In C++, the `const` keyword is used to define variables whose values cannot be changed after initialization, promoting safer and more predictable code.
Here's a code snippet demonstrating the use of `const`:
#include <iostream>
int main() {
const int maxValue = 100; // maxValue cannot be modified
std::cout << "The maximum value is: " << maxValue << std::endl;
// maxValue = 200; // Uncommenting this line will cause a compilation error
return 0;
}
Understanding `const` in C++
What is `const`?
The `const` keyword in C++ is fundamental in creating read-only variables. When you declare something as `const`, you are effectively telling the compiler, "This value should not be modified." This immutability serves not only to protect variable integrity but also aids in making the code more predictable and easier to understand.
How `const` Works
`const` serves as a gatekeeper in memory management. When variables are declared as `const`, the compiler ensures that these variables cannot be altered inadvertently during the execution of the program. This creates safer code and reduces bugs related to unintended modifications. Additionally, `const` offers compilation-time checks, providing earlier error detection while debugging.

Types of `const`
Constant Variables
Constant variables are simply variables with values that cannot be changed once assigned.
Syntax: To declare a constant variable, use the `const` keyword followed by the data type and variable name.
For example:
const int a = 10;
In this code snippet, `a` is a constant integer initialized to 10. Any attempt to modify `a` later in the code will result in a compilation error.
Constant Pointers
Pointers in C++ are variables that store memory addresses. However, when you introduce `const` into the mix, you get two fascinating concepts: constant pointers and pointers to constants.
- Constant Pointer: A constant pointer cannot be made to point to a different address once initialized. For example:
int *const ptr = &a; // 'ptr' cannot change to point to another address
In this snippet, `ptr` will always point to the same location in memory, even though the value it points to can change.
- Pointer to Constant: This type of pointer means you cannot change the value at the address it points to, but you can point to different addresses. For instance:
const int *ptr = &a; // 'ptr' cannot change the value of 'a'
In this case, while `ptr` can point to different `const int` values, it cannot modify the values themselves.
Constant Member Functions
Constant member functions in C++ play a key role in class design. By declaring a member function as `const`, you are essentially stating that this function will not modify any member variables of the class.
For example:
class MyClass {
public:
void display() const {
// This method cannot modify member variables
}
};
Using `const` with member functions improves safety and clarity, ensuring that certain methods do not lead to state changes.
Constant Objects
Constant objects are instances of classes that cannot be modified after their instantiation. This can add a layer of safety when you pass objects around in your program.
For example:
const MyClass obj;
Here, `obj` is a constant object of type `MyClass`. Any attempt to modify `obj` will result in a compilation error, ensuring that the state cannot be altered.
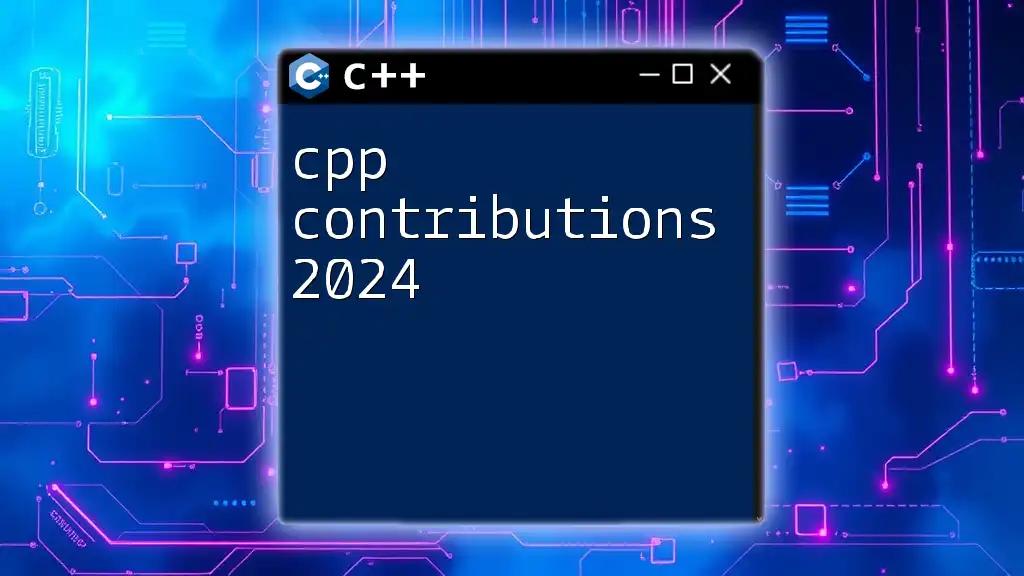
Best Practices for Using `const`
Benefits of Using `const`
Utilizing the `const` keyword extends several benefits:
- Safety and Security: It prevents accidental changes to variables, which is a common source of bugs.
- Improved Readability: When code uses `const`, other developers (or future you) can quickly see which variables should remain unchanged, making the code easier to follow.
Common Pitfalls
While there are clear advantages to using `const`, overusing it can lead to cumbersome code. For instance, declaring every variable as `const` devoid of context may reduce flexibility unnecessarily. Furthermore, understanding pointers with `const` can be confusing. Misinterpreting constant pointers and pointers to constants can lead to bugs, so it's crucial to grasp their distinct meanings thoroughly.
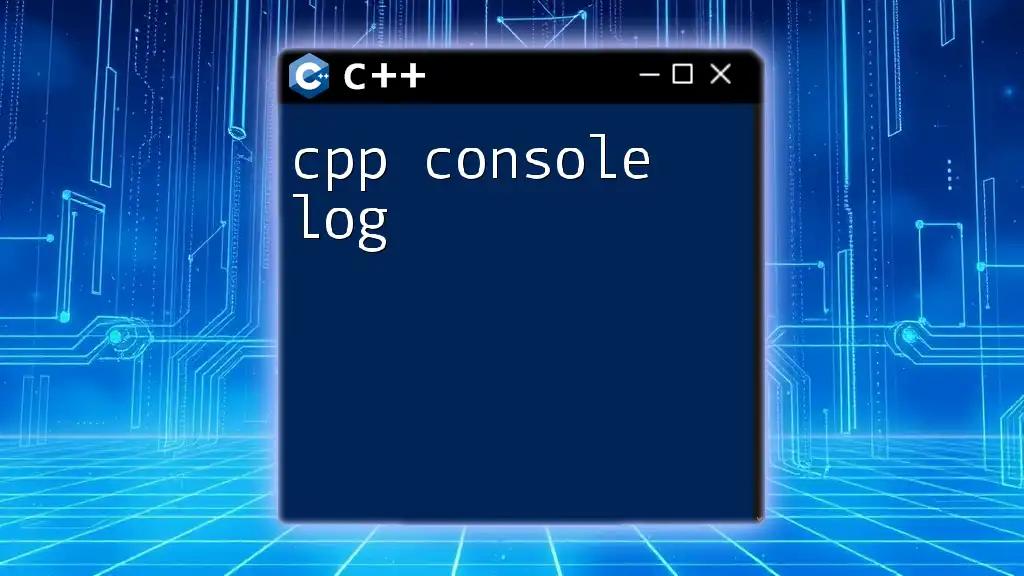
`const` and Function Parameters
Passing by Value vs. Passing by Reference
When it comes to function parameters, passing by value creates a copy of the actual variable. However, when you pass using `const reference`, you prevent copying while also ensuring the original variable remains unmodified.
Using a `const` reference, for example:
void processData(const MyClass &obj);
This method tells the compiler that `obj` should not be altered inside the function, saving overhead and protecting the data.
Benefits of Using `const` in Function Parameters
The use of `const` in function parameters leads to significant performance gains. When working with large objects, passing them by const reference instead of by value saves time and system resources. It also ensures that the input data remains unchanged, offering a safeguard against unintentional side effects.
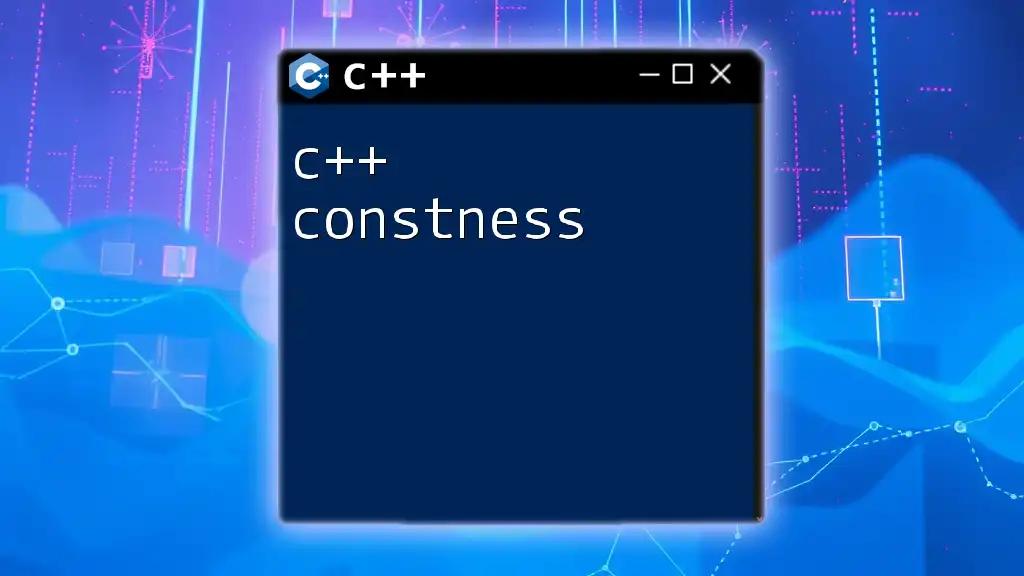
`const` vs `constexpr`
Understanding the Difference
While both `const` and `constexpr` enforce immutability, they serve different purposes.
- `const` signifies that the value of a variable will not change, but that value can be determined at runtime.
- **`constexpr`, on the other hand, indicates that the value must be known at compile time.
For instance:
const int x = 10; // value known at runtime
constexpr int y = 10; // value known at compile time
Using `constexpr` is beneficial in contexts where compile-time evaluation can improve efficiency, such as in template programming.
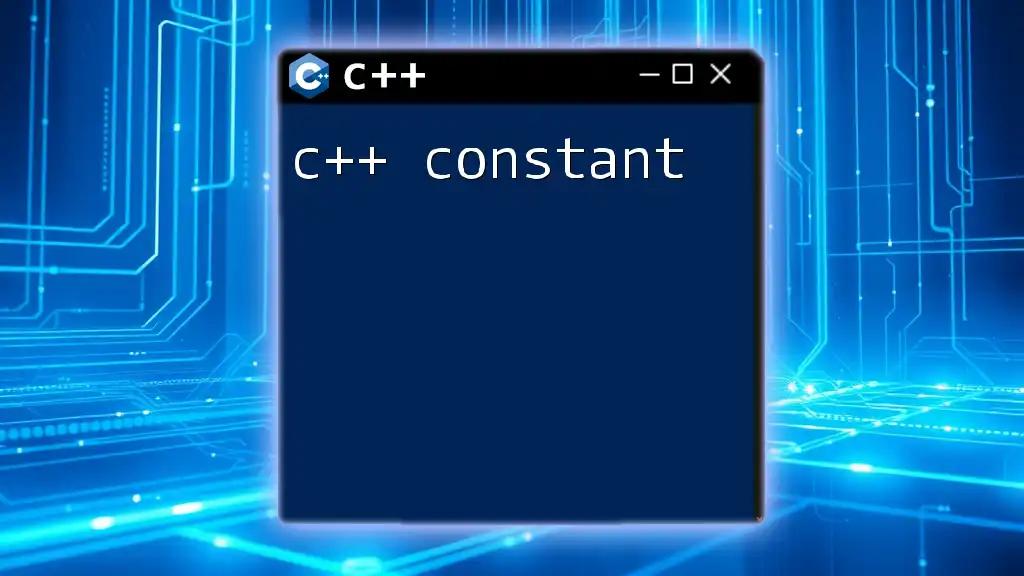
Conclusion
In summary, understanding and utilizing the keyword `const` in C++ are crucial for writing effective and maintainable code. It enforces immutability, enhances code readability, and reduces the risk of unintended errors. By adhering to best practices and knowing when to incorporate `const`, both new and experienced developers can leverage its full potential.
Call to Action
As you continue your journey in mastering C++, consider applying the principles of `const` in your projects. This simple yet powerful keyword can elevate your code quality and reliability. Explore additional resources or enroll in courses focused on deepening your C++ knowledge, and join communities where you can further hone your skills!

Additional Resources
Further Reading
To deepen your understanding of `cpp const`, consider engaging with C++ documentation and various tutorials. These resources can provide insights into best practices and advanced concepts.
Community and Support
Don’t forget to join forums or communities dedicated to C++ development where you can interact with other enthusiasts, share knowledge, and gain further insights into the language’s intricacies. The more you engage, the more proficient you will become!