In C++, you can easily add elements to a vector using the `push_back()` method, which appends a new element to the end of the vector.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec;
vec.push_back(10); // Adds 10 to the end of the vector
vec.push_back(20); // Adds 20 to the end of the vector
for (int i : vec) {
std::cout << i << " "; // Output: 10 20
}
return 0;
}
Understanding Vector Basics
What is a Vector in C++?
In C++, a vector is a sequence container that encapsulates dynamic size arrays. Vectors can grow and shrink in size, which allows for efficient insertion and removal of elements. Unlike traditional arrays, which have a fixed size, vectors manage their own storage automatically.
Key Properties of C++ Vectors:
- Dynamic Size: Vectors can change in size, expanding or contracting as needed.
- Performance: Access time for elements is constant (O(1)), similar to arrays.
- Element Storage: Supports any data type, including user-defined types.
To use vectors in your C++ program, you must include the vector library with the directive:
#include <vector>

How to Create a Vector in C++
Creating a vector in C++ is straightforward. The syntax for vector declaration allows you to create vectors of various types.
Syntax for Vector Declaration:
std::vector<type> vectorName;
You can specify an initial size and default values as well:
Example: Creating a Vector in C++
std::vector<int> numbers; // Creates an empty vector
std::vector<int> numbers(5, 0); // Creates a vector of size 5, initialized with 0

Adding Elements to a C++ Vector
Adding elements to a C++ vector can be accomplished in several ways.
Different Methods to Add Elements
Using push_back():
The `push_back()` method adds an element to the end of the vector. It is the most commonly used method for adding elements.
Example: Adding an Element Using push_back()
std::vector<int> numbers;
numbers.push_back(10); // Adds 10 to the end of the vector
Using insert():
The `insert()` method allows you to add elements at specific positions within the vector.
Example: Inserting an Element at a Specific Position
std::vector<int> numbers = {1, 2, 3};
numbers.insert(numbers.begin() + 1, 5); // Inserts 5 at index 1
Using emplace_back():
The `emplace_back()` method constructs elements in place, which can be more efficient than `push_back()`.
Example: Adding an Element Using emplace_back()
std::vector<std::string> fruits;
fruits.emplace_back("Apple"); // Adds "Apple" to the end of the vector
Adding Multiple Elements to a Vector
You may often need to add multiple elements at once. We can achieve this using the `insert()` method in combination with a range of elements from another container.
Example: Inserting a Range of Elements
std::vector<int> numbers = {1, 2, 3};
std::vector<int> moreNumbers = {4, 5, 6};
numbers.insert(numbers.end(), moreNumbers.begin(), moreNumbers.end()); // Adds 4, 5, 6 to the end

How to Check the Size and Capacity of a C++ Vector
Understanding the size and capacity of your vector is crucial for efficient memory management.
- Size: This attribute tells you the current number of elements in the vector, which you can retrieve using the `size()` method.
- Capacity: This tells you the maximum number of elements the vector can store without needing to allocate more memory and can be checked using the `capacity()` method.
Methods:
std::cout << "Size: " << numbers.size() << std::endl; // Displays the size
std::cout << "Capacity: " << numbers.capacity() << std::endl; // Displays the capacity
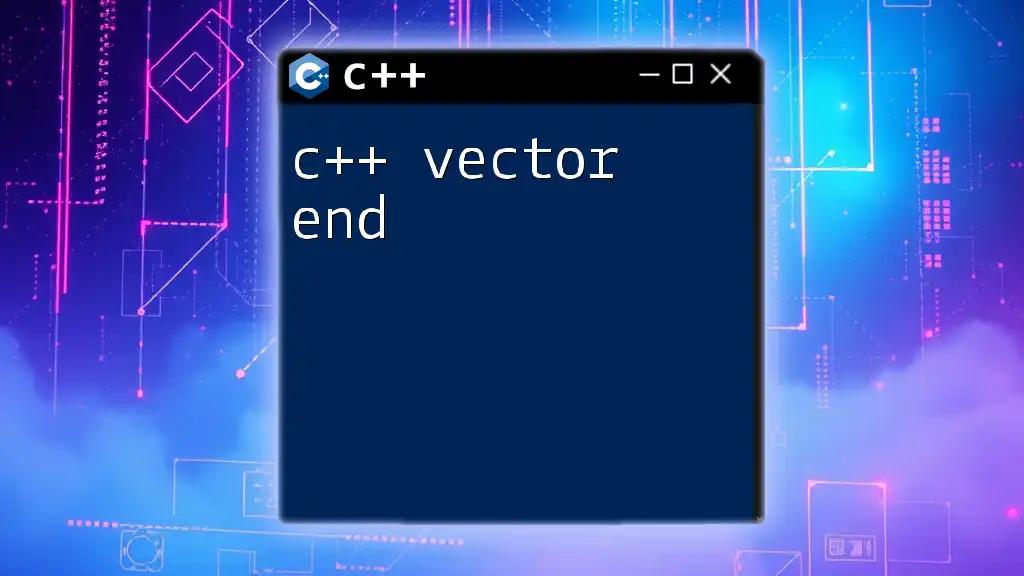
Removing Elements from a C++ Vector
To manage a vector effectively, you may want to also remove elements.
Using pop_back():
The `pop_back()` method removes the last element from the vector.
Example: Removing the Last Element
numbers.pop_back(); // Removes the last element
Using erase():
The `erase()` method allows you to remove elements located at specific positions or a range of elements.
Example: Removing an Element at a Specific Position
numbers.erase(numbers.begin() + 1); // Removes the element at index 1
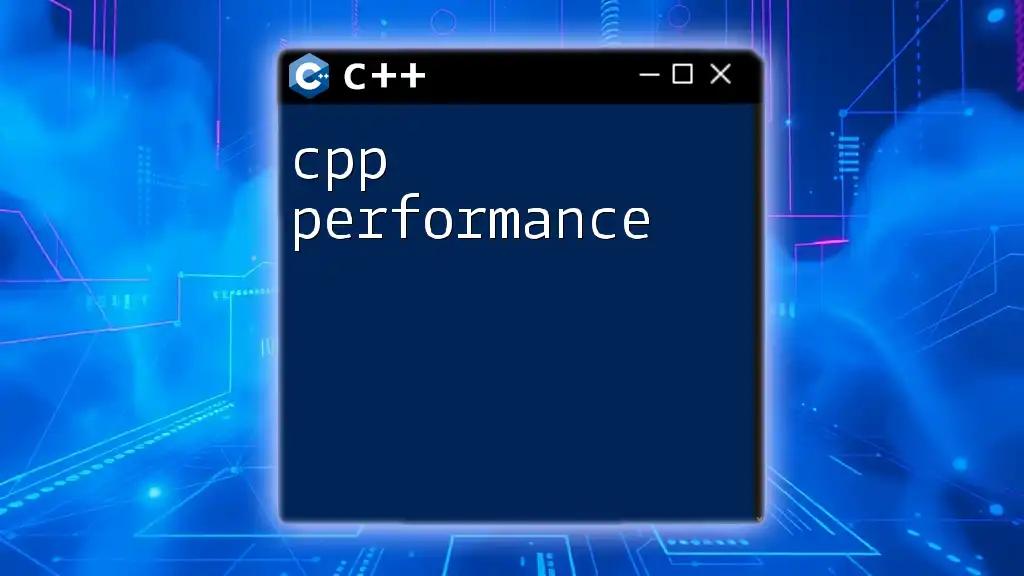
Best Practices for Managing Vectors in C++
To ensure efficient use of vectors, consider the following best practices:
- Memory Management: Always be mindful of the memory utilization, especially when dealing with large datasets.
- When to Reserve Capacity: If you know the number of elements you will add in advance, use the `reserve()` method to allocate memory upfront, which can minimize reallocations.
numbers.reserve(100); // Pre-allocates space for 100 elements
- Minimizing Copying of Vectors: Prefer pass by reference when passing vectors to functions to avoid unnecessary copying, which can be computationally expensive.
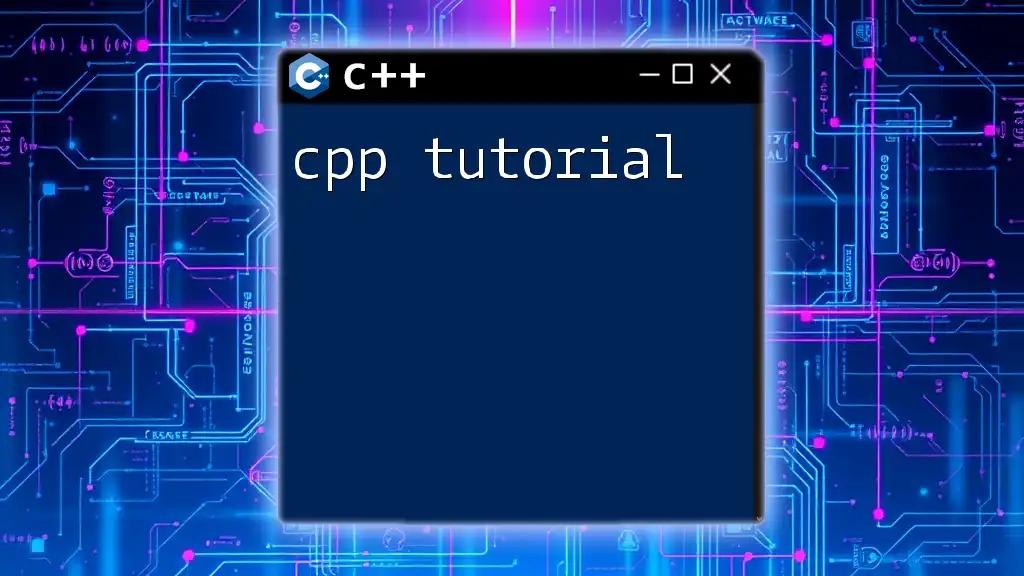
Conclusion
Manipulating vectors in C++ is essential for efficient programming. By mastering various ways to add to a vector, including methods like `push_back()`, `insert()`, and `emplace_back()`, you enhance your ability to work with dynamic data collections.
Experimenting with these methods will strengthen your understanding of vector management. Remember, practice makes perfect when dealing with the intricacies of the C++ standard library's vector functionalities.
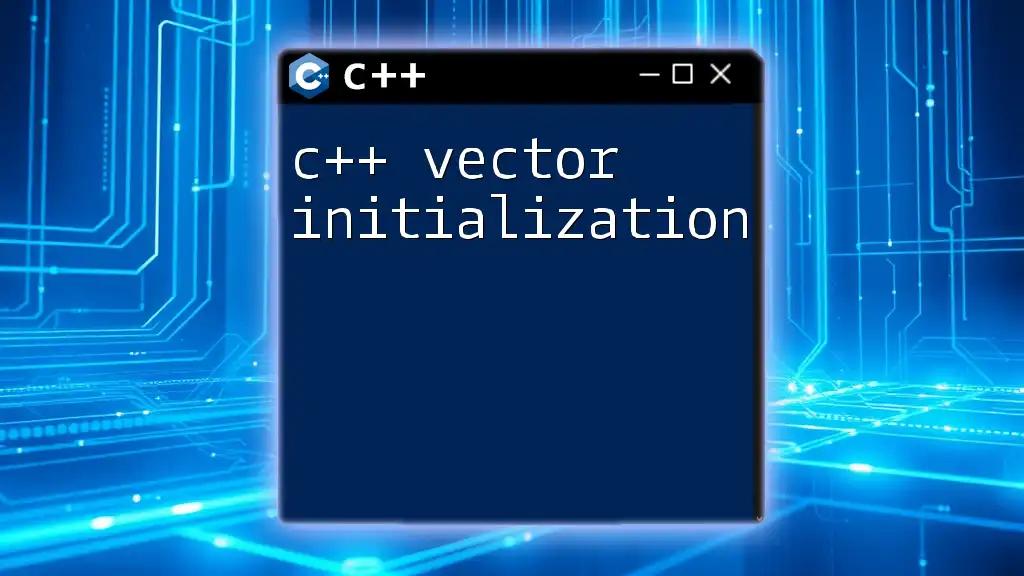
FAQs
What is the main difference between push_back and emplace_back?
`push_back` copies or moves the object into the vector, while `emplace_back` constructs the object in place, making it potentially more efficient, especially for complex objects.
Can a vector hold different types of data?
Vectors can only hold elements of the same data type. If you need to store different types, consider using a `std::variant` or a `std::any`.
What happens when a vector exceeds its capacity?
When a vector exceeds its capacity, it automatically allocates more memory, typically doubling the current capacity. This process may involve copying elements to the new memory location, which can be costly in terms of performance.