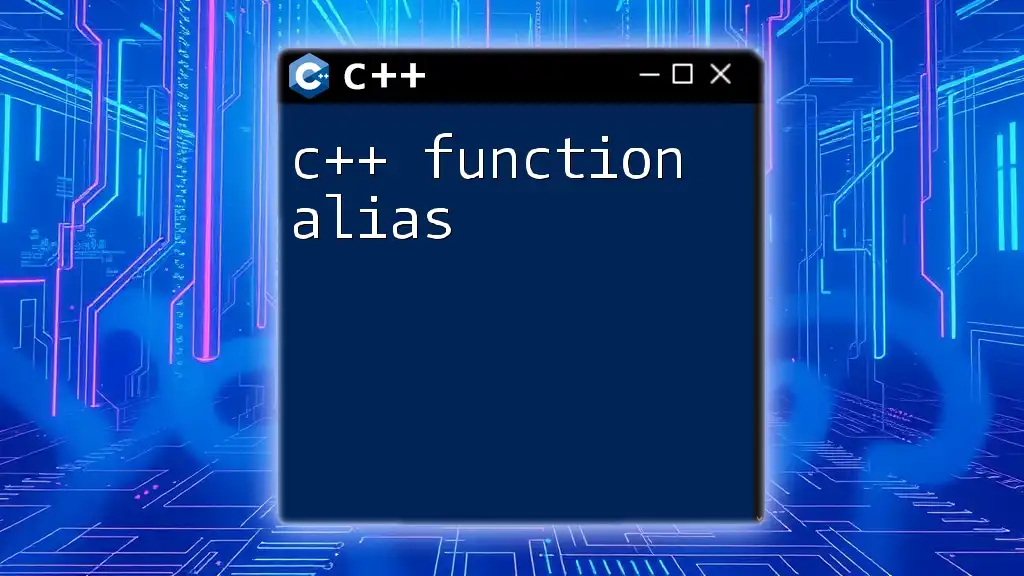
Understanding Member Functions
What are Member Functions?
Member functions are functions that are defined inside a class and operate on the class's data members. They play a crucial role in how objects interact with their data. Member functions can be classified into three categories:
- Instance Member Functions: These require an object of the class to be called.
- Static Member Functions: These can be called without an object and do not have access to instance data.
- Const Member Functions: These functions guarantee that they will not modify any member variables.
Creating a Member Function
The syntax for defining a member function within a class is straightforward. It includes specifying a return type, the function name, and any parameters. Here is an example of a basic member function:
class MyClass {
public:
void displayMessage() {
std::cout << "Hello from MyClass!" << std::endl;
}
};
In this example, `displayMessage` is a simple function that prints a message to the console. To call this function, you would first create an instance of `MyClass`:
MyClass obj;
obj.displayMessage(); // Output: Hello from MyClass!
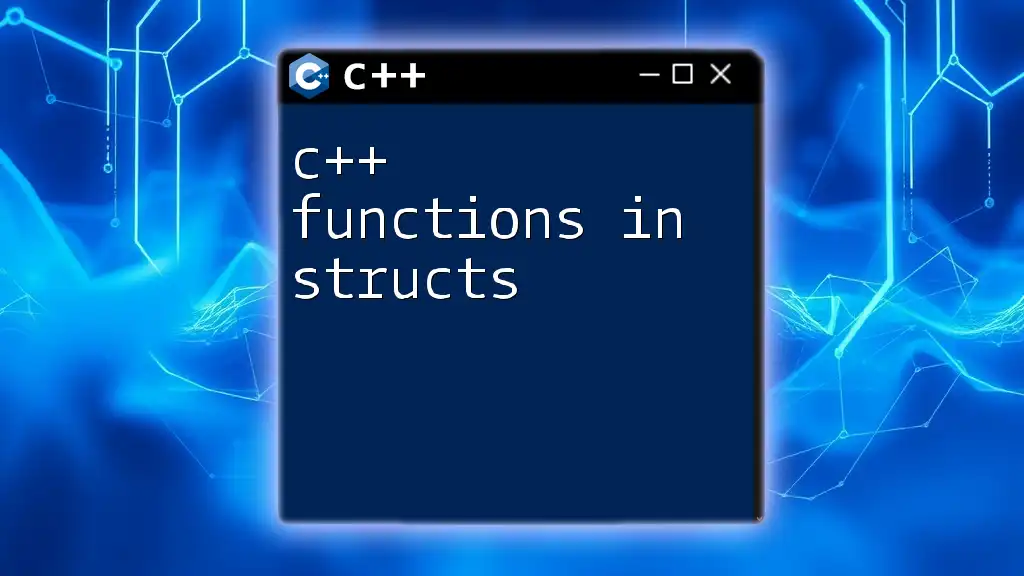
Access Modifiers and Their Impact on Member Functions
Introduction to Access Modifiers
Access modifiers in C++ dictate the accessibility of class members. There are three primary access modifiers:
- Public: Members are accessible from outside the class.
- Private: Members are accessible only within the class.
- Protected: Members are accessible in the class and by derived classes.
Using Access Modifiers with Member Functions
Understanding how access modifiers influence member functions is vital for encapsulating data properly. Here’s an example that shows how access modifiers work within a class:
class MyClass {
private:
int secretNumber;
public:
void setSecretNumber(int num) {
secretNumber = num;
}
int getSecretNumber() {
return secretNumber;
}
};
In this example, `secretNumber` is a private member, meaning it cannot be accessed directly from outside the class. Instead, you must use the public member functions `setSecretNumber` and `getSecretNumber` to interact with it.
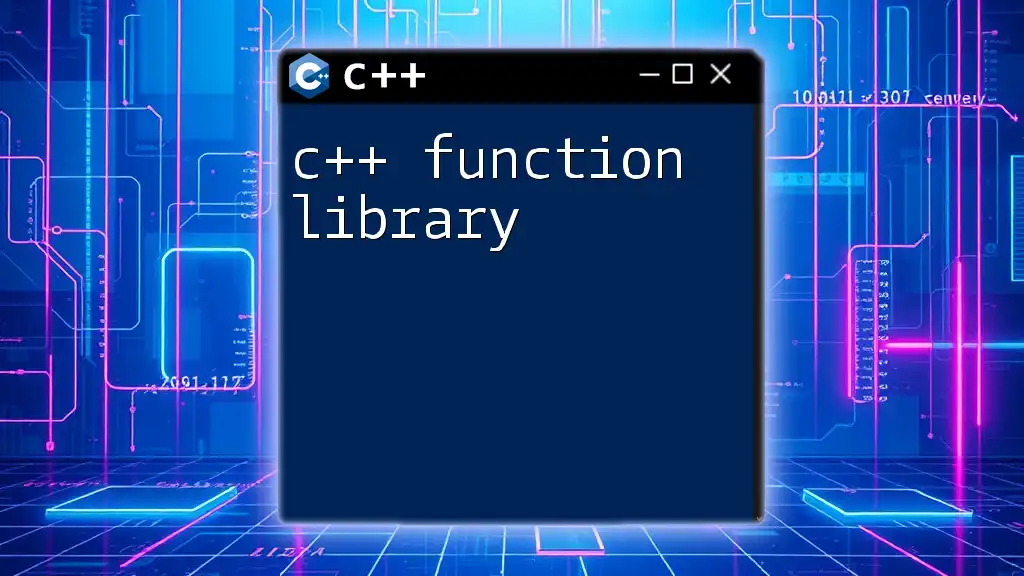
C++ Member Function Overloading
What is Function Overloading?
Function overloading is a feature in C++ that allows you to define multiple functions with the same name but different parameters. This is particularly useful for situations where you need the same logical operation to be performed in a slightly different manner.
Example of Function Overloading in Classes
Here’s a concise example of function overloading within a class:
class Calculator {
public:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
};
In the `Calculator` class, there are two `add` functions: one that takes integers and another that takes doubles. This distinction allows the same function name to operate on different types without confusion.
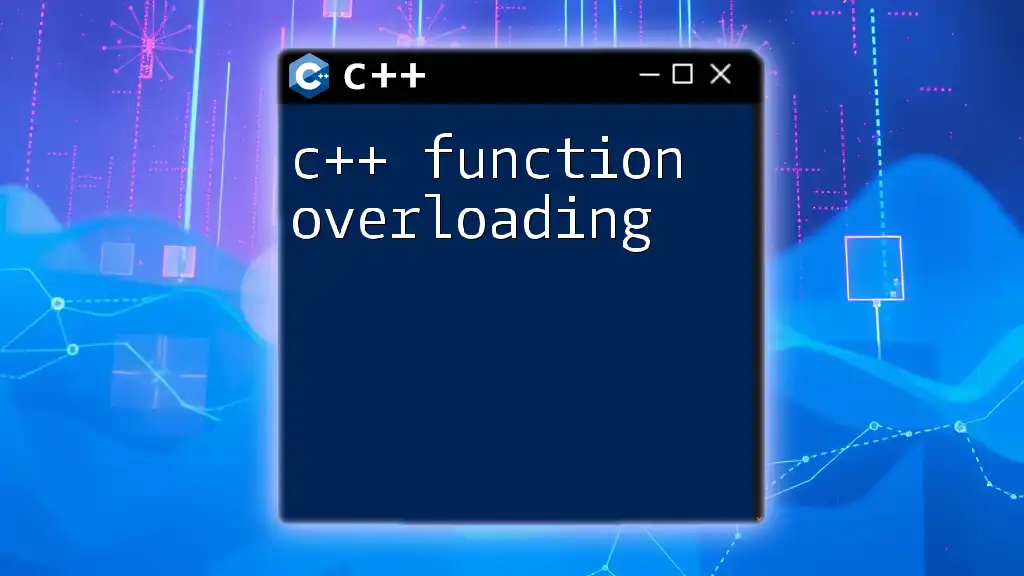
Static Member Functions
Understanding Static Member Functions
Static member functions belong to the class rather than to any specific instance. This means they can be called without creating an object of the class. However, static member functions have their limitations, such as the inability to access non-static data members directly.
When to Use Static Member Functions
Static member functions are particularly useful for utility functions that do not require object-specific data. An example is as follows:
class Counter {
private:
static int count;
public:
static void increment() {
count++;
}
static int getCount() {
return count;
}
};
// Initialize static member
int Counter::count = 0;
In this case, `count` is a static data member, and `increment` and `getCount` are static member functions. They can be called like so:
Counter::increment(); // Increments the static count
std::cout << Counter::getCount(); // Outputs the current count
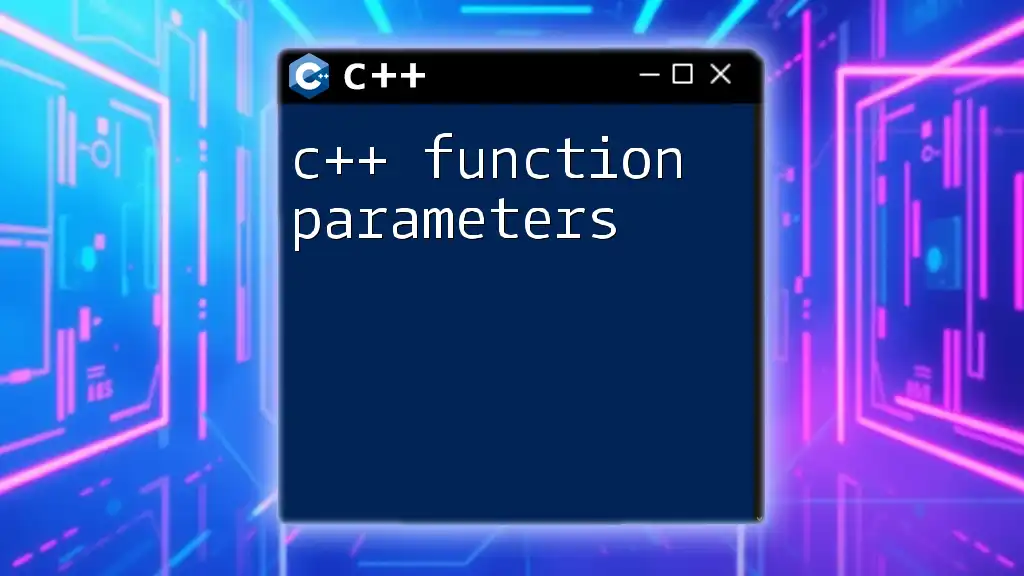
Const Member Functions
What is a Const Member Function?
A `const` member function promises not to modify any member variables of the object. It is marked with the `const` keyword at the end of its declaration, allowing it to be called on objects that are themselves `const`.
Example of Const Member Functions
Here's an example illustrating how a const member function operates:
class MyData {
private:
int data;
public:
MyData(int d) : data(d) {}
int getData() const { // Const member function
return data;
}
};
In the `MyData` class, `getData` is declared `const`, ensuring that it won’t change the `data` member when called, safeguarding the integrity of the object.
const MyData myData(10);
std::cout << myData.getData(); // Safe and reliable access to data
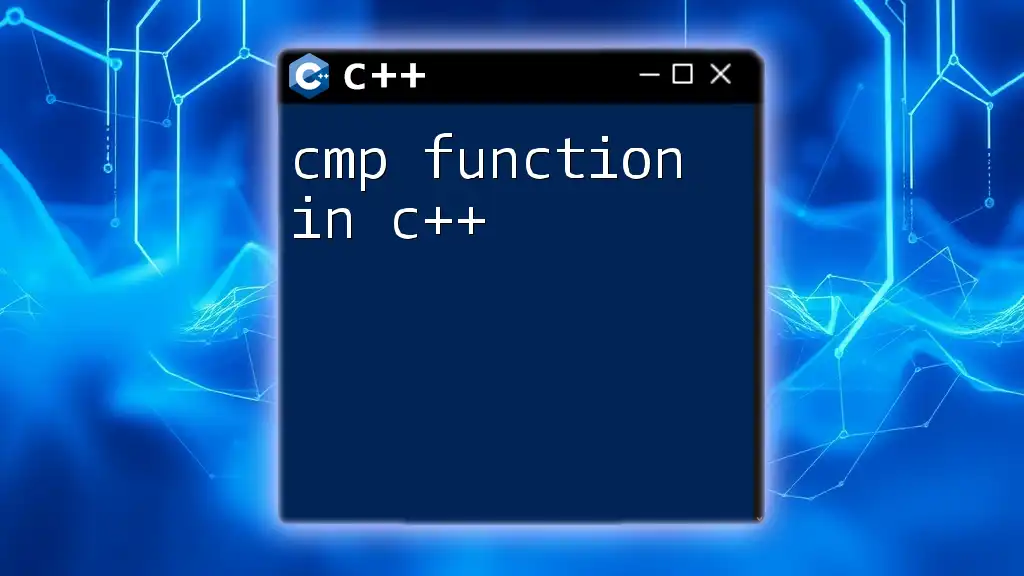
Conclusion
Understanding the nuances of the c++ function in class is essential for anyone looking to harness the real power of object-oriented programming in C++. By grasping the different types of member functions, the role of access modifiers, and concepts like overloading, static functions, and const functions, you can create robust and flexible C++ programs.
As you practice creating and using functions within classes, take the time to explore further resources and courses that can deepen your understanding and skill set. Harness the full potential of C++ for your programming projects!
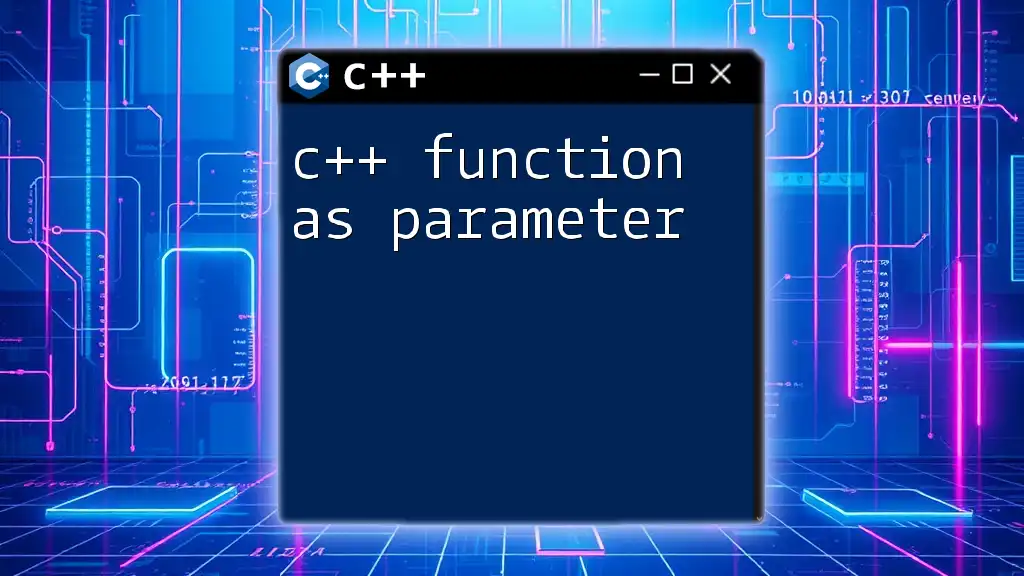
Additional Resources
Explore the following resources to expand your knowledge on C++ classes and functions:
- Official C++ documentation
- Recommended C++ programming tools and IDEs for beginners
- Online courses and tutorials dedicated to C++ programming