In C++, the `thread::join` function is used to block the calling thread until the thread identified by the `std::thread` object terminates, ensuring proper synchronization of threads.
Here's a simple code snippet demonstrating its usage:
#include <iostream>
#include <thread>
void threadFunction() {
std::cout << "Thread is running!\n";
}
int main() {
std::thread t(threadFunction); // Start a new thread
t.join(); // Wait for the thread to finish
std::cout << "Thread has finished!\n";
return 0;
}
What is Thread Join?
The term C++ thread join refers to a technique used for synchronizing the execution of threads. When we create threads in C++, they run concurrently, and the main program can proceed without waiting for them to finish. However, in certain situations, we want to ensure that a thread has completed its execution before moving on to the next task. This is where the `join()` function comes into play.
How Thread Join Works
The `join()` function is called on a thread object, and it effectively blocks the calling thread until the thread associated with the `std::thread` object has finished executing. This process ensures that resources used by the thread are cleaned up appropriately and that any results produced can be utilized only after the thread’s completion.
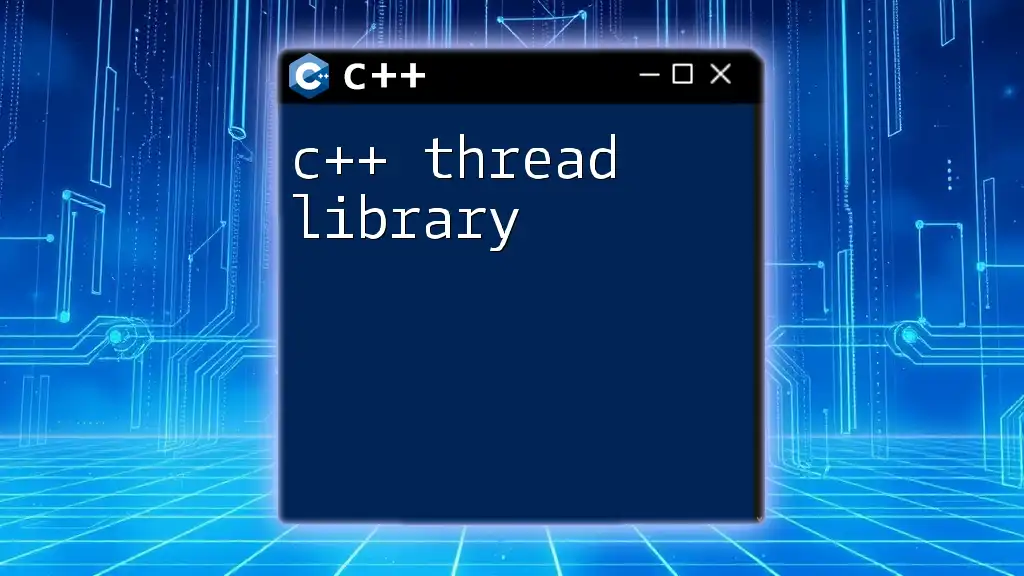
The Basics of C++ Threads
Creating Threads
In C++, we can easily create threads using the `std::thread` class. Here’s a basic example of spawning a thread:
#include <iostream>
#include <thread>
void threadFunction() {
std::cout << "Hello from the thread!" << std::endl;
}
int main() {
std::thread t(threadFunction);
t.join(); // Joining the thread
std::cout << "Thread has finished executing." << std::endl;
return 0;
}
In the example above, we define a function `threadFunction()` that the thread will execute. We then create a `std::thread` instance `t`, passing the function as an argument. By calling `t.join()`, we ensure that the main thread waits until `t` completes its execution before printing "Thread has finished executing."
Understanding Thread Lifecycles
Threads have different states throughout their lifecycles: running, ready, waiting, and terminated. Understanding these states is crucial for effective thread management, as it allows you to ensure that threads are performing as expected and that resources are managed correctly.
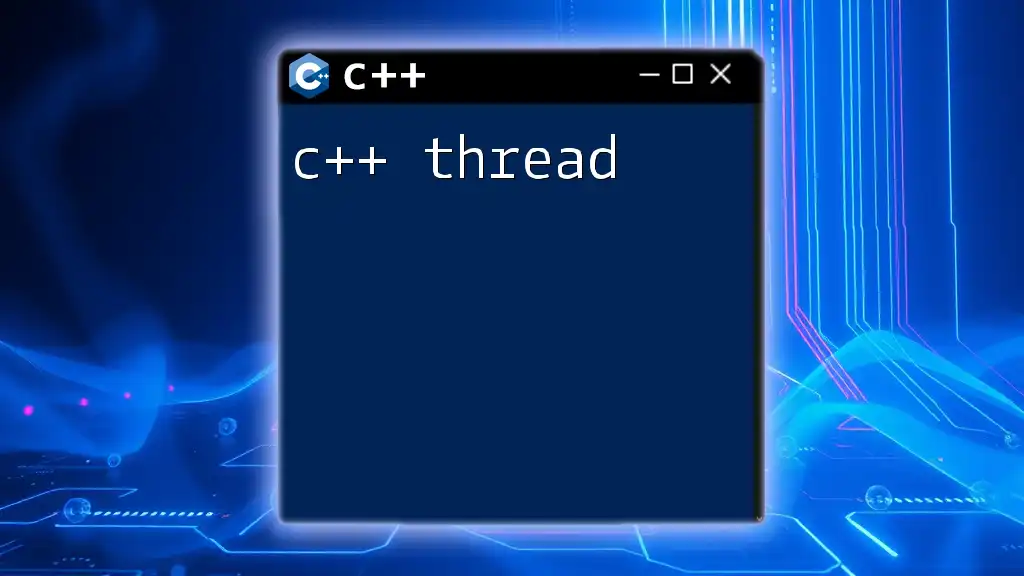
What is Thread Join C++: A Deeper Look
Definition and Purpose of Thread Join
The `join()` function is essential in the context of thread management. Its primary purpose is to coordinate the end of a thread with the rest of the program, thereby ensuring that resources are not prematurely released. This synchronization prevents race conditions—scenarios where multiple threads manipulate shared data without proper checks.
Implementing Thread Join in C++
Basic Example of Thread Join
Let’s take a closer look at using the thread join method in a simple program. Here’s how to implement it effectively:
#include <iostream>
#include <thread>
void calculateSum(int n) {
int sum = 0;
for (int i = 1; i <= n; ++i) {
sum += i;
}
std::cout << "Sum up to " << n << " is " << sum << std::endl;
}
int main() {
std::thread sumThread(calculateSum, 10);
// Perform other tasks in the main thread if needed
sumThread.join(); // Wait until sumThread completes
std::cout << "Sum calculation completed." << std::endl;
return 0;
}
In this example, we create a thread that calculates the sum of integers up to `n`. By calling `join()`, we ensure the main thread waits for the completion of `sumThread`. This synchronization is vital so that we obtain an accurate output reflecting the completed calculations.
Handling Exceptions with Thread Join
When working with threads, it's crucial to consider exception handling. If a thread encounters an error during execution, it might leave shared resources in an inconsistent state. Properly managing exceptions is necessary to ensure graceful termination and recovery:
#include <iostream>
#include <thread>
#include <stdexcept>
void threadFunction() {
throw std::runtime_error("Error in thread.");
}
int main() {
std::thread t(threadFunction);
try {
t.join(); // Attempt to join the thread
} catch (...) {
std::cout << "Thread ended with an exception." << std::endl;
}
return 0;
}
In the code above, if `threadFunction` throws an exception, it is captured in the main thread, allowing us to handle it gracefully. This approach ensures that the program remains robust and provides feedback regarding thread execution.
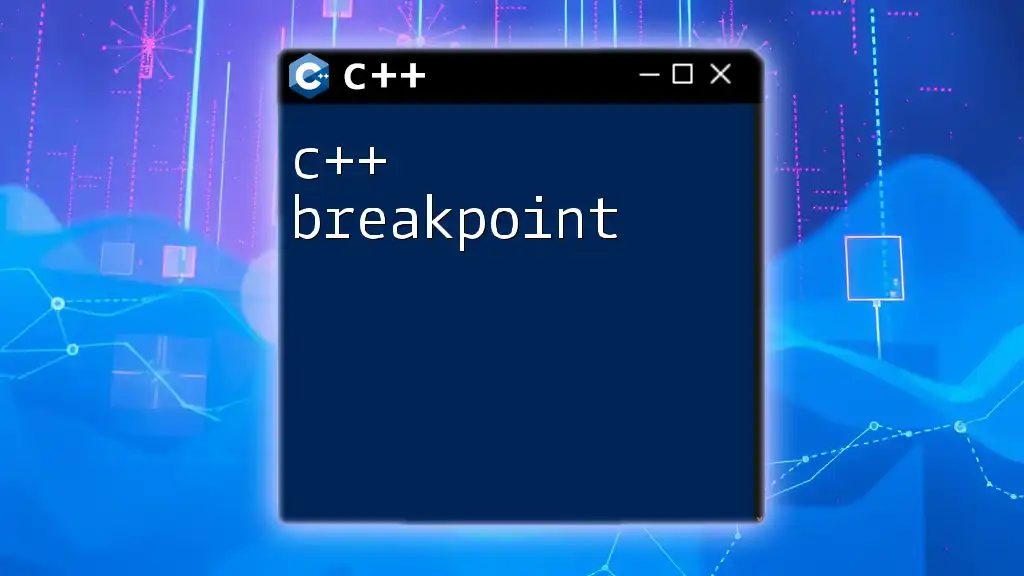
Advanced Concepts related to Thread Join
Detaching Threads vs. Joining Threads
Another important concept related to C++ thread join is the difference between joining and detaching threads. When a thread is detached using `detach()`, it runs independently from the main program. The main thread will not wait for the detached thread to finish, which is useful in scenarios where the thread does not need to return data or resources to the main program:
#include <iostream>
#include <thread>
#include <chrono>
void threadFunction() {
std::cout << "Thread is running..." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(2));
}
int main() {
std::thread t(threadFunction);
t.detach(); // The thread runs independently
std::this_thread::sleep_for(std::chrono::seconds(1));
std::cout << "Main thread finished." << std::endl;
return 0;
}
While detaching allows for parallel execution, it also bears risks: once detached, you cannot `join()` or communicate with that thread, risking resource leaks if the thread accesses shared data. Thus, understanding when to use `detach()` or `join()` is essential for efficient program design.
Best Practices for Using Thread Join
-
Always Join or Detach: A thread that has been started must either be joined or detached before the `std::thread` object goes out of scope; otherwise, it leads to program termination.
-
Handle Exceptions: Ensure robust exception handling within threads to prevent leaks and crashes. Always catch exceptions thrown in thread functions and handle them appropriately.
-
Resource Management: Be mindful of shared resources. When two threads access the same resource, utilize mutexes or other synchronization techniques to prevent race conditions.
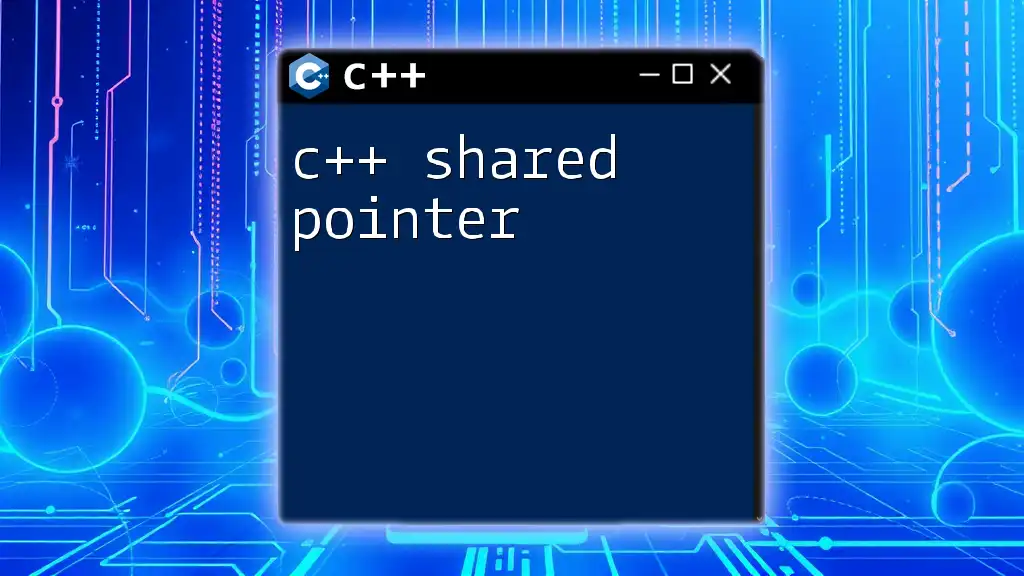
Conclusion
In summary, mastering C++ thread join is vital for any C++ programmer aiming for concurrency in their applications. By understanding how threads work, their lifecycles, and the importance of joining threads, you can create more efficient, safe, and responsive programs. Exploring these concepts further will help you better handle multi-threading challenges, paving the way for more advanced programming techniques.
Engage with the C++ community or consult additional resources to enhance your understanding of threading patterns and best practices in concurrent programming.