In C++, `std::cout` is used to output data to the standard output stream, typically the console, and here's a simple example demonstrating its usage:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is stdout?
`stdout`, short for standard output, is a predefined output stream in C++ that displays output data to the terminal or console. It plays a crucial role in programming, serving as a universal means of communicating results back to the user. Understanding how to leverage `stdout` effectively allows developers to present information succinctly and accurately, making it an essential concept for any C++ programmer.
While `stdout` is commonly used, it’s important to recognize its relationship with other output streams such as `stderr` (standard error) and `stdin` (standard input). The former is used for error messages, while the latter is utilized for receiving input data from users.
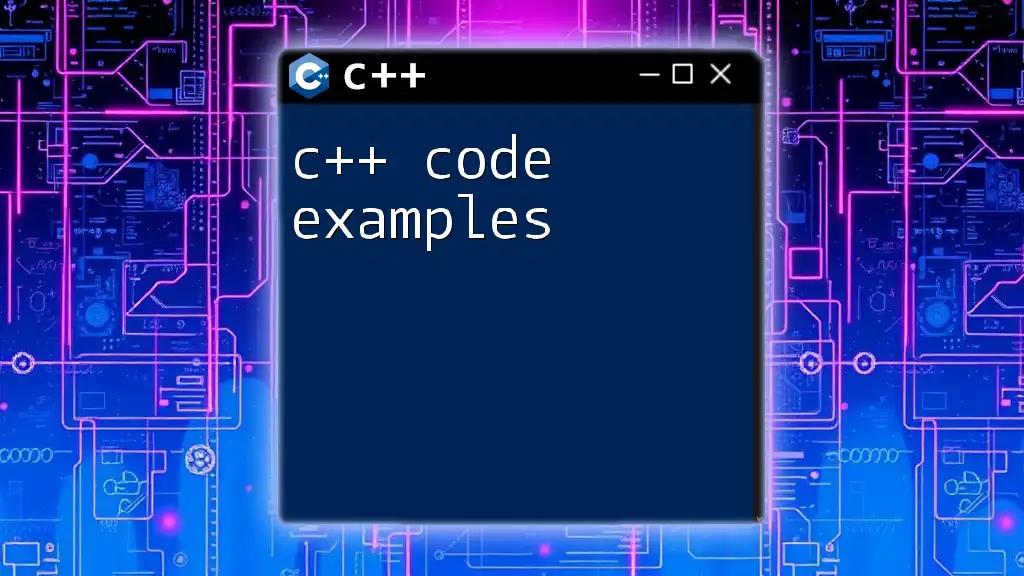
The Basics of Output in C++
Using the `<iostream>` Library
To work with output in C++, you will typically include the `<iostream>` library in your program, which provides the necessary functionality to utilize predefined output streams.
Here’s how to include the library in your code:
#include <iostream>
By adding this line at the beginning of your source file, you gain access to built-in objects like `std::cout`, which makes outputting data straightforward.
Understanding the `std::cout` Object
`std::cout` is the primary output stream used in C++. It allows you to send data to `stdout`. The `std` namespace encompasses standard functions and objects, including `std::cout`, which means you’ll typically either use it with the namespace identifier or include `using namespace std;` to eliminate the need for the `std::` prefix.
To effectively demonstrate how to use `std::cout`, here’s a simple example:
std::cout << "Hello, World!" << std::endl;
This line prints the text "Hello, World!" to the console, followed by a line break thanks to `std::endl`. This manipulation is vital as it keeps the output organized and readable.
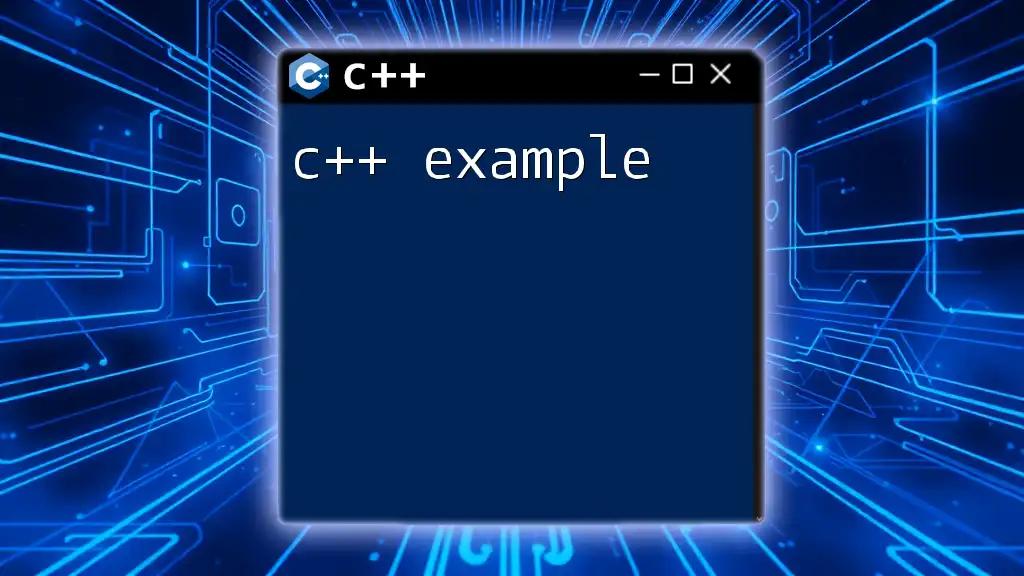
Formatting Output with `std::cout`
Combining Text and Variables
One of the strengths of `std::cout` is its ability to combine strings and variables seamlessly. This feature allows you to create dynamic output messages that can include user data, calculations, and more.
For example, consider the following code snippet that incorporates an integer variable into a string:
int age = 25;
std::cout << "I am " << age << " years old." << std::endl;
In this case, the output will read "I am 25 years old." This versatility enhances the user experience, as it enables you to provide real-time information.
Manipulating Output with Format Flags
C++ provides several manipulators that allow you to format your output, including control over decimal precision and scientific notation. This can be particularly useful when dealing with floating-point numbers.
For instance, if you want to control the decimal places shown in your output, you could use the `std::fixed` and `std::setprecision` manipulators:
#include <iomanip>
double pi = 3.14159;
std::cout << std::fixed << std::setprecision(2) << pi << std::endl;
In this example, the output will display "3.14", rounding pi to two decimal places. Using these manipulators can make your output cleaner and more professional.
Using Line Breaks and Other Special Characters
To enhance the formatting of your output, you can also utilize line breaks and special characters. In your code, you can include line breaks simply by using `\n`:
std::cout << "First Line\nSecond Line" << std::endl;
This snippet prints "First Line" and "Second Line" on separate lines, thereby improving the readability of your output.
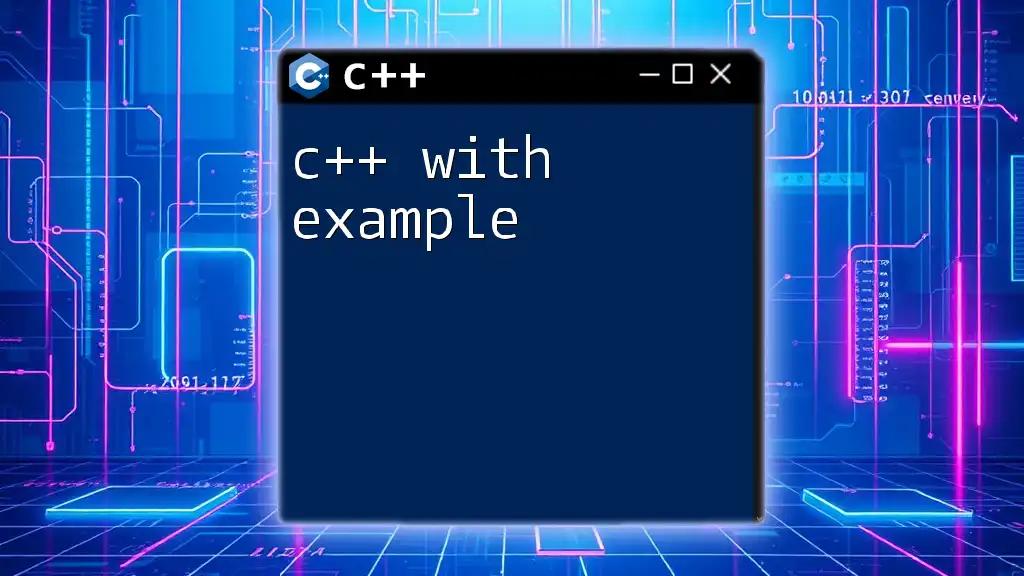
Redirecting stdout
What is Redirection?
Redirection is a powerful feature that allows you to redirect the standard output of your program to various destinations, such as files or other output streams. This capability is particularly handy during debugging or logging processes, as it can help track program behavior without cluttering the console.
Typically, you would perform redirection from the command line. For instance:
./your_program > output.txt
This command runs `your_program`, directing all output that would usually go to `stdout` into `output.txt`.
Redirection in Code
You can also redirect `stdout` directly in your C++ code. Let's say you want to redirect output to a file programmatically. Here’s an example:
#include <fstream>
std::ofstream out("output.txt");
std::streambuf* coutbuf = std::cout.rdbuf(); // save old buf
std::cout.rdbuf(out.rdbuf()); // redirect std::cout to out.txt
std::cout << "This will be written to a file!" << std::endl;
std::cout.rdbuf(coutbuf); // reset to standard output
In this code, the output "This will be written to a file!" goes into `output.txt`, demonstrating how to manage output redirection within the code directly.

Common Errors with stdout
Misusing Output Streams
Like any programming feature, developers can mismanage `stdout`. A common mistake is forgetting to flush the output buffer. When you do not flush the buffer explicitly, you may not see your intended output immediately, which can lead to confusion.
Error Handling
Effective error handling when using `stdout` is crucial. To check for errors during output operations, you can utilize `std::cerr`, which outputs error messages to the console. Employing this can help diagnose issues rapidly:
if (std::cout.fail()) {
std::cerr << "Output error occurred!" << std::endl;
}
This practice ensures that your application can handle conditions gracefully and provide informative feedback to users.

Advanced C++ Output Techniques
Creating Custom Output Functions
To facilitate reusability and maintain code clarity, consider encapsulating `std::cout` within custom functions. This approach allows you to standardize output across your application.
For instance, you can create a simple function to format and print messages:
void printMessage(const std::string& message) {
std::cout << "Message: " << message << std::endl;
}
Using this function improves readability and ensures that all messages follow a consistent format.
Understanding Buffering
Buffering determines how and when data is actually output to the console. Understanding buffering can help you optimize performance and debugging. In many cases, `std::cout` employs an output buffer that stores data temporarily. Only when the buffer is full or explicitly flushed does the output appear.
Consider the following snippet, which demonstrates when output actually shows up:
std::cout << "Buffered output";
// Output may not appear until the buffer is flushed
This behavior can lead to puzzling cases where anticipated output seems missing. Ensuring you understand how and when buffers flush can save time during debugging.

Conclusion
Understanding and utilizing `c++ stdout example` is fundamental for delivering clear, accessible output in your programs. Mastering `std::cout`, its format manipulators, and redirection techniques significantly enhances your ability to communicate with users. As you explore the intricacies of output in C++, remember that experimentation is vital. Embrace the complexity, try different methods, and don’t hesitate to share your experiences in the comments section!
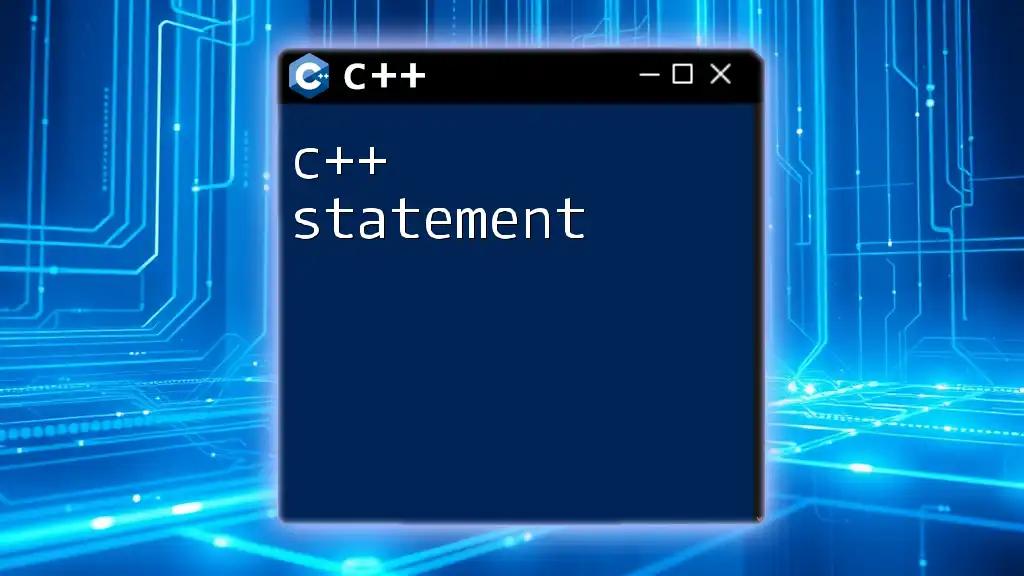
Additional Resources
For those eager to learn more about C++ and output techniques, consider exploring official C++ documentation or enrolling in courses that dive deeper into this essential programming language. Joining community forums can also be a valuable way to engage with peers and exchange knowledge!