C++ indexing refers to accessing elements of an array or a data structure using an index, which starts from zero for the first element.
#include <iostream>
int main() {
int numbers[] = {10, 20, 30, 40, 50};
std::cout << "The first element is: " << numbers[0] << std::endl; // Accessing the first element
return 0;
}
Understanding Indexing in C++
What is Indexing?
Indexing in programming is the process of accessing elements in data structures through their positions. In C++, indexing is a critical concept that allows programmers to efficiently manage and manipulate collections of data. The ability to retrieve, modify, or navigate through data based on positional references is foundational to many algorithms and data structures.
Types of Indexing in C++
Indexing can generally be categorized into two types:
-
Static Indexing: This type of indexing refers to fixed-size data structures, such as arrays. The size is determined at compile time and does not change during execution. In static indexing, the indices are typically integer values that specify the location of an element within the data structure.
-
Dynamic Indexing: This refers to the use of dynamic data structures like vectors, lists, and maps, where the size can change during program execution. Dynamic indexing allows for more flexibility, enabling programs to grow or shrink their data storage as needed.
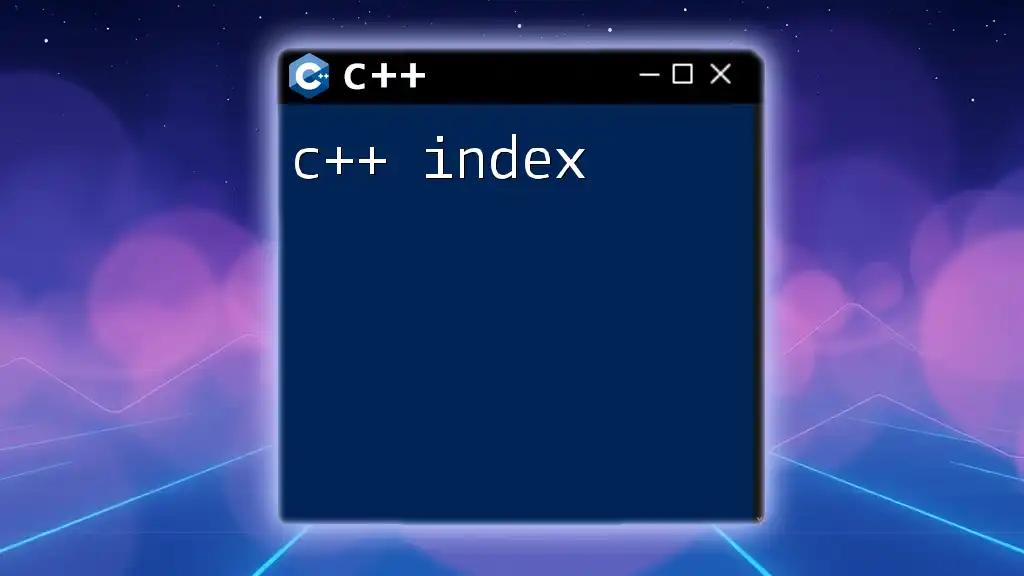
Array Indexing
Introduction to Arrays
Arrays are a fundamental data structure in C++, used to store a collection of elements of the same type. They provide a method to group related data together, making it easier to manage large datasets. While arrays can be incredibly efficient, understanding how to properly index them is essential to avoid errors and ensure optimal performance.
Accessing Array Elements
Elements in an array can be accessed through their indices, which start at 0. For example, in a one-dimensional array:
int arr[] = {10, 20, 30, 40};
cout << arr[0]; // Output: 10
This code retrieves the first element in the array. It is important to remember that indexing beyond the bounds of the array can lead to undefined behavior.
Multidimensional Arrays
Multidimensional arrays extend the concept of arrays into additional dimensions. For instance, in a two-dimensional array, you can think of it as a matrix, where each element can be accessed using two indices: one for the row and one for the column.
Here’s how you can access elements in a two-dimensional array:
int matrix[2][3] = {{1, 2, 3}, {4, 5, 6}};
cout << matrix[1][2]; // Output: 6
In this example, `matrix[1][2]` accesses the element in the second row and third column.

Using Vectors for Indexing
What are Vectors?
Vectors are a part of the C++ Standard Template Library (STL) that provide a dynamic array-like structure. Unlike traditional arrays, vectors can grow and shrink in size, which makes them more versatile for handling containers of data that may change in size over time.
Indexing with Vectors
Accessing elements in a vector is similar to accessing elements in an array. Each element is indexed using an integer value, starting from 0. Here’s an example:
vector<int> vec = {10, 20, 30};
cout << vec[1]; // Output: 20
Dynamic Resizing
Vectors automatically manage memory for you. When you add new elements using the `push_back` method, the vector resizes as necessary. For example:
vec.push_back(40);
cout << vec[3]; // Output: 40
In this case, after pushing back `40`, the vector increases its size, and the new element can be accessed seamlessly.
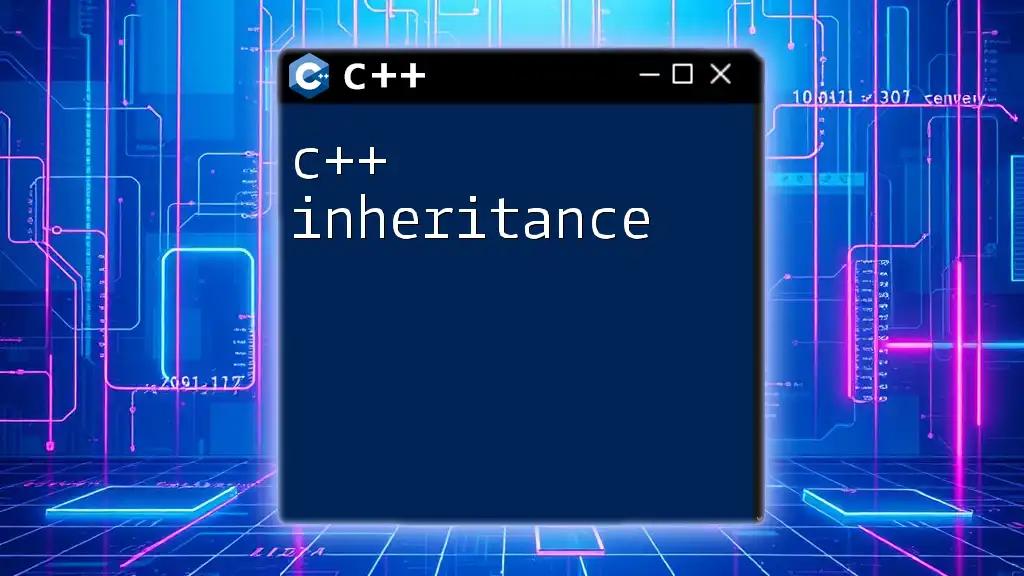
String Indexing
Understanding Strings in C++
In C++, a string is essentially a sequence of characters. Just like arrays and vectors, strings can be indexed, allowing developers to access or modify individual characters. Understanding string indexing is essential for tasks that involve reading or manipulating text.
Accessing Characters in a String
Accessing characters in a string follows similar principles as array indexing. For example:
string str = "Hello";
cout << str[0]; // Output: H
The above code retrieves the first character of the string, which is `H`.
String Manipulation
Strings in C++ can also be modified through indexing. Here’s how you can change a character in a string:
str[1] = 'a';
cout << str; // Output: Halo
This line modifies the second character of the string from `e` to `a`, showcasing the flexibility of C++ string indexing.
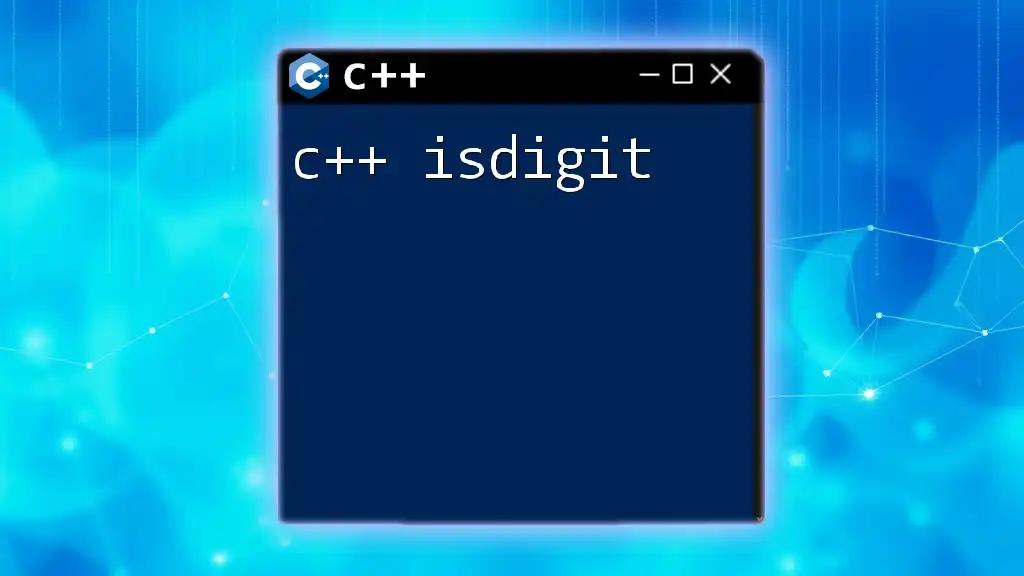
Indexing with Maps and Sets
Introduction to Maps
Maps in C++ are associative containers that store elements in key-value pairs like a dictionary. Indexing in maps is done through keys instead of numerical indices, providing a way to look up values based on something other than their position.
Accessing and Modifying Map Elements
Here’s how you can create a map and access elements using indexing:
map<string, int> age;
age["John"] = 25;
cout << age["John"]; // Output: 25
In this example, the key `"John"` is used to access the value `25`.
Indexing in Sets
Sets are another C++ STL container that stores unique elements. Although sets are unordered, you can still check for the presence of an element using indexing-like behavior through the `find` method.
set<int> numbers = {1, 2, 3};
if (numbers.find(2) != numbers.end()) {
cout << "Found 2";
}
In this code snippet, the `find` function checks for the presence of the number `2` in the set and prints a message if found.

Best Practices for Efficient Indexing
Choosing the Right Data Structure
The type of data structure you choose for storing data significantly influences performance. When c++ indexing is involved, consider the necessity for dynamic resizing, element uniqueness, or the need for fast access times. Choosing the correct data structure aligns the indexing method with the specific requirements of your application.
Avoiding Common Pitfalls
One common pitfall in indexing is going out of bounds. This can lead to undefined behavior, especially in static indexing scenarios like arrays. Always ensure that your indices are within the valid range of the container.
Performance Considerations
Accessing elements via indexing can differ in time complexity. While arrays and vectors provide O(1) time complexity, accessing elements in maps and sets can take longer, generally around O(log n) due to their underlying implementations. Always be mindful of this when designing algorithms that rely heavily on indexing.
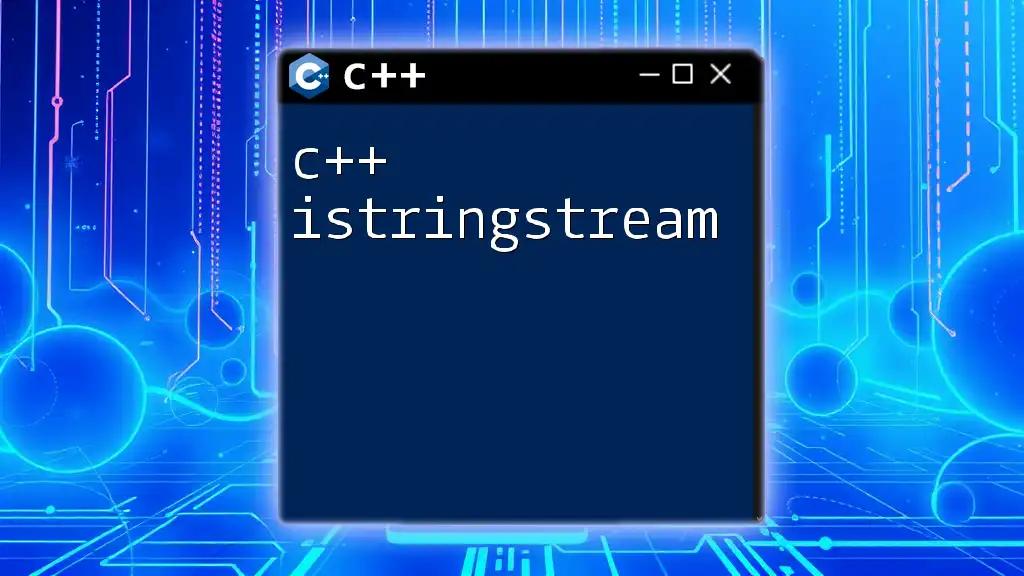
Conclusion
Understanding c++ indexing is a vital skill that enhances your ability to manage data efficiently. Whether you are working with simple arrays, dynamic vectors, or more complex data structures like maps and sets, proper indexing guarantees that your programs run effectively and accurately. Practice with different data structures and become familiar with their indexing methods to sharpen your programming skills.
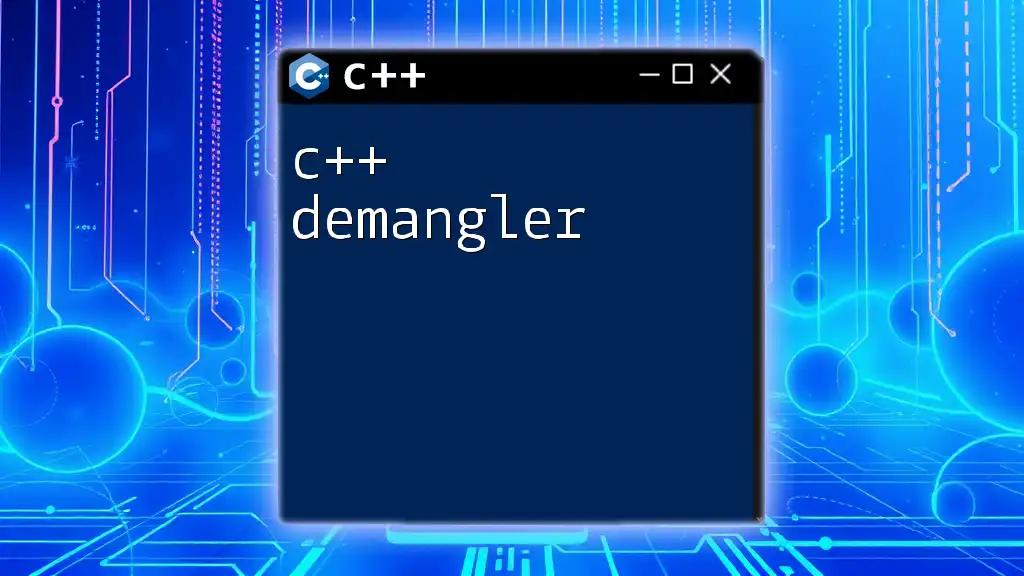
Additional Resources
For further learning, refer to C++ documentation on STL containers and data structures. Online courses, tutorials, and recommended books can provide deeper insights into effective coding practices in C++. Investing time in understanding these elements will significantly contribute to your programming proficiency.