C++ linking is the process of combining multiple object files or libraries into a single executable, allowing your program to reference code from different sources.
Here's a simple example of how to link external libraries in a C++ project:
g++ main.cpp -o my_program -lm
In this example, `main.cpp` is compiled and linked with the math library (`-lm`), creating an executable named `my_program`.
Understanding C++ Linking
What is Linking?
Linking is a crucial step in the software development process that involves combining various pieces of code and data into a single, executable program. In the context of C++, linking allows developers to integrate multiple files, libraries, and resources, enabling them to build larger and more complex applications efficiently.
The significance of linking in C++ cannot be overstated. It facilitates modular programming, allowing developers to divide their code into manageable segments, which enhances readability and maintainability. Additionally, linking promotes code reuse and supports better organization of significant projects, making it easier to manage dependencies and share code among multiple projects.
Types of Linking
Static Linking
Static linking involves incorporating all library code directly into the executable file during the compile-time process. This approach results in a standalone executable that does not rely on external libraries when running.
Example Scenario: A developer might choose static linking when creating a command-line tool that must run on a target machine without ensuring the necessary libraries are installed.
Here’s a simple example of static linking in a C++ application:
// main.cpp
#include <iostream>
#include "mylib.h"
int main() {
std::cout << "Result: " << myFunction() << std::endl;
return 0;
}
// mylib.cpp
int myFunction() {
return 42;
}
To compile and link statically, you would use:
g++ main.cpp mylib.cpp -o myapp
Dynamic Linking
Dynamic linking, on the other hand, involves loading library code at runtime, which can significantly reduce the size of the executable. This method allows executables to share common libraries, resulting in lower memory usage.
Example Scenario: A GUI application might use dynamic linking to incorporate shared libraries that provide UI components, allowing multiple applications to utilize the same library without duplicating code.
An example of dynamic linking might look like this:
// main.cpp
#include <iostream>
#include <dlfcn.h>
typedef int (*myFunctionType)();
int main() {
void* handle = dlopen("libmylib.so", RTLD_LAZY);
myFunctionType myFunction = (myFunctionType)dlsym(handle, "myFunction");
std::cout << "Result: " << myFunction() << std::endl;
dlclose(handle);
return 0;
}
To compile this dynamically, you would first compile the library:
g++ -shared -fPIC mylib.cpp -o libmylib.so
g++ main.cpp -o myapp -ldl

The Linking Process
Phases of the Linking Process
The linking process comprises two primary phases: Compilation Phase and Linkage Phase.
Compilation Phase
During the compilation phase, the C++ source code is converted into object code. Each source file is compiled independently, producing `.o` files. This modular approach allows for faster compilation times since only changed files need recompiling.
Linkage Phase
In the linkage phase, the linker resolves external references among `.o` files. It ensures that all functions and variables used in the code are correctly linked together, addressing any symbol references. This step is vital for producing the final executable from the object files.
Tools Involved in the Linking Process
Linker
A linker is a utility that plays a pivotal role in the compilation process. It takes all the object files produced during the compilation phase and combines them into a single executable. One of the most common linkers used in C++ development is GNU ld.
Build Systems
Build systems simplify the process of managing builds, including linking. Tools like Makefile and CMake automate the compilation and linking process, allowing developers to specify their build configurations in a concise manner.
For example, a simple Makefile for a C++ project might look as follows:
CXX=g++
CXXFLAGS=-Wall -g
all: myapp
myapp: main.o mylib.o
$(CXX) -o myapp main.o mylib.o
main.o: main.cpp
$(CXX) $(CXXFLAGS) -c main.cpp
mylib.o: mylib.cpp
$(CXX) $(CXXFLAGS) -c mylib.cpp
clean:
rm -f *.o myapp

Common Linking Errors and Solutions
Undefined Reference Errors
One of the most common issues developers encounter during linking is undefined reference errors. These errors occur when the linker cannot find the definition of a function or variable that has been declared.
Causes: This typically happens if a function is declared but not implemented.
Here’s an example of code that could cause this error:
// main.cpp
#include <iostream>
extern void myFunction();
int main() {
std::cout << "Result: " << myFunction() << std::endl;
return 0;
}
Solutions:
- Ensure every declared function is implemented in the source files that are linked.
- Check if the linker is finding all necessary object files and libraries.
Duplicate Symbols
Another linking problem arises when the linker encounters duplicate symbols. This error occurs when the same variable or function is defined in multiple object files.
Causes: Multiple definitions result from including the same file in several source files without the appropriate guards.
Here’s an example that could lead to duplicate symbols:
// mylib.h
int myVariable = 10; // This will cause issues when included in multiple .cpp files
// mylib.cpp
#include "mylib.h"
Solutions:
- Use header guards to prevent multiple inclusions of the same file, as shown below:
#ifndef MYLIB_H
#define MYLIB_H
extern int myVariable;
#endif
- Managing the use of `static` for file-scoped variables can also help resolve these conflicts.
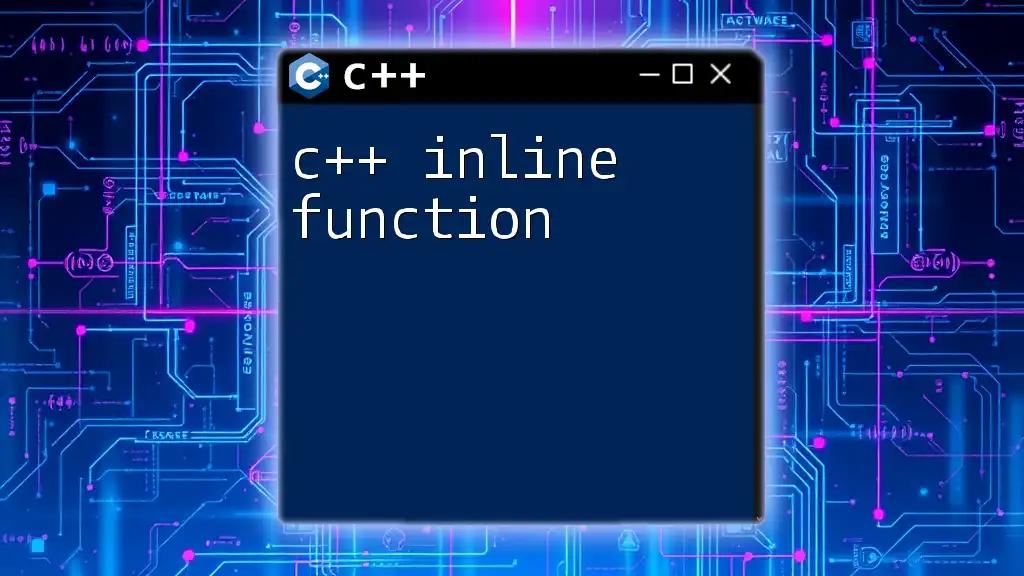
Best Practices for Linking in C++
Organizing Your Code
To make linking more manageable, it’s essential to employ modular programming principles. By structuring your code into well-defined modules, you enhance readability and isolation between different parts of the program.
An organized C++ project directory structure might look like this:
/Project
/src
main.cpp
mylib.cpp
/include
mylib.h
/lib
libmylib.so
Using Version Control
Incorporating version control into your development process can help track changes related to linking. When collaborating with multiple developers, maintaining a clear history of code changes can prevent linking errors due to conflicting modifications.
Recommended Practices:
- Commit linked files along with their dependencies.
- Write clear commit messages that describe changes related to linking.
Documentation of Libraries
An often-overlooked aspect of development is keeping documentation up to date. Providing comprehensive documentation for linked libraries is crucial for other developers and maintainers of your code.
Example Documentation Structure:
- Overview of the library functionality.
- Dependency requirements.
- Instructions for building and linking the library effectively.
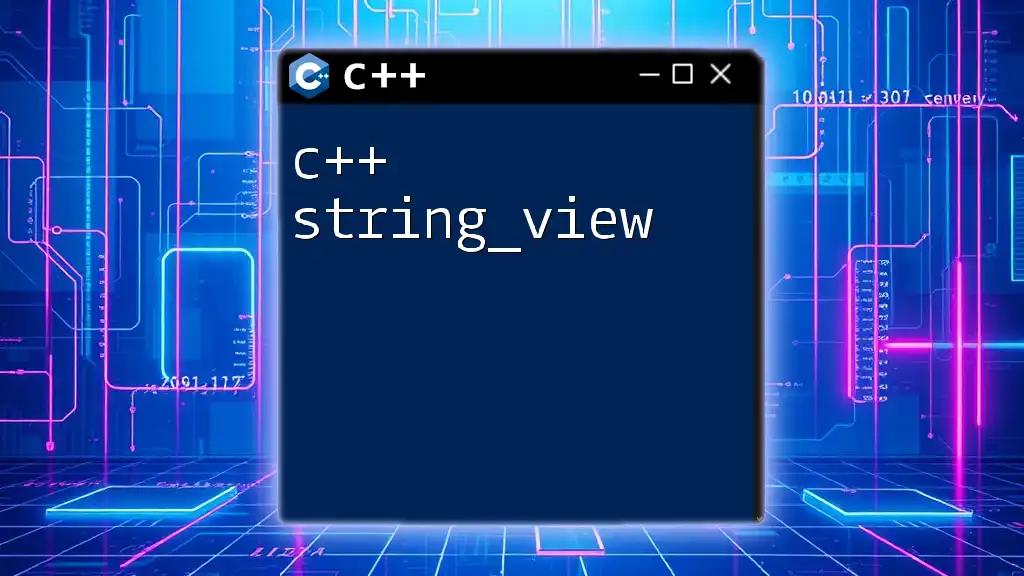
Conclusion
In this guide, we have explored the fundamentals of C++ linking, from its definition and types to the phases involved in the linking process. We’ve also addressed common linking errors and best practices to follow for successful C++ development.
Understanding linking is essential for effective C++ programming. As you continue your journey in C++, we encourage you to practice linking in your projects and become familiar with its intricacies. By mastering this vital process, you can create efficient, reliable C++ applications.
For those seeking to deepen their knowledge, consider exploring additional resources, introductory courses, or attending workshops focused on C++ programming concepts.