In C++, the `sizeof` operator can be used to determine the size (in bytes) of a pointer, which varies depending on the architecture (typically 4 bytes on a 32-bit system and 8 bytes on a 64-bit system).
Here’s a code snippet demonstrating its use:
#include <iostream>
int main() {
int* ptr;
std::cout << "Size of pointer: " << sizeof(ptr) << " bytes" << std::endl;
return 0;
}
Understanding Pointers in C++
What is a Pointer?
A pointer in C++ is a variable that stores the memory address of another variable. Pointers are a fundamental aspect of C++ programming, allowing developers to manage memory directly and enhance the performance of applications. They facilitate dynamic memory allocation, complex data structures like linked lists, and efficient array manipulations.
To visualize the concept, imagine that every variable in your program has a unique address in memory, like a house on a street. A pointer acts as a mailing address, directing you to where the data is stored in memory.
The Importance of Pointer Size
Understanding the size of pointers is crucial in C++. Knowing how much memory a pointer consumes helps optimize memory usage, especially when working with large amounts of data. Pointer arithmetic is heavily influenced by pointer size. When performing operations on pointers, the size of the data type they point to determines how the memory addresses are calculated.
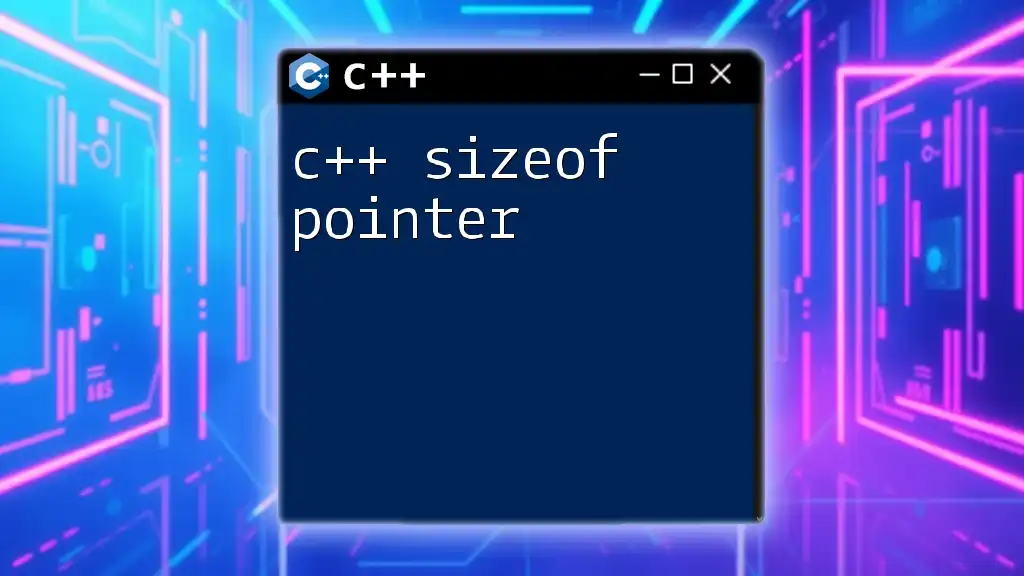
Size of Pointers in C++
The `sizeof` Operator
The `sizeof` operator in C++ is used to determine the size, in bytes, of a variable or data type. This operator is essential when dealing with pointers, as it provides the size of a pointer variable itself.
Here is how you can use the `sizeof` operator to determine pointer size:
size_t size = sizeof(pointerVariable);
For example, consider the following code snippet:
int* ptr;
std::cout << "Size of int pointer: " << sizeof(ptr) << " bytes" << std::endl;
This snippet declares a pointer to `int`, and using `sizeof`, it prints out the size of the pointer.
Typical Sizes of Pointers
Pointer sizes can differ based on the architecture of the system you are working on. On a 32-bit system, a pointer typically occupies 4 bytes of memory. Conversely, on a 64-bit system, a pointer usually takes up 8 bytes.
Here’s a code example demonstrating this:
int* intPtr;
double* doublePtr;
std::cout << "Size of int pointer: " << sizeof(intPtr) << " bytes" << std::endl;
std::cout << "Size of double pointer: " << sizeof(doublePtr) << " bytes" << std::endl;
You will find that both `intPtr` and `doublePtr` output the same number of bytes, reflecting the architecture of your machine rather than the data types they point to.
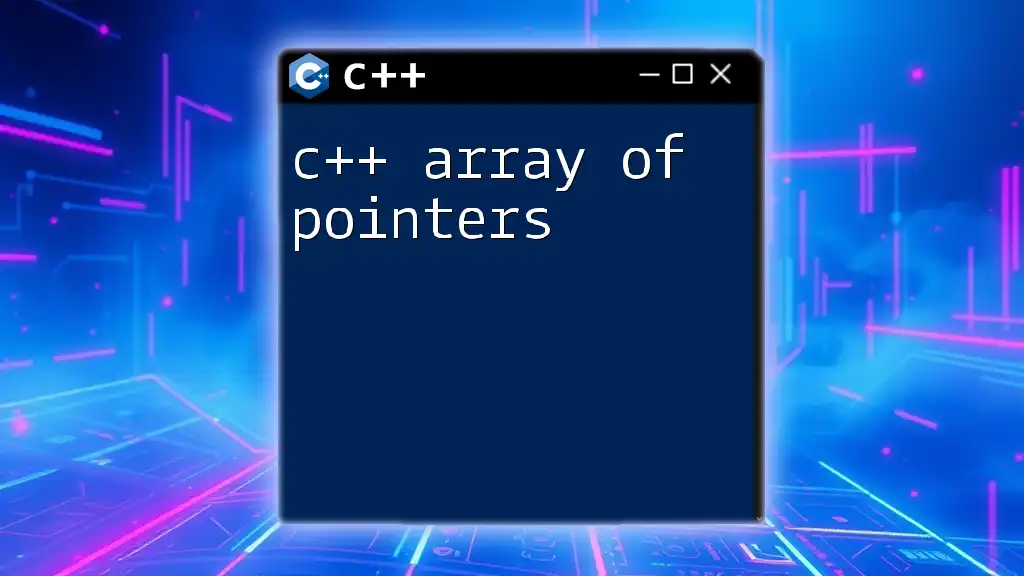
Specifics on Pointer Types
Different Pointer Types
C++ supports various pointer types, each with its characteristics regarding size.
- Standard Pointers: Pointers like `int*`, `char*`, and `double*` have the same size—this size is consistent across these types on any given architecture.
- Void Pointers: A void pointer (`void*`) is a special kind of pointer that can point to any data type. The size of a void pointer is comparable to that of other pointers.
Here’s an example demonstrating the size of a void pointer:
void* voidPtr;
std::cout << "Size of void pointer: " << sizeof(voidPtr) << " bytes" << std::endl;
Pointers to Pointers
A pointer can also point to another pointer, which is referred to as a pointer to a pointer (e.g., `int**`). It allows for more complex data structures and dynamic memory management.
When you calculate the size of a pointer to a pointer, it typically matches the size of a standard pointer, as shown in the following code snippet:
int** ptrToPtr;
std::cout << "Size of pointer to pointer: " << sizeof(ptrToPtr) << " bytes" << std::endl;
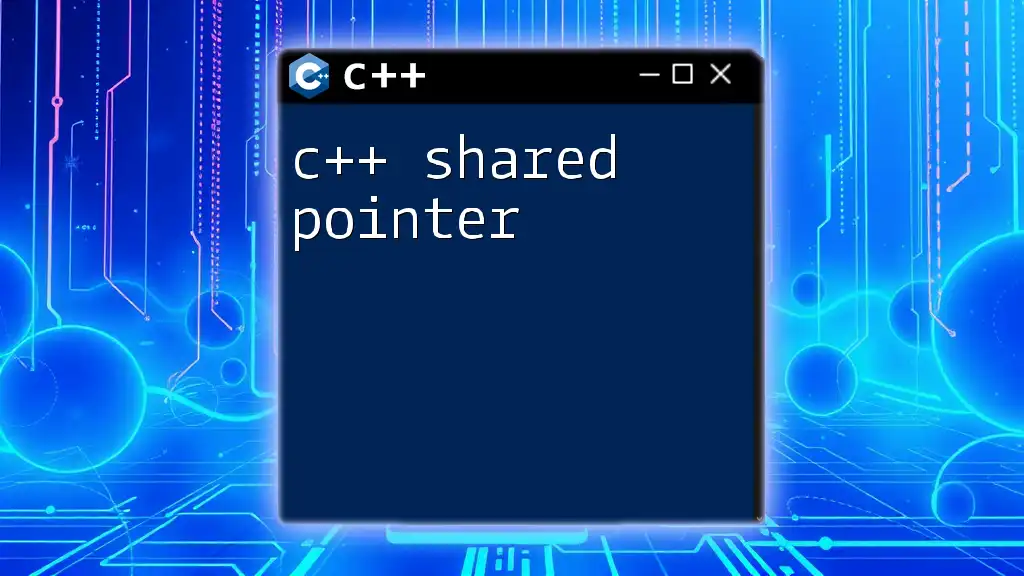
Practical Implications of Pointer Size
Memory Management and Optimization
Pointers play a vital role in dynamic memory allocation. They enable you to allocate memory at runtime using operators like `new` and `delete`. For instance:
int* arr = new int[5]; // Dynamic allocation
delete[] arr; // Deallocation
Understanding the size of pointers ensures efficient memory management by helping prevent memory leaks and ensuring you allocate the correct amount of memory based on your needs.
Best Practices for managing memory with pointers include always initializing pointers before use, checking for null pointers before dereferencing, and properly deallocating any dynamically allocated memory.
Debugging Pointer Issues
When working with pointers, you may encounter a variety of issues, particularly if you are unfamiliar with how they operate in C++. Common problems can include dereferencing null pointers, memory corruption, and leaks.
To debug these issues effectively, you can use functions such as `cout` to check pointer values or leverage debugging tools available in your IDE. Ensuring that pointers are correctly sized and that they point to valid addresses is crucial in eliminating many pointer-related errors.
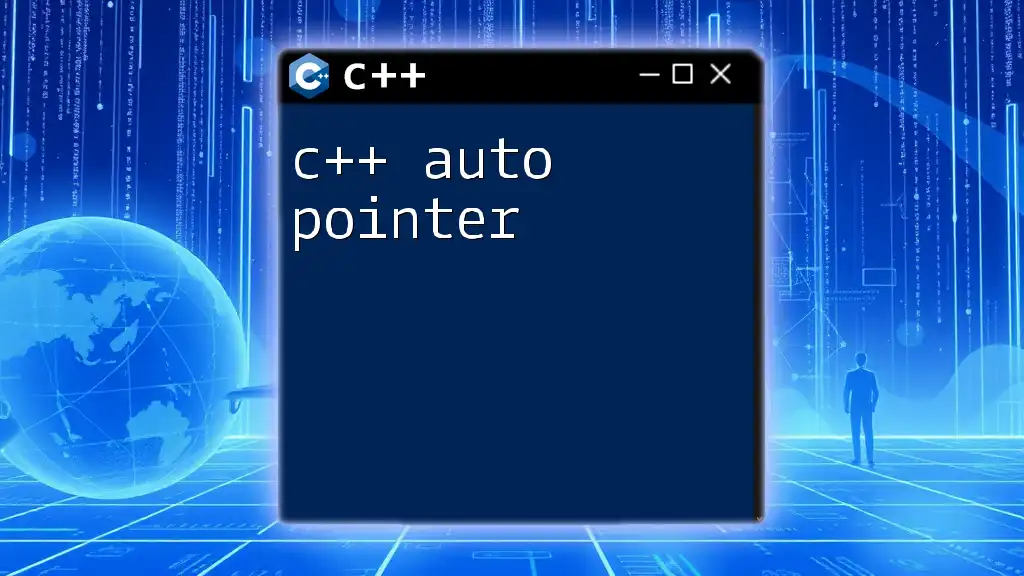
Advanced Concepts
Pointers in Different Data Structures
Pointers are integral to using and managing arrays efficiently. An array name acts as a pointer to its first element, which is essential in pointer arithmetic and manipulation. Consider this code example:
int arr[5];
int* arrPtr = arr; // Pointer to the first element
std::cout << "Size of array pointer: " << sizeof(arrPtr) << " bytes" << std::endl;
Here, `arrPtr` will have the same size as any other pointer, demonstrating the relationship between arrays and pointers in C++.
Function Pointers
Function pointers are another advanced concept that allows you to store the address of a function and call it through that pointer. Knowing the size of function pointers can help you understand their behavior in your applications.
Here’s a practical example:
void (*funcPtr)() = nullptr;
std::cout << "Size of function pointer: " << sizeof(funcPtr) << " bytes" << std::endl;
Function pointers provide great flexibility, allowing for dynamic decision-making in your code by passing different functions to be executed at runtime.
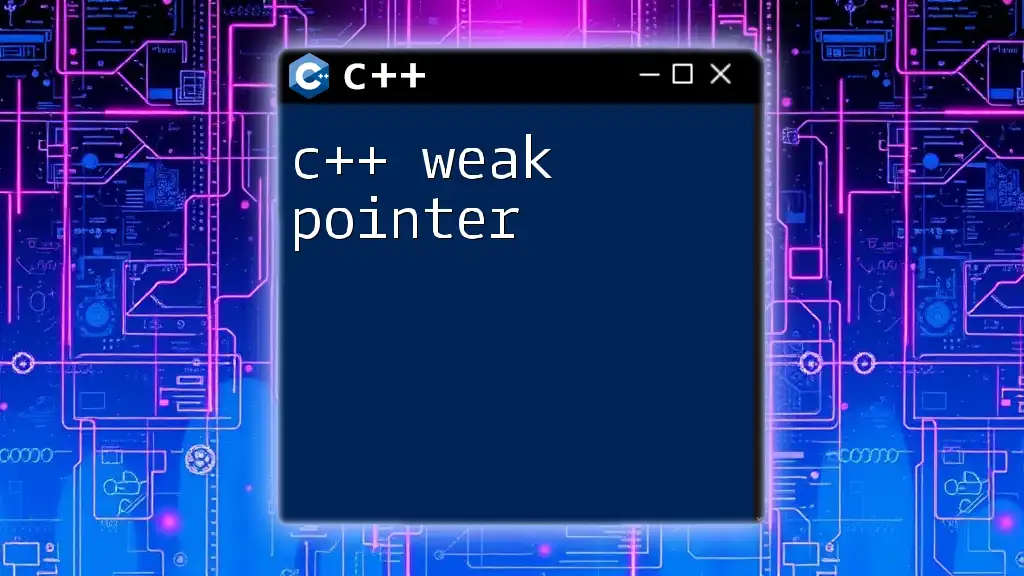
Conclusion
Understanding the C++ size of pointer is vital for efficient memory management and effective software development. By grasping the basics such as pointer types, the `sizeof` operator, and the implications of pointer size on operations, you can design and write robust C++ applications that are both efficient and error-free.
For further reading, consider exploring topics such as advanced memory management techniques, data structures, and algorithms involving pointers. Practice is crucial—try out various pointer examples to solidify your understanding and implement best practices in your programming journey.