A C++ weak pointer is a smart pointer that holds a non-owning reference to an object managed by a shared pointer, preventing circular references and allowing the object to be destroyed while still maintaining a reference to it.
Here’s a code snippet demonstrating the use of a weak pointer:
#include <iostream>
#include <memory>
class MyClass {
public:
MyClass(int value) : value(value) {}
int value;
};
int main() {
std::shared_ptr<MyClass> sharedPtr = std::make_shared<MyClass>(10);
std::weak_ptr<MyClass> weakPtr = sharedPtr;
std::cout << "Shared pointer value: " << sharedPtr->value << std::endl;
if (auto tempPtr = weakPtr.lock()) {
std::cout << "Weak pointer value: " << tempPtr->value << std::endl;
} else {
std::cout << "Weak pointer is expired." << std::endl;
}
return 0;
}
Understanding Pointer Ownership
What are Smart Pointers in C++?
Smart pointers are a fundamental feature in C++ that provide automatic memory management. Unlike raw pointers, which require manual memory management, smart pointers help prevent memory leaks and dangling pointers. There are three primary types of smart pointers in C++:
- Unique Pointers (`std::unique_ptr`): These pointers ensure that only one unique pointer can own a resource. When the unique pointer goes out of scope, the resource is automatically freed.
- Shared Pointers (`std::shared_ptr`): Multiple shared pointers can share ownership of a resource. The resource is only freed when the last shared pointer owning it is destroyed.
- Weak Pointers (`std::weak_ptr`): These pointers provide a non-owning reference to a resource that is managed by a `shared_ptr`. Weak pointers do not affect the reference count of the shared pointer, making them essential for certain design patterns.
The Concept of Ownership in C++
In C++, ownership refers to the entity that is responsible for the lifecycle of a resource (like memory). When ownership is poorly managed, it can lead to problems such as memory leaks, where memory is allocated but never deallocated, or dangling pointers, which reference memory that has already been freed.
One common issue that arises is circular references. This occurs when two or more shared pointers point to each other, creating a scenario where neither pointer can be destroyed because they are both waiting for the other to release its ownership. This is where weak pointers come into play.
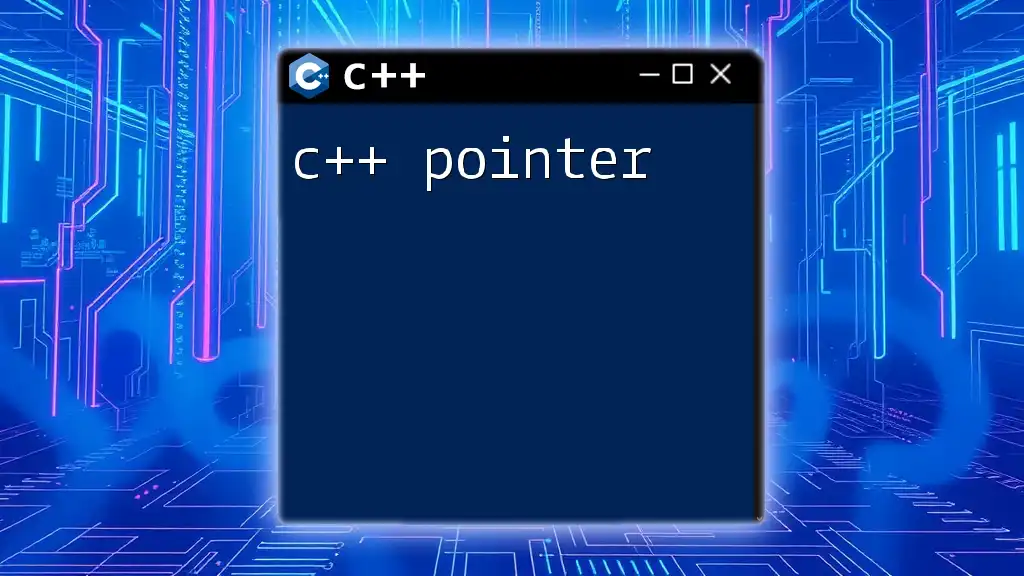
What is a Weak Pointer?
Definition of Weak Pointer
A weak pointer is a smart pointer that does not participate in the ownership of an object. Rather, it holds a non-owning reference to an object that is managed by a `shared_ptr`. The primary purpose of a weak pointer is to provide a way to observe an object without owning it, thereby preventing potential memory leaks caused by circular dependencies.
Use Cases for Weak Pointers
Weak pointers are particularly useful in scenarios where you need to maintain a relationship between objects without committing to ownership. Common use cases include:
- Preventing Memory Leaks: Weak pointers allow resource observers to refer to a resource without preventing its memory from being reclaimed.
- Observer Patterns: In the observer design pattern, a subject needs to notify its observers about changes without keeping them alive unnecessarily.
- Caching Mechanisms: Weak pointers can be used in caching strategies where cached objects can be reclaimed if there are no strong references left.
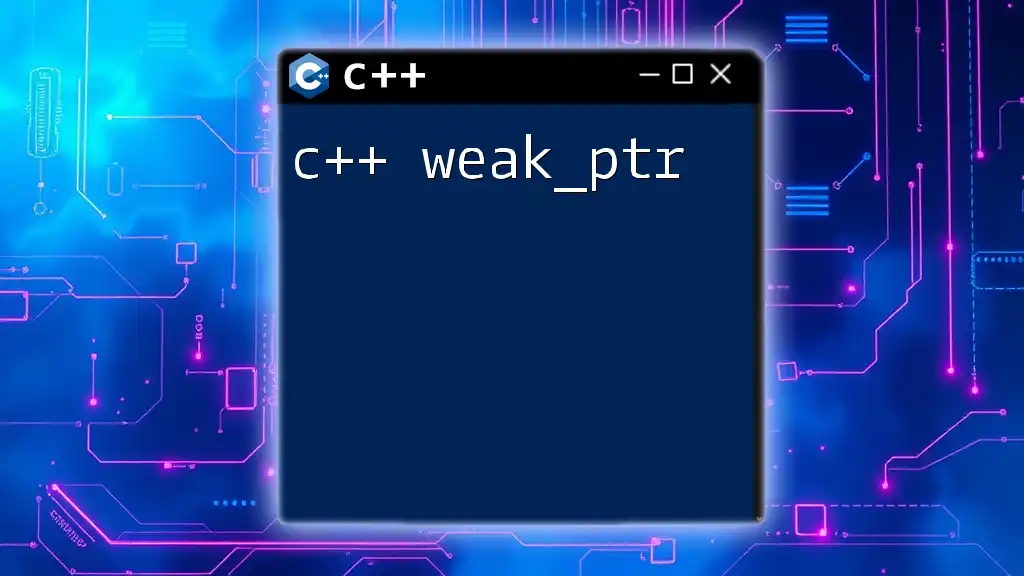
Creating and Using Weak Pointers in C++
Syntax of Weak Pointer
The syntax for declaring a weak pointer is straightforward:
std::weak_ptr<Type> weakPtr;
Initializing a Weak Pointer
To create a weak pointer, you typically initialize it with a shared pointer:
std::shared_ptr<Type> sharedPtr = std::make_shared<Type>();
std::weak_ptr<Type> weakPtr(sharedPtr);
This establishes a weak reference to the resource owned by `sharedPtr`.
Checking Weak Pointer Validity
Before using a weak pointer, it's crucial to check if the resource is still valid. The `expired()` method can help you determine this:
if (weakPtr.expired()) {
// Handle the case where the weak pointer is no longer valid
}
This method returns `true` if the resource has been deleted.
Converting a Weak Pointer to a Shared Pointer
To access the resource held by a weak pointer, you must first lock it, which attempts to create a temporary shared pointer:
std::shared_ptr<Type> sharedPtrFromWeak = weakPtr.lock();
if (sharedPtrFromWeak) {
// Successfully converted to shared_ptr; use the resource
}
If the locking fails (indicating the original resource has been freed), `sharedPtrFromWeak` will be `nullptr`.
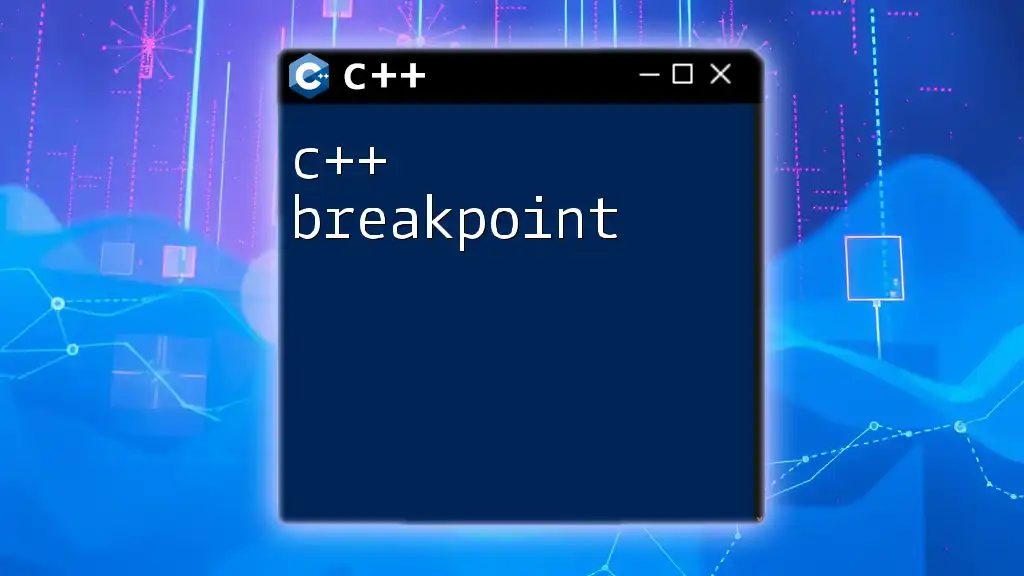
Common Mistakes and Best Practices
Mistakes to Avoid with Weak Pointers
One common mistake when using weak pointers is neglecting to check their validity before dereferencing. Always ensure your weak pointer is still valid by using `expired()` or trying to acquire a shared pointer through `lock()`.
Another pitfall is confusing weak pointers with raw pointers. Unlike raw pointers, weak pointers do not manage resource ownership; they simply observe.
Best Practices for Weak Pointers
To effectively use weak pointers, consider the following best practices:
- Use weak pointers when you need to reference an object without claiming ownership, especially in cases involving circular references.
- Always check the validity of a weak pointer before using it. This will help avoid dereferencing null or dangling pointers.
- Be intentional about your choice between shared and weak pointers based on your ownership needs within your application architecture.
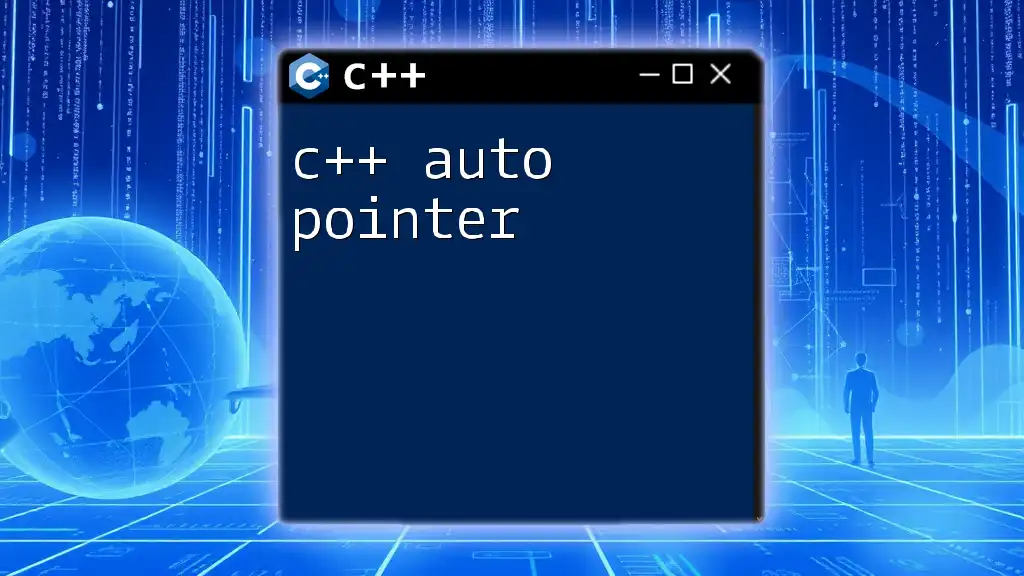
Real-World Example of Weak Pointer
Use Case: Implementing an Observer Pattern
The observer pattern is a design pattern that allows an object (the subject) to notify other objects (the observers) about changes in its state. Here’s a simplified implementation using weak pointers:
class Observer {
public:
virtual void update() = 0;
};
class Subject {
private:
std::vector<std::weak_ptr<Observer>> observers;
public:
void addObserver(std::shared_ptr<Observer> obs) {
observers.push_back(obs);
}
void notifyObservers() {
for (const auto& weakObs : observers) {
if (auto obs = weakObs.lock()) {
obs->update(); // Notify valid observers
}
}
}
};
In this example, `Subject` maintains a weak reference to its `Observers`, ensuring that it does not prevent them from being destroyed when no longer needed. This creates a clean and efficient way to manage the lifecycle of both the subjects and their observers.
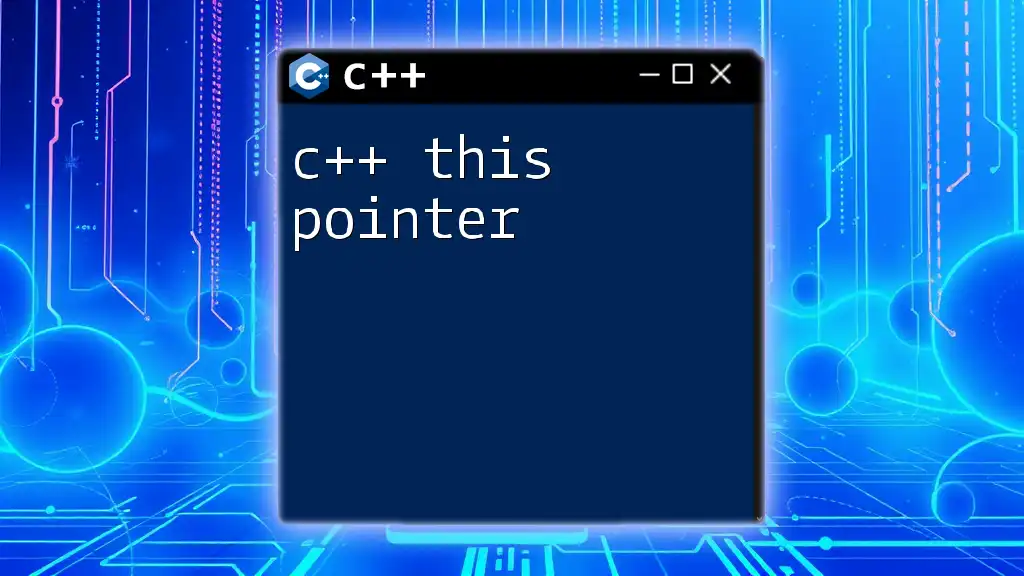
Conclusion
C++ weak pointers are a powerful tool for effective memory management and maintaining relationships between objects while avoiding circular dependencies. By using weak pointers, developers can prevent memory leaks, improve design patterns like observers, and efficiently manage resource lifetimes.
Remember to practice using `std::weak_ptr` and embrace its unique capabilities in your C++ projects to become a more efficient and effective programmer.
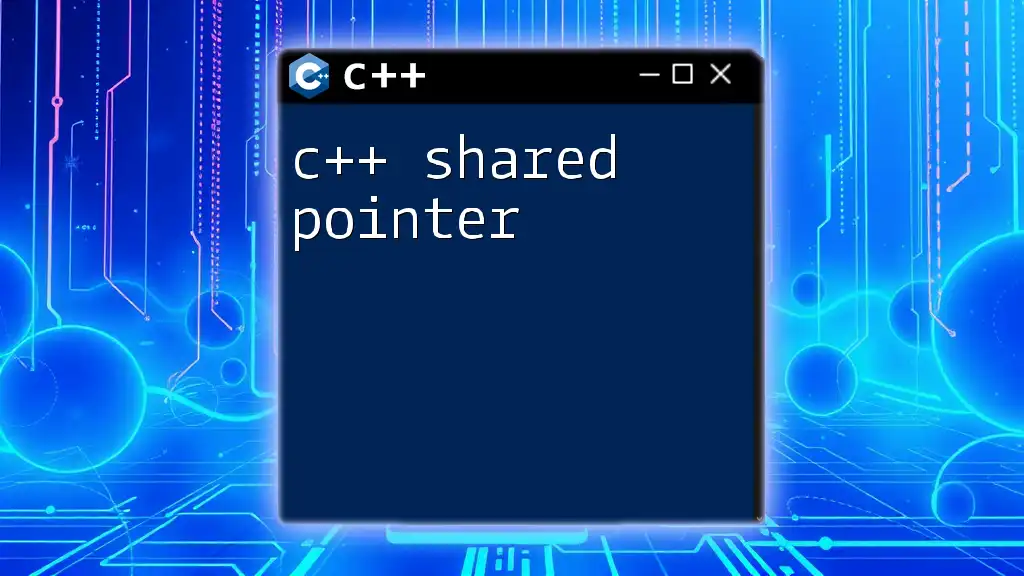
Resources for Further Learning
Recommended Books and Online Courses
To deepen your understanding of C++ memory management and smart pointers, consider exploring recommended books and online courses. These resources typically provide insights into practical implementation and best practices.
Community and Discussion Platforms
Engaging with the C++ community through forums and discussion platforms can greatly enhance your learning. These platforms offer opportunities to ask questions, share knowledge, and participate in discussions about C++ and its features.
Final Thoughts
As you continue your journey with C++, leveraging weak pointers effectively will greatly enhance your applications' robustness and efficiency. Explore and experiment with these concepts to gain confidence and mastery in your programming endeavors.