The C++ cosine function calculates the cosine of a given angle (in radians) using the `cos` function from the `<cmath>` library.
Here's a simple code snippet demonstrating its usage:
#include <iostream>
#include <cmath>
int main() {
double angle = 0.5; // angle in radians
double cosineValue = cos(angle);
std::cout << "The cosine of " << angle << " is " << cosineValue << std::endl;
return 0;
}
What is the Cosine Function?
The cosine function is a fundamental trigonometric function that relates the angle of a right triangle to the ratio of the adjacent side to the hypotenuse. In programming, particularly in C++, understanding how to use the cosine function is essential for tasks involving periodic functions, rotations, and wave simulations.
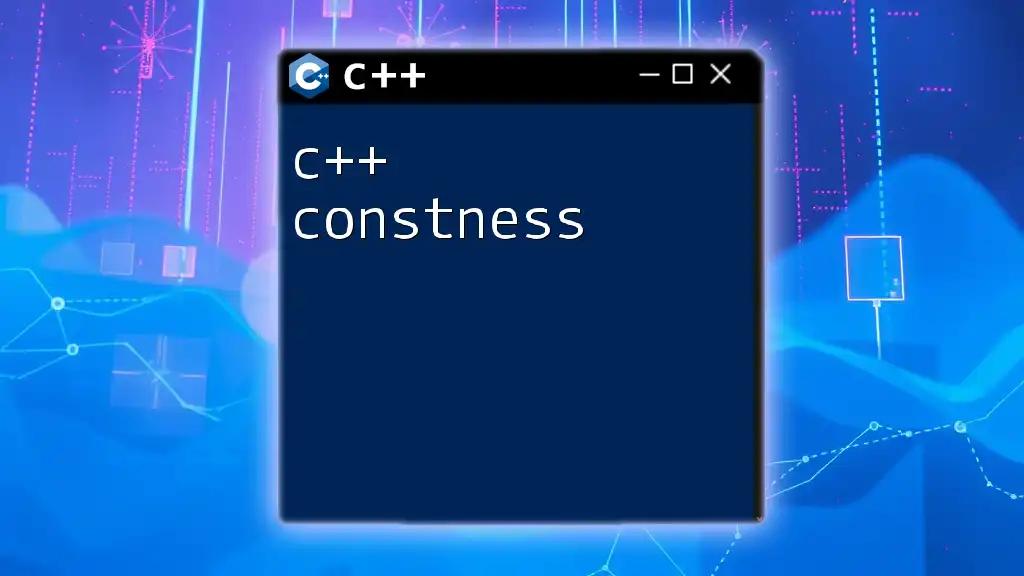
Overview of C++ Math Library
To utilize cosine and other mathematical functions in C++, you need to include the `<cmath>` header file. This library provides built-in functions for performing complex mathematical calculations, making it easier to implement formulas in your code without having to define them from scratch.
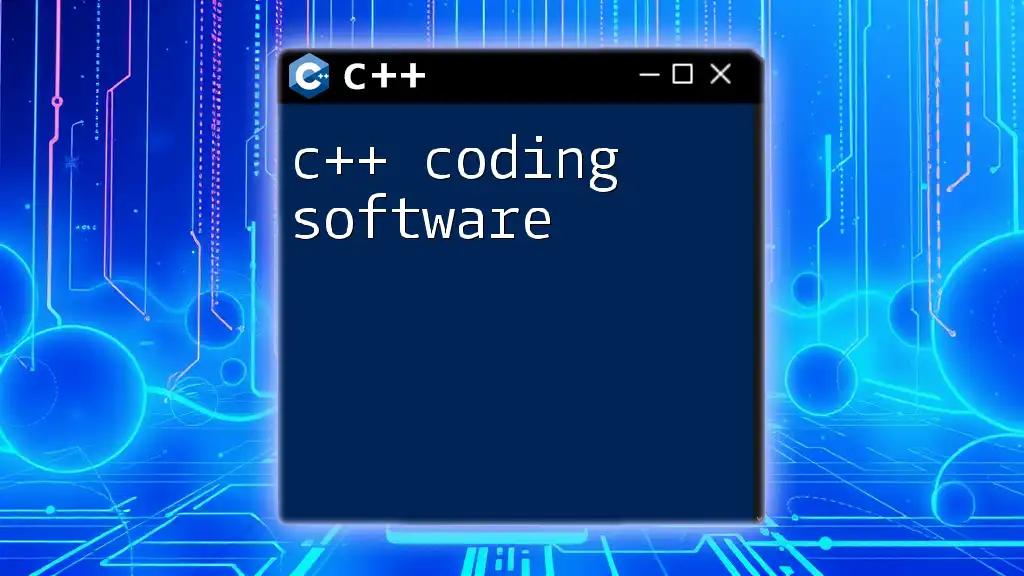
Syntax of the Cosine Function
The syntax for using the cosine function in C++ is straightforward:
double cos(double x);
Parameters and Return Value
The `cos()` function takes one parameter, which is the angle in radians, and returns the cosine of that angle as a double-precision floating-point number. This means that when you pass an angle to `cos()`, it will provide a value from -1 to 1, representing the cosine of the angle.
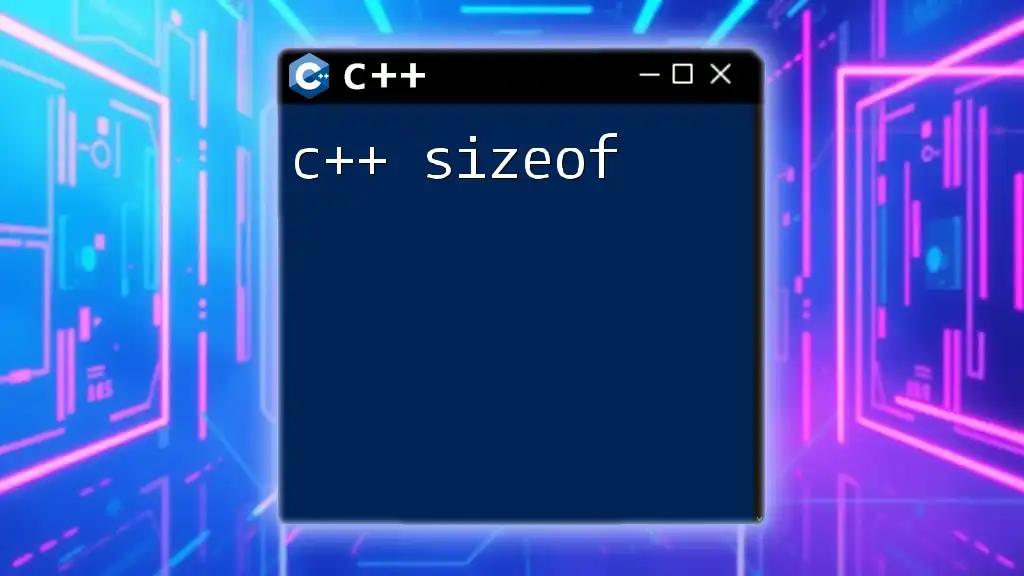
How to Use the Cosine Function
Including the Required Header
To start using the cosine function, you need to include the `<cmath>` library in your code:
#include <cmath>
Basic Usage of the `cos()` Function
Here’s a simple example demonstrating how to compute the cosine of an angle:
#include <iostream>
#include <cmath>
int main() {
double angle = 0; // Angle in radians
double result = cos(angle); // Calculate cosine
std::cout << "Cosine of " << angle << " is: " << result << std::endl;
return 0;
}
In this example, we calculate the cosine of 0 radians, which results in a value of 1, confirming the property of the cosine function.
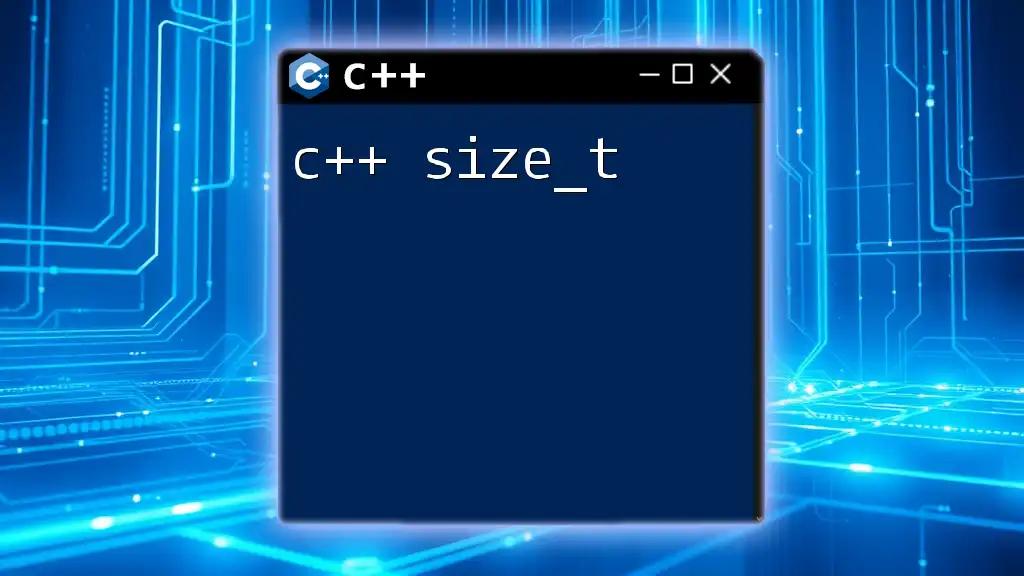
Practical Examples of Using Cosine Function
Example 1: Calculating Cosine of Multiple Angles
To illustrate the `cos()` function further, you can compute the cosine values for various angles using a loop. This allows you to easily see the changes in cosine as the angle varies:
#include <iostream>
#include <cmath>
int main() {
const int numAngles = 5;
double angles[numAngles] = {0, M_PI/6, M_PI/4, M_PI/3, M_PI/2};
for(int i = 0; i < numAngles; ++i) {
std::cout << "Cosine of " << angles[i] << " is: " << cos(angles[i]) << std::endl;
}
return 0;
}
In this example, we define an array of angles in radians and loop through it, calculating the cosine for each angle, providing important insights into how the cosine function behaves across different values.
Example 2: Using Cosine Function in a Real-World Scenario
The cosine function can be applied in various real-world contexts, such as determining the horizontal component of a force or vector. For instance, if we have a vector with a certain magnitude and angle, we can use the cosine function to find its horizontal component:
#include <iostream>
#include <cmath>
int main() {
double magnitude = 10.0; // Vector magnitude
double angle = M_PI / 4; // 45 degrees in radians
double horizontalComponent = magnitude * cos(angle);
std::cout << "Horizontal component: " << horizontalComponent << std::endl;
return 0;
}
In this example, we find the horizontal component of a vector with a magnitude of 10 at a 45-degree angle, which results in a value that helps inform decisions in physics and engineering problems.
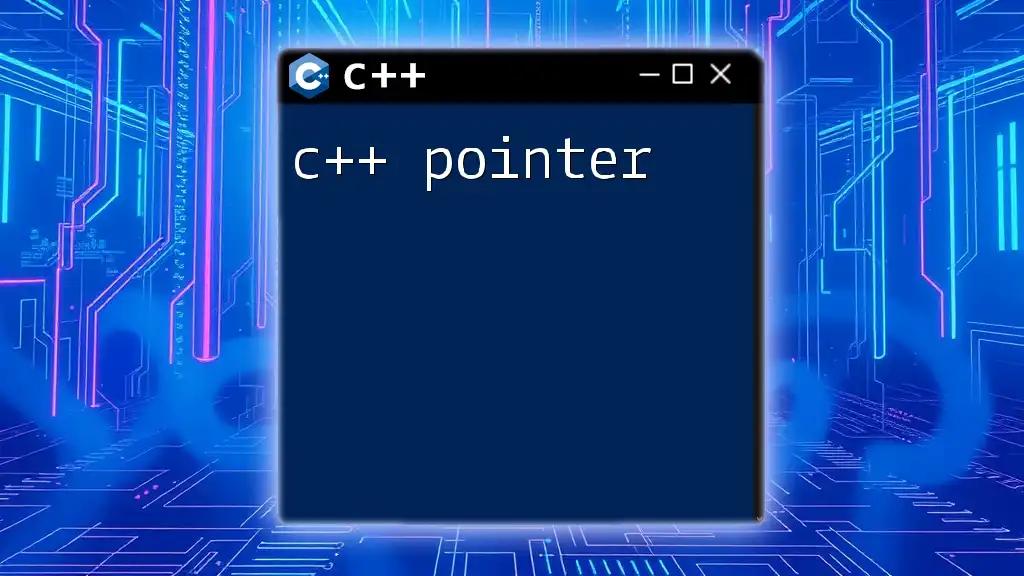
Common Pitfalls and Best Practices
Working with Radians vs. Degrees
One common pitfall when using the `cos()` function is neglecting that the function expects the angle in radians rather than degrees. To convert degrees to radians, you can use the following formula:
double degreesToRadians(double degrees) {
return degrees * (M_PI / 180.0);
}
Handling Precision Issues
When working with floating-point numbers in C++, precision can become an issue due to the nature of binary representations. It’s important to be mindful of how your calculations might introduce small errors, particularly when performing operations like additions or subtractions on very close values.
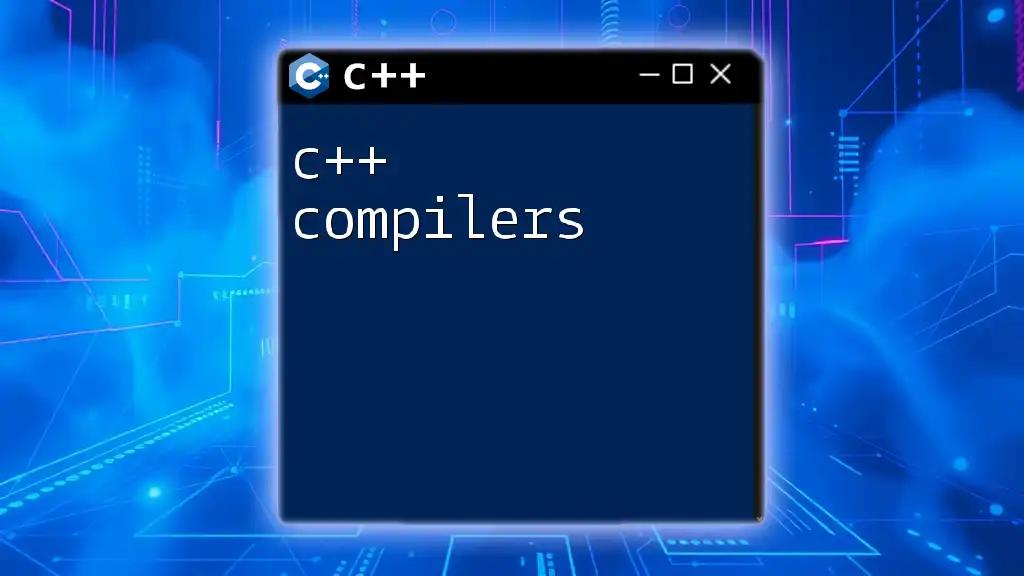
Applications of the Cosine Function
In Computer Graphics
In the realm of computer graphics, the cosine function is often used for rendering circles and animations. By controlling the x and y coordinates through cosine and sine functions, developers can create smooth movements and rotations.
In Physics Simulations
Cosine plays a vital role in physics simulations, especially in modeling oscillatory motions such as waves. Wave functions often utilize cosine to describe the displacement of points over time.
In Signal Processing
In signal processing, cosine functions help transform signals and are integral to algorithms like the Discrete Cosine Transform (DCT), which is widely used in image and audio compression.
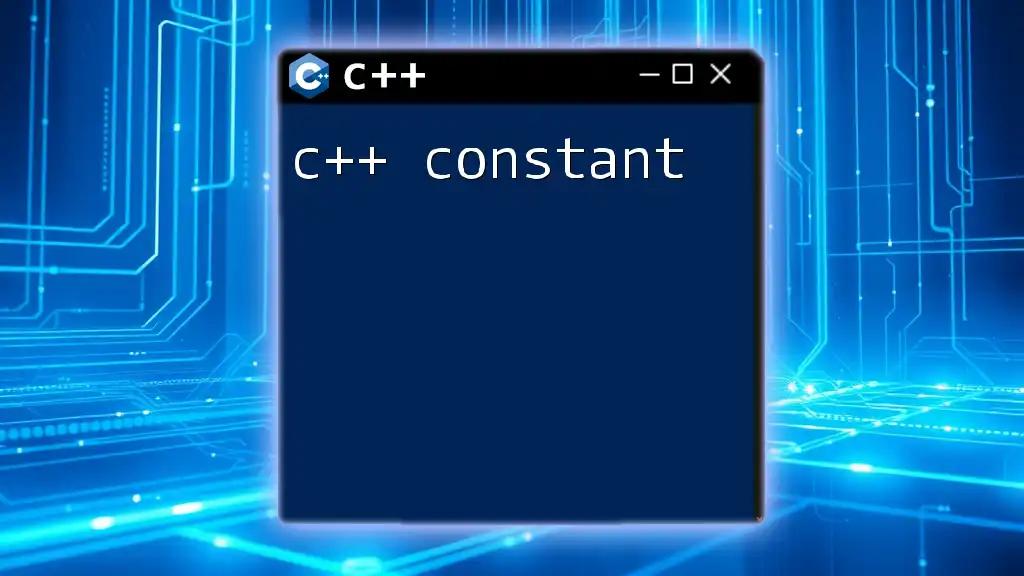
Conclusion
In summary, the C++ cosine function is a powerful tool for performing mathematical calculations related to angles. Whether you're working on graphics, physics, or other fields requiring precise calculations, understanding how to implement the `cos()` function effectively will enhance your programming skills. It’s important to explore further resources to deepen your grasp of not only cosine but the vast functionalities offered by the `<cmath>` library.
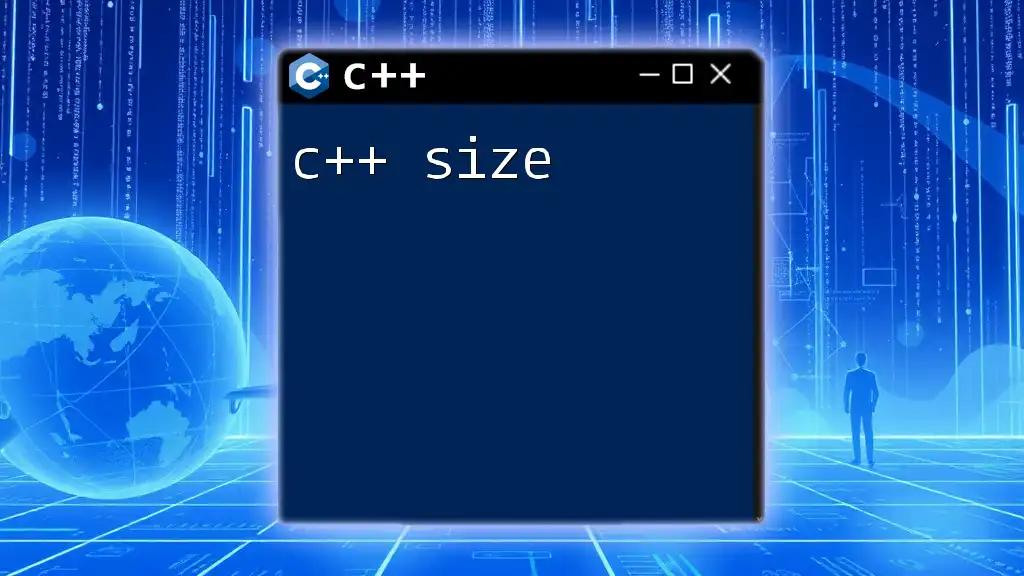
Frequently Asked Questions (FAQs)
What is the difference between `cos()` and `cosh()`?
The `cos()` function computes the cosine of an angle in radians, while `cosh()` computes the hyperbolic cosine. The two functions serve different purposes and are used in different mathematical contexts.
Why are the inputs in radians?
Radians are used in the functions due to their natural relationship with the unit circle, where one full rotation (360 degrees) corresponds to \(2\pi\) radians. This mathematical convention simplifies many calculations.
Can I use `cos()` with angles in degrees?
To use angles in degrees with the `cos()` function, you must first convert them to radians using the conversion method mentioned earlier. `cos(degreesToRadians(angle))` will allow you to get the desired result effortlessly.