The C++ `std::vector` is a dynamic array that can resize itself automatically when elements are added or removed, allowing for efficient management of collections of data.
Here’s a simple example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
numbers.push_back(6); // Adding an element to the vector
for(int num : numbers) {
std::cout << num << " "; // Output: 1 2 3 4 5 6
}
return 0;
}
Understanding std::vector
Characteristics of std::vector
Dynamic Size A `std::vector` can grow and shrink dynamically as elements are added or removed. Unlike arrays, which have a fixed size, vectors automatically manage memory allocation as needed. This makes them an essential tool in C++ for programming where the number of elements is not known at compile time.
Performance Vectors are often more efficient than arrays, especially when it involves resizing or frequent additions/removals of elements. However, it's important to note that when a vector exceeds its current capacity, it reallocates memory, which can temporarily slow down performance. Understanding how a vector's capacity grows is key to leveraging its strengths.
Memory Management With `std::vector`, memory management is mostly automatic. When vectors go out of scope, their destructors ensure that allocated memory is released. This reduces the risk of memory leaks, which are a common issue when using raw pointers and dynamic memory management.
Basic Syntax and Declaration
To use `std::vector`, you first need to include the appropriate header:
#include <vector>
You can declare a vector for any data type, including custom objects, as follows:
std::vector<int> myVector;
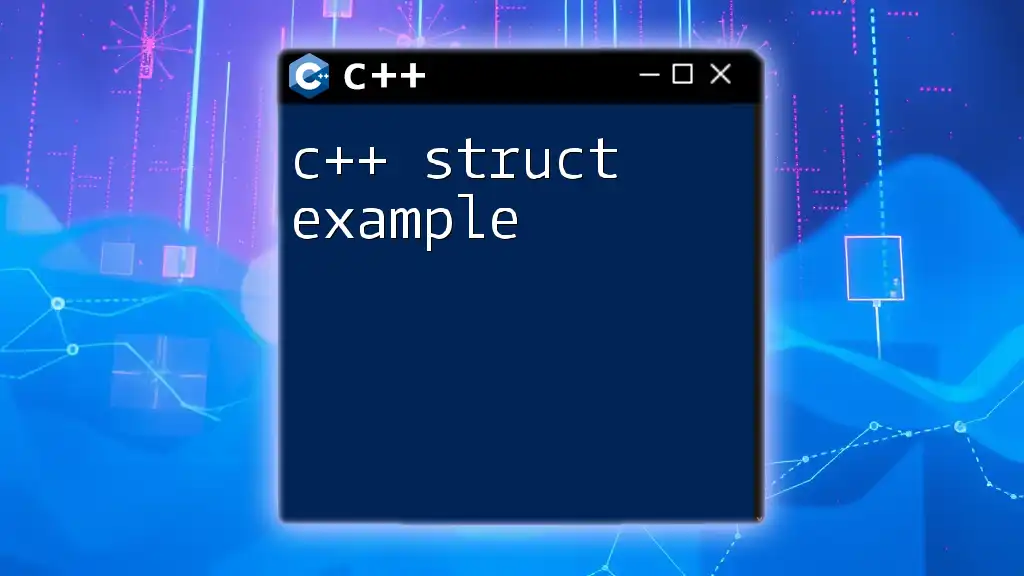
Creating and Initializing std::vector
Default Initialization
To create an empty vector, simply declare it without any parameters. For example:
std::vector<int> vec;
Initialization with Values
You can also initialize a vector with predefined values at declaration:
std::vector<int> vec = {1, 2, 3, 4, 5};
This initializes `vec` with five integer elements.
Using the Resize Function
If you want to change the size of the vector after its declaration, you can use the resize function:
vec.resize(10); // Resize vector to hold 10 elements
If you resize a vector to a larger size than it currently holds, new elements will be default-initialized.
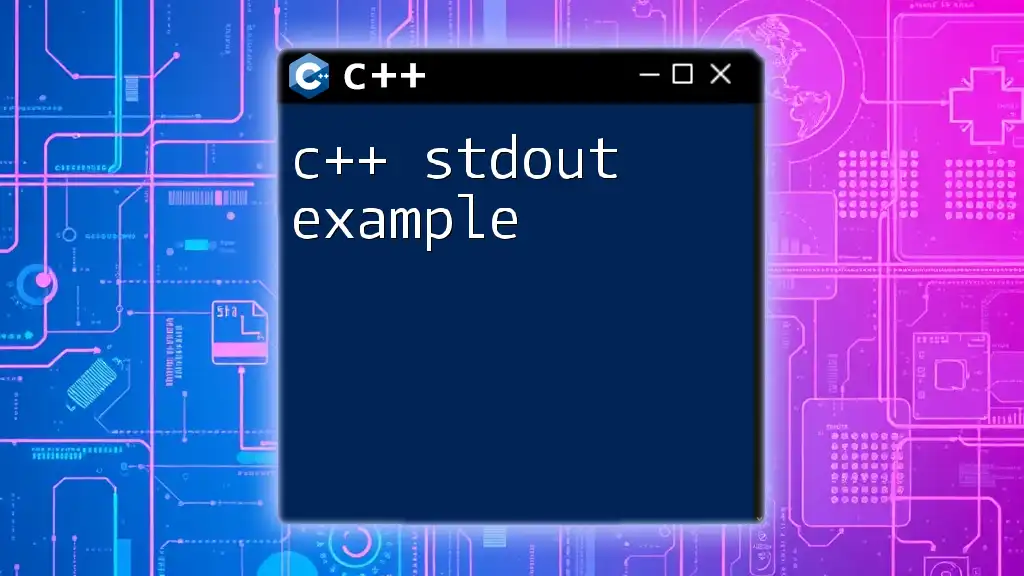
Accessing Elements in std::vector
Using the at() Method
The `at()` method provides a safe way to access elements. It checks boundaries and throws an exception if the index is out of range:
int value = vec.at(2);
Using the Operator[]
Directly accessing elements can be done with the `[]` operator, but be cautious, as this doesn't check bounds:
int value = vec[2];
Iterating Over a Vector
One of the most straightforward ways to iterate through a vector is using a range-based for loop:
for (auto val : vec) {
std::cout << val << " ";
}
This approach simplifies reading the contents of the vector without needing to manage indices manually.
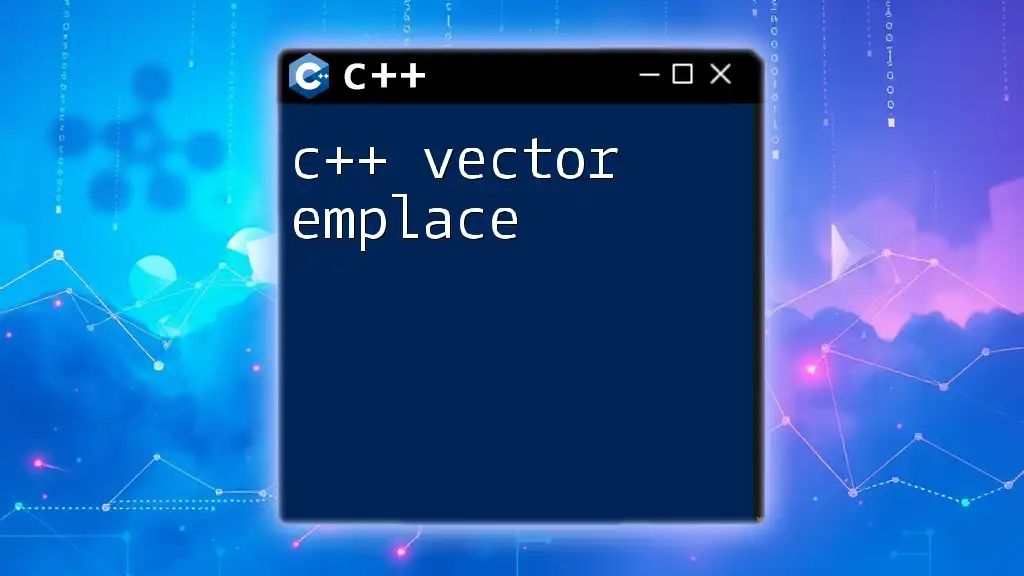
Modifying std::vector
Adding Elements
Using push_back()
To add an element at the end of the vector, use the `push_back()` method:
vec.push_back(6); // Adds 6 to the end of the vector
This method ensures that the vector will grow as needed to accommodate additional elements.
Using emplace_back()
The `emplace_back()` function constructs an element in place at the end of the vector, which is often more efficient:
vec.emplace_back(7); // Constructs 7 at the end of the vector
Inserting Elements
You can insert an element at a specific position using the `insert()` function:
vec.insert(vec.begin() + 2, 99); // Insert 99 at index 2
This allows for greater flexibility in managing the order of elements within the vector.
Removing Elements
Using pop_back()
To remove the last element from the vector, you can use `pop_back()`:
vec.pop_back(); // Removes the last element
Using erase()
If you need to remove an element from a specific index, use `erase()`:
vec.erase(vec.begin() + 1); // Removes element at index 1
This function also accommodates the removal of a range of elements by providing two iterators.
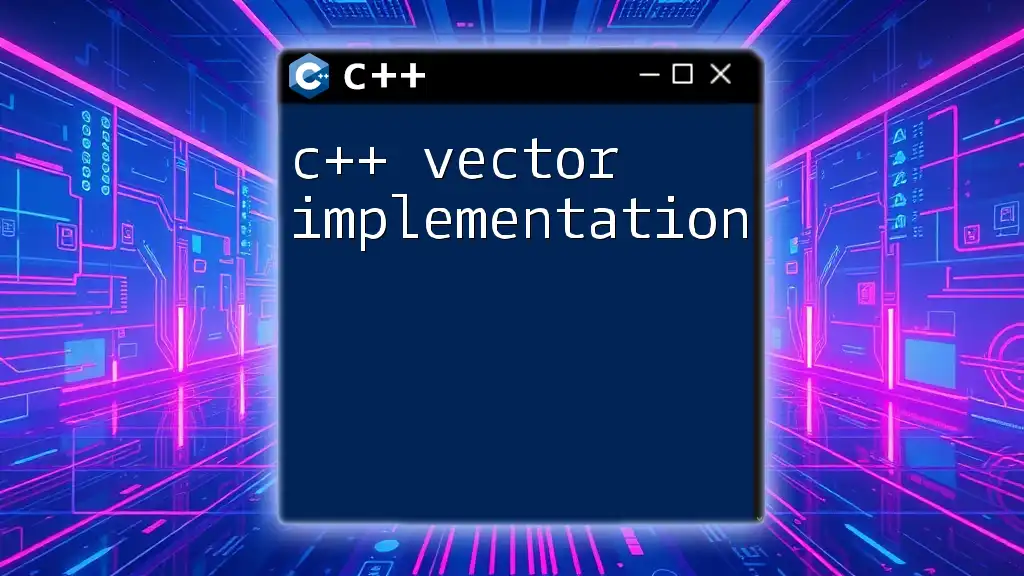
Advanced Usage of std::vector
Sorting a Vector
You can easily sort a vector using the `sort()` function from the `<algorithm>` header:
#include <algorithm>
// Sort the vector in ascending order
std::sort(vec.begin(), vec.end());
Searching Elements
To find an element in a vector, use `std::find`:
#include <algorithm>
auto it = std::find(vec.begin(), vec.end(), 3);
if (it != vec.end()) {
std::cout << "Element found!" << std::endl;
}
Capacity Management
Understanding the difference between the size and capacity of a vector is crucial. The `size()` method returns the number of elements currently in the vector, while `capacity()` returns the total number of elements the vector can hold before it needs to resize:
std::cout << "Size: " << vec.size() << std::endl;
std::cout << "Capacity: " << vec.capacity() << std::endl;
If you want to allocate memory in advance, use the `reserve()` function:
vec.reserve(100); // Reserve space for 100 elements
By doing this, you minimize reallocations when you know the expected size of the vector upfront.
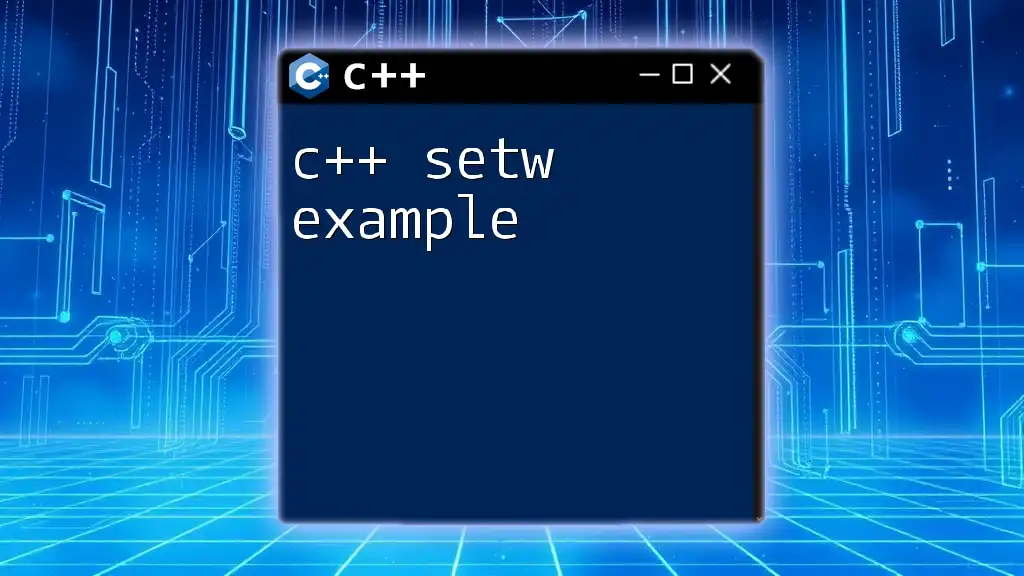
Common Pitfalls to Avoid
Exceptions
One of the common mistakes when using std::vector is trying to access an index that is out of bounds. The use of `at()` mitigates this risk since it throws an `std::out_of_range` exception.
Performance Issues
Avoiding Unnecessary Copies When passing vectors to functions, consider passing them by reference to avoid costly copy operations. Use `std::move` when necessary to transfer ownership without copying.
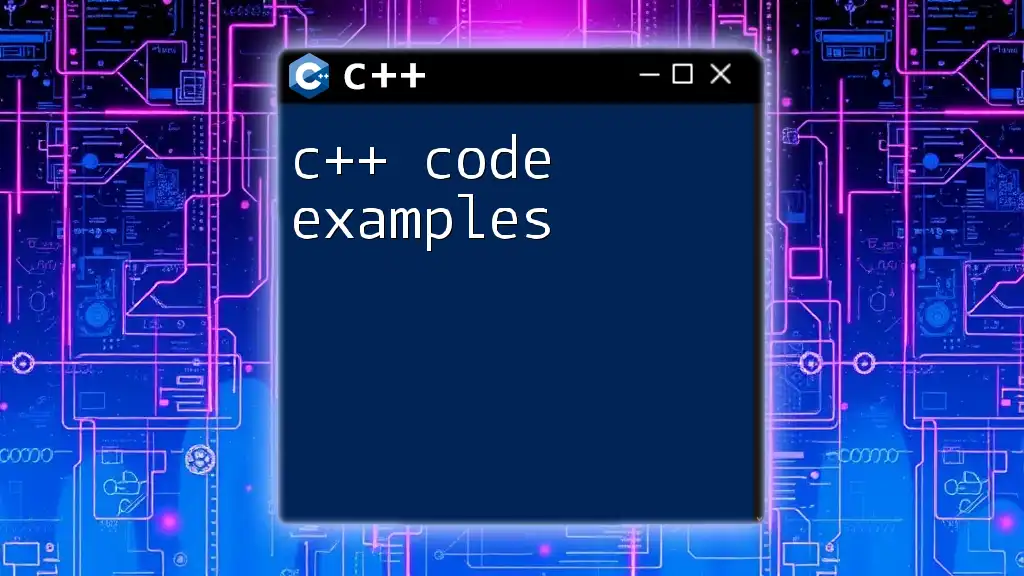
Conclusion
In summary, `std::vector` provides an incredibly powerful and flexible way to manage collections of data in C++. Its dynamic sizing, ease of use, and built-in functionality make it a favorite among developers.
Consider practicing with different vector operations—such as initializing, accessing, and modifying—to solidify your understanding. The knowledge of vectors is essential for efficient C++ programming, so take advantage of online resources and communities to enhance your skills.
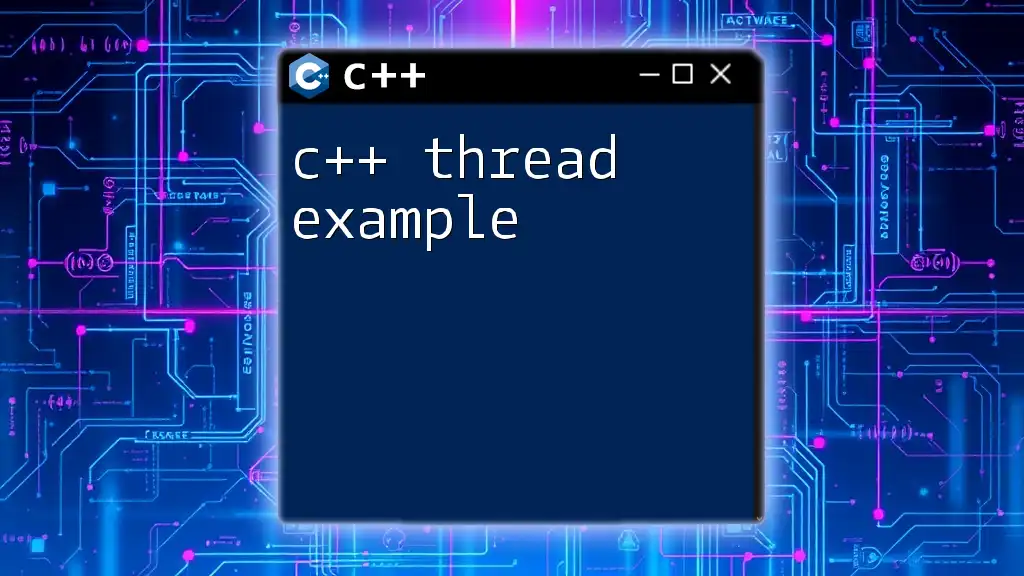
Call to Action
Join our C++ learning community to dive deeper into programming concepts and stay updated on the latest tutorials. Whether you're a beginner or looking to sharpen your skills, there's something for everyone!
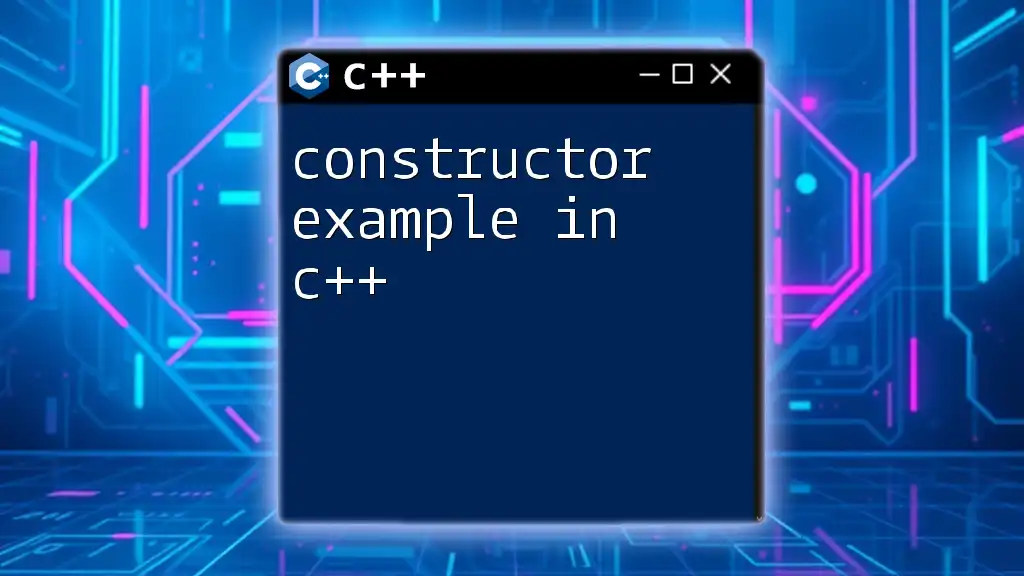
FAQs about std::vector
What is the difference between std::vector and std::array?
`std::vector` offers dynamic sizing and memory management, while `std::array` has a fixed size determined at compile time. Choose `std::vector` for flexibility and `std::array` for scenarios where the size remains constant.
When should I prefer std::vector over other containers?
Use `std::vector` when you need a dynamic array with quick access times and frequently changing sizes. For scenarios that require fast inserts and deletes, consider `std::list`; for associative key-value pairs, `std::map` or `std::unordered_map` may be more suitable.