A C++ shared pointer is a smart pointer that retains shared ownership of an object through a pointer and automatically deletes the object when the last shared pointer to it is destroyed or reset.
#include <iostream>
#include <memory>
int main() {
std::shared_ptr<int> ptr1 = std::make_shared<int>(10); // Create a shared pointer
{
std::shared_ptr<int> ptr2 = ptr1; // Share ownership
std::cout << "Value: " << *ptr2 << ", Count: " << ptr2.use_count() << std::endl;
} // ptr2 goes out of scope here
std::cout << "Count after ptr2 goes out of scope: " << ptr1.use_count() << std::endl;
return 0;
}
Understanding Smart Pointers
What are Smart Pointers?
Smart pointers are an advanced way to manage memory in C++. Unlike raw pointers, which require manual memory management and can lead to leaks and undefined behavior, smart pointers automatically manage the lifetime of allocated memory. This ensures that resources are properly released when they are no longer needed, drastically reducing memory management issues in your code.
Types of Smart Pointers in C++
C++ offers various types of smart pointers, each tool suited for different scenarios:
- `std::unique_ptr`: This represents exclusive ownership of a resource. It cannot be copied, only moved.
- `std::shared_ptr`: It allows multiple pointers to share ownership of a single resource. The underlying resource is destroyed only when the last `shared_ptr` pointing to it is destroyed.
- `std::weak_ptr`: This acts as a non-owning reference to a resource managed by a `shared_ptr`, preventing cyclic references.
The focus of this article will be on `std::shared_ptr`.
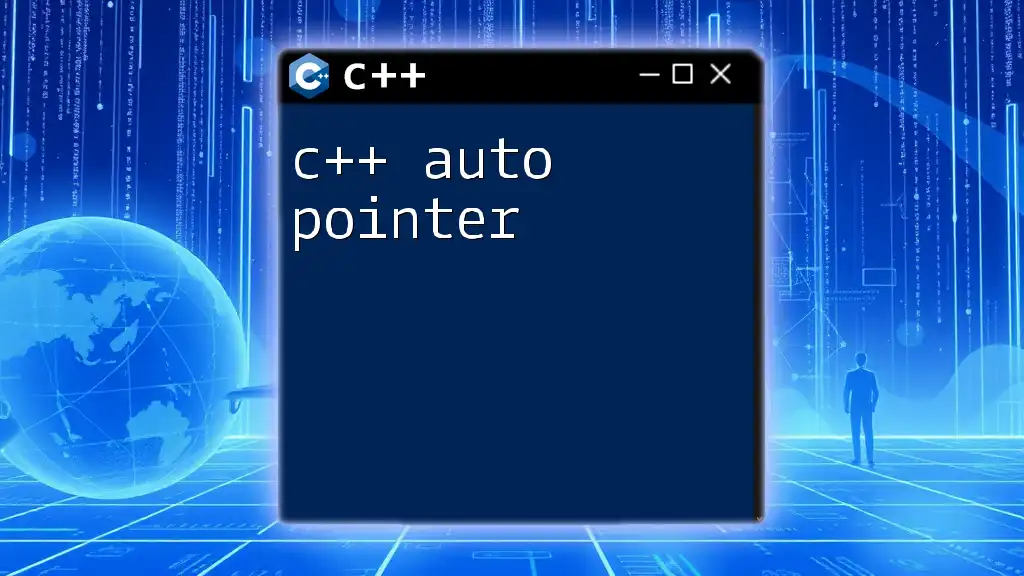
Introducing `std::shared_ptr`
What is `std::shared_ptr`?
A `std::shared_ptr` is a smart pointer that retains shared ownership of an object through a pointer. When the last `shared_ptr` referring to the object is destroyed, the object is automatically deleted. This mechanism provides a convenient way of managing dynamically allocated memory without the need to manually delete it.
How `std::shared_ptr` Works
Reference Counting Mechanism: A `shared_ptr` uses a reference count to keep track of how many pointers refer to the same resource. Each time a new `shared_ptr` is created from an existing one, the reference count increases. Conversely, when a `shared_ptr` goes out of scope or is reassigned, the count decreases. When the count reaches zero, the allocated memory is freed.
Memory Lifecycle Management: This dynamic management of memory ensures that resources are freed as soon as they are no longer needed, thus preventing memory leaks.
Basic Syntax of `std::shared_ptr`
To create a `std::shared_ptr`, you can utilize the `make_shared` function, which constructs the object in a single memory allocation, leading to better performance and exception safety:
#include <memory>
std::shared_ptr<int> ptr = std::make_shared<int>(10);
Creating a `std::shared_ptr`
You can create a `std::shared_ptr` in two primary ways:
-
Using `make_shared`: This is the recommended approach as it simplifies memory allocation.
auto ptr1 = std::make_shared<int>(20);
-
Direct initialization: You can also create a `shared_ptr` using its constructor, but this is less efficient due to separate memory allocations.
std::shared_ptr<int> ptr2(new int(30)); // Less efficient
Using `make_shared` is preferred for both performance and safety.
Accessing and Modifying Values with `std::shared_ptr`
Once you have a `shared_ptr`, accessing and modifying the value it points to is straightforward. You can dereference it just like a raw pointer:
ptr = std::make_shared<int>(40);
std::cout << *ptr; // Output: 40
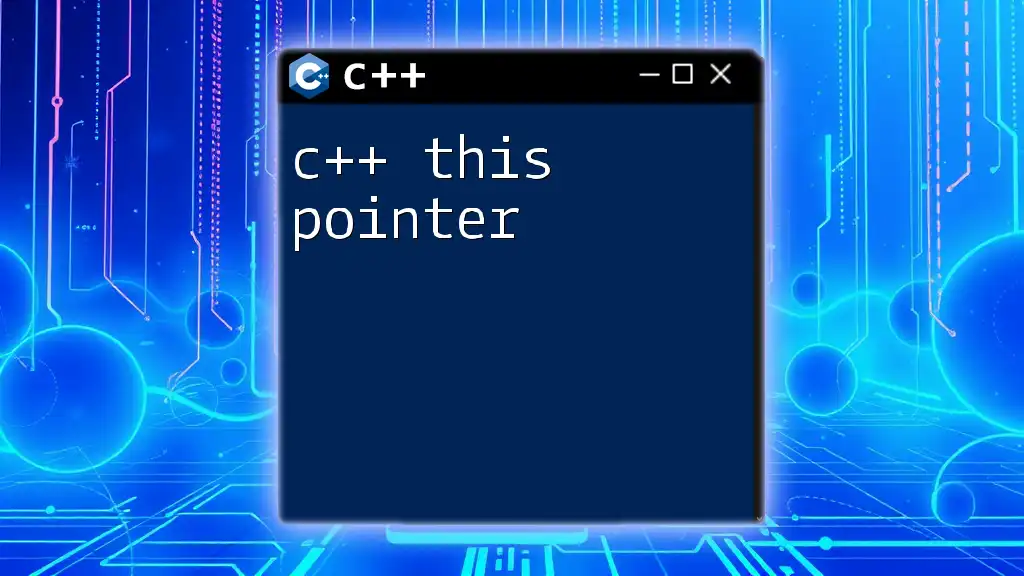
Advanced Usage of `std::shared_ptr`
Sharing Ownership
A key feature of `std::shared_ptr` is its ability to allow multiple owners for a single resource. When a `shared_ptr` is assigned to another `shared_ptr`, both share the ownership of the pointed-to resource, as shown in the example below:
std::shared_ptr<int> ptr1 = std::make_shared<int>(50);
std::shared_ptr<int> ptr2 = ptr1; // ptr2 shares ownership of ptr1
std::cout << *ptr1; // Output: 50
std::cout << *ptr2; // Output: 50
Managing Cyclic References
While `std::shared_ptr` is efficient for managing resource ownership, cyclic references can lead to memory leaks if two or more `shared_ptr` instances form a loop. In these cases, the memory will not be freed even when the pointers go out of scope.
To solve this issue, you can use `std::weak_ptr`. This pointer type does not increment the reference count, thus allowing the resources to be released:
struct Node {
std::shared_ptr<Node> next;
// Use weak_ptr to prevent cycle
std::weak_ptr<Node> previous;
};
Performance Considerations
While `std::shared_ptr` offers flexibility, there is an overhead involved due to its reference counting. Each copy and assignment involves incrementing or decrementing the reference counter which, in performance-critical sections of code, may outweigh the benefits of convenience. In these cases, prefer `std::unique_ptr` for scenarios where single ownership is sufficient.
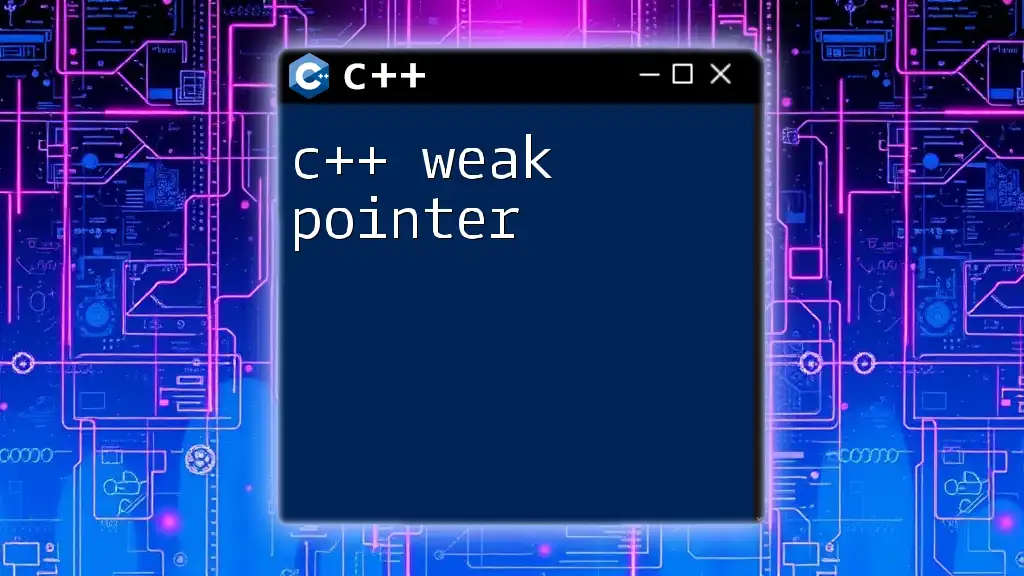
Best Practices for Using `std::shared_ptr`
When to Use `std::shared_ptr`
Use `std::shared_ptr` in scenarios where:
- You need shared ownership of an object.
- The object's lifecycle cannot be easily managed by a single owner.
Avoiding Common Pitfalls
When using `std::shared_ptr`, keep the following guidelines in mind:
- Avoid circular references by pairing `shared_ptr` with `weak_ptr`.
- Don’t use `shared_ptr` if exclusive ownership is enough; prefer `std::unique_ptr` for simplicity and performance.
- Be wary of excessive copying, as each copy incurs overhead.
Exception Safety with `std::shared_ptr`
Using `std::shared_ptr` enhances exception safety in your code. If an exception occurs, `shared_ptr`'s destructor automatically manages memory cleanup. To ensure that memory management remains efficient and safe, always use `make_shared` rather than manual memory allocation wherever possible.
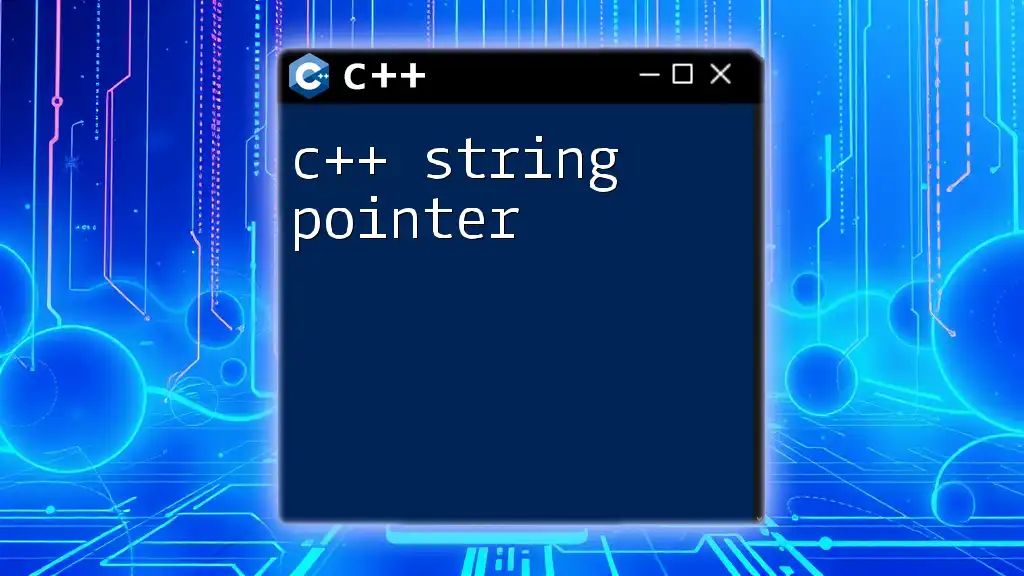
Real-World Applications of `std::shared_ptr`
Use Cases in Modern C++ Development
In modern C++ software development, `std::shared_ptr` is particularly advantageous when working with complex class hierarchies or resources that are shared across multiple components. For example, consider a graphical application where multiple objects need to share access to textures or configuration data.
Example Project: Memory Management
Here’s a succinct example demonstrating the use of `std::shared_ptr` in a real-world context:
#include <iostream>
#include <memory>
struct Resource {
Resource() { std::cout << "Resource acquired\n"; }
~Resource() { std::cout << "Resource released\n"; }
};
void example() {
std::shared_ptr<Resource> res1 = std::make_shared<Resource>();
{
std::shared_ptr<Resource> res2 = res1; // Shared ownership
} // res2 goes out of scope, but resource is not released
}
In this example, the `Resource` will only be released when the last `shared_ptr` pointing to it is destroyed, effectively managing memory and reducing the likelihood of leaks.
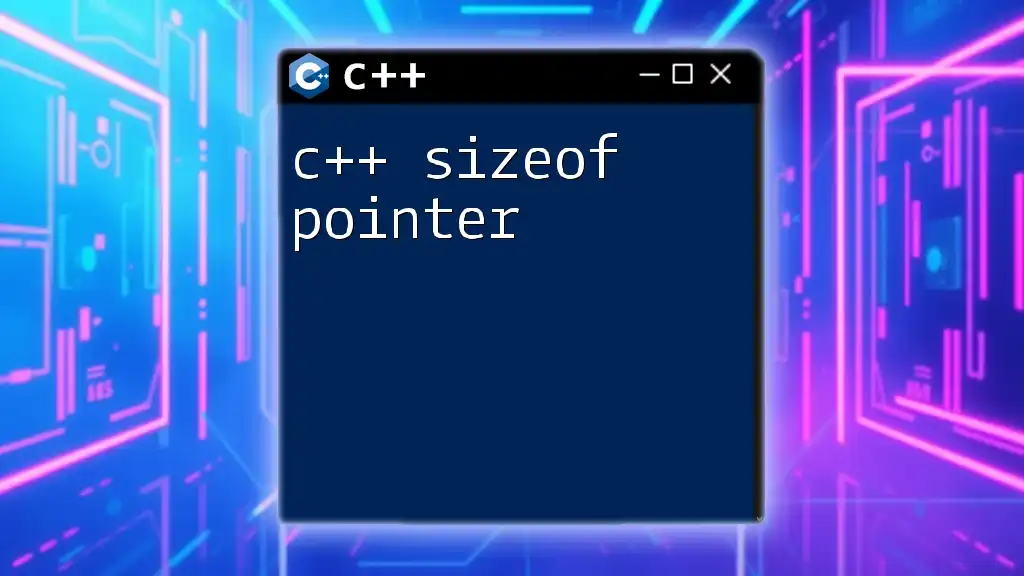
Conclusion
In summary, C++ shared pointers are a powerful feature that enhances memory management by allowing multiple pointers to share ownership of a resource. Through reference counting and automatic memory management, `std::shared_ptr` significantly reduces the risk of memory leaks and undefined behaviors associated with raw pointers. However, understanding its mechanisms and adhering to best practices is crucial to ensuring optimal performance and safety in your applications.
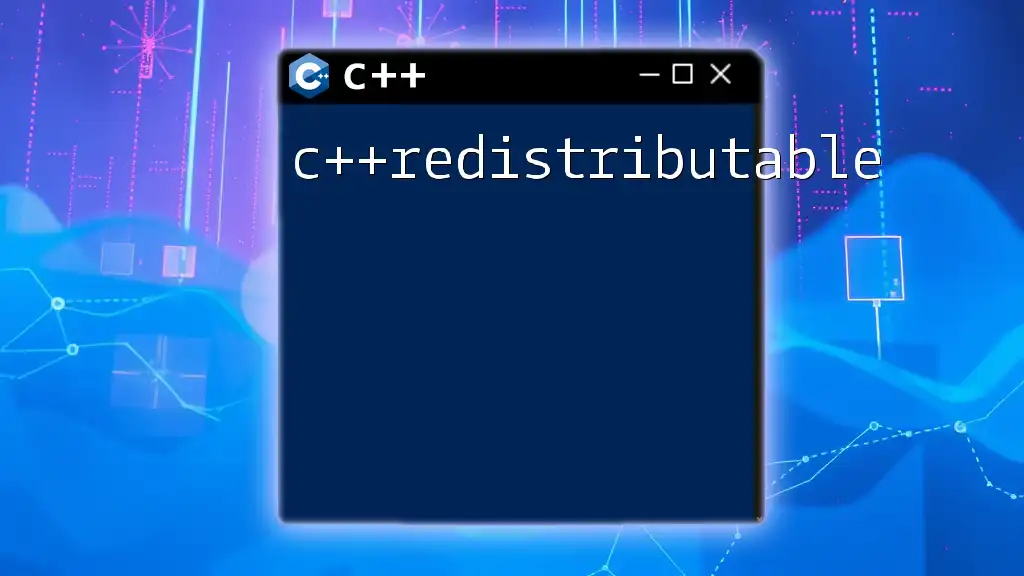
Further Reading and Resources
For those seeking to delve deeper into the world of smart pointers, consider checking out the official C++ documentation, as well as books and online resources focused on modern C++ practices. Engaging with online communities can also provide valuable insights and assistance.
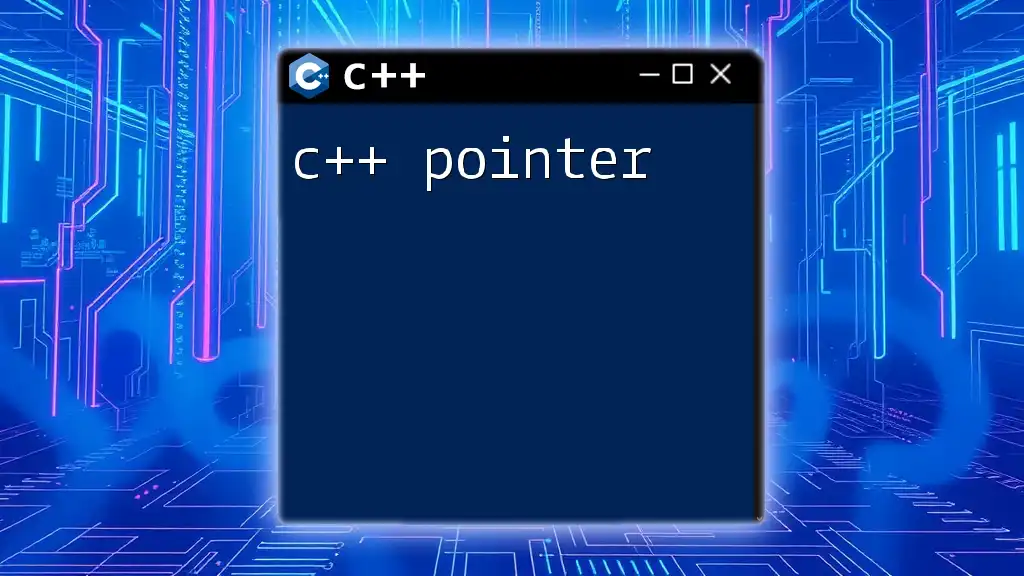
FAQs
What are the advantages of `std::shared_ptr` over raw pointers?
The primary advantage lies in automatic memory management. Unlike raw pointers, `shared_ptr` ensures that memory is released once all owners are done with it, reducing the chances of memory leaks and dangling pointers.
Can `std::shared_ptr` be used for arrays?
You should avoid using `std::shared_ptr` directly for arrays. Instead, use `std::shared_ptr` with single objects or pair with arrays using custom deleters to properly manage the memory.
How do I prevent memory leaks when using `std::shared_ptr`?
To prevent memory leaks, be vigilant about circular references. Utilize `std::weak_ptr` where necessary to break cycles, and prefer `std::make_shared` for safer memory allocation practices.