CPP textbooks provide essential knowledge and practical guidance for mastering C++ programming through comprehensive explanations and examples, enabling learners to effectively utilize cpp commands. Here's a simple example of defining a function in C++:
#include <iostream>
void greet() {
std::cout << "Hello, World!" << std::endl;
}
int main() {
greet();
return 0;
}
Understanding C++ Textbooks
What are C++ Textbooks?
C++ textbooks serve as foundational resources for learners who wish to master the language. They are meticulously written to guide individuals through various aspects of C++ programming, including syntax, principles, and best practices. Unlike online tutorials or courses, textbooks provide a structured approach, often taking readers from basic concepts to more advanced topics systematically.
Who Should Use C++ Textbooks?
C++ textbooks cater to a diverse audience.
- Beginners can find valuable resources that introduce fundamental concepts, ensuring their understanding of the language’s core principles.
- Intermediate learners can deepen their existing knowledge, tackling more sophisticated C++ features and nuances.
- Advanced programmers may seek specialized texts that focus on niche areas such as optimization, memory management, or software design patterns specific to C++.
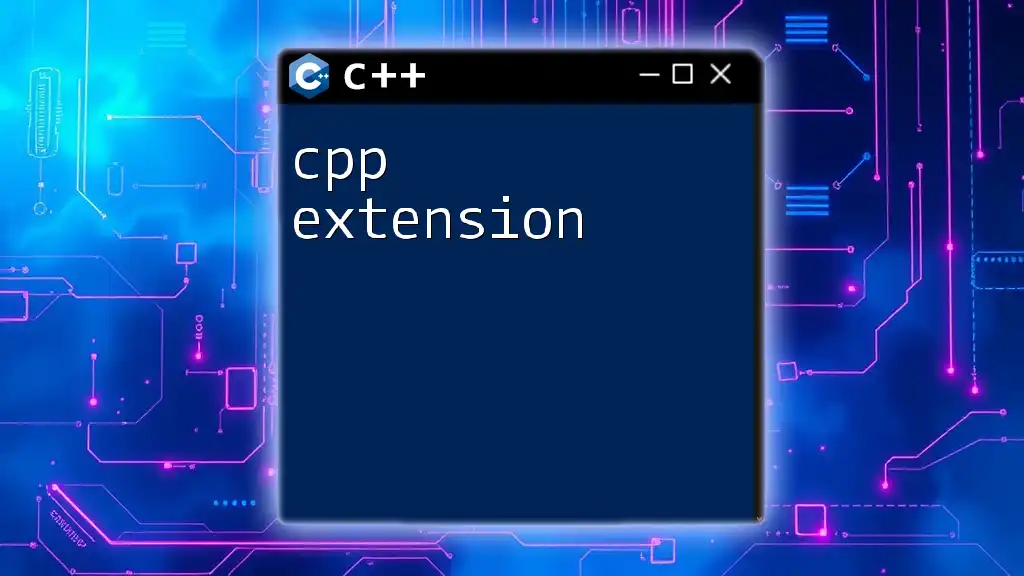
Types of C++ Textbooks
Beginner-Focused Textbooks
Beginner-focused textbooks are designed to ease readers into the realm of C++. They often include simple explanations, examples, and exercises that build foundational knowledge.
Recommended Titles
-
"C++ Primer" by Stanley B. Lippman
This textbook is a favorite among newcomers, detailing the C++ language comprehensively. It introduces concepts such as classes, functions, and templates while providing rich examples that help to demystify complex topics. -
"Programming: Principles and Practice Using C++" by Bjarne Stroustrup
Written by the creator of C++, this book aims to teach programming as a discipline in addition to C++. It emphasizes practical programming as well as theory, making it perfect for learners who seek a balanced approach.
Intermediate and Advanced Textbooks
As learners grow more confident with C++, they need resources that challenge their skill set and introduce more complex topics.
Recommended Titles
- "Effective C++" by Scott Meyers
Focused on best practices, this book consists of 55 specific ways to improve your programs and designs. For example, one of the rules, “The Rule of Three,” recommends defining a destructor, copy constructor, and copy assignment operator to manage resources effectively:
class Example {
public:
Example(const Example& other); // Copy constructor
Example& operator=(const Example& other); // Copy assignment
~Example(); // Destructor
};
- "The C++ Programming Language" by Bjarne Stroustrup
This comprehensive book covers nearly every significant aspect of C++. Its detailed explanations and examples serve both as a reference guide and an educational text, benefiting readers at all levels.
Specialized Textbooks
Specialized textbooks dive into particular applications of C++. These books tend to focus on frameworks, libraries, or specific domains within computing.
Recommended Titles
-
"C++ GUI Programming with Qt"
This book guides readers through developing graphical user interfaces using Qt, making it a suitable resource for those interested in desktop application development. -
"C++ Data Structures and Algorithms"
Aimed at teaching data structure theory alongside practical implementation using C++, this book is essential for learners wishing to master algorithms and their real-world applications.
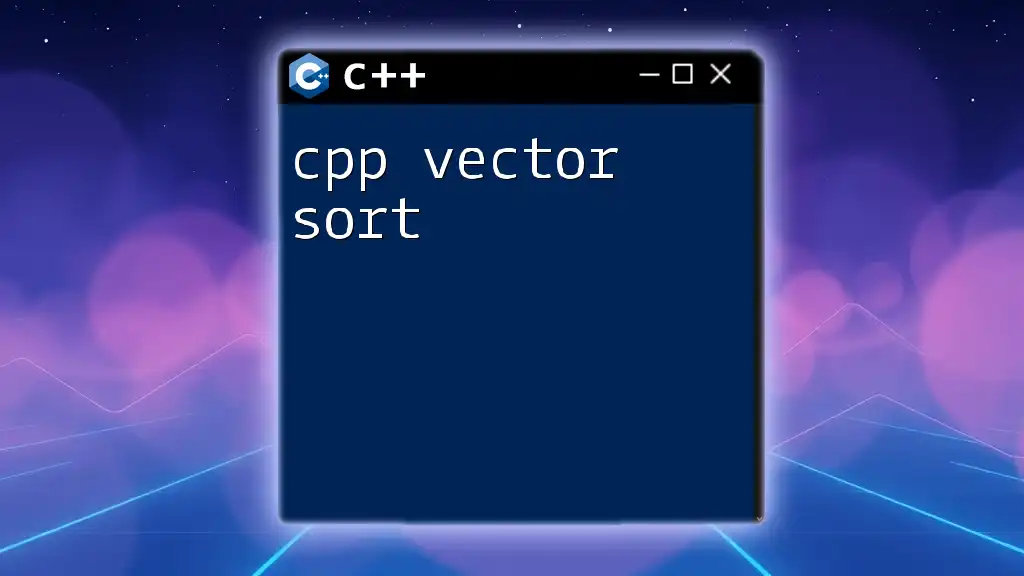
What to Look For in a C++ Textbook
Clarity and Accessibility
When selecting a C++ textbook, clarity in writing should be a top criterion. A good textbook articulates concepts in a straightforward manner, often employing analogies and practical examples. Consider evaluating excerpts from different books to gauge their accessibility before making a choice.
Comprehensive Coverage
An effective C++ textbook should encompass essential topics, including but not limited to:
- Basic data types and control structures
- Object-oriented programming principles (inheritance, polymorphism)
- The Standard Template Library (STL) and its utilities
This comprehensive approach ensures that learners can pivot between fundamental and advanced topics seamlessly.
Examples and Practice Problems
A hallmark of quality C++ textbooks is the inclusion of comprehensible examples combined with practice problems. These features encourage active participation, allowing learners to apply concepts promptly and gain hands-on experience.
Author Credentials
The expertise and background of the author significantly affect a textbook's value. Selecting books authored by recognized experts in C++ brings credibility to the learning material, assuring readers of the reliability of the information presented.
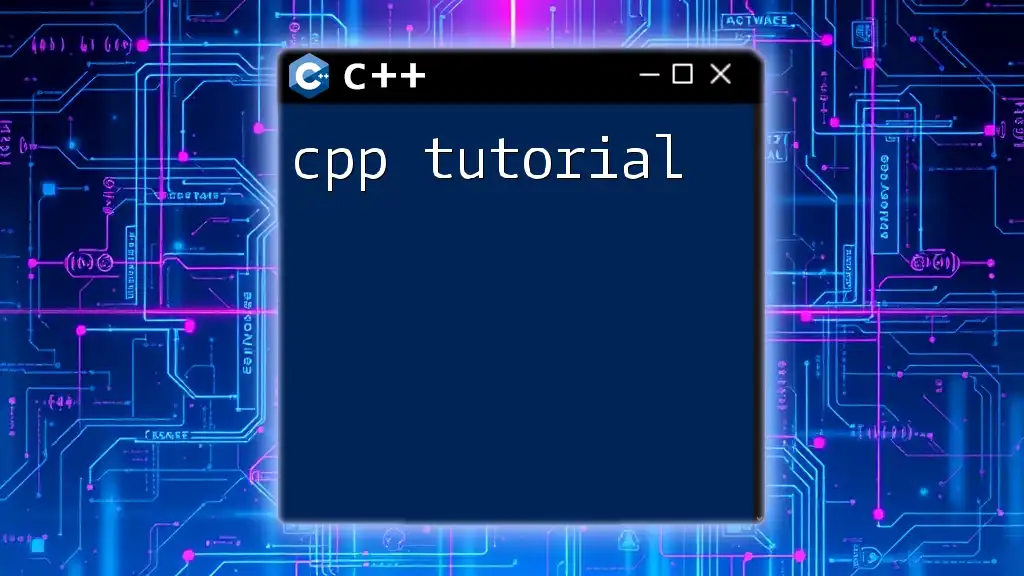
Supplementing Textbook Learning
Online Resources
While textbooks provide substantial knowledge, they can be enhanced through online resources. Websites like Stack Overflow and C++ reference sites allow learners to engage in community discussions, troubleshoot real-world problems, and deepen their understanding.
C++ Coding Challenges
Practicing coding challenges on platforms such as LeetCode, HackerRank, or Codecademy reinforces lessons learned. A simple coding challenge might involve creating a function to reverse a string. Here’s an example of a straightforward implementation:
#include <iostream>
#include <algorithm>
#include <string>
std::string reverseString(const std::string &str) {
std::string reversed = str;
std::reverse(reversed.begin(), reversed.end());
return reversed;
}
int main() {
std::string original = "Hello, World!";
std::cout << reverseString(original) << std::endl; // Output: !dlroW ,olleH
}
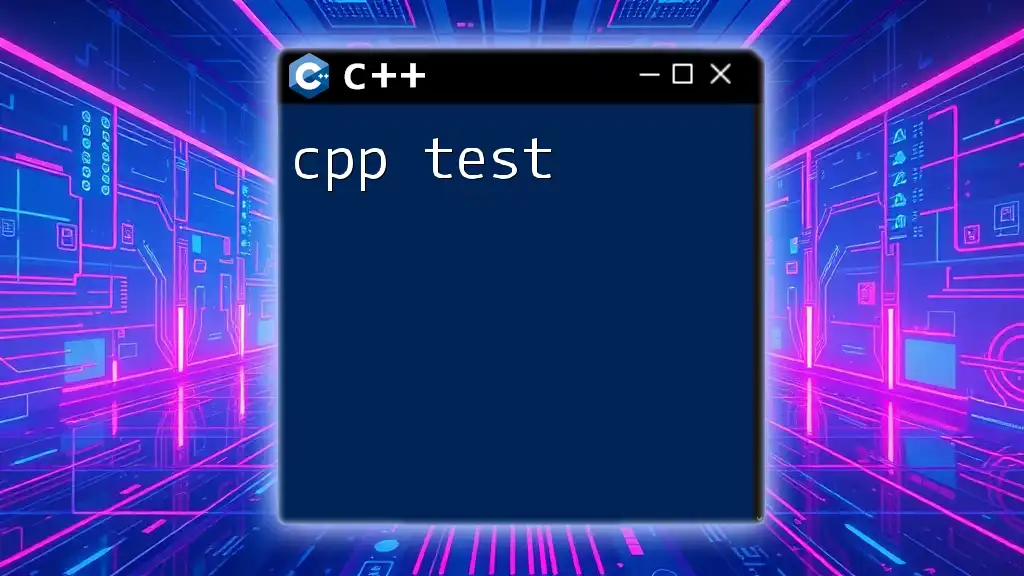
Tips for Using C++ Textbooks Effectively
Setting Goals and Tracking Progress
Establishing defined learning objectives is essential for success. Setting milestones such as completing chapters, understanding core concepts, or successfully coding examples can help track consistent progress. Aim for clarity in your goals, such as "Complete Chapter 5 by the end of the week."
Active Learning Techniques
To maximize retention, utilize active learning techniques. These could include taking structured notes, summarizing concepts in your own words, or implementing sample projects based on learned principles. Actively engaging with the material enables a more profound comprehension.
Review and Revision Strategies
Consistent review of material is crucial for long-term retention. Revisiting chapters, glossaries, and exercise answers reinforces learning. Aim to revisit each major topic multiple times over an extended period to solidify knowledge.
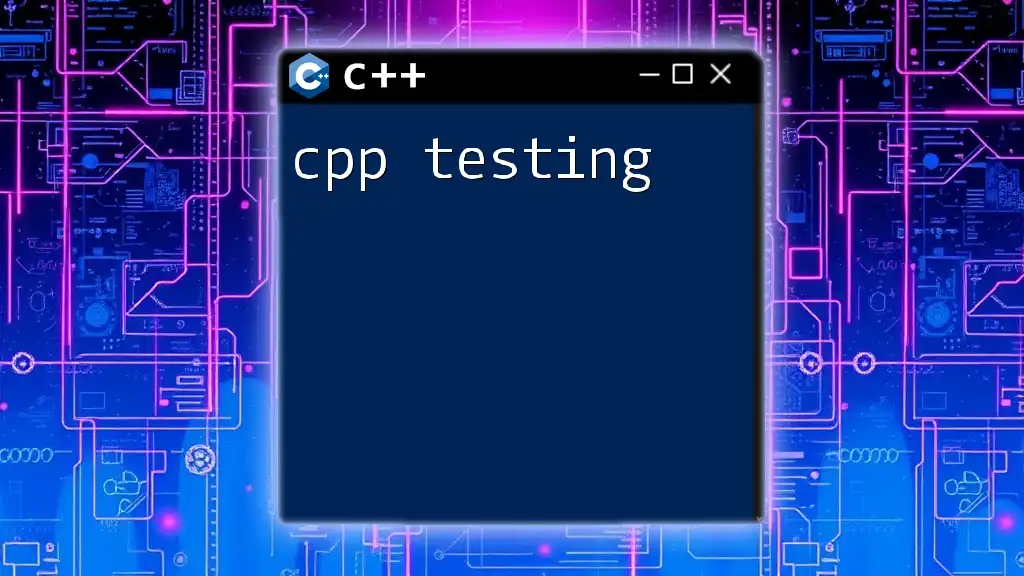
Conclusion
Selecting the right C++ textbooks can profoundly impact your programming journey. By understanding the types of textbooks available, identifying what to look for, and supplementing your reading with active practices, you can develop a robust C++ skill set. The journey may be challenging, but the rewards of mastering C++ are invaluable in today’s tech-centric world.
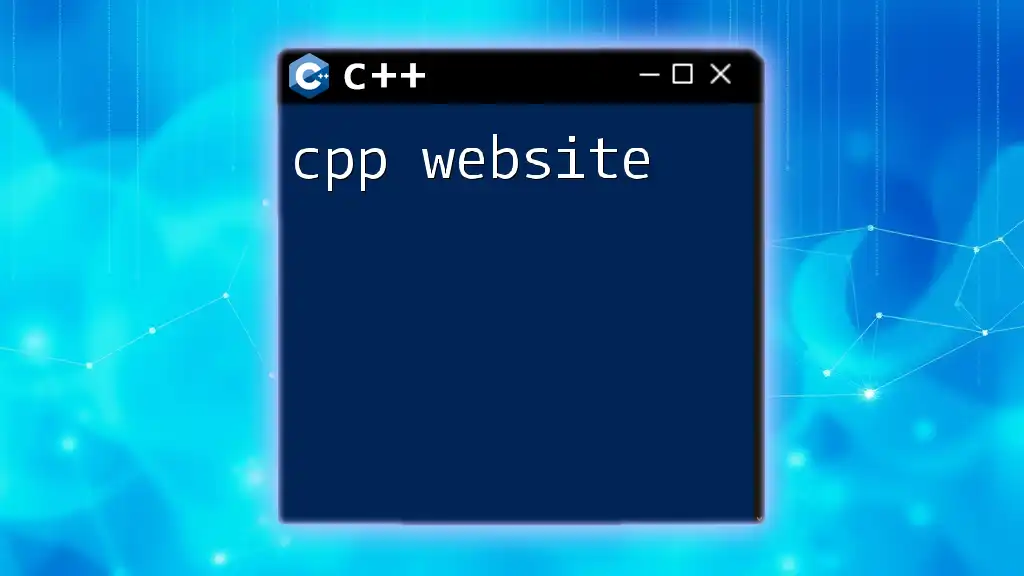
Call to Action
What are your favorite C++ textbooks? Share your recommendations and feedback in the comments below, and let’s enhance our collective learning experience!