A C++ Integrated Development Environment (IDE) is a software application that provides comprehensive facilities to programmers for software development in C++, including coding, debugging, and project management.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is an IDE?
An Integrated Development Environment (IDE) is a powerful tool that helps programmers write, test, and debug their code efficiently. It combines various development tools into a single interface, making the coding process smoother and faster. For C++ developers, using an IDE is exceptionally beneficial due to the language’s complexity and extensive features.
Why Choose C++?
C++ is a versatile programming language known for its performance and reliability. It is widely used across various sectors, including game development, systems programming, and applications that require high-speed processing. The ability to write efficient, low-level code while maintaining higher-level programming capabilities makes C++ a popular choice among developers.
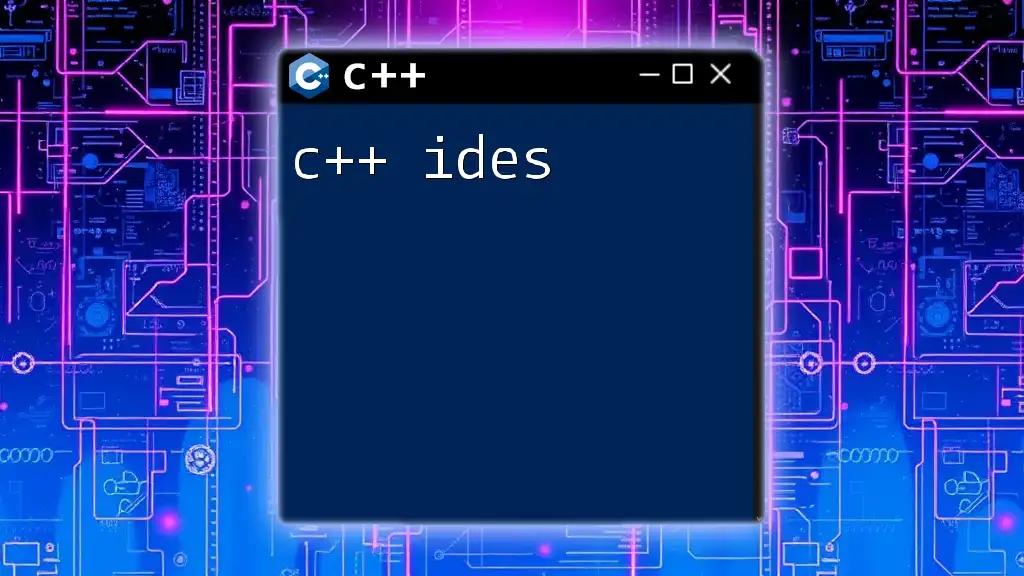
Key Features of an IDE for C++
An effective C++ IDE should come equipped with several essential features that enhance the programming experience and streamline development. Below are some of the crucial features:
Code Editing Tools
One of the main advantages of using an IDE is its robust code editing tools. Features like syntax highlighting, code completion, and real-time linting help developers write cleaner code more efficiently.
-
Syntax Highlighting: Different elements of the code (keywords, variables, comments) are color-coded, making it easier to read and understand the structure at a glance.
-
Code Completion: IDEs often provide suggestions for completing code, reducing typing time and minimizing errors.
-
Real-time Linting: This feature catches syntax errors and potential issues as you write, offering suggestions for correction on-the-fly.
Project Management
A good IDE allows developers to manage their projects effectively. This includes:
-
Organizing Files and Folders: An IDE provides a structured view of your project's files, making it easy to navigate through source code and resources.
-
Version Control Integration: Many IDEs come with built-in Git support, making it easier to track changes and collaborate with teammates.
Build Automation
Build automation is crucial for any development environment. An IDE manages the compilation process, handling tasks such as:
-
Compiling Code Effectively: The IDE should automatically identify changes in your code and compile only what's necessary, speeding up development.
-
Managing Dependencies: Keeping track of dependencies is vital, especially for larger projects. The IDE simplifies this process by handling library installations and updates.
Debugging Capabilities
Debugging is an inevitable part of coding, and an effective IDE provides robust debugging features:
-
Breakpoints and Step-through Debugging: This allows developers to pause the execution of their program at certain lines, examine the state, and continue step-by-step.
-
Variable Inspection and Modification: IDEs enable developers to view and change variable values during debugging, facilitating quick problem resolution.
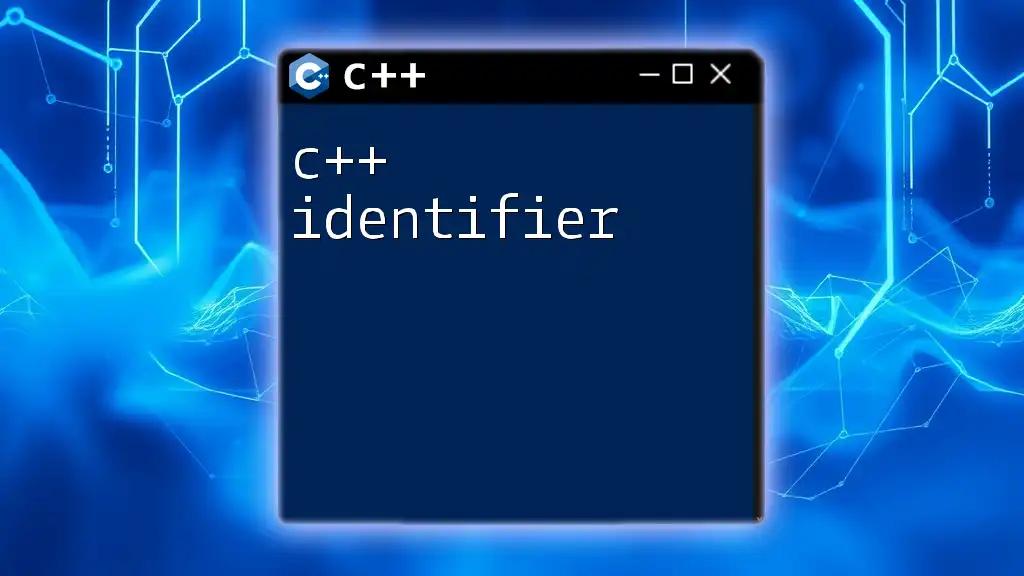
Popular C++ IDEs
Several IDEs are specifically designed for C++ development, each with its own unique features and advantages:
Visual Studio
Visual Studio is a feature-rich IDE that supports C++ development with advanced tools.
-
Overview and Installation: Available on Windows, Visual Studio can be installed from Microsoft's official website. During installation, choose the C++ development workload.
-
Key Features: It offers extensive debugging tools, intuitive interface, and seamless integration with Azure DevOps.
-
Example: To create a simple C++ project, user can follow these steps:
- Launch Visual Studio.
- Select "Create a new project."
- Choose "Console App" under C++ category.
- Write your C++ code in the editor and run it directly.
Eclipse CDT
Eclipse CDT is an open-source IDE that provides various plugins for C++ development.
-
Overview and Installation: It can be downloaded from the Eclipse website and requires minimal setup with the C/C++ Development Tooling (CDT) plugin.
-
Key Features: Eclipse comes with a powerful set of tools for code editing, building modules, and managing projects.
-
Example: To configure and run a basic C++ application:
- Open Eclipse and create a new C++ project.
- Add your code in the source file and build your project.
- Run the application from the IDE.
Code::Blocks
Code::Blocks is a free and open-source IDE that is lightweight yet powerful.
-
Overview and Installation: Downloadable from the Code::Blocks website, it provides a quick installation process.
-
Key Features: Its customizable interface and integrated debugger streamline the development process.
-
Example: Setting up a new project involves:
- Launching Code::Blocks and selecting "Create a new project."
- Choosing the "Console Application" option.
- Writing your C++ code and recompiling whenever changes are made.
CLion
CLion is a modestly priced IDE from JetBrains, known for its smart code analysis capabilities.
-
Overview and Installation: It can be obtained from the JetBrains website, with options for a free trial.
-
Key Features: CLion includes powerful code navigation, refactoring tools, and integrated support for CMake.
-
Example: Refactoring C++ code in CLion is seamless:
- Right-click on the function name.
- Select "Refactor" and choose your desired refactoring option, such as renaming.
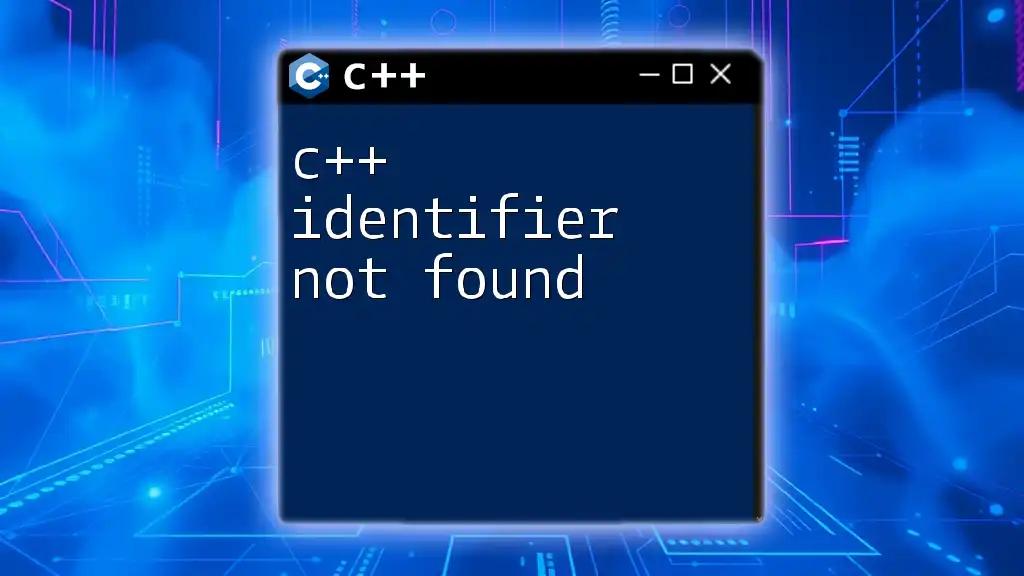
Comparison of Popular C++ IDEs
When selecting a C++ IDE, comparing options is crucial. Here are some key factors:
User Interface and Experience
Different IDEs provide various user interfaces. A user-friendly layout can significantly enhance productivity, while an overly complicated interface can hinder development.
Performance
The speed of compilation and execution varies across IDEs. For instance, some IDEs like Visual Studio may use more system resources, so consider your environment when making a choice.
Community and Support
A strong community around an IDE ensures ample resources for troubleshooting. IDEs like Visual Studio and Eclipse have extensive online documentation and forums that users can leverage for help.
Pricing
While some IDEs are free, others like CLion come with a price. The choice often depends on whether you prioritize advanced features or availability of free tools.
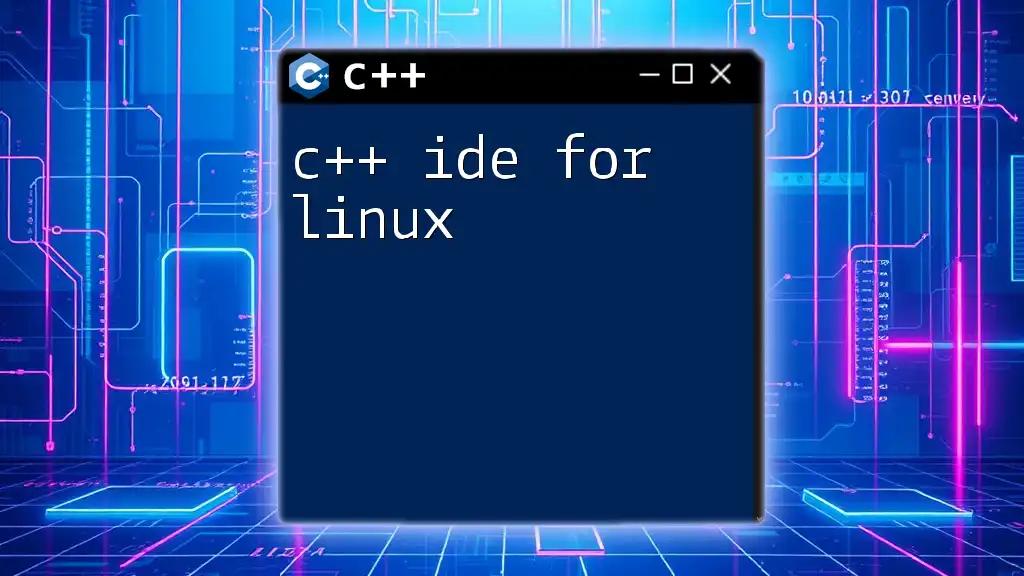
Getting Started with Your First C++ Project
To start your C++ journey, installing your chosen IDE is the first step. Here’s a brief installation guide for Visual Studio:
- Visit the Visual Studio website and download the installer.
- Run the Installer and select the "Desktop development with C++" workload.
- Complete the installation and launch Visual Studio.
Writing Your First C++ Program
It’s time to write your first simple C++ program. Below is an example of a classic “Hello World” program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Building and Running the Program
Once your code is complete, you can build and run the program directly within the IDE. Simply click on the Run button, and the output will display in the console window.
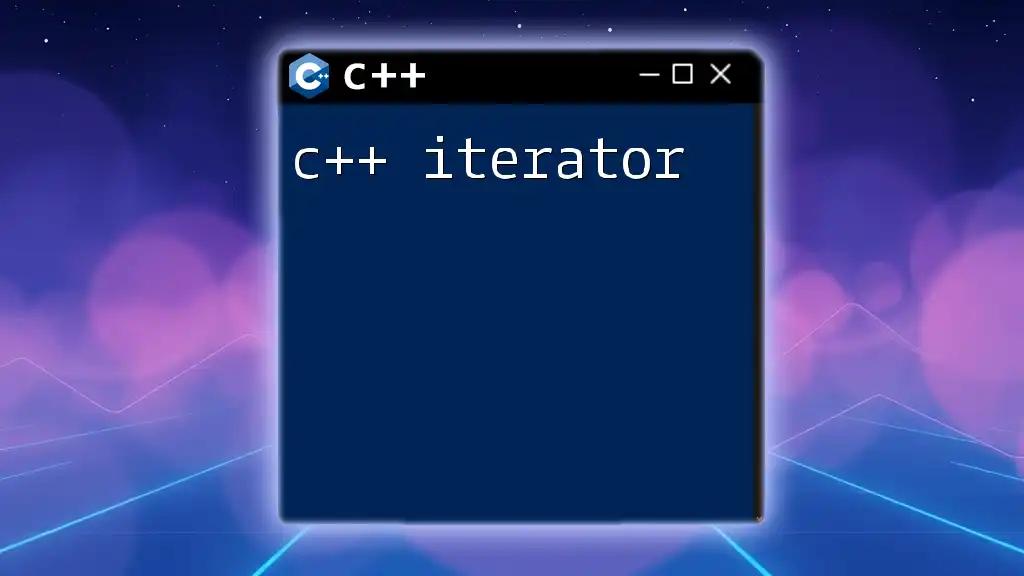
Optimizing Your Development Workflow
To maximize productivity within your C++ IDE, consider the following tips:
Shortcuts and Hotkeys
Using keyboard shortcuts can save time. Familiarize yourself with the most essential shortcuts in your IDE to enhance your coding efficiency.
Customizing the IDE
Most IDEs allow customization of themes and plugins, which can significantly improve your user experience. Explore available options and tailor the IDE to your preferences.
Effective Debugging Strategies
Debugging can be daunting, but having a methodical approach helps. Familiarize yourself with the debugging tools of your chosen IDE and utilize breakpoints to isolate issues quickly.
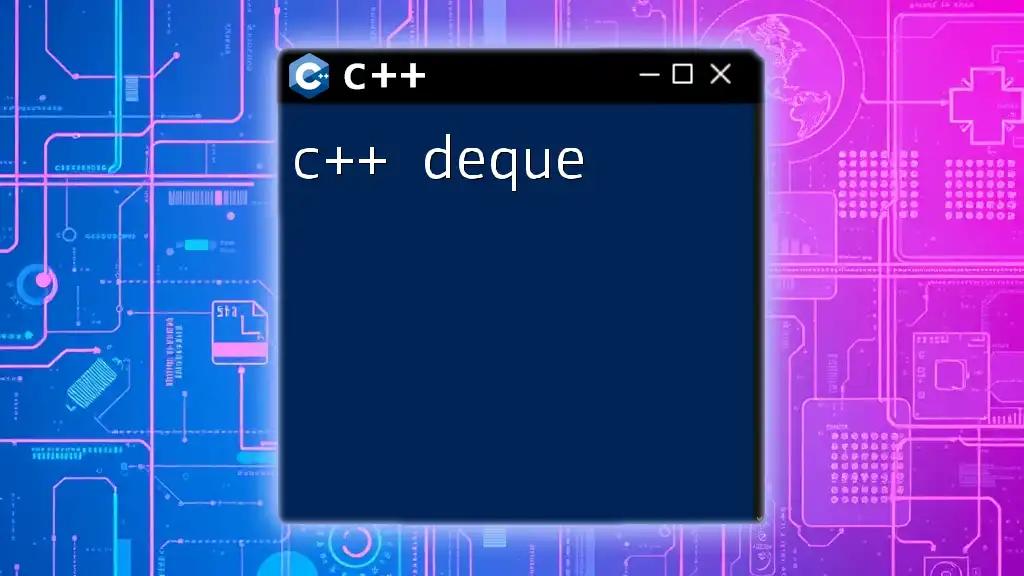
Conclusion
Using a C++ IDE not only enhances your coding experience but also significantly improves your productivity. It consolidates all the essential tools you'll need into a single interface, making the development process smoother and more organized. As you embark on your C++ programming journey, take the time to explore different IDE options, gather resources, and find the setup that best suits your development style.

FAQs
What is the best C++ IDE?
The best C++ IDE varies depending on your needs. Visual Studio is highly recommended for Windows users, while CLion and Eclipse CDT are preferred for cross-platform development.
Can I use C++ in other editors?
Yes, many developers use text editors like Sublime Text or VS Code with appropriate C++ extensions. However, they may lack integrated features that IDEs offer.
Is there a free C++ IDE?
Absolutely! Options like Code::Blocks and Eclipse CDT are free and provide excellent features for beginners and seasoned developers alike.