The `sizeof` operator in C++ is used to determine the size, in bytes, of a data type or an object, allowing developers to allocate memory efficiently and manage data types effectively.
#include <iostream>
int main() {
int number;
std::cout << "Size of int: " << sizeof(number) << " bytes" << std::endl;
return 0;
}
What is `sizeof`?
The `sizeof` operator in C++ is a key feature that allows developers to determine the size in bytes of various data types and variables. It plays a vital role in memory management and enhances programming efficiency by providing a means to gauge how much memory is required for storing data. Understanding how to utilize `sizeof` can lead to better performance and effective resource allocation in C++ applications.

Syntax of `sizeof`
The syntax for the `sizeof` operator is straightforward. You can use it in two primary forms:
sizeof(type)
sizeof variable
Here, `type` can be any data type, and `variable` refers to a specific variable whose size you want to know.

How `sizeof` Works
The `sizeof` operator evaluates the size of the operand provided to it, which can be a built-in data type, a variable, an array, or a user-defined type such as structs and classes. It returns a value of type `size_t`, which represents the size in bytes. This operator can be extremely useful for ensuring that your program efficiently uses memory.
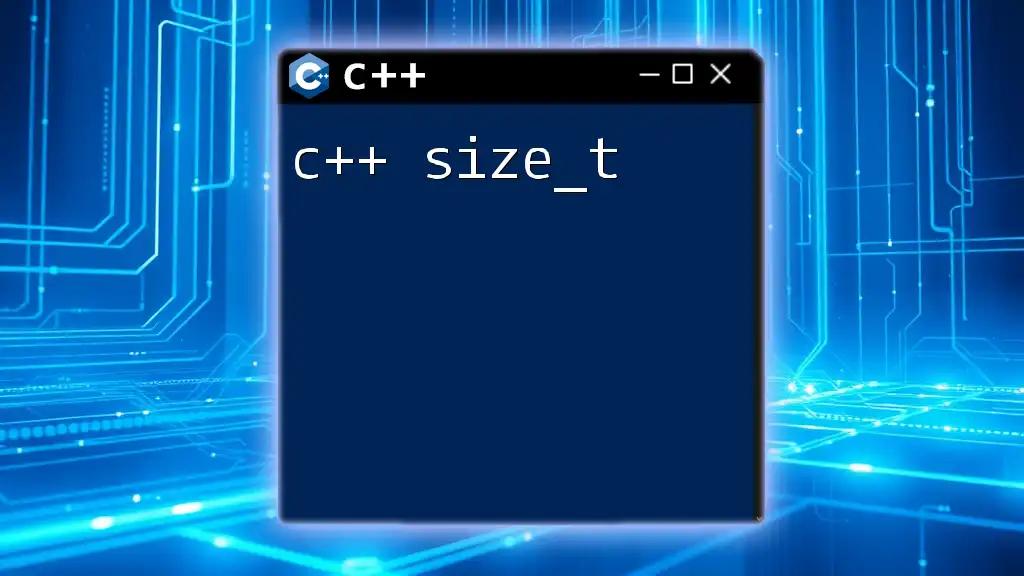
Using `sizeof` with Data Types
Basic Data Types
Understanding how `sizeof` interacts with basic data types forms the foundation of its use in C++. For example:
-
Integers: The size of an integer can be evaluated using:
int num; std::cout << "Size of int: " << sizeof(num) << " bytes" << std::endl;
-
Floating-Point Types: For `float`, the use of `sizeof` would look like:
float f; std::cout << "Size of float: " << sizeof(f) << " bytes" << std::endl;
-
Characters: You can determine the size of a character using:
char c; std::cout << "Size of char: " << sizeof(c) << " bytes" << std::endl;
User-Defined Data Types
Structures
When working with structures, `sizeof` can be used to determine the size of the entire structure, including all its members:
struct Person {
char name[50];
int age;
};
std::cout << "Size of Person: " << sizeof(Person) << " bytes" << std::endl;
This example shows how the size of a structure can vary based on the types of its member variables.
Classes
Similar to structures, classes incorporate data members, constructors, and methods. The size can be determined similarly:
class Car {
public:
int wheels;
float engine_capacity;
};
std::cout << "Size of Car: " << sizeof(Car) << " bytes" << std::endl;
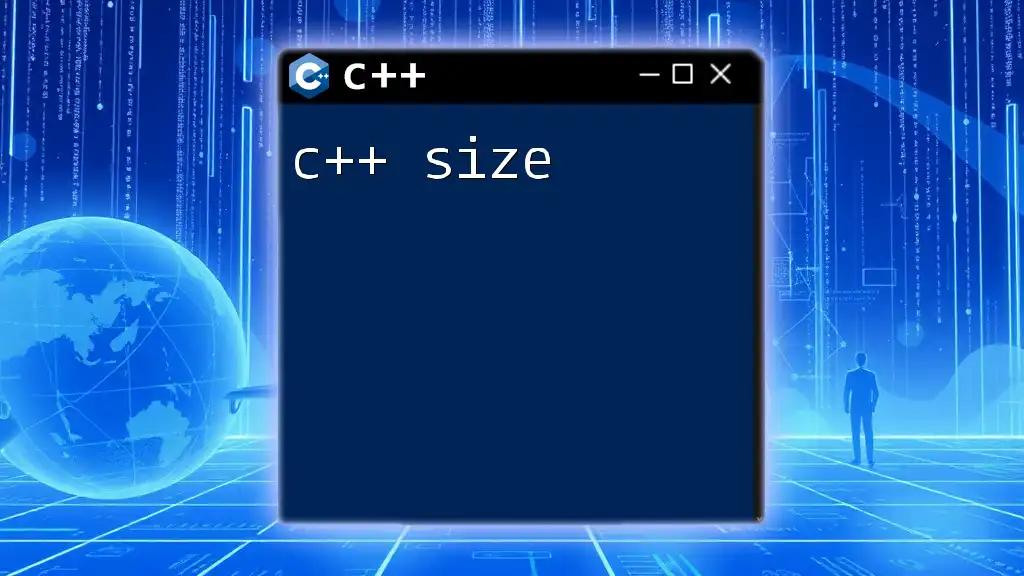
Special Cases in `sizeof`
Arrays
Arrays have special considerations when it comes to `sizeof`. The operator computes the total size of the array based on the size of the individual elements multiplied by the number of elements:
int myArray[10];
std::cout << "Size of myArray: " << sizeof(myArray) << " bytes" << std::endl;
This will output `40 bytes` on most systems, as an `int` typically occupies `4 bytes`.
Pointers
Pointer sizes can vary depending on the architecture of the system (e.g., whether it is a 32-bit or 64-bit system). For instance:
int *p;
std::cout << "Size of pointer: " << sizeof(p) << " bytes" << std::endl;
Here, regardless of the data type it points to, the size of `p` will be either `4 bytes` or `8 bytes`, depending on your system's architecture.
Dynamic Allocation
When working with dynamically allocated memory, `sizeof` behaves differently. For instance:
int *arr = new int[10];
std::cout << "Size of allocated memory: " << sizeof(*arr) << " bytes" << std::endl;
delete[] arr;
In this example, *`sizeof(arr)` returns the size of an `int`, not the total size of the allocated array.

Limitations of `sizeof`
While `sizeof` is a powerful operator, it has certain limitations that developers should be aware of. For example:
- Incomplete Types: You cannot use `sizeof` with incomplete types, such as an incomplete class or struct defined without a body.
- Polymorphism: In the case of polymorphic types, `sizeof` does not account for the dynamic size of the object. This limitation arises because the true size of the object isn't known at compile time, due to the presence of virtual functions.

Practical Applications of `sizeof`
Memory Allocation
One of the most practical applications of `sizeof` is its use in memory allocation functions. For instance, when using `malloc`, it's crucial to specify how much memory to allocate:
int *dynamicArray = (int *) malloc(10 * sizeof(int));
This example ensures that you allocate enough space for ten integers.
Array Bounds Checking
By utilizing `sizeof`, you can enhance safety when working with arrays. You can determine how many elements the array contains, which can help prevent buffer overflows:
for (size_t i = 0; i < sizeof(myArray) / sizeof(myArray[0]); i++) {
std::cout << myArray[i] << std::endl;
}
This will iterate over the elements of `myArray` safely.

Conclusion
The `sizeof` operator is indispensable for C++ developers who want to manage memory efficiently. By comprehensively understanding its capabilities, you can leverage this operator to enhance your code's performance and safety. Experimenting with `sizeof` in different scenarios can lead to valuable insights and improved coding practices.
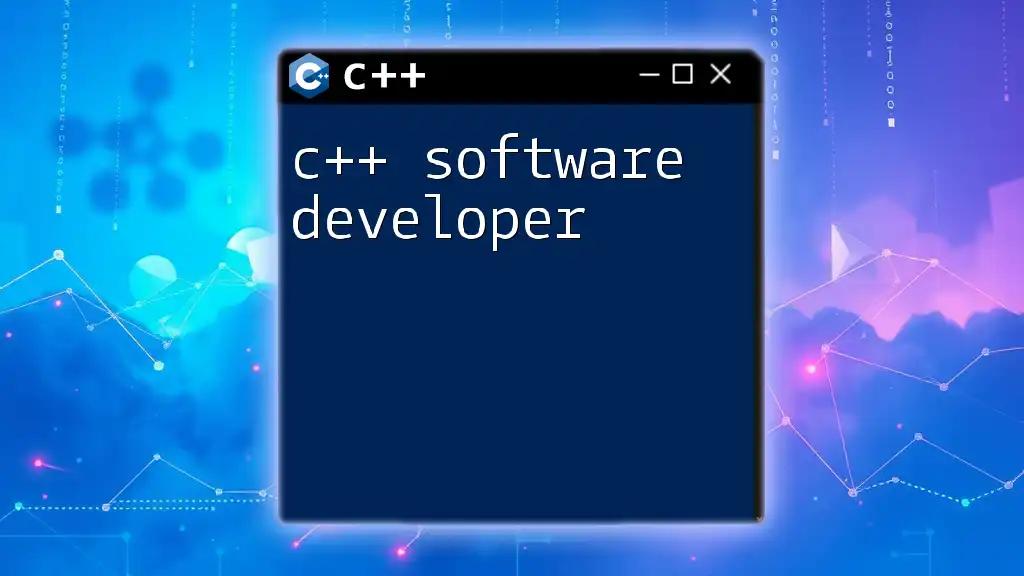
Additional Resources
For those eager to explore further, consider leveraging online compilers and debugging tools. Additionally, you can enhance your knowledge of C++ memory management techniques through recommended books and online courses tailored to C++ programming.
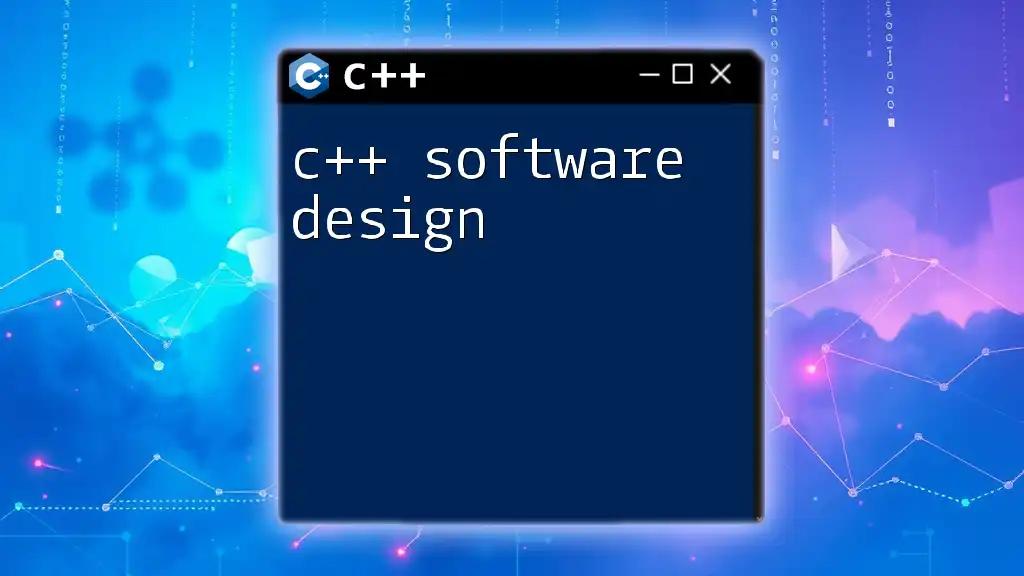
Call to Action
We encourage readers to share their experiences or queries related to the use of `sizeof` in their projects. Your insights can help others learn and grow in their understanding of this core C++ operator.