C++ boilerplate refers to the standard code structure and components needed to set up a basic C++ program, allowing developers to focus on implementing their logic rather than worrying about repetitive tasks.
Here's a simple C++ boilerplate code snippet:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding the Structure of a C++ Program
Basic Components of a C++ Program
A `C++ boilerplate` effectively starts with understanding the basic components that form a C++ program. Here’s a breakdown of what you’ll typically find in your C++ code:
Preprocessor Directives: These are the commands that instruct the C++ compiler to include certain files before actual compilation. They serve as the foundation of your program.
#include <iostream>
In this example, the `<iostream>` library allows the program to perform input and output operations.
Namespaces: They help in avoiding naming conflicts. By declaring a namespace, you can specify the context of the identifiers you're using. For instance:
using namespace std;
This line ensures that you can use standard C++ features, such as `cout` and `cin`, without needing the `std::` prefix.
The Main Function
Every C++ program requires a `main()` function; it serves as the entry point of execution. This is where the program begins its run, and its return type typically indicates whether it completed successfully.
Here’s a simple example:
int main() {
return 0;
}
In this case, a return value of `0` generally signifies successful execution.
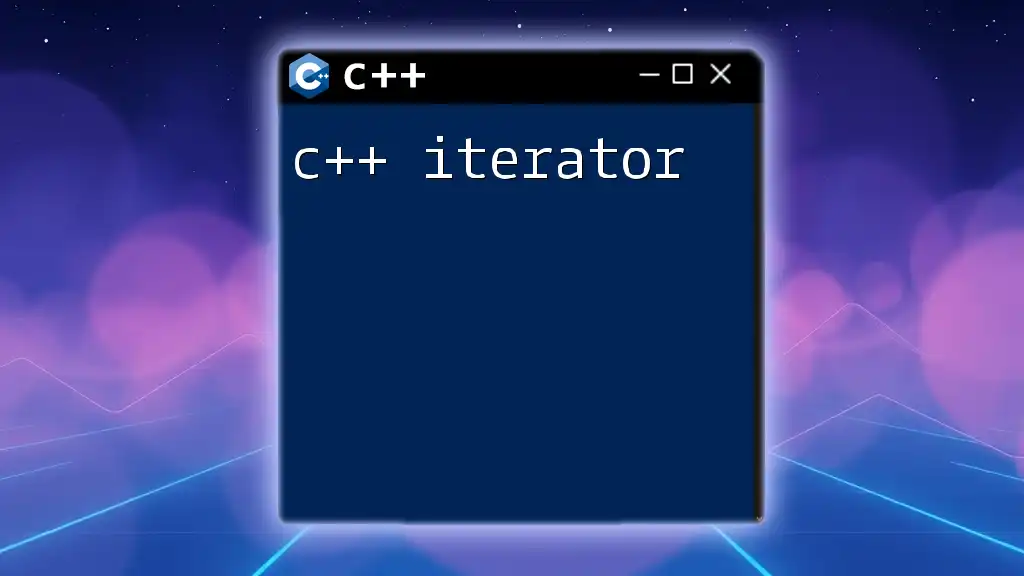
Essential Elements of a C++ Boilerplate
Including Libraries
One of the first steps when setting up your C++ boilerplate is to include the necessary libraries. Below are commonly utilized libraries that many developers include at the beginning of their programs:
#include <iostream>
#include <vector>
The `<vector>` library allows the use of dynamic arrays (vectors), which are essential in modern C++ programming.
Setup and Initialization
Following the inclusion of libraries, it’s beneficial to have a function dedicated to setup and initialization tasks. This function can be called at the beginning of `main()`, allowing the user to set necessary parameters or configurations.
void setup() {
// Initialization code here
}
This setup function could, for instance, initialize global variables or handle resource-loading tasks before the main program logic runs.
Error Handling
In robust programming, error handling cannot be overlooked. Using a basic `try-catch` structure allows developers to manage exceptions gracefully, making the program more resilient.
try {
// Code that may throw exceptions
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
Through this structure, you can define how your program responds to various errors, thus improving its stability and usability.
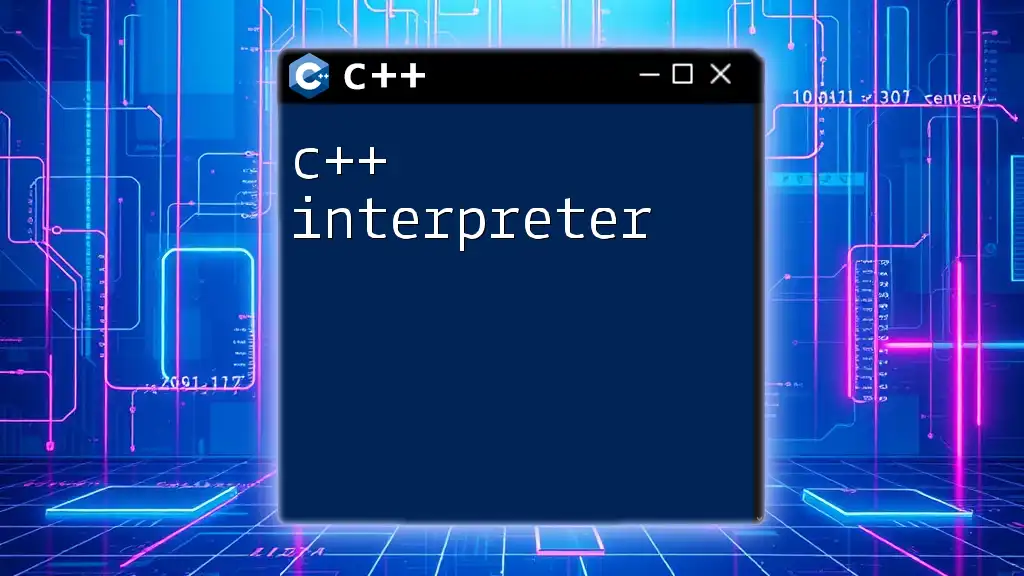
Basic C++ Boilerplate Template
Basic Template Example
Bringing together everything discussed, here’s a simple boilerplate template for a C++ program:
#include <iostream>
using namespace std;
void setup() {
// Initialization code here
}
int main() {
setup();
cout << "Hello, World!" << endl;
return 0;
}
This template introduces the structure seen in nearly all C++ programs while performing the basic function of outputting "Hello, World!" to the console.
Customizing Your Boilerplate
Your `C++ boilerplate` is not a one-size-fits-all solution. As your projects grow in complexity, you may need to customize your boilerplate by adding libraries specific to your needs or tweaking namespaces. Always ensure that your boilerplate reflects the core functionality of your particular application.
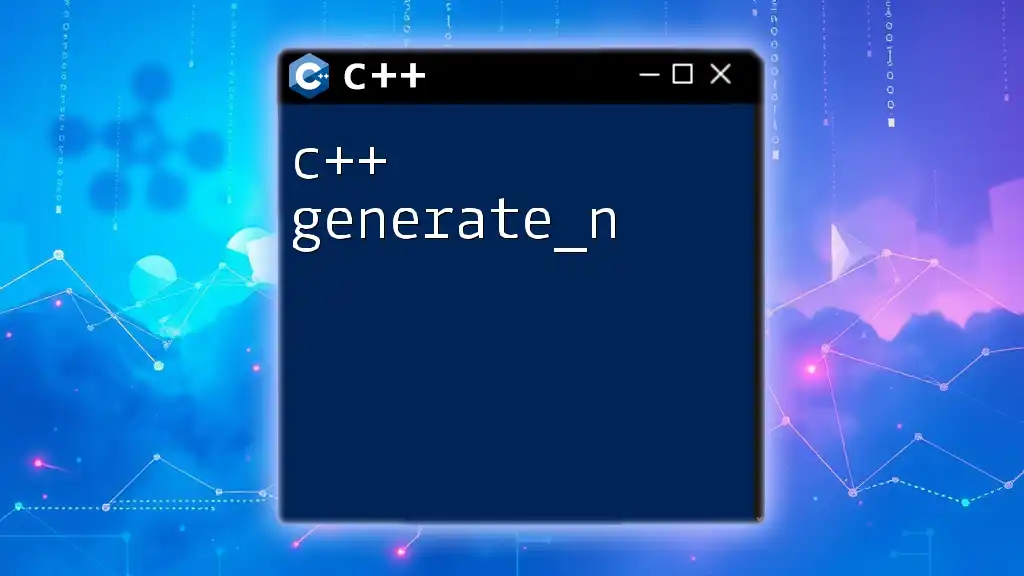
Advanced Boilerplate Techniques
Using Classes and Objects
As you dive deeper into C++, you will discover the power of object-oriented programming. Integrating classes into your boilerplate offers flexibility and organization.
class MyClass {
public:
void display() {
cout << "MyClass Display" << endl;
}
};
By creating objects from such classes, you can encapsulate functionality and maintain cleaner code.
Advanced Error Management
For professional projects, employing advanced error management strategies enhances the reliability of your software. You can create custom exceptions for specific scenarios:
class MyException : public std::exception {
public:
const char* what() const noexcept override {
return "Custom exception occurred";
}
};
This allows for refined error tracking within your application, giving a clearer context when things go wrong.
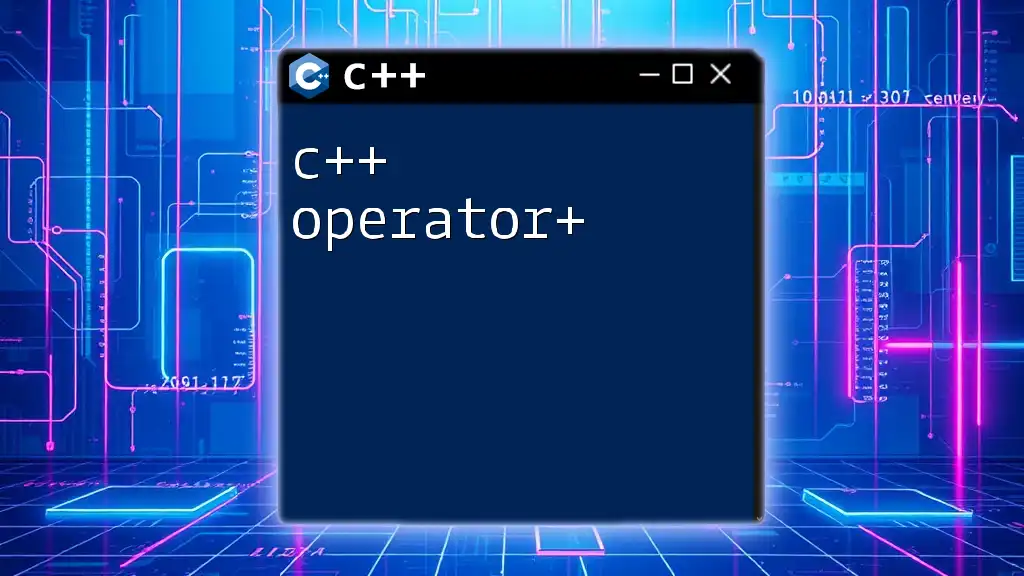
Tools for Creating and Managing Boilerplate
IDEs and Text Editors
Development environments offer various tools that aid in managing your C++ boilerplate effectively. Popular IDEs like Visual Studio, Eclipse, and CLion come with robust features that streamline the coding process. From syntax highlighting to debugging capabilities, they greatly enhance productivity.
Using Templates and Snippets
Many IDEs allow the creation of templates or code snippets, which can be a real time-saver when starting new projects. For example, in Visual Studio Code, you can create snippets that instantly populate boilerplate code:
cppmain: Creates a basic C++ main boilerplate
This feature expedites the development process, allowing you to focus on the unique aspects of your program.
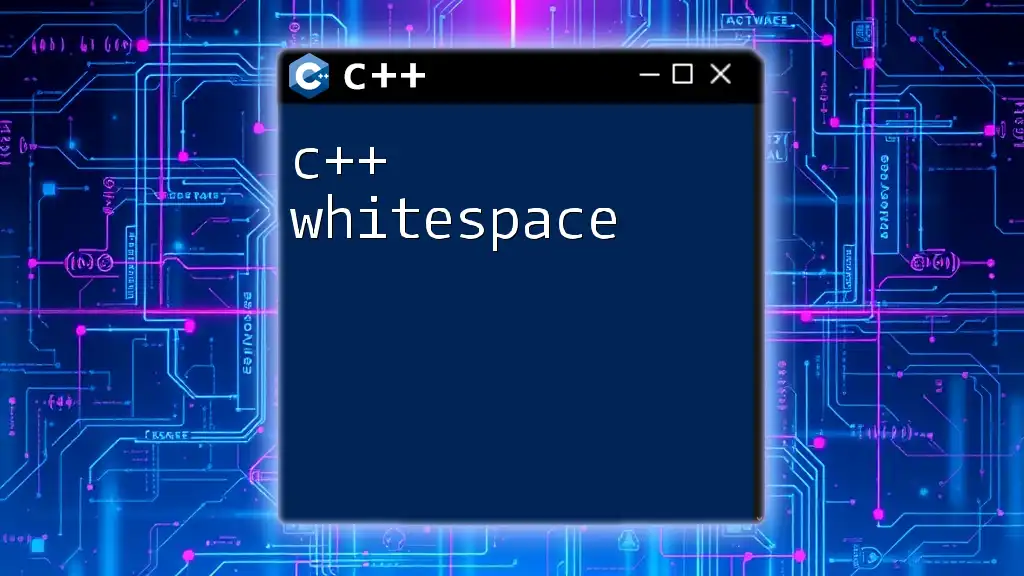
Best Practices for C++ Boilerplate Code
Keeping It Clean and Maintainable
Writing clean, well-documented code is paramount in software development. Comments should clearly explain the why behind complex logic, helping future developers—or even you—understand the code quickly when revisited. Utilize commenting best practices:
// This function initializes necessary components
void setup() {
Well-structured code enhances maintainability and reduces bugs.
Version Control and Collaboration
Utilizing version control systems, such as Git, is essential for managing your `C++ boilerplate` effectively, especially in collaborative settings. Version control helps to keep track of code changes, allowing you and your team to work on the same project without conflicts.
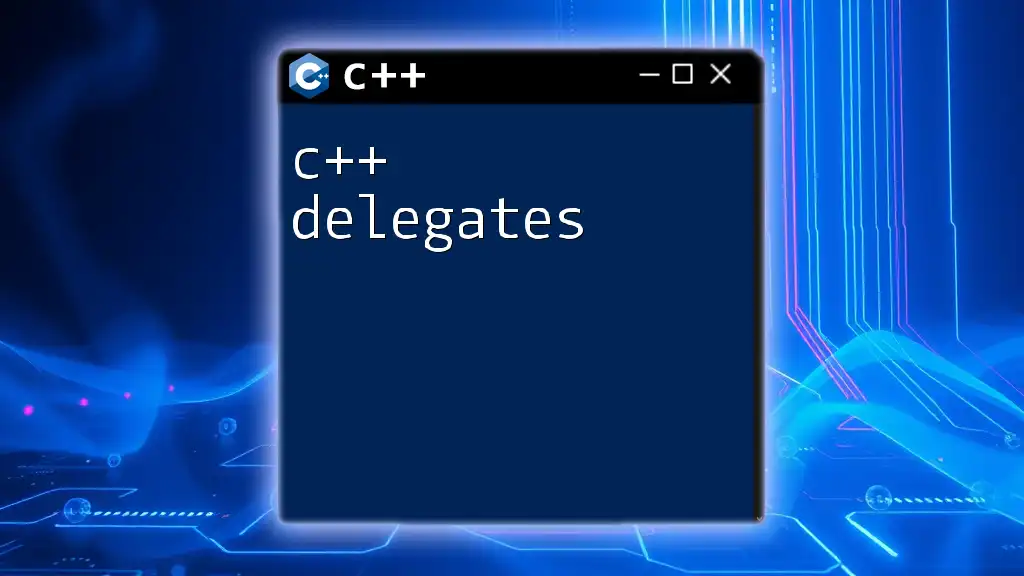
Conclusion
In summary, a well-crafted `C++ boilerplate` is vital for simplifying the development process while ensuring best practices in coding are observed. Understanding its fundamental components, structuring it correctly, and adopting advanced techniques empowers developers to create efficient and robust C++ applications. As you refine your boilerplate, remember to customize, collaborate, and keep learning. Your next project can always benefit from your insights and creativity—so do share your own boilerplate tips!
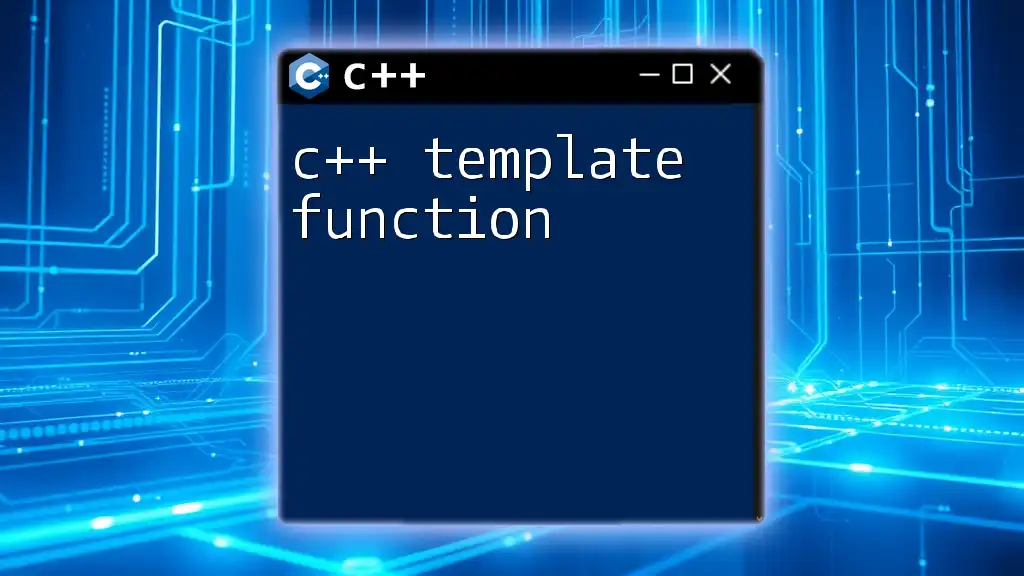
Additional Resources
To deepen your understanding and skills in C++, consider exploring various books, online courses, and reputable C++ forums. Engaging with ongoing learning opportunities will help further enhance your programming expertise.
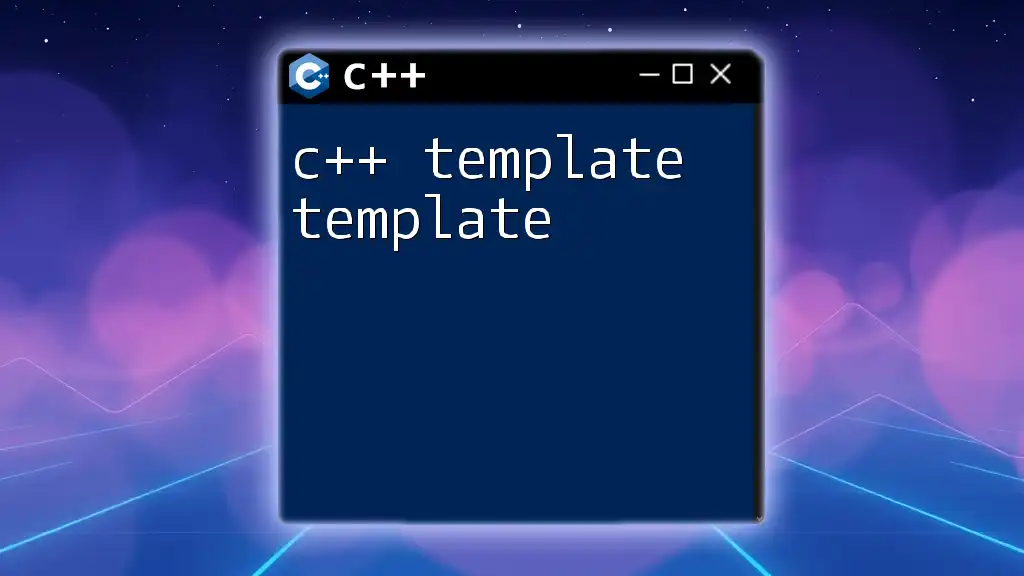
FAQs
As you progress, you might encounter common questions regarding `C++ boilerplate`. Don’t hesitate to seek answers or share your own experiences to foster a community of learning and growth.