In C++, a function return statement sends a value back to the part of the program that called the function, defined by the function's return type.
#include <iostream>
using namespace std;
int add(int a, int b) {
return a + b; // Returning the sum of a and b
}
int main() {
cout << "Sum: " << add(5, 3) << endl; // Outputs: Sum: 8
return 0;
}
Understanding C++ Function Return
What is a Function in C++?
In C++, a function is a block of code designed to perform a particular task. Functions help organize code, making it more modular and reusable. By defining functions, programmers can encapsulate complex operations into manageable parts, allowing for easier debugging and maintenance.
The Concept of Return in C++
The `return` statement is crucial in a C++ function; it defines the end of the function's execution and specifies the value that the function will output. When a `return` statement is executed, control is returned to the calling code along with the associated return value. This is essential for performing calculations or retrieving data that other parts of the program can use.
Why Use a Return Statement?
Utilizing a return statement provides multiple benefits:
- Clarity: It makes it clear what a function is supposed to do by specifying what it outputs.
- Reusability: Functions designed to return values can be easily reused with different inputs.
- Function Chaining: Returned values can be utilized as inputs for other functions, facilitating complex workflows.
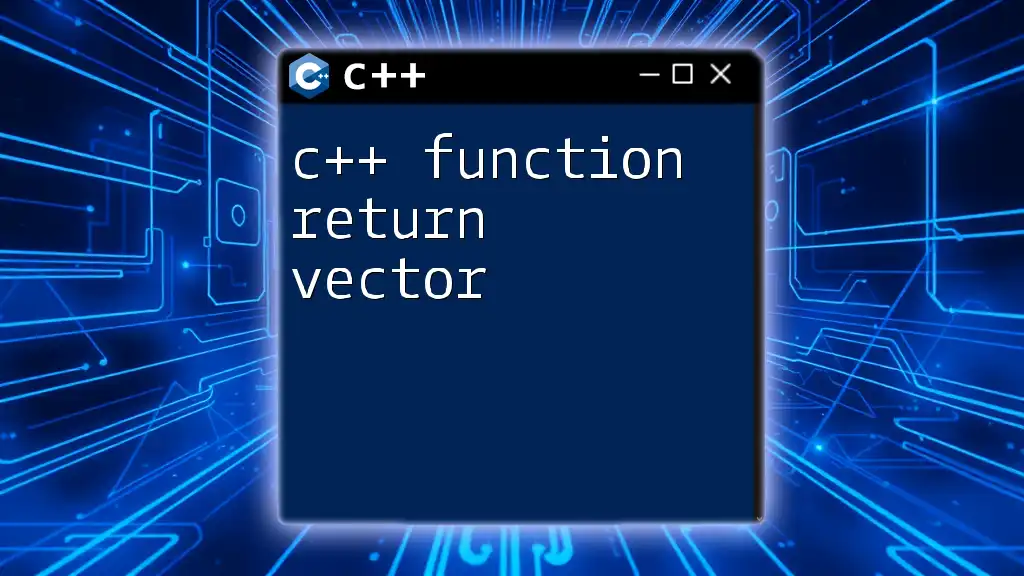
C++ Return Types
Basic Return Types
C++ supports various return types, commonly including primitive data types such as `int`, `float`, and `char`. Here’s an example of a function that returns an integer value:
int add(int a, int b) {
return a + b;
}
In this case, the `add` function takes two integers as arguments and returns their sum.
Complex Return Types
Beyond primitive types, C++ allows returning more sophisticated structures, including classes and structs. Here’s an example of a function returning a class object:
class Point {
public:
int x, y;
Point(int x, int y) : x(x), y(y) {}
};
Point createPoint(int x, int y) {
return Point(x, y);
}
In this example, `createPoint` creates a `Point` object and returns it, encapsulating both `x` and `y` values.
Functions with No Return Value
A function can also be defined to perform an action without returning any value, using the `void` keyword. Here’s an example:
void printHello() {
std::cout << "Hello, World!" << std::endl;
}
This function simply executes a command but does not return a value.
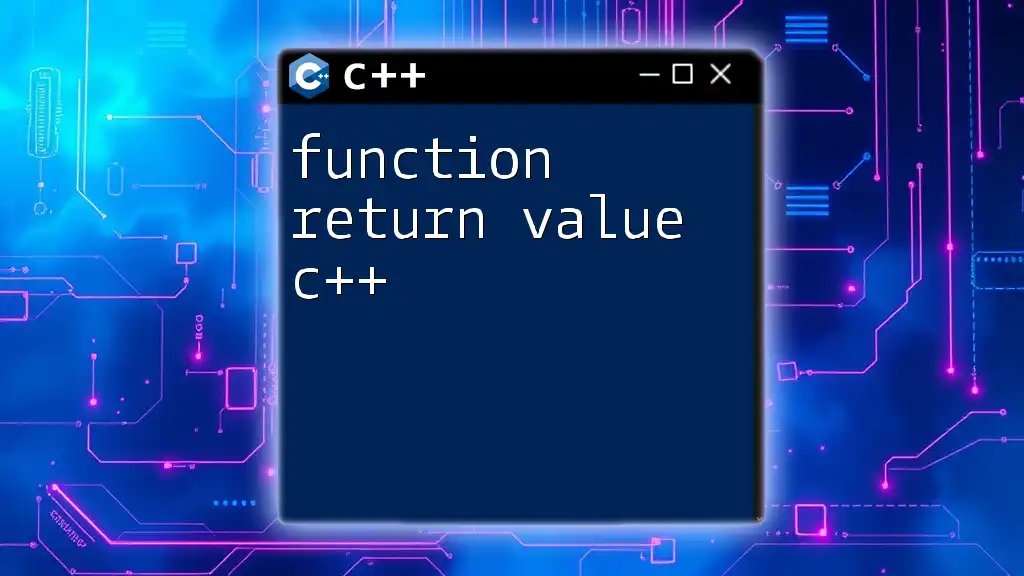
The Syntax of the Return Statement in C++
Basic Syntax
The basic syntax for the return statement in C++ is quite straightforward. To return a value, you simply use:
return value;
This simple structure allows you to indicate what the function is producing as its output.
Returning Expressions
You can also return the result of an expression directly. For instance:
double multiply(double a, double b) {
return a * b;
}
In this case, the function `multiply` returns the multiplication of two parameters without storing the result in a variable first.
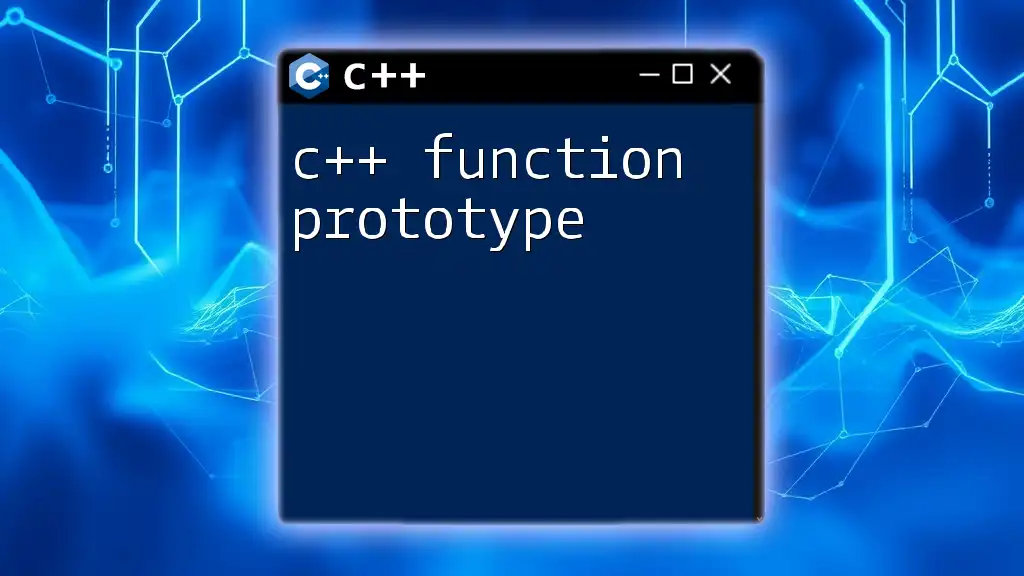
How to Handle Return Values
Using Returned Values
When you call a function that returns a value, you can assign that return value to a variable for further use. For example:
int result = add(5, 3);
std::cout << "Result: " << result << std::endl;
In this code snippet, the result of the `add` function call is stored in the `result` variable, and then printed.
Error Checking with Return Values
You can also use return values to indicate the success or failure of operations. For instance:
bool divide(double numerator, double denominator, double &result) {
if (denominator == 0) {
return false; // Return false for error
}
result = numerator / denominator;
return true; // Return true for success
}
In this example, the `divide` function checks for division by zero. It uses a `bool` return value to indicate whether the operation succeeded, while also providing the result through a reference parameter.
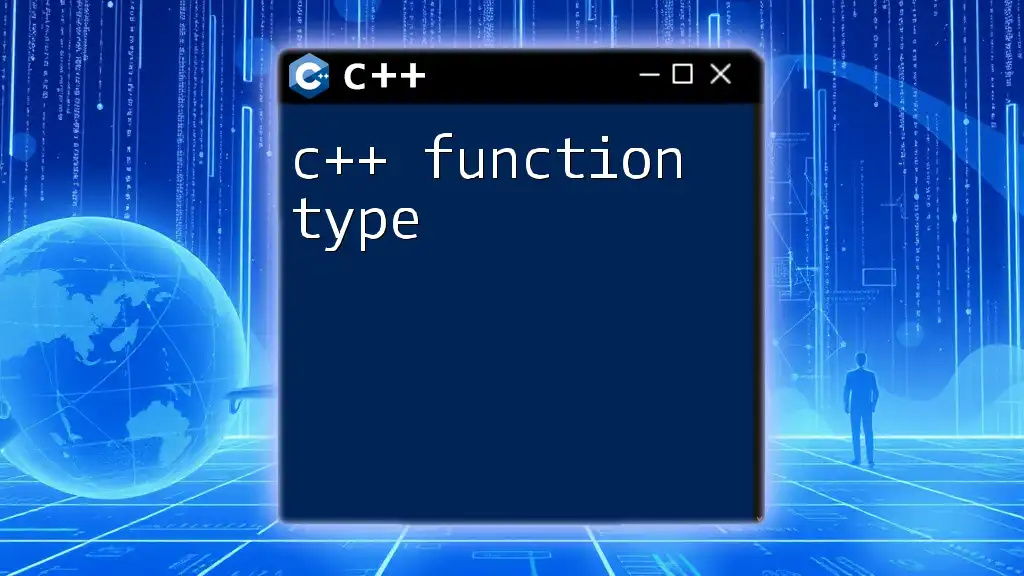
Best Practices for C++ Return Functions
Consistency in Return Types
It’s essential to choose return types that are appropriate for the tasks your functions perform. For instance, if a function performs a mathematical operation, it should return a numerical type. Being consistent with return types enhances readability and maintainability of code.
Use of `nullptr` and Special Values
In C++, you can also use `nullptr` to indicate that a pointer does not point to a valid location. Here’s an example:
int* getPointer() {
return nullptr; // Indicating no valid address
}
This can be useful for error handling, as it clearly shows that the function did not successfully return a valid pointer.
Performance Considerations
You should also consider performance when choosing to return by `value` versus `reference`. Returning by reference can enhance performance, especially for large objects, as it avoids copying them. Here’s an example:
const std::string& getGlobalValue() {
static std::string globalValue = "Hello";
return globalValue; // Return reference
}
In this case, `getGlobalValue` returns a reference to a static string, ensuring that the object remains valid while reducing overhead.
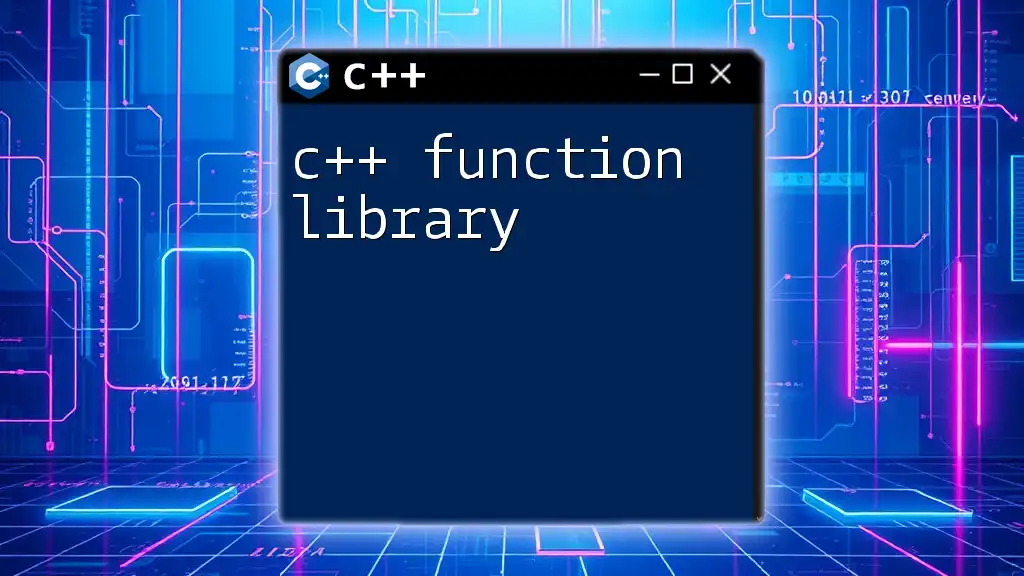
Conclusion
In summary, understanding the intricacies of the C++ function return is fundamental for effective programming. It underscores the significance of return values, illustrates best practices, and emphasizes the flexibility of types that can be returned. By mastering this concept, you can enhance code clarity and functionality, paving the way for more efficient C++ development. Consider practicing these techniques in your projects to solidify your understanding and elevate your programming skill set.