A C++ `unique_ptr` is a smart pointer that ensures exclusive ownership of a dynamically allocated object, automatically deallocating the memory when the `unique_ptr` goes out of scope.
#include <iostream>
#include <memory>
int main() {
std::unique_ptr<int> ptr = std::make_unique<int>(42);
std::cout << *ptr << std::endl; // Outputs: 42
return 0;
}
What are Unique Pointers?
C++ unique pointers, represented by `std::unique_ptr`, are smart pointers that manage the lifetime of dynamically-allocated objects. They ensure that there is a single owner for the resource they manage, eliminating the risk of double deletions and memory leaks that can occur with raw pointers. This ownership semantic is a critical feature in modern C++ programming, allowing for more maintainable and safer code.
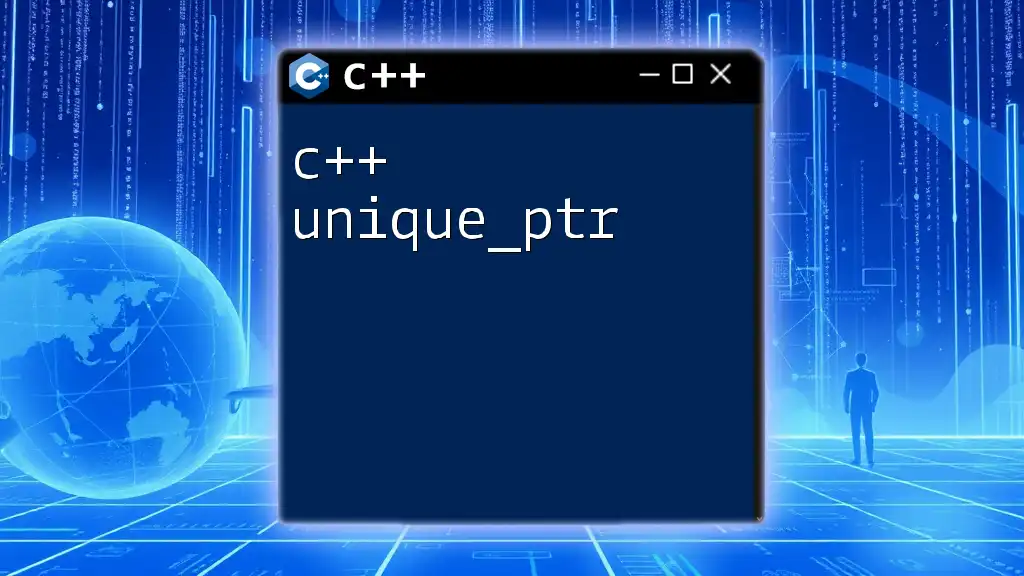
Why Use Unique Pointers?
Using unique pointers has several distinct advantages:
- Memory Management: Unique pointers automatically release the memory when they go out of scope, reducing the likelihood of memory leaks.
- Ownership Semantics: Unique pointers clarify ownership in your code. If a unique pointer is passed to a function, its ownership is transferred, and the original pointer becomes null, preventing accidental usage.
- Exception Safety: In the event of an exception, unique pointers automatically clean up the resources they own, promoting safer and cleaner resource management.
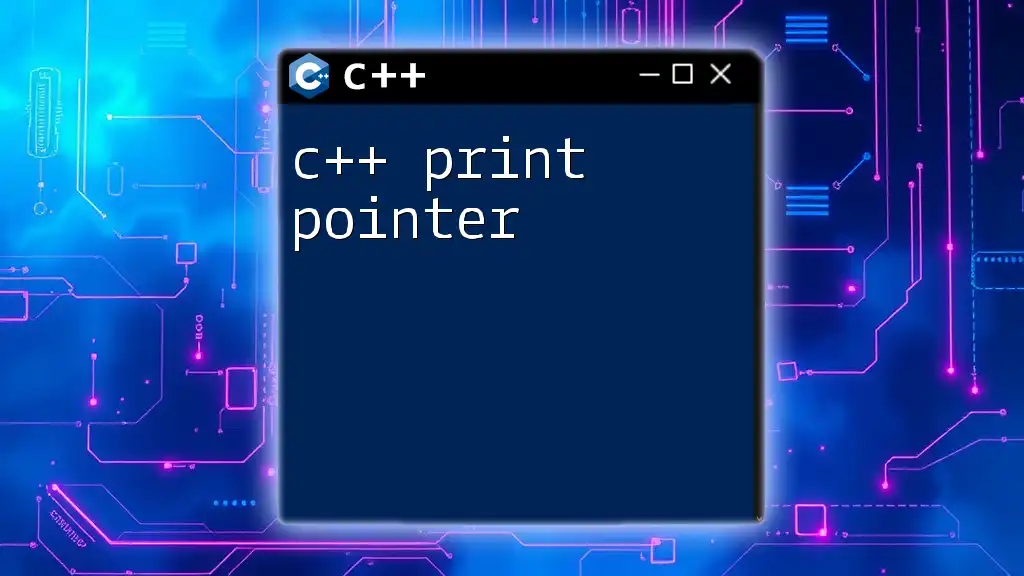
Getting Started with Unique Pointers
Including the Required Header
To use unique pointers, you must include the `<memory>` header in your program. Without this header, you won't have access to `std::unique_ptr`.
#include <memory>
Creating a Unique Pointer
The simplest way to create a unique pointer is using `std::make_unique`, which was introduced in C++14. This function simplifies memory allocation and ensures exception safety.
std::unique_ptr<int> ptr = std::make_unique<int>(5);
In this example, `ptr` now owns a dynamically-allocated integer initialized to 5. The use of `make_unique` avoids potential memory leaks as it handles memory allocation and deallocation safely.
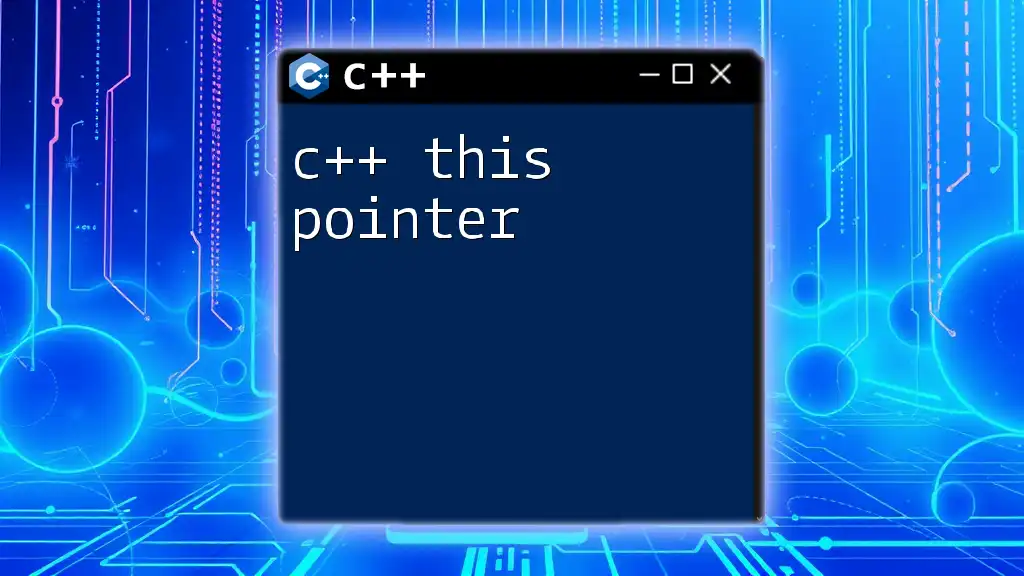
Working with Unique Pointers
Accessing the Object
Once you have a unique pointer, you can access the underlying object using the dereference operator (`*`) or the arrow operator (`->`).
*ptr = 10; // Change value
std::cout << *ptr << std::endl; // Output: 10
Here, the `*ptr` dereferences the unique pointer to access the integer, allowing us to modify its value. Accessing members of a class through a unique pointer works similarly using `ptr->someMember`.
Transferring Ownership
Moving Unique Pointers
One of the most essential features of unique pointers is that their ownership can be transferred using the `std::move` function, adhering to C++'s move semantics.
std::unique_ptr<int> ptr2 = std::move(ptr);
In this code snippet, ownership of the integer is transferred from `ptr` to `ptr2`. After the move, `ptr` will be null, ensuring that there's no risk of two pointers managing the same memory.
Passing Unique Pointers to Functions
When passing unique pointers as function parameters, it's best to pass by value. This promotes ownership transfer to the function.
void process(std::unique_ptr<int> p) {
std::cout << *p << std::endl;
}
In this example, the ownership of the resource is given to the `process` function, and `p` will manage the resource locally. This design pattern is beneficial for maintaining clear ownership semantics.
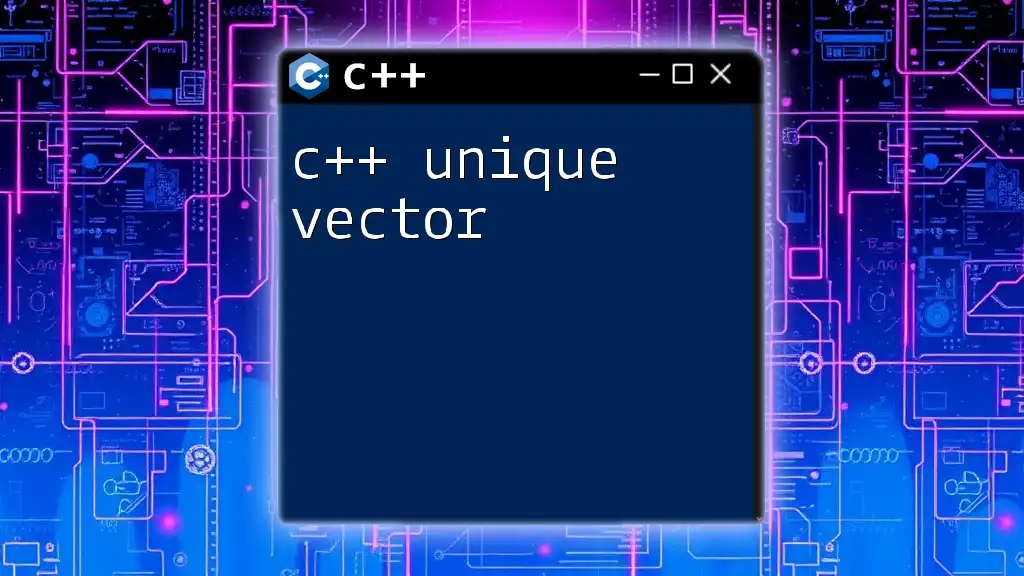
Memory Management with Unique Pointers
Automatic Memory Management
Unique pointers automatically release managed memory when they go out of scope. This feature eliminates the need for explicit `delete` calls, which are often sources of bugs in C++ programs.
Resetting Unique Pointers
Sometimes, you may want to replace the object owned by a unique pointer. The `reset()` method can be used for this purpose:
ptr.reset(new int(20)); // Resets the pointer
In this scenario, the previous integer object is deleted, and `ptr` now owns a new integer with a value of 20. This mechanism ensures the old memory is returned safely to the system, preventing leaks.
Releasing Ownership
If you need to release the ownership of the resource managed by a unique pointer, you can use the `release()` method.
int* rawPtr = ptr.release();
This code transfers ownership from `ptr` to the raw pointer `rawPtr`. After this operation, `ptr` becomes null, and it is the programmer's responsibility to manage the lifetime of `rawPtr`.
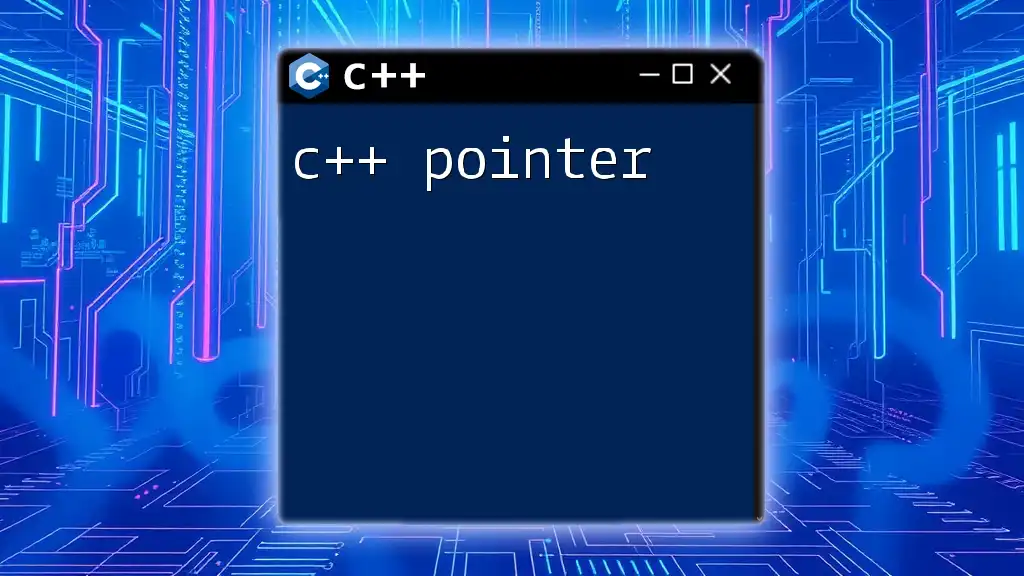
Unique Pointer in Collections
Using Unique Pointers in Containers
Unique pointers can be stored in standard library containers, enabling dynamic allocation while still managing resource ownership effectively.
std::vector<std::unique_ptr<int>> vec;
vec.push_back(std::make_unique<int>(5));
This example demonstrates how to use unique pointers in a `std::vector`. Here, `vec` manages a vector of integers, stored as unique pointers. This design leverages automatic memory management while still benefiting from the capabilities of STL containers.
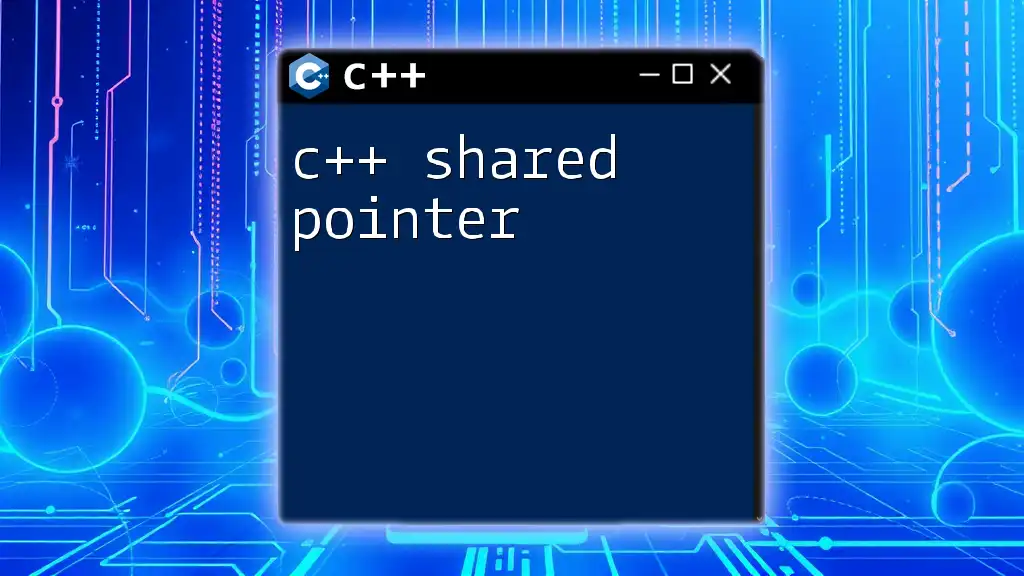
Common Pitfalls and Best Practices
Common Mistakes with Unique Pointers
- Misusing `std::move`: It is crucial to remember that after moving a unique pointer, the source pointer becomes null. Attempting to access or use it afterward can lead to undefined behavior.
- Forgetting to Include `<memory>`: Neglecting this header will lead to compilation errors. Always ensure it is present when working with smart pointers.
Best Practices for Using Unique Pointers
To maximize the benefits of unique pointers, consider these best practices:
- Use `std::make_unique` for better safety and simplicity.
- Prefer passing unique pointers by value to functions to clarify ownership semantics.
- Be cautious when moving unique pointers to avoid dangling pointers.
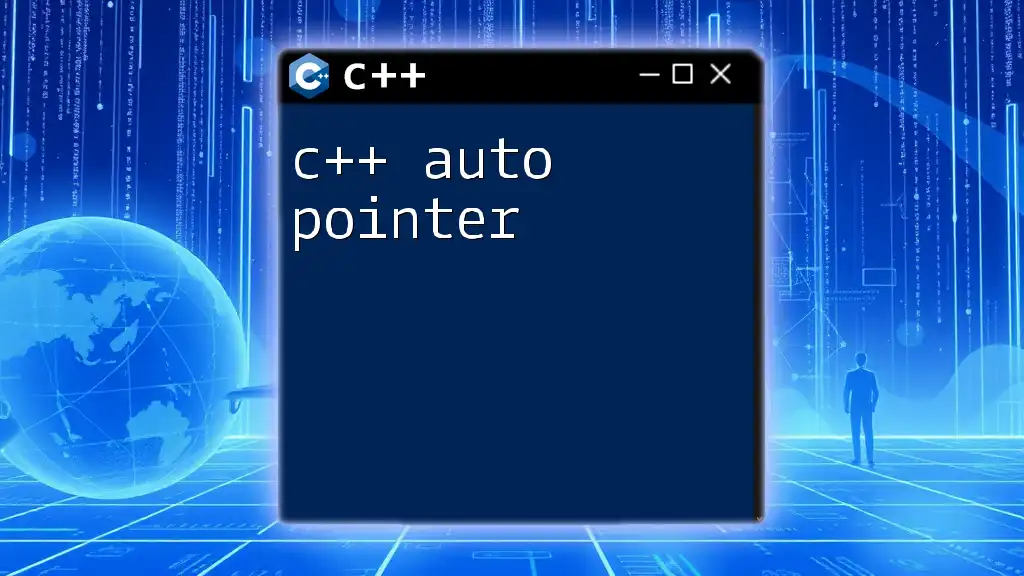
Conclusion
In summary, the C++ unique pointer is an invaluable tool for modern C++ developers. It simplifies memory management, clarifies ownership, and provides robust exception safety. The unique pointer's features promote cleaner, safer code, making it an essential component of effective C++ programming. Embrace unique pointers in your projects to enhance your coding practices and memory handling strategies.
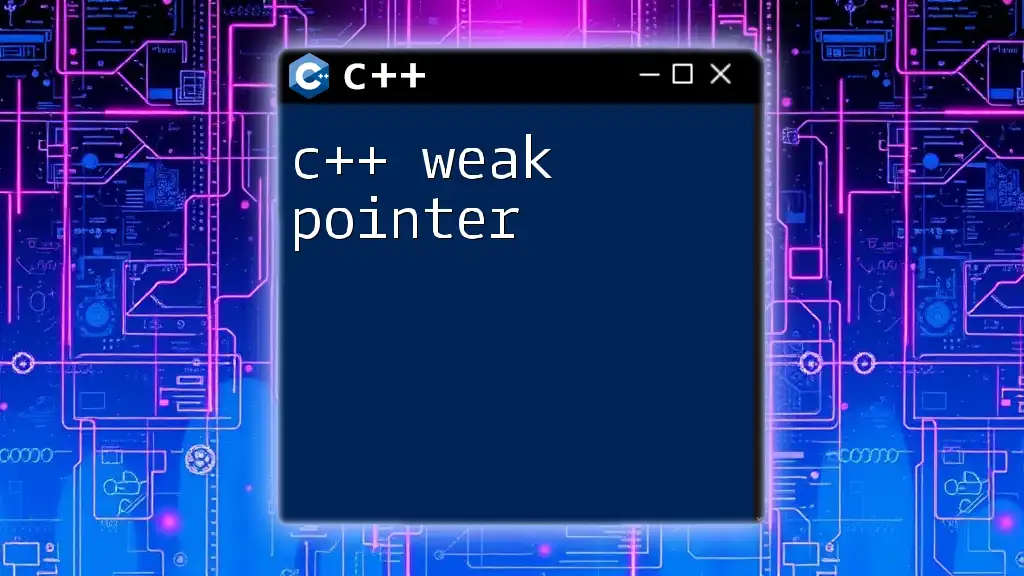
References
- For further reading, consult C++ standard documentation and look into highly recommended books on smart pointers and resource management strategies in C++.