A C++ function prototype is a declaration of a function that specifies its name, return type, and parameters, allowing the function to be called before its definition.
int add(int a, int b); // Function prototype
Understanding Function Prototypes in C++
What is a Function Prototype?
A function prototype is a declaration of a function that specifies the function's name, return type, and parameters. It serves as a forward declaration to the compiler, letting it understand what to expect when the function is called later in the code.
Importance: Function prototypes are vital in C++ development as they enable better code organization and help catch errors at compile time, rather than at runtime. This organization is especially useful in larger projects where functions may be defined in separate files.
Syntax of a C++ Function Prototype
The syntax of a function prototype follows a specific structure. Here are the components that make up a prototype:
- Return Type: This indicates the type of value that the function will return. It could be `int`, `double`, `void`, etc.
- Function Name: A unique identifier for the function that should describe its purpose.
- Parameters: A list of input variables, including their types, that the function takes.
The general syntax for a function prototype is:
returnType functionName(parameterType1 parameter1, parameterType2 parameter2);
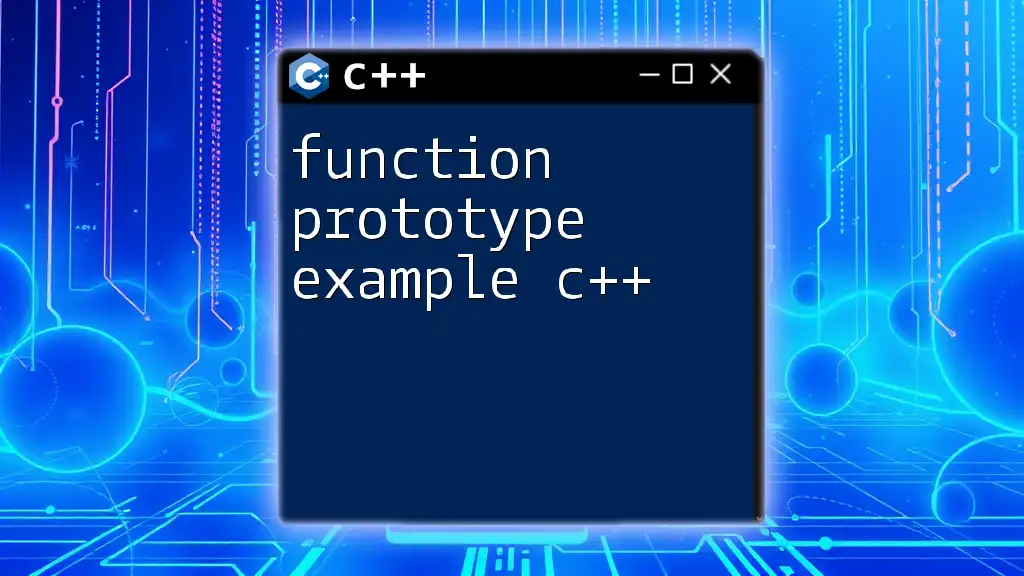
The Role of Function Prototypes in C++
Benefits of Using Function Prototypes
Using function prototypes comes with several significant advantages:
- Enhanced Readability: Prototypes serve as a roadmap for your code, making it easier to follow the program's logic without having to jump back and forth between function calls and definitions.
- Error Prevention: Prototypes help the compiler catch errors early. If there is a mismatch between the function call and its definition, the compiler will flag it.
- Flexibility in Code Organization: Prototypes allow you to separate the declaration and definition of functions. This is essential for maintaining clean and organized code, especially in larger projects.
When to Use Function Prototypes
Function prototypes are particularly useful in various scenarios, including:
- Multiple Function Definitions: When you define a function after its first call in the code, a prototype ensures that the compiler knows about it beforehand.
- Large Projects: In larger applications with many files, prototypes help modularize your code, improving maintainability and readability.
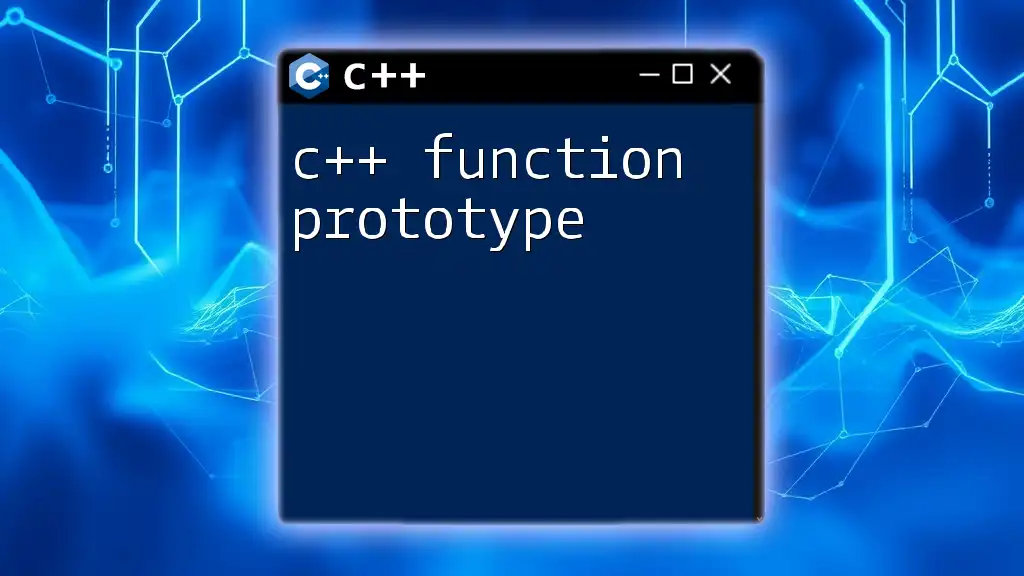
Creating a Function Prototype in C++
Basic Example of a Function Prototype
Let’s look at a straightforward example of a function prototype. Consider the following:
int add(int a, int b);
Here, `int` is the return type, `add` is the function name, and `int a, int b` are the parameters.
Example of a Complete Function with Prototype
Let’s see how a function prototype works in conjunction with a function definition. Below is a simple example:
#include<iostream>
using namespace std;
// Function Prototype
int add(int a, int b);
int main() {
cout << "Sum: " << add(5, 10) << endl;
return 0;
}
// Function Definition
int add(int a, int b) {
return a + b;
}
In this code:
- The prototype `int add(int a, int b);` declares the function before it is invoked in `main()`.
- The `main` function calls `add(5, 10)`, allowing the program to utilize the functionality of the `add` function.
- The definition of `add` subsequently provides the actual implementation, returning the sum of the parameters.
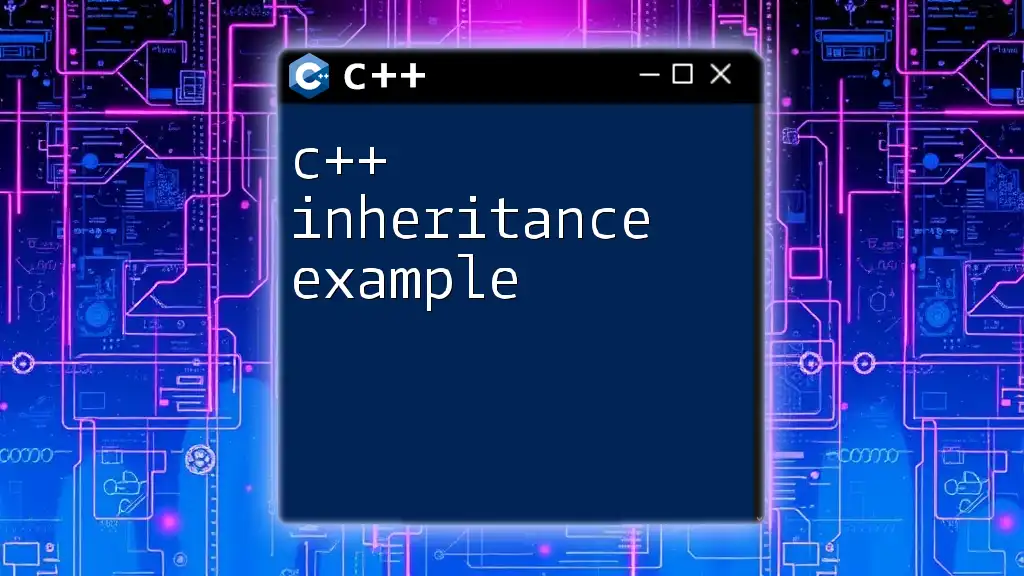
Advanced Concepts in Function Prototyping
Passing Different Types of Parameters
Function prototypes can handle various parameter types, including:
- Primitive Types: Such as `int`, `char`, `float`.
- Objects: Custom classes can also be passed as parameters.
- References and Pointers: Understanding how to pass by reference or by pointer can optimize performance and memory usage in C++.
Return Types
Defining the return type of a function prototype is crucial. If you mistakenly assign a void return type for a function that returns a value, it can lead to compilation errors or unexpected behavior. Examples of different return types include:
- `int`
- `double`
- `void` (function does not return a value)
Overloading Function Prototypes
Function overloading allows you to define multiple functions with the same name but different parameters, enhancing function usability. Here’s how you can overload function prototypes:
int multiply(int a, int b);
double multiply(double a, double b);
In this example, you have two `multiply` functions that differ based on input types. The compiler distinguishes between the two based on the type of arguments passed.
Inline Function Prototypes
Inline functions can improve performance by suggesting that the compiler insert the function's code directly at the point of call, avoiding the overhead of a function call. Here’s an example:
inline int square(int x) {
return x * x;
}
Using inline keywords is beneficial for small, frequently called functions.
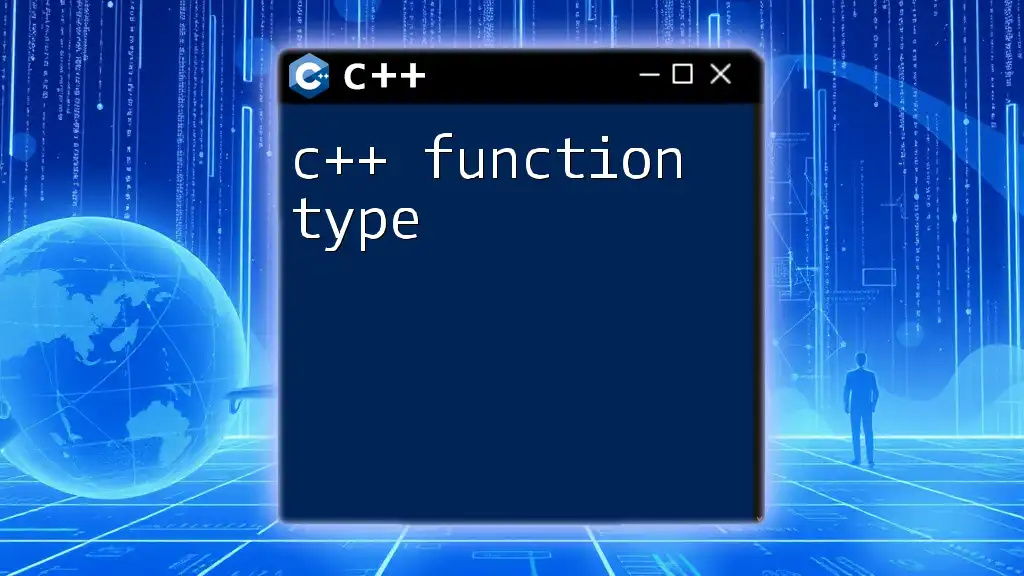
Best Practices for Using Function Prototypes
Consistency in Naming Conventions
Consistency in naming your prototypes is paramount. Select clear and descriptive names that convey the function's purpose, as this will drastically improve readability and maintainability of your code.
Keeping Prototypes Organized
Organizing your function prototypes makes your code cleaner and easier to navigate. Consider grouping related functions together and using header files for declarations in larger projects.
Avoiding Common Errors
Several common mistakes can occur when defining function prototypes. Here are a few to watch out for:
- Misaligned Types: Ensure that the types in your function calls match those in your function prototype.
- Omitting the Return Type: Always specify the return type; a missing return type results in a compilation error.
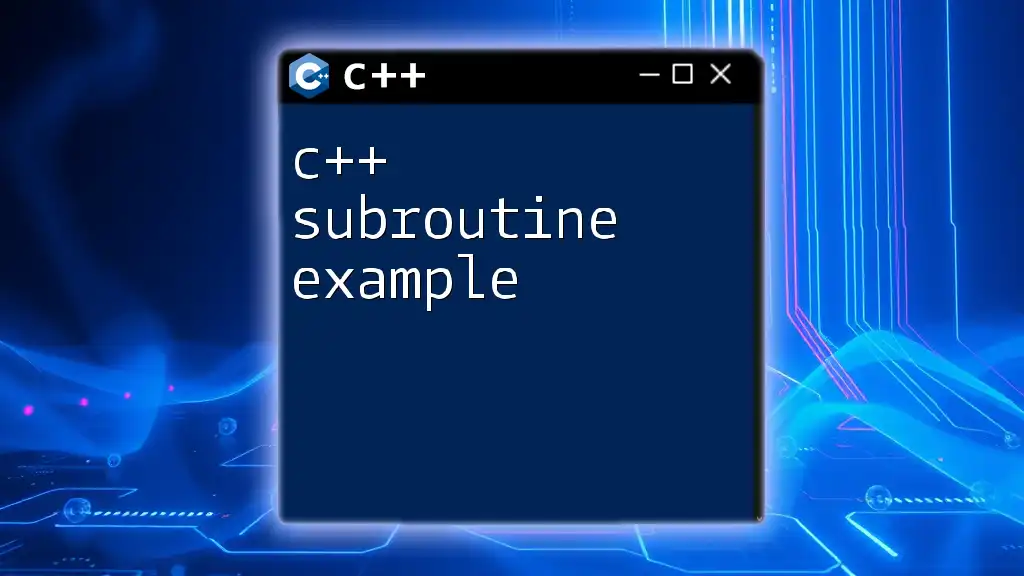
Conclusion
Recap of Function Prototypes
Function prototypes serve as an essential building block of C++ programming. They enhance code organization, prevent errors, and increase readability.
Encouragement to Practice
As you continue your journey of learning C++, don’t hesitate to practice writing your own function prototypes. Experimentation will deepen your understanding.
Additional Resources
Refer to additional C++ textbooks or online resources for exercises and further reading on function prototyping to solidify your skills.
Call to Action
Ready to master C++? Sign up for our classes or tutorials to enhance your skills, with a focus on hands-on learning that will make concepts like function prototypes second nature to you.