`std::thread` in C++ is a class that allows you to create and manage threads for concurrent execution of tasks.
Here’s a simple example of how to use `std::thread`:
#include <iostream>
#include <thread>
void hello() {
std::cout << "Hello from thread!" << std::endl;
}
int main() {
std::thread t(hello); // Create a thread that runs the hello function
t.join(); // Wait for the thread to finish
return 0;
}
Understanding Multithreading
What is Multithreading?
Multithreading is a programming technique that allows multiple threads to execute concurrently within a single process. Threads are lightweight processes, and they share the same memory space, which makes context switching between them faster than between traditional processes.
Advantages of Multithreading:
- Improves application performance: By utilizing multiple CPU cores effectively, applications can run faster and handle more tasks simultaneously.
- Better resource utilization: Threads can help keep processors busy by not idling during I/O operations.
Disadvantages of Multithreading:
- Complexity: Multithreading introduces challenges, such as race conditions and deadlocks, that require careful coding.
- Debugging difficulties: Tracking issues in multithreaded applications can be more complicated than in single-threaded applications.
The Need for Parallelism in Modern Applications
In today’s software development landscape, applications ranging from gaming to web servers benefit significantly from multithreading. For instance, web servers that handle multiple requests concurrently can significantly enhance their response time and throughput. Video games perform various tasks simultaneously, such as rendering graphics and processing user inputs, which leads to smoother gameplay experiences. By leveraging multithreading, developers can create more responsive and efficient applications that capitalize on the full capabilities of modern processors.
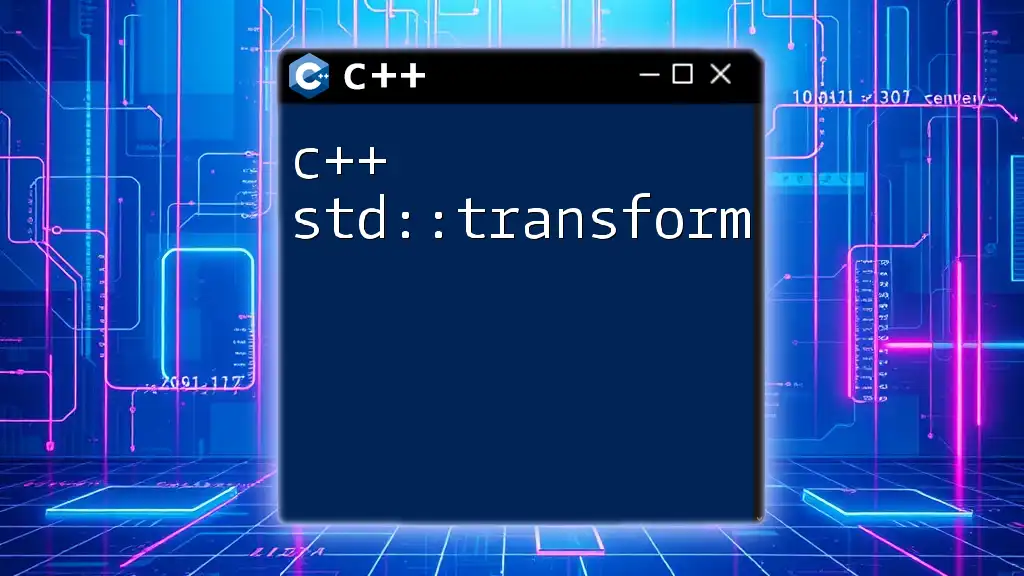
Introduction to std::thread
What is std::thread?
`std::thread` is a C++ class, introduced in the C++11 standard, that simplifies the creation and management of threads. It encapsulates a thread of execution and provides various methods to manage thread behavior. Unlike the traditional operating system threads, `std::thread` is easier to use and integrates smoothly into C++ projects.
Basic Concepts of Threads
A thread in C++ is a sequence of instructions that can run independently from the main execution thread. When a new thread is created using `std::thread`, it can run a function or a callable object in parallel with other threads and the main program.
Threads have a lifecycle defined by several states: creation, execution, and termination. Understanding this lifecycle is crucial for effective thread management and ensures that resources are released appropriately.
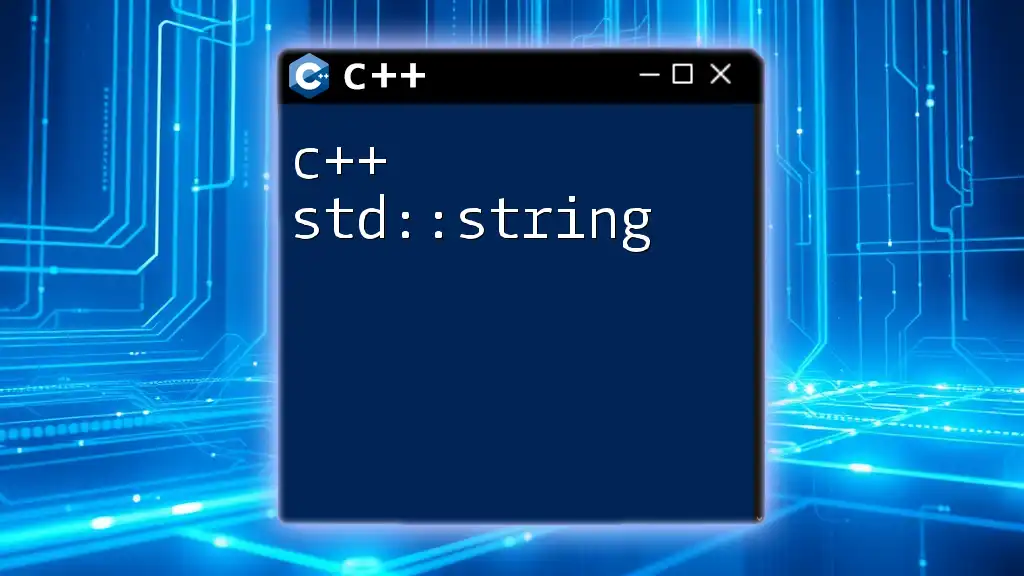
Creating and Managing Threads
How to Create a Thread
Creating a thread using `std::thread` is straightforward. You simply instantiate a `std::thread` object, passing the function that the thread will run as an argument:
#include <iostream>
#include <thread>
void function() {
std::cout << "Hello from a thread!" << std::endl;
}
int main() {
std::thread t(function);
t.join(); // Wait for the thread to finish
return 0;
}
In this example, the `function` is executed in its own thread, and the `join()` function ensures that the main program waits for the thread to finish before continuing.
Joining and Detaching Threads
Managing the thread's lifecycle is crucial. The `join()` method blocks the calling thread until the thread whose `join()` method has been called completes its execution. Here's an example:
void worker() {
std::cout << "Worker thread executing." << std::endl;
}
int main() {
std::thread t(worker);
t.join();
std::cout << "Worker thread completed." << std::endl;
return 0;
}
Conversely, the `detach()` method allows a thread to run independently. After detaching, you can no longer control that thread. Here’s how you can use detach:
void detachedFunction() {
// Some operations
}
int main() {
std::thread t(detachedFunction);
t.detach(); // Allow the thread to run independently
// No longer able to join t.
return 0;
}
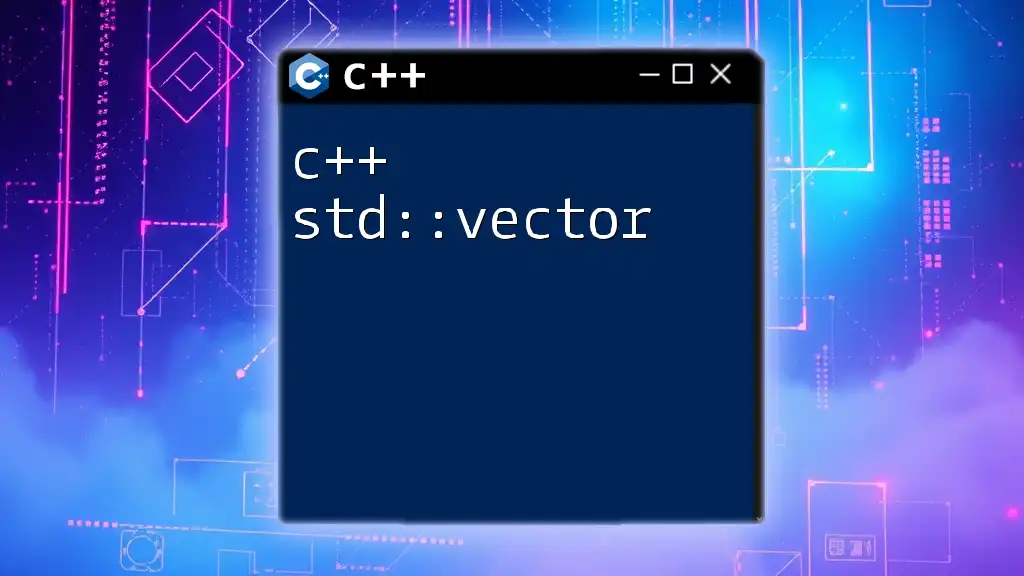
Passing Arguments to Threads
Using Standard Function Objects
When you create a thread, you can pass arguments to the function it runs. This can be done straightforwardly:
#include <iostream>
#include <thread>
void printSum(int a, int b) {
std::cout << "Sum: " << a + b << std::endl;
}
int main() {
std::thread t(printSum, 5, 10); // Passing arguments
t.join();
return 0;
}
In this example, two arguments (5 and 10) are passed to the `printSum` function when the thread is created.
Using Lambda Expressions
Lambda expressions provide a concise way to define the function to run in a new thread, and they can easily capture variables from the surrounding scope. Here's an example:
int main() {
std::thread t([](int a, int b) {
std::cout << "Lambda Sum: " << a + b << std::endl;
}, 7, 3);
t.join();
return 0;
}
In this case, a lambda function is defined and executed in a thread, and it computes the sum of the two numbers passed to it.
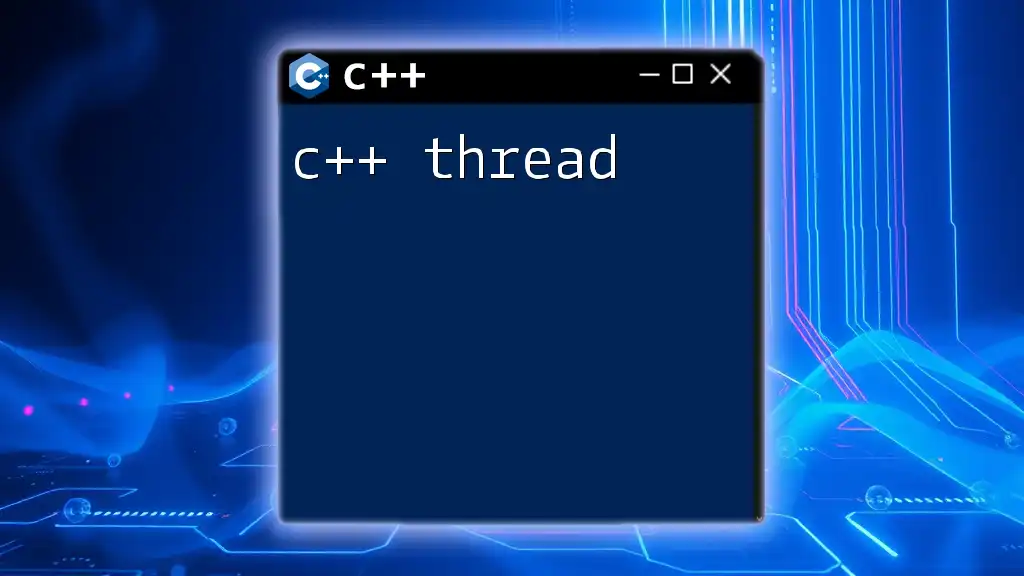
Thread Safety and Synchronization
The Importance of Thread Safety
When multiple threads access shared data, it can lead to race conditions where the result of operations depends on the sequence or timing of uncontrollable events. Ensuring thread safety is essential in these scenarios to maintain data integrity.
Synchronization Mechanisms
To handle shared data safely, C++ provides synchronization mechanisms like mutexes. A mutex (short for mutual exclusion) prevents multiple threads from accessing shared data simultaneously. Here's a simple example using `std::mutex`:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx; // Mutex for critical section
void safePrint(int id) {
std::lock_guard<std::mutex> lock(mtx);
std::cout << "Thread ID: " << id << std::endl;
}
int main() {
std::thread t1(safePrint, 1);
std::thread t2(safePrint, 2);
t1.join();
t2.join();
return 0;
}
In this snippet, two threads are created, and they both attempt to access the `safePrint` function. The mutex ensures that the output from one thread does not interfere with the output from another, maintaining thread safety.
Condition Variables
Condition variables are essential for signaling between threads, allowing threads to wait for certain conditions to be met. They work in conjunction with mutexes to protect shared data while threads are in a waiting state. A simple implementation of `std::condition_variable` can allow one thread to signal another when it can proceed.
#include <iostream>
#include <thread>
#include <condition_variable>
std::condition_variable cv;
std::mutex mtx;
bool ready = false;
void waitForSignal() {
std::unique_lock<std::mutex> lock(mtx);
cv.wait(lock, [] { return ready; }); // Wait until ready is true
std::cout << "Thread received signal!" << std::endl;
}
int main() {
std::thread t(waitForSignal);
std::this_thread::sleep_for(std::chrono::seconds(1)); // Simulate work
{
std::lock_guard<std::mutex> lock(mtx);
ready = true; // Set condition
}
cv.notify_one(); // Send signal to waiting thread
t.join();
return 0;
}
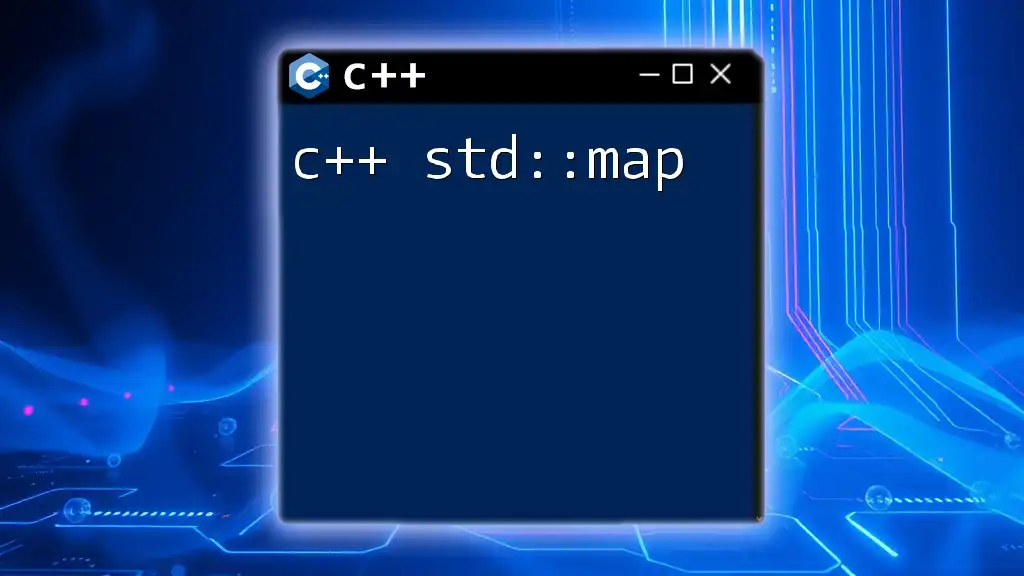
Best Practices for Using std::thread
Avoiding Common Pitfalls
Understanding thread lifetimes is crucial. Make sure that threads do not outlive the data they access. Passing pointers or references to threads can be dangerous if the data they depend on is out of scope. Use smart pointers when managing shared resources to help alleviate potential issues.
Performance Considerations
While multithreading can enhance application performance, it’s important to evaluate overhead versus benefits. In some scenarios, the cost of managing threads may outweigh the performance gains. Always test and profile applications to determine if adding threads is beneficial.
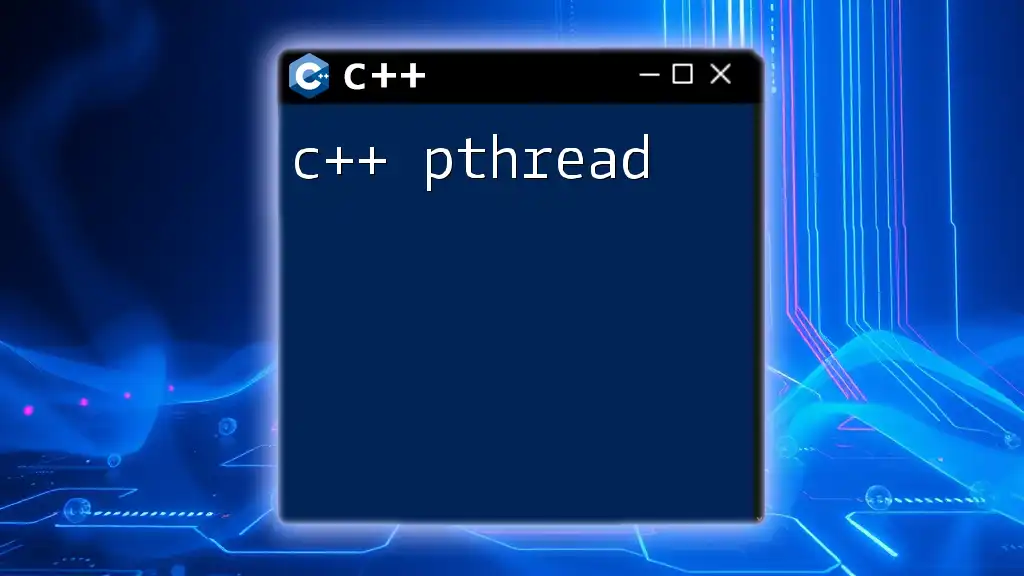
Conclusion
In conclusion, `std::thread` is a powerful tool in C++ that provides both simplicity and flexibility in implementing multithreading. Understanding its features, advantages, and proper usage is essential for developing high-performance applications. By embracing multithreading, you can enhance responsiveness and efficiency in your programs, driving better performance in today’s competitive software landscape.
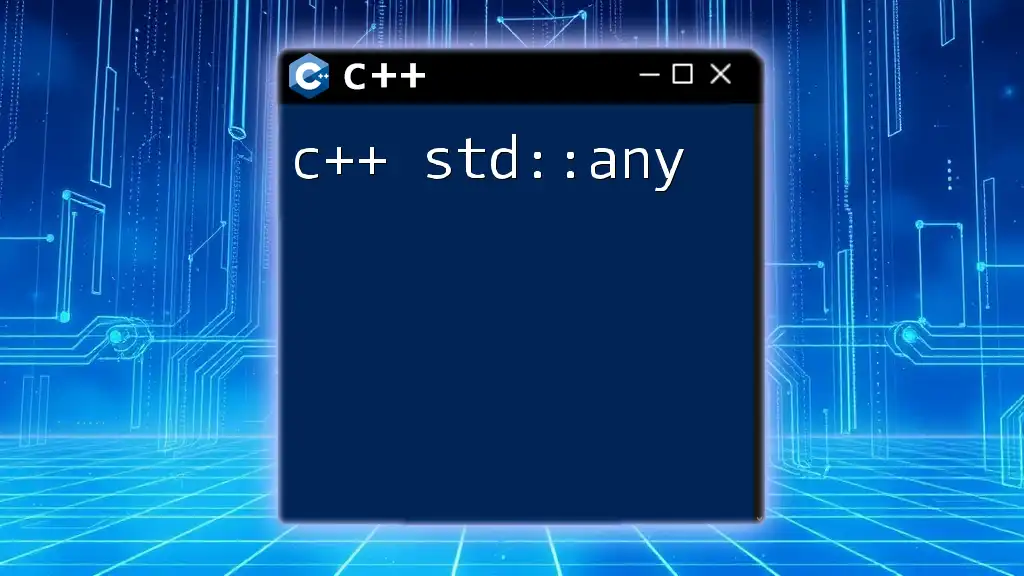
Additional Resources
For more information on the C++ standard, refer to the [official C++ documentation](https://en.cppreference.com/w/cpp/thread). For an in-depth understanding of multithreading concepts, consider exploring books like C++ Concurrency in Action by Anthony Williams or online courses from reputable platforms.
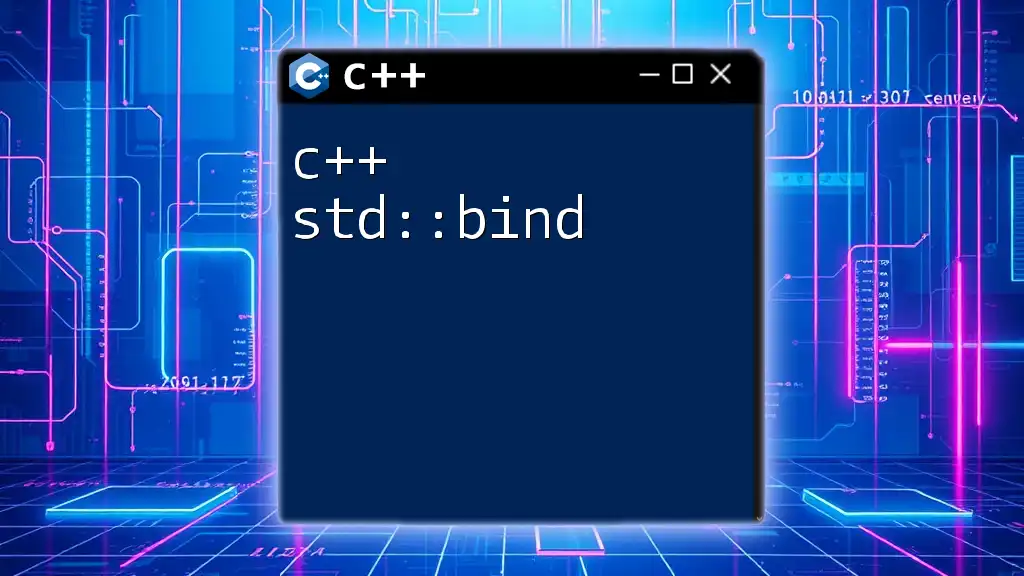
Call to Action
Have you used `std::thread` in your programming projects? What challenges did you face? Share your experiences, insights, or questions in the comments below! Your feedback helps foster a community of learners eager to improve their skills in C++.