In C++, `const int` declares an integer constant whose value cannot be changed after initialization, ensuring that the variable remains immutable throughout its scope. Here's a code snippet demonstrating its usage:
#include <iostream>
int main() {
const int MAX_VALUE = 100; // Declare a constant integer
std::cout << "The maximum value is: " << MAX_VALUE << std::endl;
// MAX_VALUE = 200; // Uncommenting this line will cause a compilation error
return 0;
}
What is const int?
In C++, `const int` is a declaration that defines a constant integer variable. When you declare an integer as `const`, it indicates that the value of this variable cannot be modified after it is initialized. This provides a means of safeguarding your code against unintended changes, ensuring that certain values remain fixed throughout the program's execution.
Key Characteristics of const int
- Immutability: Once a `const int` variable is assigned a value, it cannot be altered. This helps prevent accidental changes that could lead to bugs.
- Constant Expressions: `const int` can be used in constant expressions (e.g., as array sizes or in case statements), thus facilitating more efficient code execution.
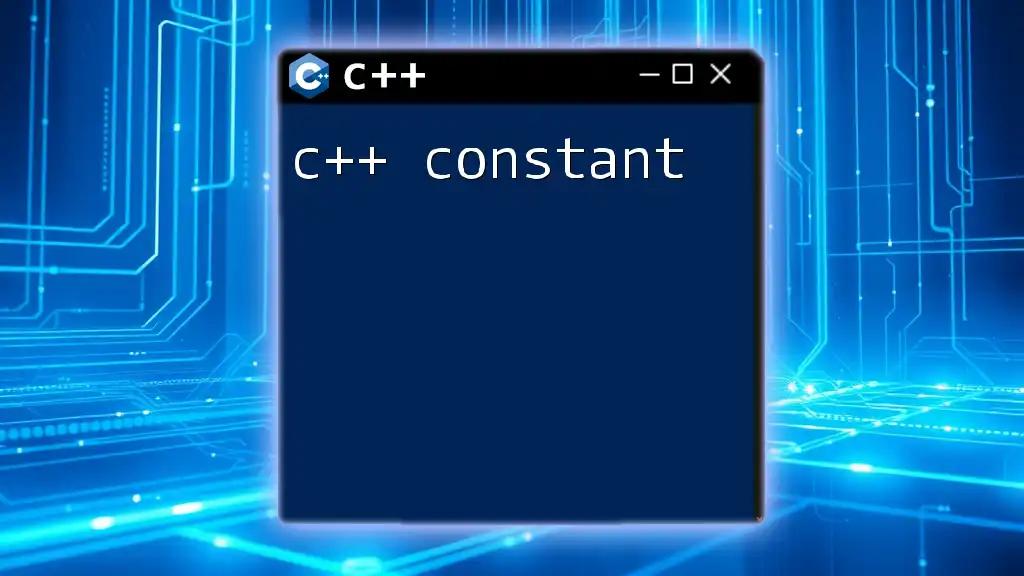
Why Use const int?
Benefits of Using const int
Utilizing `const int` brings numerous advantages, especially for software development:
- Enhanced Code Safety: By marking a variable as `const`, you safeguard it against changes that might compromise the program’s logic. This acts as a safeguard, increasing reliability.
- Improved Readability: Code maintenance and readability improve significantly. Others (or future you) can quickly identify constants that have been defined, leading to clearer intent within the code.
- Optimization: Compilers can optimize `const` variables more efficiently, as their immutability allows for potential enhancements in resource management.
Real-world Scenarios for const int
- Mathematical Constants: When working with mathematical operations, constants such as the value of Pi might be defined as `const int` to prevent modifications.
const int PI = 3; // Often Pi is defined as 3.14 for more precision, but this serves as an example.
- Fixed Configurations: Applications that require fixed settings—like the maximum number of users allowed in a system—can benefit significantly from using `const int`.
const int MAX_USERS = 50;
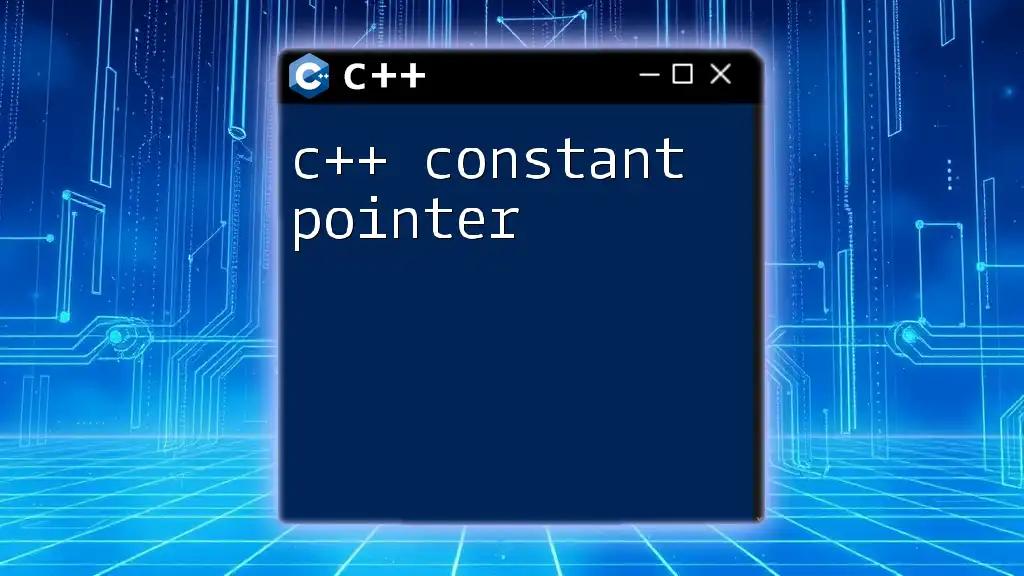
Declaring const int
Basic Syntax of const int
To declare a `const int`, the syntax is straightforward. The keyword `const` precedes the data type followed by the variable name and its assigned value:
const int MAX_VALUE = 100;
Initialization of const int
The initialization is crucial as a `const int` must be assigned a value at the time of declaration. Failing to do so will result in a compile-time error. Consider the following example:
const int DAYS_IN_WEEK = 7; // Proper initialization at declaration
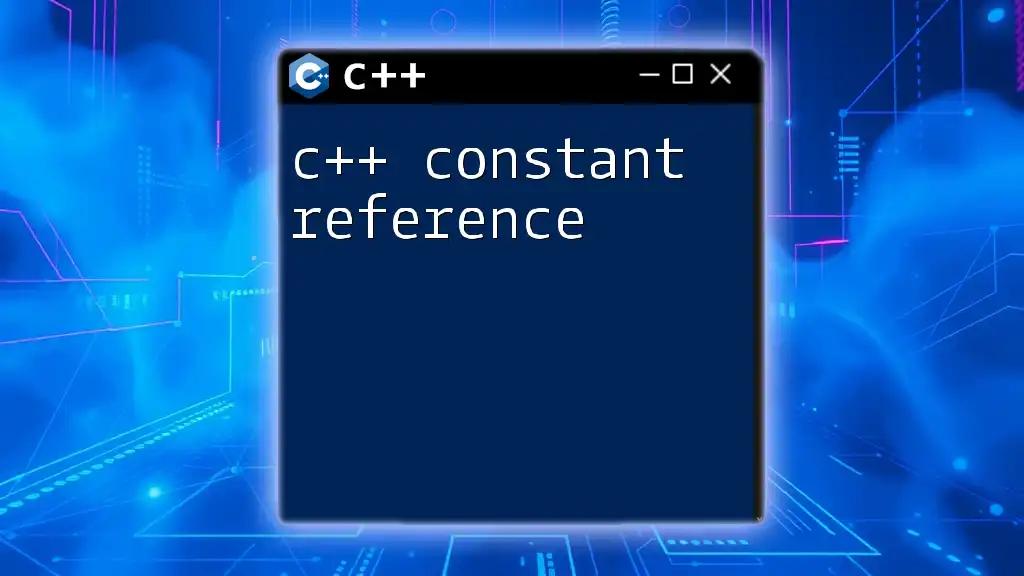
Using const int in Functions
Passing const int as Function Parameters
When you pass a `const int` as a parameter to a function, it indicates that the function will not modify the value. This is important for preserving the integrity of the data. Here’s an example:
void printMax(const int max) {
std::cout << "The maximum value is: " << max << std::endl;
}
In the above function, `max` cannot be changed within `printMax`, reinforcing the function's reliability.
Returning const int from Functions
You can also have a function return a `const int`, which can be useful when returning fixed values:
const int getConstantValue() {
return 42; // A constant value returned, ensuring it cannot be altered.
}
This practice also allows you to communicate to the function caller that the returned value is inherently fixed.
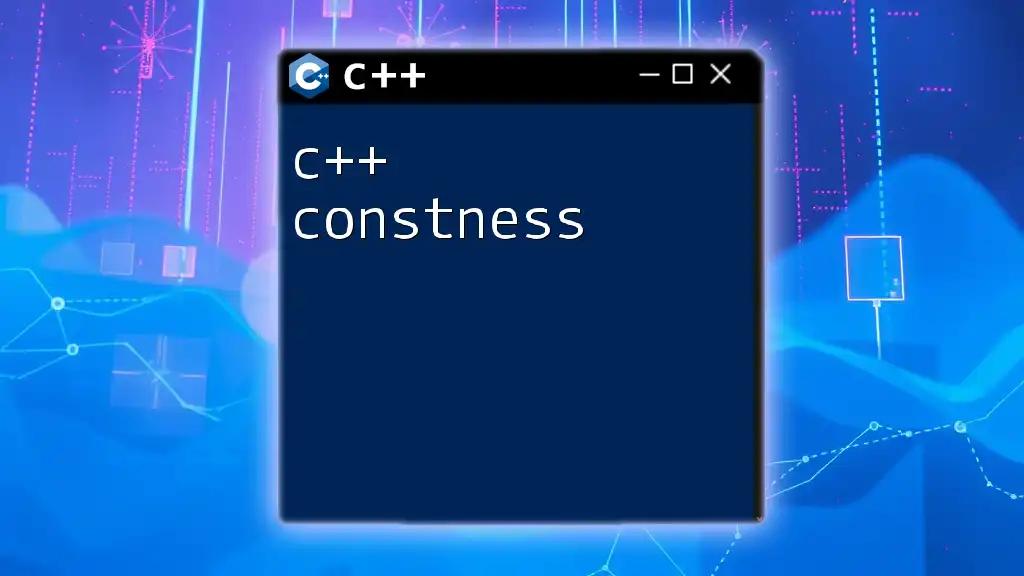
Constness and Pointers
Pointers to const int
Working with pointers and `const` can be complex but is also powerful. A pointer to a `const int` allows you to reference constant integer values without the ability to modify them:
const int* ptr = &MAX_VALUE; // Pointer to a constant integer
In this case, while `ptr` can point to `MAX_VALUE`, it cannot alter its value, thereby maintaining its integrity.
const int with References
Using `const` references can enhance efficiency, especially with larger data types. For instance, when you want to avoid copying data but still protect against modifications, you can use:
void displayValue(const int &value) {
std::cout << "Value is: " << value << std::endl;
}
This method emphasizes that `value` will not be modified while improving performance by avoiding copying.

Common Mistakes with const int
Misunderstanding const
A prevalent misunderstanding is assuming that `const` merely suggests the intent to refrain from modification. It is crucial to remember that `const` enforces restrictions entirely; if you try to change a `const int`, you will receive a compilation error.
Incorrect Usage of const int
Misusing the `const` keyword may lead to unexpected behaviors. Consider the following line:
const int FIXED = 10;
FIXED = 20; // Compilation error
This mistake emphasizes that once a value is assigned as `const`, any attempt to alter it will result in a compile-time error, reinforcing the necessity of understanding `const` attributes.
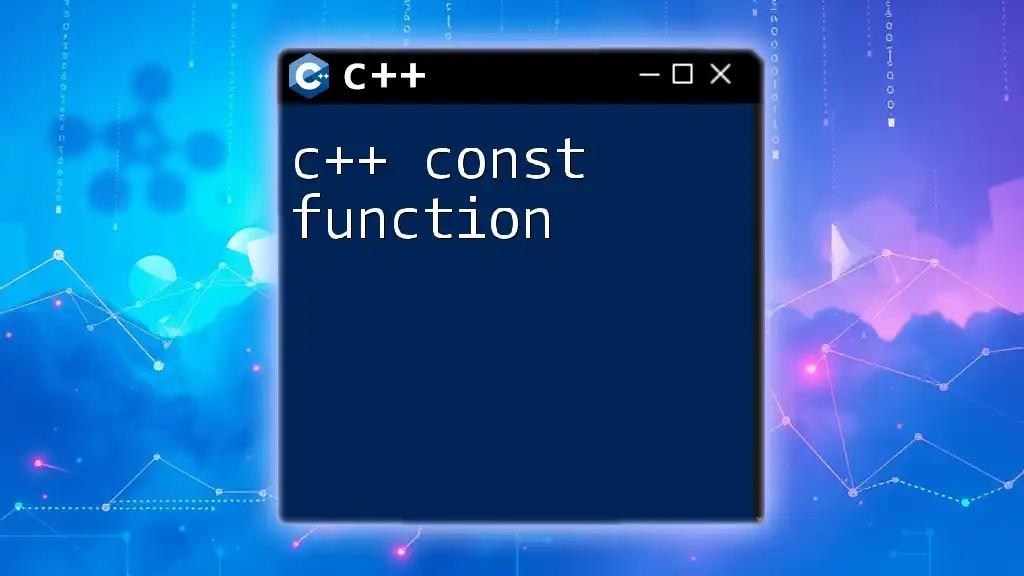
Best Practices for Using const int
To maximize the utility of `const int`, some best practices include:
- Use Descriptive Names: When naming constants, create intuitive names (e.g., `const int MAX_CONNECTIONS`) to make the intention clear.
- Group Related Constants: In larger applications, group related `const int` values together for better organization.
- Document Your Constants: Adding comments or documentation about why a value is defined as `const` can provide further clarity for future developers.

Conclusion
Incorporating `const int` into your C++ programming repertoire enhances code safety, readability, and performance. By recognizing the immutable nature of `const int`, you can design your code with greater reliability and maintainability. Use `const int` to define constants that are integral to your application’s operation, ensuring a smoother programming experience and fewer bugs over the code’s lifespan.
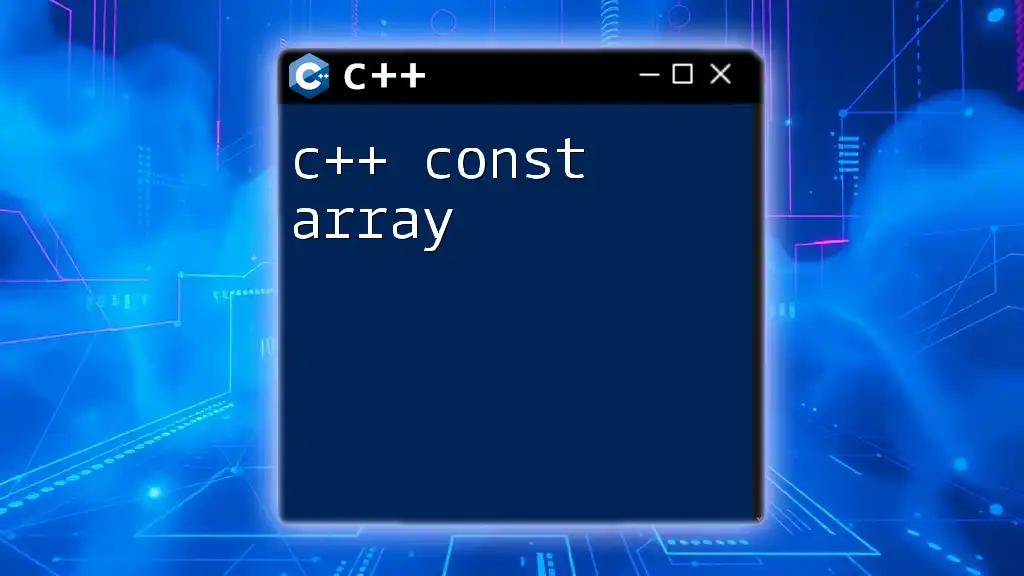
Additional Resources
To delve deeper into C++ programming and explore the nuances of `const int`, consider the following resources:
- Online courses and tutorials devoted to C++
- Recommended literature such as "Effective C++" by Scott Meyers, which offers insights into best practices
- Community forums and documentation for collaborative learning and troubleshooting
By leveraging these tools, you can further solidify your understanding and application of `const int` in various coding scenarios.