C++ modding refers to the practice of modifying or extending existing software applications or games using C++ code to enhance functionality or add new features.
Here's a simple code snippet that illustrates how to modify a class in C++:
class Game {
public:
void start() {
std::cout << "Game started!" << std::endl;
}
};
class ModdedGame : public Game {
public:
void start() override {
std::cout << "Modded game started with new features!" << std::endl;
// Additional modifications or features can be added here
}
};
What is C++ Modding?
C++ modding refers to the practice of modifying games and software using the C++ programming language. This involves altering the game's code or adding new features to enhance gameplay or user experience. Modding is particularly significant in the gaming community, where it allows talented coders to create new content, fix bugs, or customize existing gameplay.
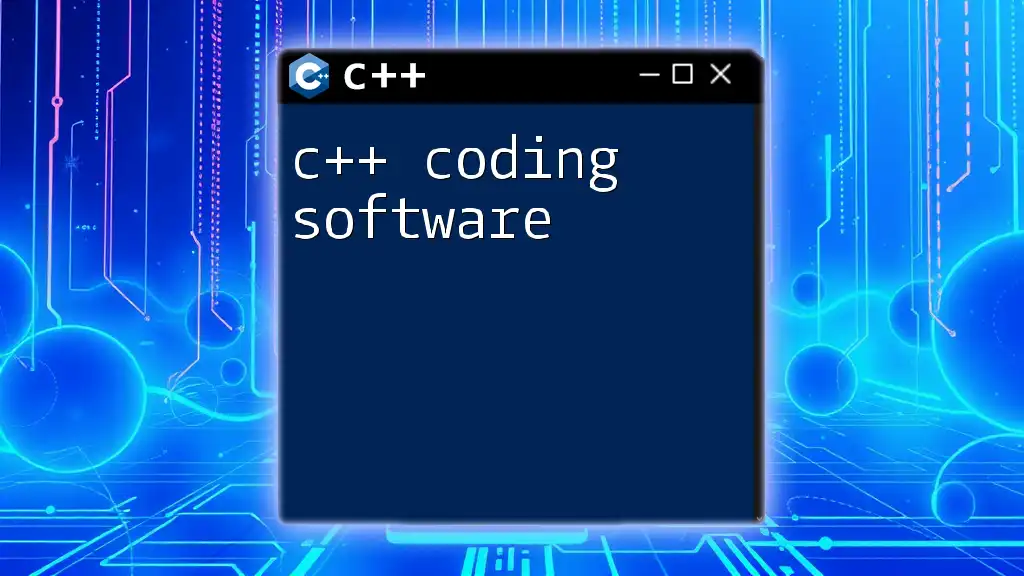
Why Mod with C++?
There are several compelling reasons to use C++ for modding:
- Performance: C++ is a compiled language, offering high performance, which is crucial for real-time applications like games.
- Low-level Memory Management: With powerful features like pointers and direct memory access, C++ provides modders the ability to make significant changes that may not be possible in higher-level languages.
- Extensive Libraries: C++ has a rich ecosystem of libraries that facilitate various functionalities, from graphics rendering to handling audio.
Popular mods, such as those found in games like Skyrim or Counter-Strike, are often built using C++. These mods can range from simple tweaks to extensive additions, allowing for a richer gaming experience.
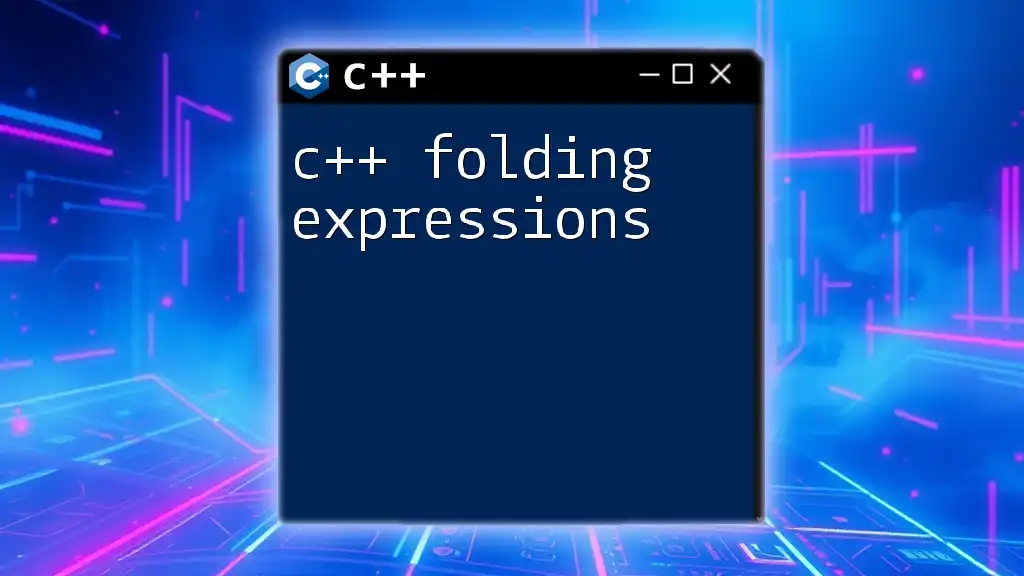
Getting Started with C++ Modding
Setting Up Your Environment
To start modding with C++, you need an Integrated Development Environment (IDE). Popular choices include Visual Studio and Code::Blocks.
- Installation Instructions: Download your chosen IDE and follow the setup instructions. Ensure you install the C++ components during the setup process.
- Configurations: After installation, configure your IDE to point to the necessary SDKs and libraries required by the game engine you are targeting.
Understanding the Basics of C++
Having a foundational understanding of C++ is vital for effective modding. The key aspects of C++ to grasp include:
- Basic Syntax: Familiarize yourself with C++ syntax, including variables, functions, loops, and conditional statements.
- Object-Oriented Programming (OOP): Modding often involves creating and manipulating objects, so understanding concepts like classes, inheritance, and polymorphism is essential.
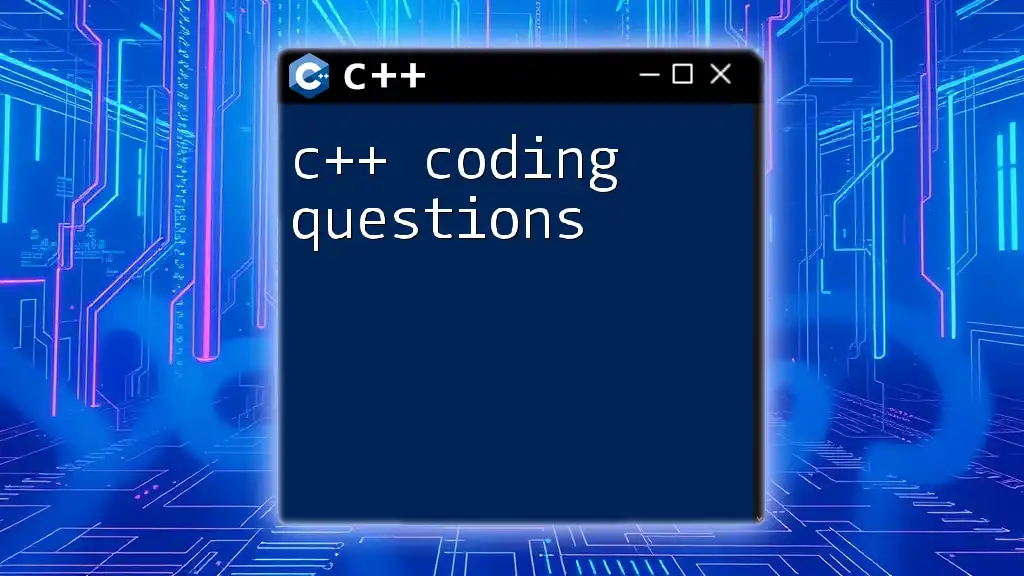
Core Concepts in C++ Modding
Understanding Game Engines and APIs
When modding, understanding the game engine at hand is crucial. Popular engines, such as Unreal Engine and Unity, often utilize C++ for performance-critical systems.
- APIs: C++ modding commonly involves using specific APIs (Application Programming Interfaces) that allow you to interact with game components. These enable modifications like altering graphics, gameplay mechanics, or even sound.
Working with Libraries
Libraries can enhance your modding experience by providing additional functionalities without the need to code everything from scratch. Notable examples include:
- SDL: Simple DirectMedia Layer, commonly used for graphics and audio manipulation. For instance, using SDL, you can easily handle window creation and rendering.
#include <SDL.h>
int main(int argc, char* argv[]) {
SDL_Init(SDL_INIT_VIDEO);
SDL_Window* window = SDL_CreateWindow("My Mod", SDL_WINDOWPOS_UNDEFINED, SDL_WINDOWPOS_UNDEFINED, 800, 600, SDL_WINDOW_SHOWN);
// Clean up
SDL_DestroyWindow(window);
SDL_Quit();
return 0;
}
- SFML: Simple and Fast Multimedia Library, also geared towards handling multimedia and displaying graphics.
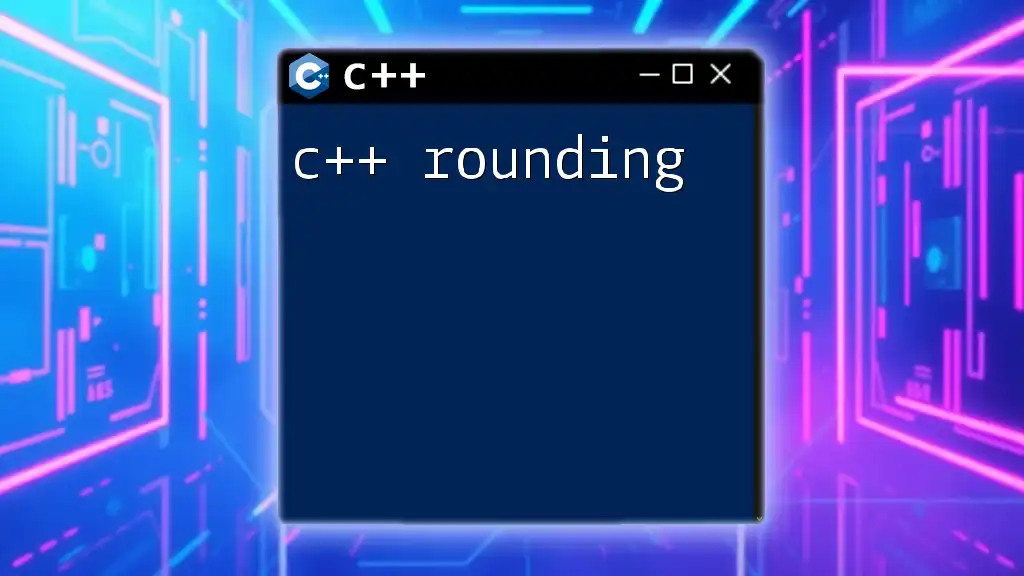
Advanced Modding Techniques in C++
Memory Manipulation
Understanding how memory works is fundamental for creating effective mods. In C++, pointers and references allow you to manipulate memory directly.
For example, when you want to change an in-game character's health, you can manipulate the memory address where that health value is stored.
int* playerHealthAddress = (int*)0x12345678; // Example memory address
*playerHealthAddress = 200; // Set health to 200
This method can yield powerful results but must be approached with caution to avoid crashes or undefined behaviors.
Using Hooks and DLL Injection
Hooks are techniques used to intercept calls or messages between different parts of a program. DLL (Dynamic Link Library) injection is a common practice for modifying a game's code while it is running.
Creating a simple hook can look like this:
// Example of a simple function hook
typedef void (*OriginalFunctionType)();
OriginalFunctionType OriginalFunction;
void HookFunction() {
// Code to run before the original function
OriginalFunction(); // Call the original function
// Code to run after the original function
}
void SetupHook() {
// Code to inject hook into the target process
}
This allows modders to add functionality or change how game mechanics work without requiring access to the original source code.
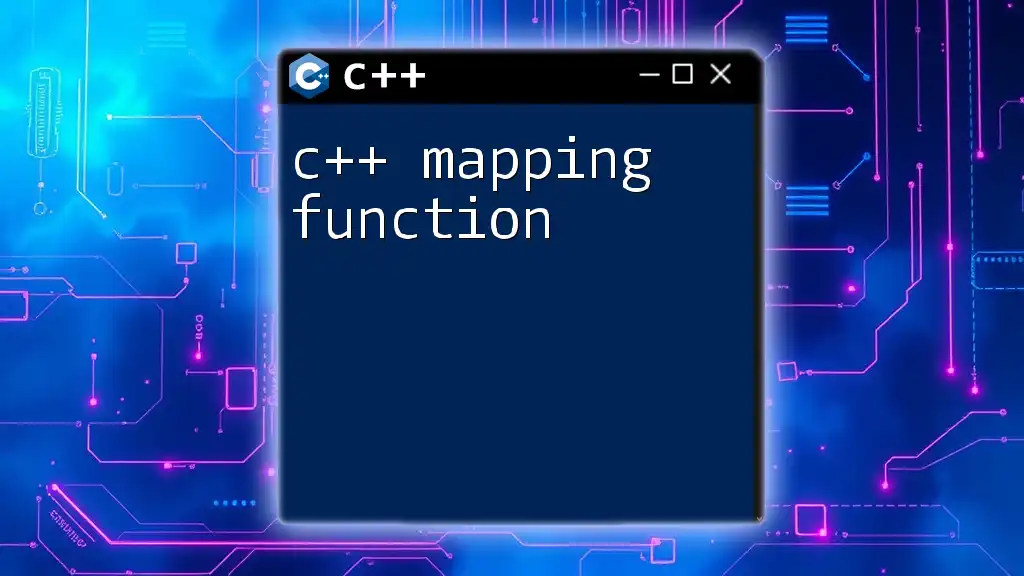
Creating Your First Mod
Choosing Your Target Game
Selecting the right game is crucial for your modding journey. Consider games with established modding communities and developer support, as these often provide the most resources and tools.
Building a Simple Mod: A Step-by-Step Guide
To create your first mod, follow these steps:
- Project Setup: Create a new project in your C++ IDE, ensuring you link any necessary libraries.
- Code Example: Here’s a basic example of a mod that increases player speed in a hypothetical game.
// Simple mod to increase player speed
void IncreasePlayerSpeed(float increaseAmount) {
float* playerSpeedAddress = (float*)0xABCDEF00; // Replace with the actual address
*playerSpeedAddress += increaseAmount; // Increment player speed
}
Compile and test the mod within the game environment.
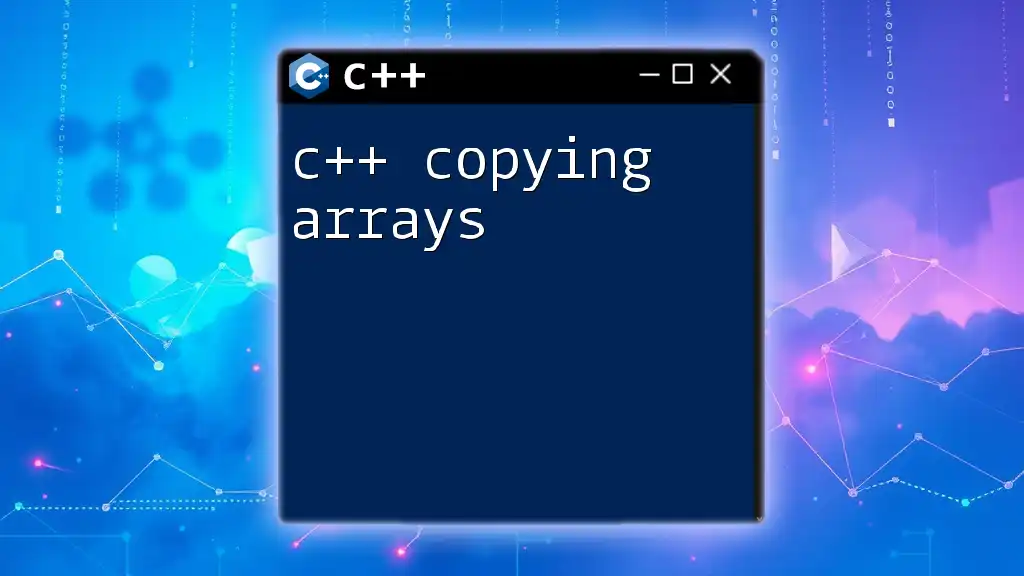
Testing and Debugging Your Mod
Introduction to Debugging Techniques
Debugging is an integral part of the development process. Using tools available in your IDE, like breakpoints and watches, helps you troubleshoot issues effectively. Common errors in C++ might include segmentation faults, which occur when accessing invalid memory.
Testing Your Mod: Best Practices
To ensure a successful mod, test it thoroughly under different conditions. Gather player feedback to further refine and enhance the experience.
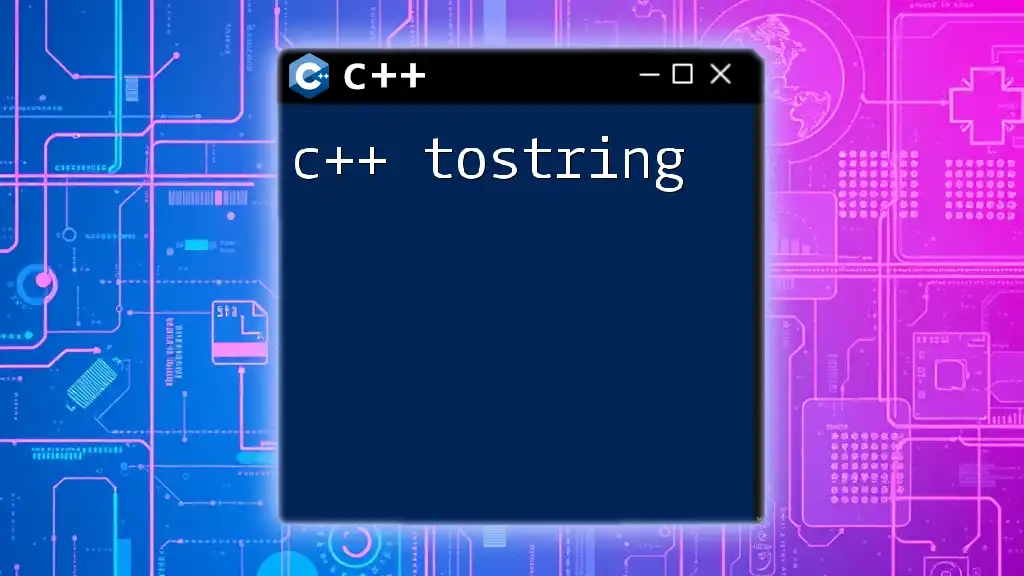
Distribution and Community
Packaging Your Mod for Distribution
Once your mod is ready, it's essential to package it well. Organize files in a meaningful way and provide clear documentation on installation and usage. Consider creating an installer to automate the installation process.
Engaging with the Modding Community
Sharing your mod is crucial for gaining recognition and feedback. Platforms like Nexus Mods and GitHub allow you to showcase your work to a broader audience. Engage with the community by responding to feedback, participating in discussions, and collaborating with other modders.
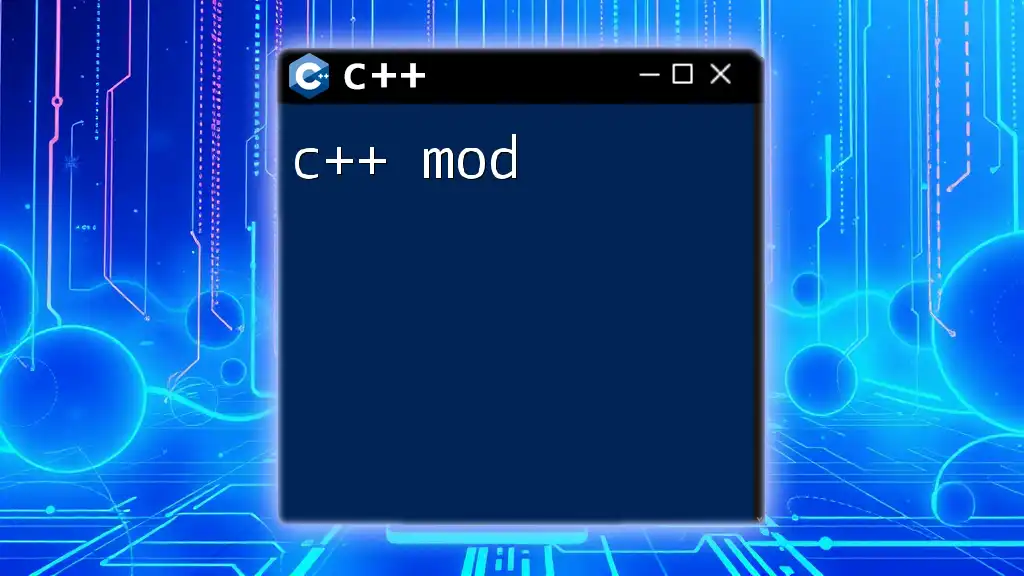
Conclusion
In summary, C++ modding provides a vast landscape of opportunities for creativity and enhancement in gaming and software. By mastering C++ and understanding the concepts discussed, you'll be well on your way to creating impactful mods. Remember to keep learning and exploring new techniques – the modding community is always evolving, and so should you!
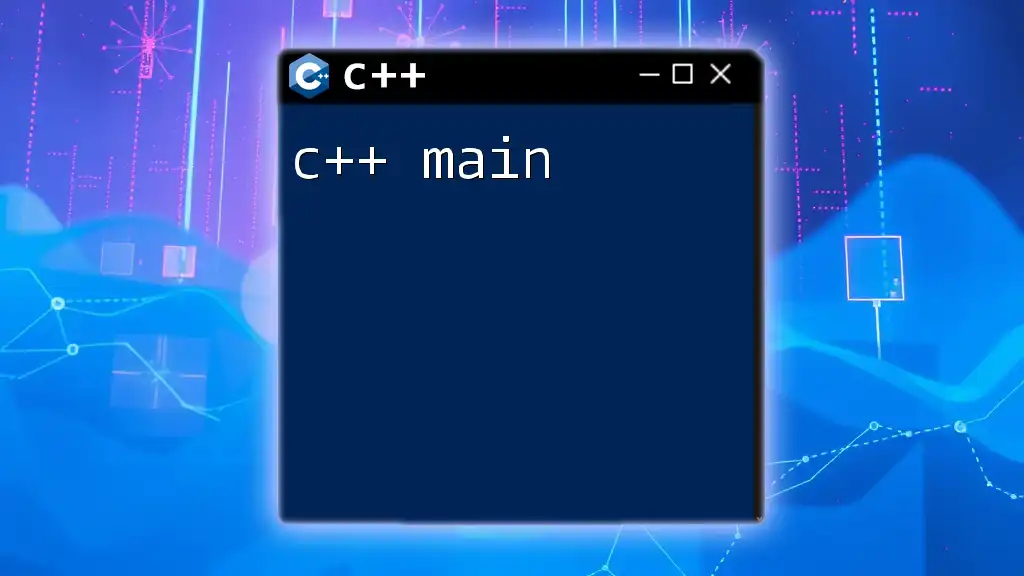
Additional Resources
To continue your journey in C++ modding, consider exploring the following resources:
- C++ Documentation: Official C++ websites and guides for syntax and standards.
- Suggested Reading: Books like "Effective C++" and online courses dedicated to C++ programming and game development.
With passion and persistence, you'll unlock the potential of C++ modding to captivate and inspire gamers around the world!