C++ Wikibooks is a collaborative platform that offers comprehensive, community-driven resources and tutorials to help learners understand and master C++ programming.
Here’s a simple C++ code snippet that demonstrates a basic "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is Wikibooks?
Definition and Purpose
Wikibooks is a project under the Wikimedia Foundation designed to provide a platform for free educational resources. It serves as a collaborative space where individuals can create and edit books on various subjects, making knowledge more accessible to everyone. The primary purpose of Wikibooks is to support the learning process through a wealth of information, without the barrier of cost typically associated with textbooks.
How Wikibooks Works
The collaborative nature of Wikibooks allows users from around the world to contribute, share knowledge, and improve existing resources. Anyone can edit the content, which means that the information can be constantly updated and refined. This open-editing format encourages a sense of community and helps ensure that the materials remain relevant and accurate.
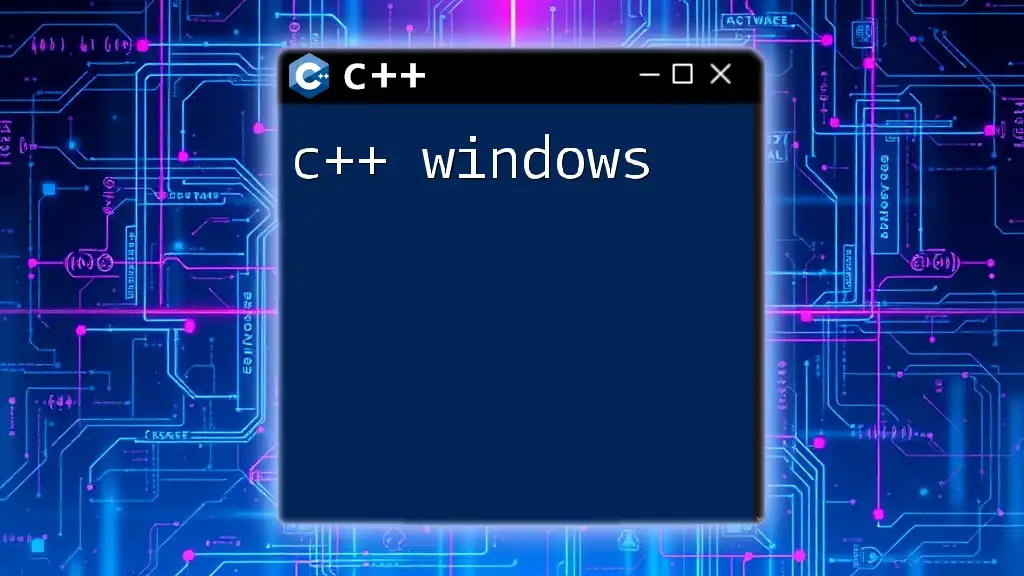
C++ Wikibooks: An Overview
Purpose of C++ Wikibooks
C++ Wikibooks aims to provide learners with a detailed understanding of C++ programming. The content is designed to cater to individuals at various skill levels, from beginners just starting to explore programming to advanced users looking to refine their skills or understand new concepts. The simplicity and clarity of the entries make it easier for readers to grasp complex topics.
Structure of C++ Wikibooks
C++ Wikibooks is typically organized into several sections that comprehensively cover essential topics. An example structure might include:
- Introduction to C++: A gentle introduction, covering the history and significance of the language.
- Basic Syntax: Fundamental grammar and conventions in writing C++ code.
- Data Types: An overview of different data types in C++, including integers, floats, characters, and strings.
- Control Structures: Understanding statements that control the flow of the program.
- Functions: Defining and using functions to improve modularity and reusability in code.
- Object-Oriented Programming: Principles such as classes, objects, inheritance, and polymorphism.
- Advanced Topics: Covering templates, exception handling, and standard template library usage.
- Best Practices: Guidance on writing clean, efficient, and maintainable C++ code.
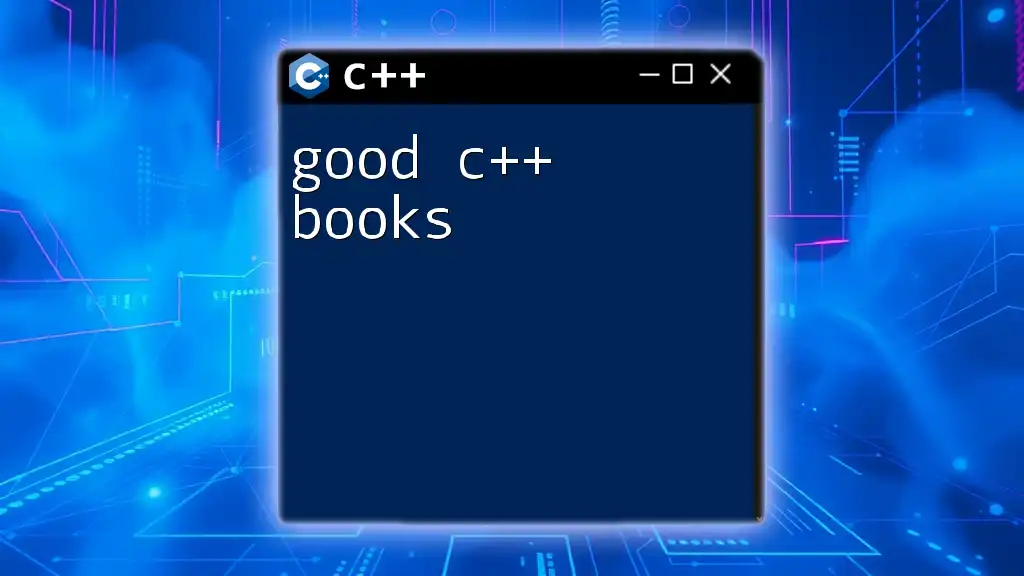
Key Benefits of Using C++ Wikibooks
Accessibility and Cost
One of the standout features of C++ Wikibooks is that it is completely free. Learners can access the material anytime and anyplace via the internet, providing unprecedented convenience. This aspect makes it an invaluable resource for students who may lack access to commercial programming books or formal classes.
Community-driven Learning
The collaborative nature of Wikibooks means that readers can glean insights not just from the material but also from the community. Contributions from various individuals enhance the quality and breadth of content. By participating in discussions or editing existing entries, learners become an active part of the knowledge-sharing ecosystem, which fosters a deeper understanding of the subject matter.
Up-to-date Information
Wikibooks is dynamic; it’s regularly updated by contributors to reflect new developments in C++. As the language evolves, the content on C++ Wikibooks can be revised to include the latest standards, practices, and examples that are aligned with current programming trends.
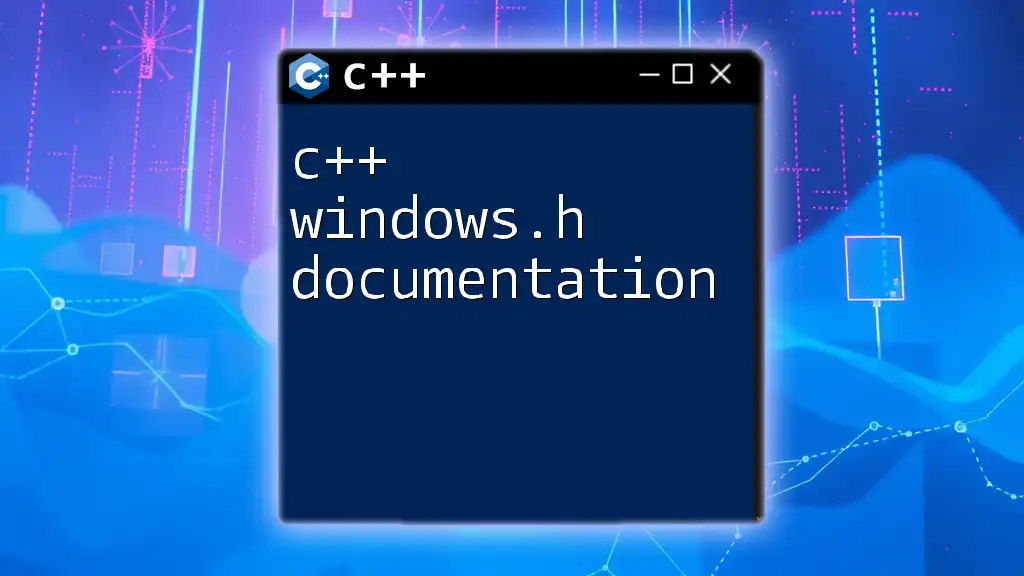
Navigating C++ Wikibooks
Finding Content
Finding specific C++ topics on Wikibooks can be simple and intuitive. The site typically features a comprehensive table of contents that can guide you through the various categories. Additionally, the search function allows you to explore subjects quickly, helping you locate the information you need efficiently.
Utilizing the Content
When utilizing C++ Wikibooks, it’s important to know how to extract useful information effectively. Focus on key sections that pertain to your current learning objectives, and consider taking notes while you read. Hands-on practice with the code examples can reinforce your understanding and help you retain new concepts. Implement the learned concepts through real-world problems or small projects to solidify your knowledge.
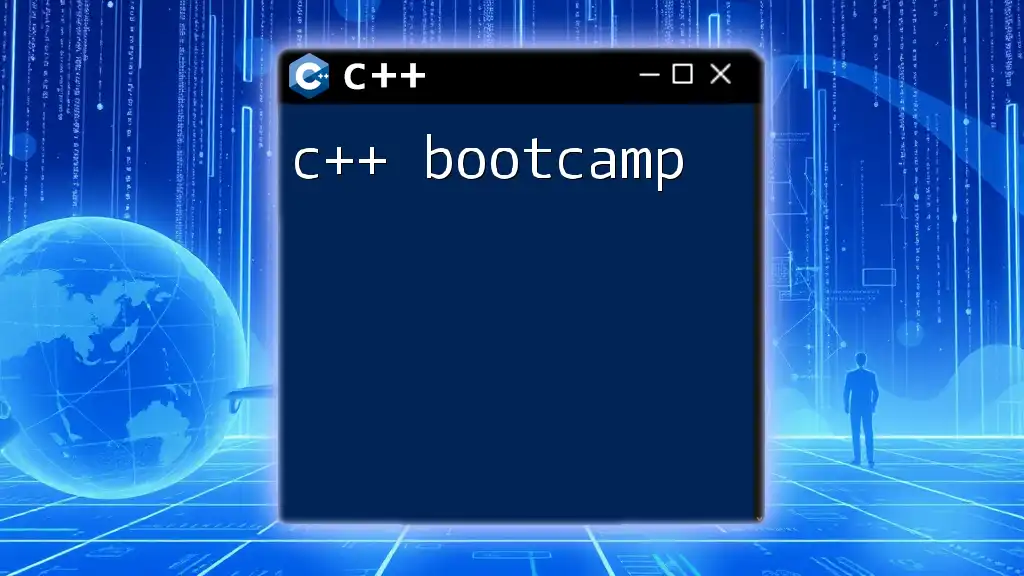
Examples and Code Snippets from C++ Wikibooks
Basic Syntax and Data Types
To illustrate basic syntax, consider the following code snippet:
#include <iostream>
using namespace std;
int main() {
int number = 10; // Integer data type
cout << "The number is: " << number << endl;
return 0;
}
This example showcases the structure of a simple C++ program. The `#include <iostream>` directive allows the use of standard input-output streams, while the `main` function is the entry point of any C++ program. The code initializes an integer variable and displays it using `cout`, which outputs text to the console.
Control Structures
Understanding control structures is crucial for effective programming. The following code snippet demonstrates a simple for loop:
for(int i = 0; i < 5; i++) {
cout << "This is iteration: " << i << endl;
}
This code iteratively prints out a message five times, illustrating how loops can be utilized to repeat actions. Control structures like loops and conditionals are foundational concepts that enable you to dictate the flow of a program.
Functions
Functions promote code organization and reusability. Here’s an example of a basic function that adds two numbers:
int add(int a, int b) {
return a + b;
}
In this snippet, we define a function named `add` that takes two integer parameters and returns their sum. Understanding functions will help you not only organize your code but also encourage collaboration and code-sharing, making your programming efforts more efficient.
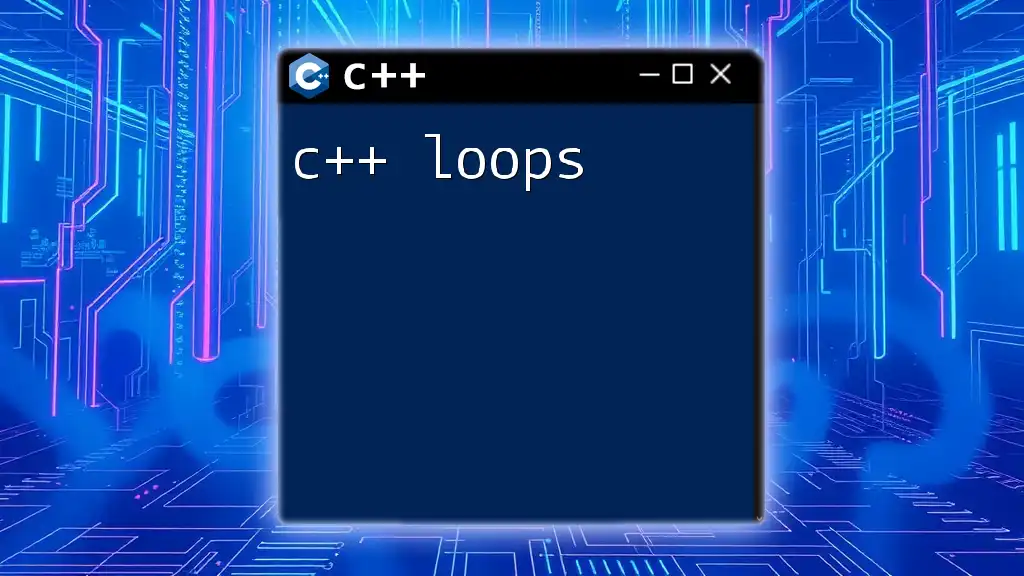
Tips for Learning C++ Using Wikibooks
Set Learning Goals
Establishing clear learning objectives is key to successful study. Define what you aim to achieve with your C++ skills and track your progress. Whether it's completing a project or mastering specific concepts, setting goals can keep you motivated.
Practice Regularly
Consistent practice is essential for mastering C++. Utilize coding challenges, exercises, and project ideas to reinforce what you learn. The more you code, the more comfortable you become with different concepts and syntax.
Engage with the Community
Participate in discussions, join forums, and seek guidance from others in the Wikibooks community. Sharing your insights and asking questions can lead to new understandings and improvements in your learning process.
Supplement with Other Resources
While C++ Wikibooks is an excellent primary resource, consider complementary materials such as advanced textbooks, online courses, or coding platforms. A multi-faceted approach to learning will deepen your understanding and expose you to various perspectives and methods.
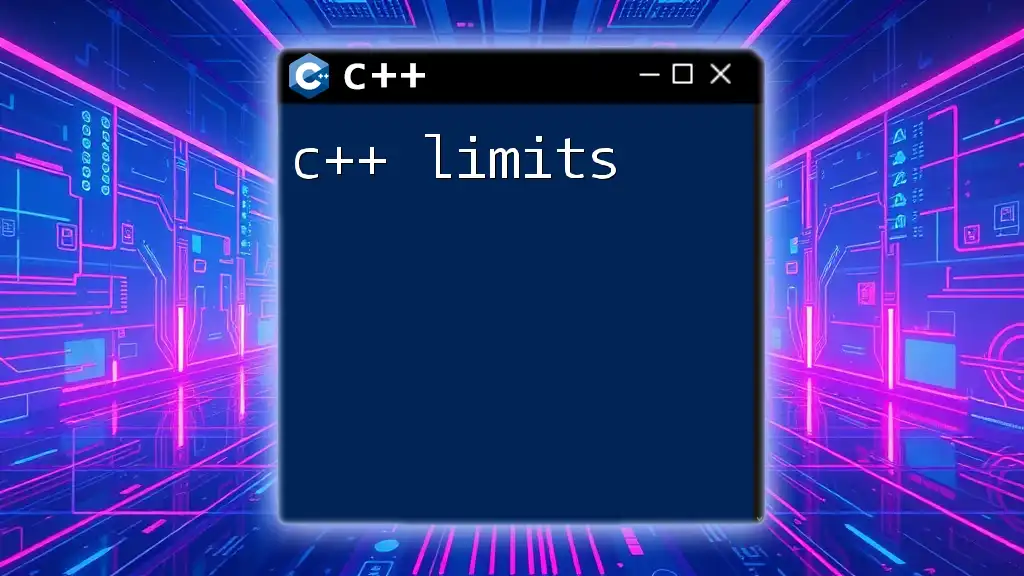
Conclusion
C++ Wikibooks stands as a vital resource for anyone looking to embark on or advance their C++ programming journey. The combination of community collaboration, constant updates, and free access positions Wikibooks as an invaluable tool for aspiring programmers. Embrace the opportunity to explore its content, interact with fellow learners, and contribute to this unique educational platform.
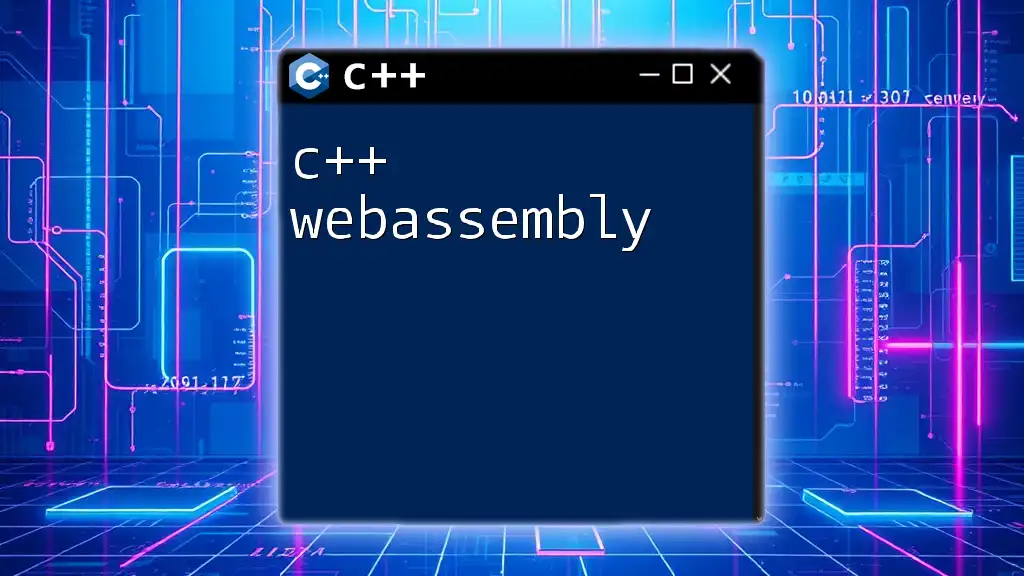
Call to Action
Take the plunge into C++ Wikibooks today and start enhancing your programming skills. Reflect on your learning experiences, and don’t hesitate to share your feedback or contribute to the community. Your journey in mastering C++ begins here!