In C++, the `sizeof` operator returns the size in bytes of a pointer, which is typically 4 bytes on a 32-bit system and 8 bytes on a 64-bit system.
Here’s a code snippet demonstrating this:
#include <iostream>
int main() {
int* ptr;
std::cout << "Size of pointer: " << sizeof(ptr) << " bytes" << std::endl;
return 0;
}
Understanding Pointers
What is a Pointer?
A pointer in C++ is a variable that stores the memory address of another variable. This allows programmers to manipulate memory directly, enabling a deeper level of control over data storage and manipulation. Pointers are essential for dynamic memory management, arrays, and building complex data structures like linked lists and trees.
Syntax of Pointers
To declare a pointer, you use the `*` operator in conjunction with the data type. For example:
int* ptr;
In this case, `ptr` is a pointer to an integer. The asterisk (*) signifies that the variable is a pointer.
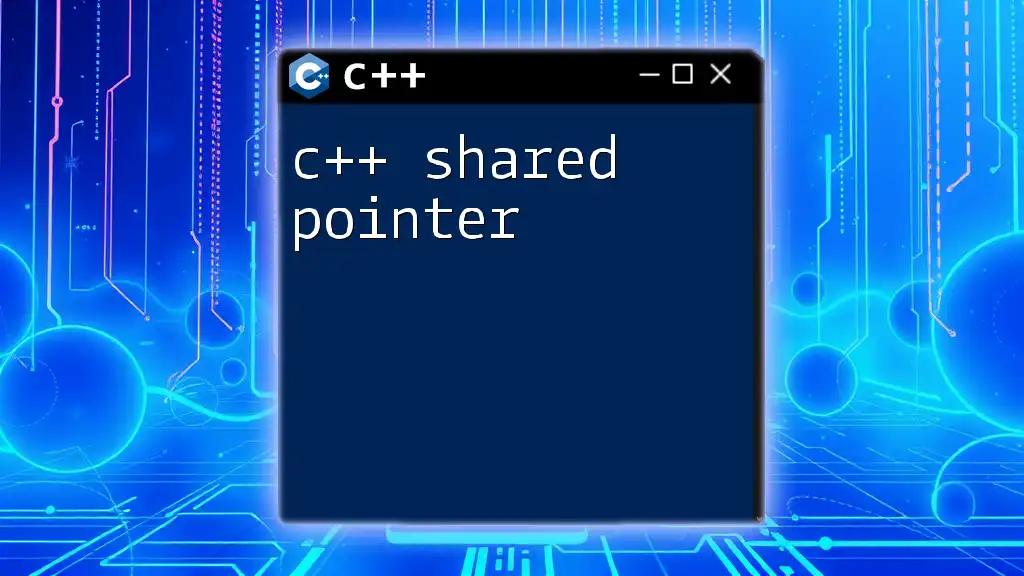
The sizeof Operator
What is sizeof?
The `sizeof` operator in C++ is used to determine the size, in bytes, of a variable or data type. It provides a way to understand how much memory is allocated for different data types and can be a helpful tool in debugging memory allocations or managing memory usage in your applications.
How sizeof Works with Pointers
When used with pointers, `sizeof` calculates the size of the pointer itself, not the size of the variable it points to. The actual size of the pointer depends on the architecture of the system (32-bit vs. 64-bit). For example:
int a;
int* ptr;
std::cout << sizeof(a) << " " << sizeof(ptr) << std::endl;
In this code, `sizeof(a)` would typically return `4` on a 32-bit system and `8` on a 64-bit system. However, `sizeof(ptr)` will return `4` or `8`, depending on the architecture, regardless of the data type it points to.

Practical Examples of sizeof Pointer
Size of Different Pointer Types
Different pointer types also share the same size depending on their architecture. When declaring pointers to various types, such as `int`, `double`, and `char`, you may see the following in your outputs:
int* intPtr;
double* doublePtr;
char* charPtr;
std::cout << "Size of int pointer: " << sizeof(intPtr) << std::endl;
std::cout << "Size of double pointer: " << sizeof(doublePtr) << std::endl;
std::cout << "Size of char pointer: " << sizeof(charPtr) << std::endl;
No matter the data type pointed to, the output on a 64-bit system will typically show `8` for each pointer, while on a 32-bit system, it may show `4` for each. This uniformity emphasizes the concept that the size of a pointer does not depend on the data type it references.
Size of Const and Volatile Pointers
Const pointers and volatile pointers behave similarly when it comes to their size. They provide a means of guaranteeing immutability or allowing for hardware optimization without affecting memory size. Here’s how you might check their sizes:
const int* constPtr;
volatile int* volatilePtr;
std::cout << "Size of const pointer: " << sizeof(constPtr) << std::endl;
std::cout << "Size of volatile pointer: " << sizeof(volatilePtr) << std::endl;
In both cases, the result will typically be the same as that for a standard pointer of the appropriate type.

Factors Affecting Pointer Size
Platform and Architecture Influence
The size of pointers varies based on the platform and the architecture of the system. On a 32-bit architecture, pointers are usually `4 bytes`, while on a 64-bit architecture, they are typically `8 bytes`. For example:
std::cout << "sizeof(int*): " << sizeof(int*) << std::endl;
This line will print `4` on a 32-bit system and `8` on a 64-bit system. Understanding this concept is crucial for writing robust C++ code that runs correctly across different platforms.
Compiler Influence
Different compilers may also produce pointers of varying sizes depending on how they optimize code. Some compilers may allow you to specify certain flags that define the maximum size of pointers, such as `-m32` or `-m64` in GCC. Awareness of these options can help you control memory more effectively, especially in memory-constrained environments.
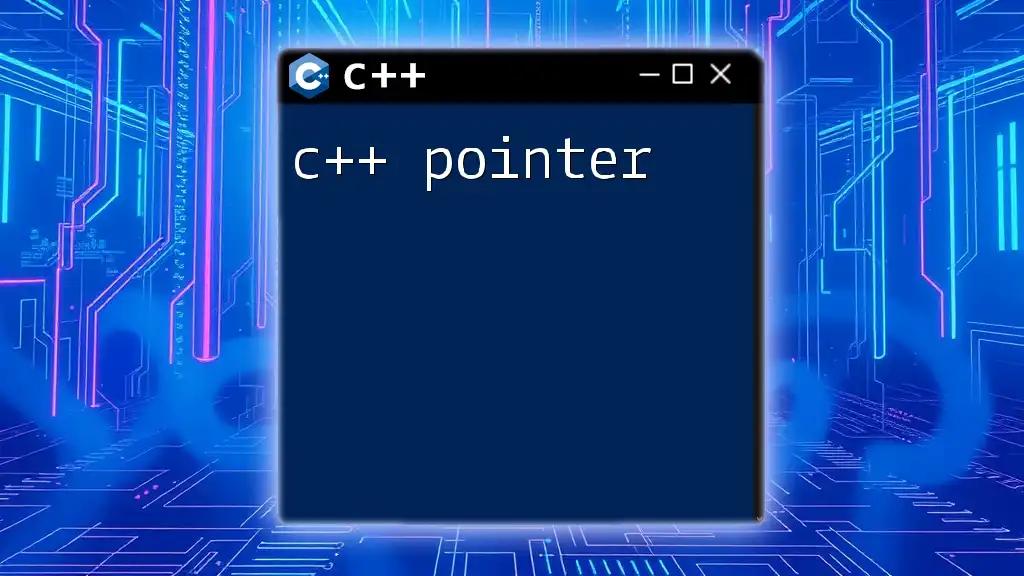
Best Practices when Working with Pointers
Managing Memory Safely
Proper memory management is vital when using pointers. Always ensure that your pointers are initialized before use. Pointers must point to allocated memory, and neglecting this can lead to undefined behavior. Utilize `new` to allocate memory dynamically and remember to use `delete` to free up memory when it’s no longer needed to avoid memory leaks.
Avoiding Common Pitfalls
C++ pointers can be tricky, and several common pitfalls can arise. Here are a few:
- Dangling pointers occur when a pointer still points to memory that has been deallocated. Make sure to set your pointers to `nullptr` after deletion.
- Pointer arithmetic can lead to errors if not used cautiously. Ensure you understand how pointer arithmetic works, especially when navigating through arrays.
- Misunderstanding pointer sizes can lead to performance issues or even crashes. Be mindful of the architecture of the system you are developing for.
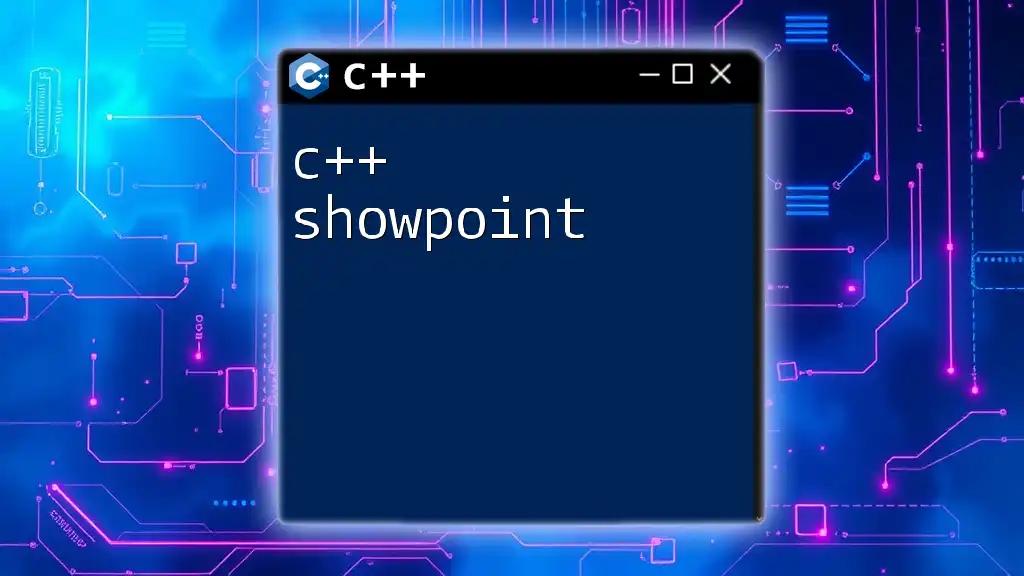
Conclusion
Understanding the `c++ sizeof pointer` is crucial for any C++ programmer. It not only involves knowing how large pointers are in relation to different data types but also entails understanding their underlying architecture. The knowledge acquired through managing pointers goes a long way in writing efficient, safe, and effective code in C++. As you continue to work with pointers, practice is key. Play around with these concepts, and gradually, you'll become proficient in managing pointers and memory in C++.

Additional Resources
For readers looking to expand their understanding of pointers and memory management further, a plethora of resources is available online, including tutorials, books, and courses specifically tailored to advanced C++ topics. Seek out reputable materials to enhance your learning journey!