In C++, you can insert elements into a vector at a specified position using the `insert` method, as shown in the following example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector = {1, 2, 4, 5};
myVector.insert(myVector.begin() + 2, 3); // Insert 3 at index 2
for (int num : myVector) {
std::cout << num << " "; // Output: 1 2 3 4 5
}
return 0;
}
Understanding C++ Vectors
What is a Vector?
A vector in C++ is a dynamic array that can resize itself automatically when elements are added or removed. Unlike standard arrays, which have a fixed size, vectors provide flexibility for handling varying amounts of data. This makes them suitable for situations where the size of the dataset can change throughout the program.
Basic Characteristics of Vectors
Vectors can expand and shrink in size, enabling easy memory management. Each insertion or deletion operation adjusts the vector size without the need for additional code. Additionally, vectors allow random access to elements, making it easy to retrieve values using an index, similar to arrays.
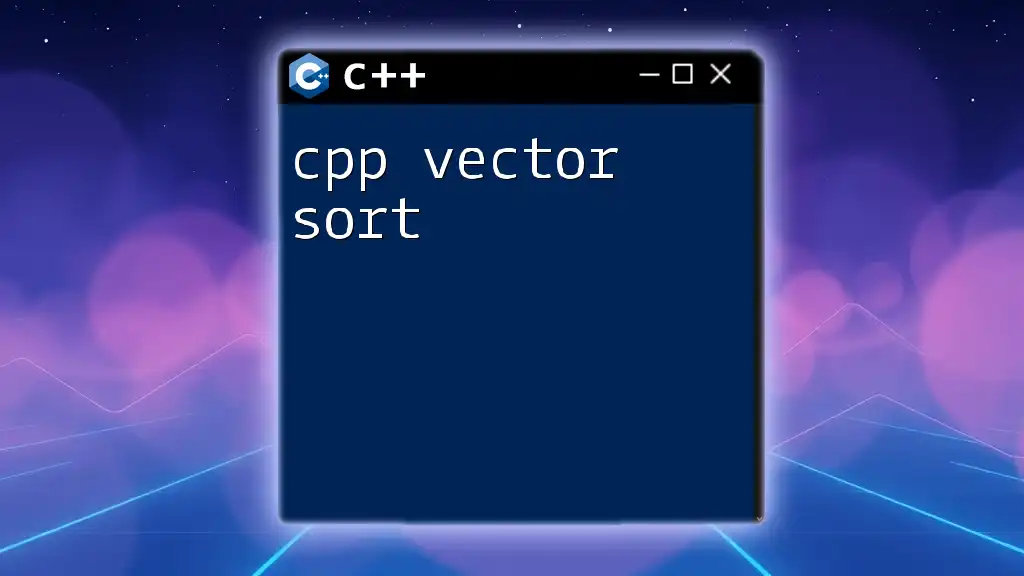
The `insert` Function Overview
What is the `insert` Function?
The `insert` function is a member function of the `std::vector` class that allows you to add elements at specified positions within the vector. Unlike `push_back`, which adds an element always at the end, `insert` enables placement at any index, providing more control over the structure of the vector.
Syntax of `insert`
The general syntax for the `insert` function is as follows:
vector.insert(position, element);
Here, `position` can be an iterator pointing to the desired location within the vector, and `element` denotes the value to be inserted.
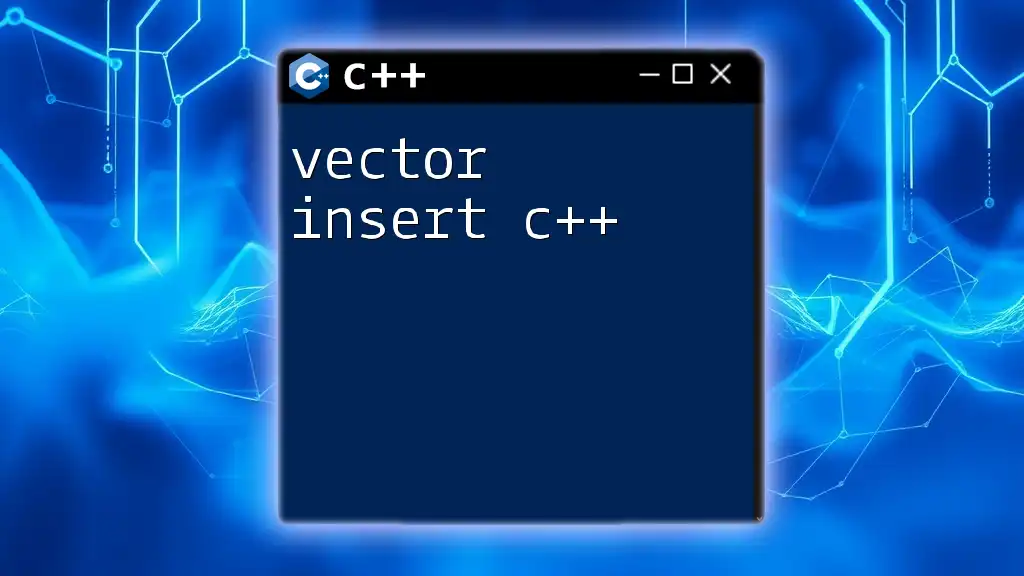
How to Use the `insert` Function
Inserting a Single Element
To insert a single element at a specific position in the vector, you simply specify the position where you want to place it. Here’s a straightforward example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 4, 5};
vec.insert(vec.begin() + 2, 3); // Insert 3 at index 2
for (int x : vec) {
std::cout << x << " "; // Output: 1 2 3 4 5
}
return 0;
}
In this code snippet, we create a vector initialized with the elements `{1, 2, 4, 5}`. By using `vec.insert(vec.begin() + 2, 3)`, we insert the value `3` at the third position (index 2). The output confirms that the insertion was successful.
Inserting Multiple Elements
The `insert` function can also be used to add multiple elements at once. This is done by providing a range of elements using iterators. Here's an example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 5};
std::vector<int> toInsert = {3, 4};
vec.insert(vec.begin() + 2, toInsert.begin(), toInsert.end());
for (int x : vec) {
std::cout << x << " "; // Output: 1 2 3 4 5
}
return 0;
}
In this example, we insert multiple values by calling `vec.insert` with `toInsert.begin()` and `toInsert.end()`, thus placing `3` and `4` into the vector at index `2`. This demonstrates the flexibility of the `insert` method in managing complex data sets.
Inserting Elements Using Fill
You may want to insert multiple identical elements at a specific position in your vector. This can be done easily with the following code:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 5};
vec.insert(vec.begin() + 2, 3, 100); // Insert 100 three times
for (int x : vec) {
std::cout << x << " "; // Output: 1 2 100 100 100 5
}
return 0;
}
Here, `vec.insert(vec.begin() + 2, 3, 100)` inserts the value `100` three times at index `2`. This demonstrates the function's capability to fill the vector with duplicates efficiently.
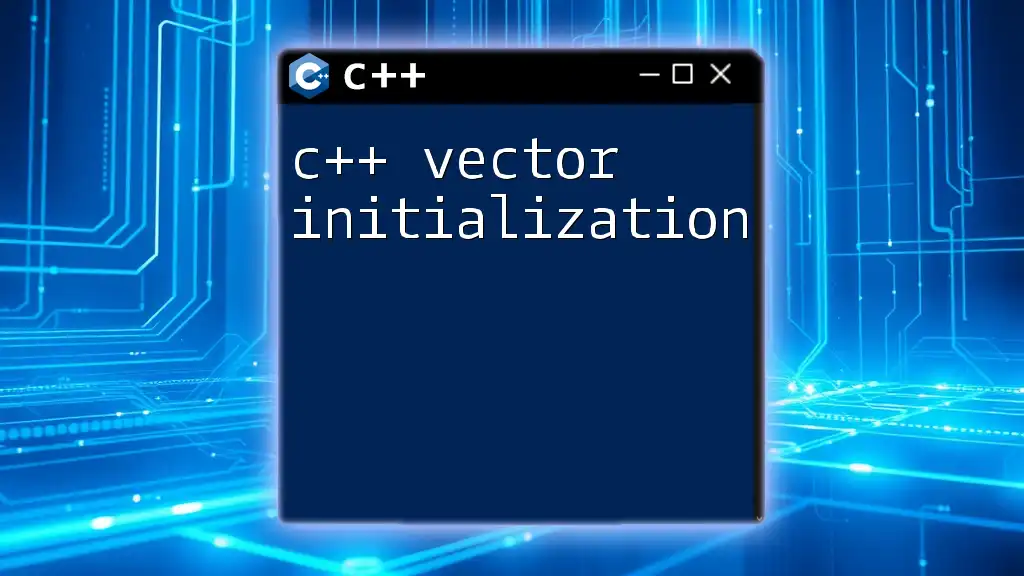
Best Practices When Using `insert`
Memory Considerations
Whenever you insert elements into a vector, it may lead to resizing if the current capacity cannot accommodate the new elements. This resizing involves allocating new memory and copying existing elements, which can significantly impact performance. To minimize these effects, preallocate the size of the vector whenever possible using the `reserve` function.
Choosing the Right Position
When using `insert`, the choice of position is crucial for performance. Inserting at the beginning or the middle of a vector requires shifting subsequent elements, which can be computationally expensive. Therefore, if your application frequently inserts elements, consider using `std::deque` or `std::list`, which may perform better for such operations.
Avoiding Redundant Inserts
To enhance the efficiency of your program, consider checking for duplicates before insertion. For example:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3};
int newValue = 2;
if (std::find(vec.begin(), vec.end(), newValue) == vec.end()) {
vec.insert(vec.begin() + 1, newValue); // Only insert if not present
}
for (int x : vec) {
std::cout << x << " "; // Output: 1 2 3
}
return 0;
}
In this code, we use `std::find` to check if `newValue` is already in the vector. This helps avoid inserting duplicate elements, thus maintaining efficient vector use.
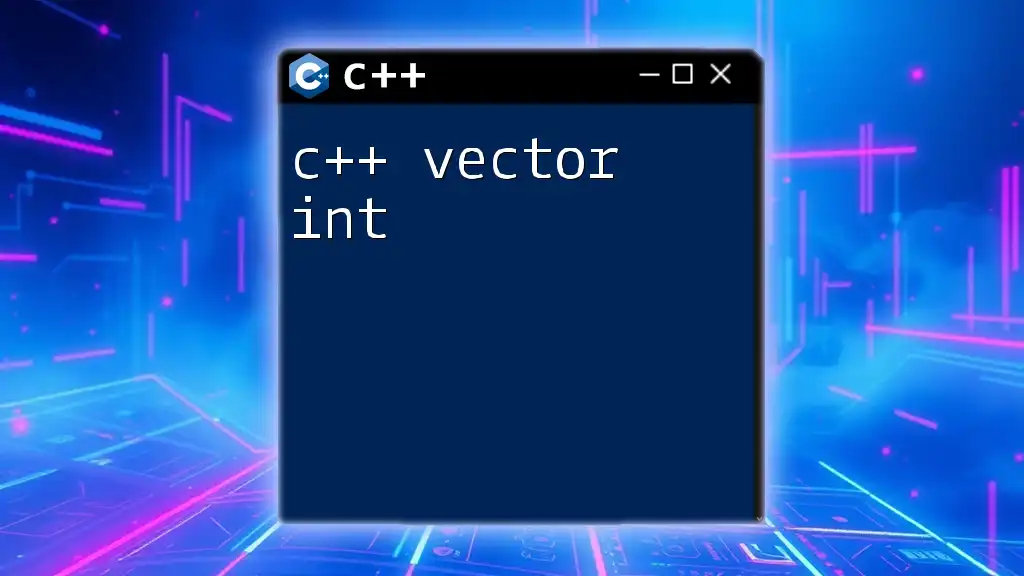
Common Errors and Troubleshooting
Invalid Iterator Positions
One common error when using `insert` is providing an invalid iterator. Always ensure that the iterator points to a valid position within the vector. If the iterator exceeds the bounds (e.g., pointing past `end()`), the program may crash. Always check the condition or wrap the insertion in a safety check.
Capacity Issues
Inserting elements into a vector that is already at maximum capacity may lead to unexpected behavior. When this happens, the vector will automatically resize, leading to a potential performance hit. It’s crucial to be aware of this, especially when dealing with large volumes of data. You can use `capacity()` and `size()` to monitor these metrics during runtime.
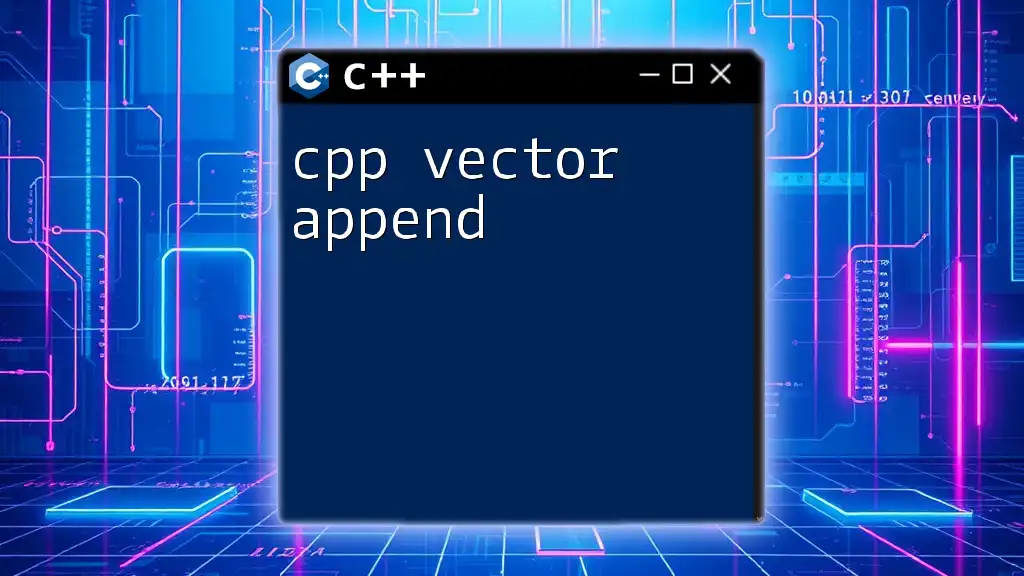
Conclusion
In summary, understanding how to use the `cpp vector insert` function effectively can enhance your ability to manipulate vectors in C++. By recognizing its flexibility in inserting single or multiple elements at defined positions, along with following best practices concerning memory management and avoiding duplicates, you can write more efficient and cleaner C++ code. Don't hesitate to explore more resources and practice examples to master vectors and their functionalities further.