The `std::generate_n` function in C++ generates a sequence of values by applying a specified generator function to produce a given number of elements.
Here’s a code snippet demonstrating its usage:
#include <iostream>
#include <vector>
#include <algorithm>
#include <random>
int main() {
std::vector<int> numbers(10);
std::generate_n(numbers.begin(), numbers.size(), []() { return rand() % 100; });
for (const auto& num : numbers) {
std::cout << num << " ";
}
return 0;
}
What is `std::generate_n`?
`std::generate_n` is a standard algorithm in C++ that allows you to generate a sequence of values and store them in a provided output iterator. This function is part of the `<algorithm>` header and is commonly used to fill a range with generated elements. Its syntax resembles that of a typical C++ function:
template<class OutputIterator, class Size, class Generator>
OutputIterator generate_n(OutputIterator first, Size n, Generator g);
The function's main purpose is to apply a generator (a callable object such as a function or a lambda) to produce values that are then assigned to a specified number of locations referenced by the output iterator. This makes `std::generate_n` an efficient way to initialize or modify collections with custom-generated data.
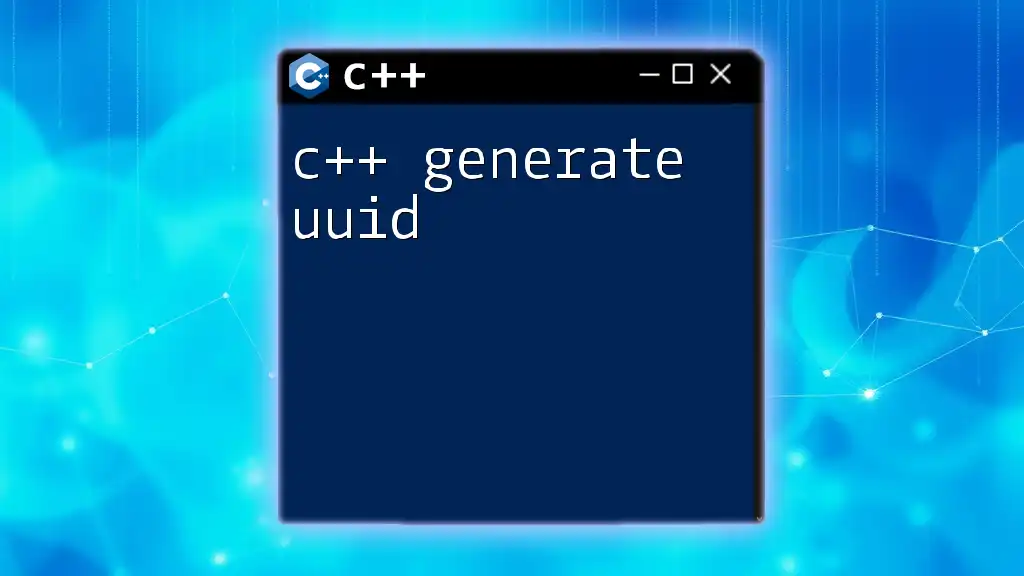
How `std::generate_n` Works
Parameters Explained
- OutputIterator: This represents the beginning of the destination range where the generated values will be stored. It could be a vector, a list, or any other type of output iterator.
- Size: This indicates the number of elements you want to generate.
- Generator: A callable object that generates the values. This can be a simple function, a lambda expression, or any functor.
Workings of the Function
The optimal functioning of `std::generate_n` begins with providing your desired output iterator, the count of how many elements you wish to produce, and the mechanism required for generating those values. The function then calls the generator repeatedly to obtain `n` values, each of which it places into the output range. At the end of this operation, the iterator returned points to the location where the next value would be inserted, allowing for further manipulation or filling.
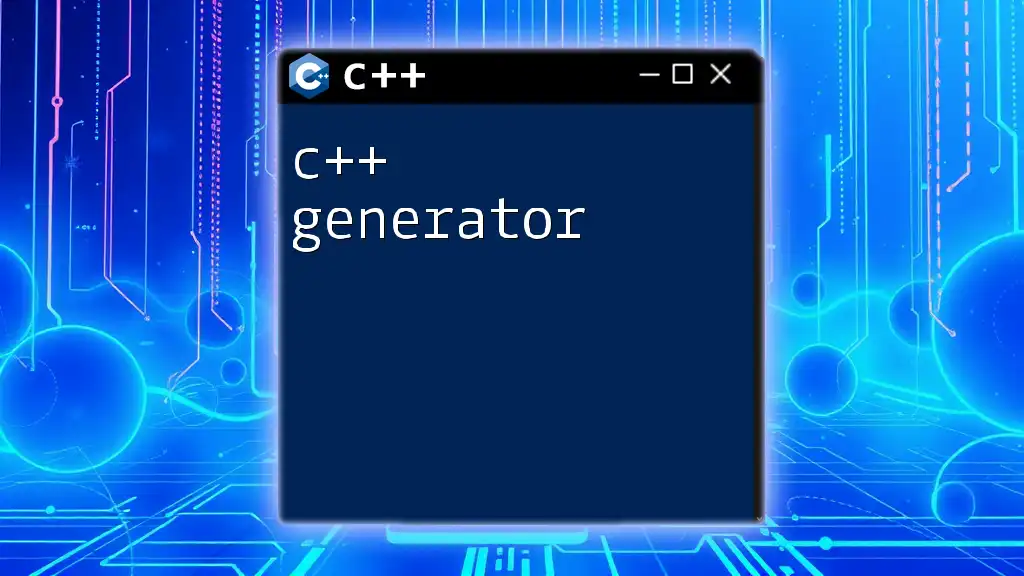
Practical Usage of `std::generate_n`
Basic Example
Consider a situation where you want to fill a vector with ten random integers. Here’s how you could employ `std::generate_n` to achieve that:
#include <iostream>
#include <vector>
#include <algorithm>
#include <cstdlib>
int main() {
std::vector<int> vec(10);
std::generate_n(vec.begin(), 10, []() { return rand() % 100; });
for (int n : vec) {
std::cout << n << ' ';
}
return 0;
}
In this code, we create a vector of ten integers and then use `std::generate_n` to fill the vector with random values from 0 to 99. The lambda function provided to `generate_n` is quite simple: it calls `rand() % 100` to generate a random integer within the desired range. The output of the program is a series of random numbers, illustrating the immediate utility and simplicity of using `std::generate_n`.
Advanced Example
Let’s say you want to generate a sequence of floating-point numbers uniformly distributed between 0.0 and 1.0. This can be accomplished with a more complex generator function. Here’s an example:
#include <iostream>
#include <vector>
#include <algorithm>
#include <random>
double generate_random_double() {
static std::default_random_engine e{};
static std::uniform_real_distribution<double> dist{0.0, 1.0};
return dist(e);
}
int main() {
std::vector<double> vec(5);
std::generate_n(vec.begin(), 5, generate_random_double);
for (double n : vec) {
std::cout << n << ' ';
}
return 0;
}
In this code snippet, we define a function `generate_random_double` that uses a random number generator to produce double values. The state of the random engine is kept static to ensure varied results across multiple calls. The call to `std::generate_n` fills up a vector of five floating-point numbers with these random values. When you run this program, you will see five random floating-point numbers displayed, reinforcing the versatility of `std::generate_n` in generating different types of data.
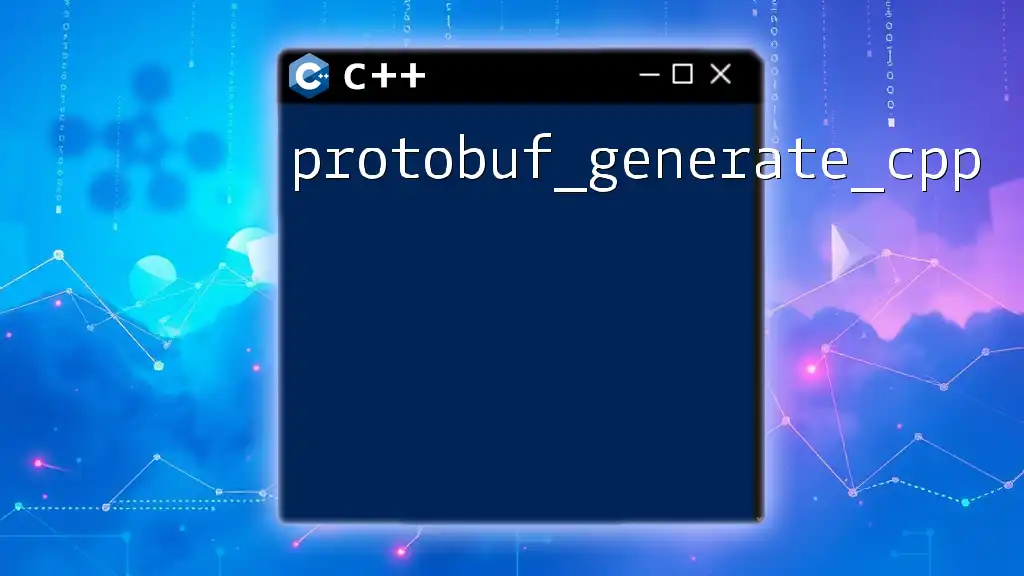
Performance Considerations
When to Use `std::generate_n`
Using `std::generate_n` is ideal in situations where you need to fill a container with values generated on-the-fly, as it avoids the overhead associated with manual loops and repetitive calls to the generator. This makes it not just cleaner, but also more efficient, especially when dealing with larger datasets. Furthermore, it encourages functional programming practices and can be combined seamlessly with other algorithms.
Complexity Analysis
The time complexity of `std::generate_n` is O(n), where n is the number of elements generated. Each call to the generator results in generating a single value, necessitating exactly n calls overall. The space complexity is O(1), as the function operates in place and doesn't require additional storage beyond what is already allocated for the output iterator. Understanding this complexity is critical for developers working on performance-sensitive applications.
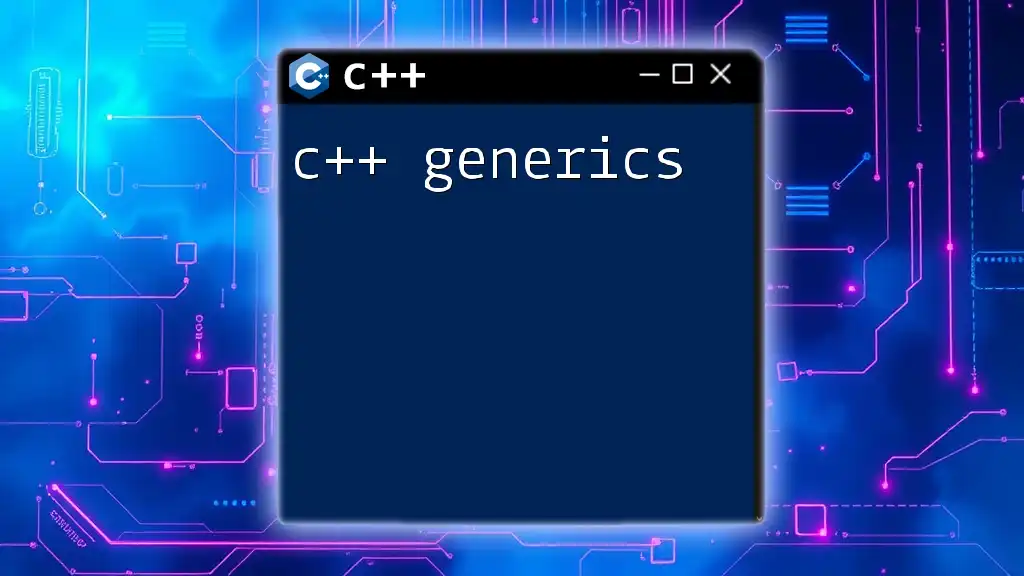
Common Mistakes and Pitfalls
Failures When Generating Too Many Elements
One of the common mistakes is attempting to generate more elements than the allocated space in the container. This can lead to undefined behavior or runtime errors. Always ensure that the output iterator has enough space allocated for the number of elements you're trying to generate. Consider using `.resize()` on vectors or preallocating storage if necessary.
Misusing Function Objects
Another prevalent issue arises from improperly using callable objects as generators. For instance, misconfigured lambdas or functions can result in unexpected behavior or repeated values. Always validate your generator to ensure it produces unique or intended values with each call. For example, if your generator shares state between calls, you may inadvertently generate the same value multiple times.
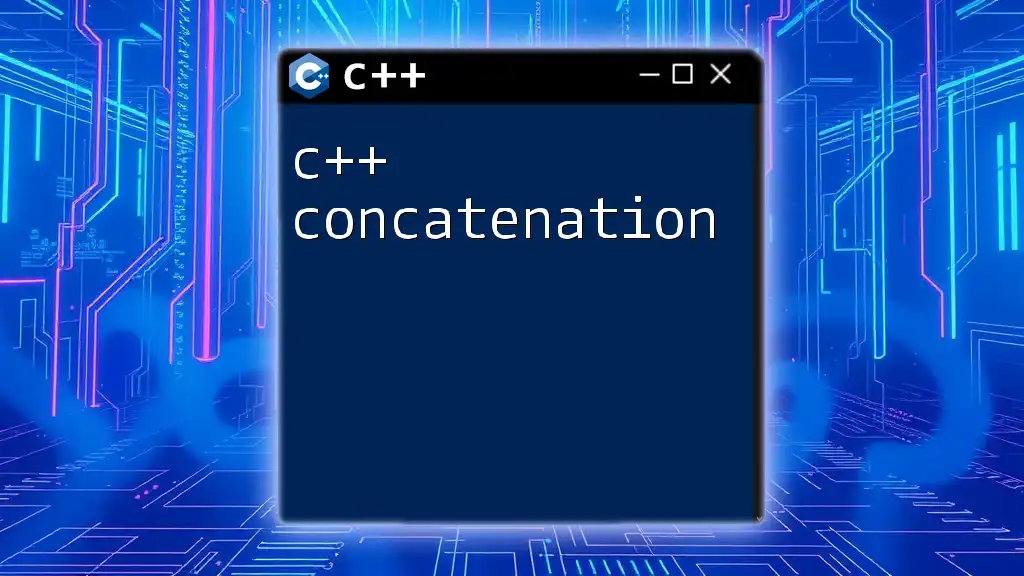
Conclusion
In summary, `std::generate_n` is a potent tool for generating sequences in C++. Its ability to accept a generator allows for flexibility and efficiency, making it applicable to a variety of scenarios—from initializing simple arrays to generating complex data types. As you explore C++, experimenting with `std::generate_n` can reveal new patterns and algorithms to enhance your coding experience. Dive deeper into the world of C++ algorithms and discover the many tools that can simplify your programming tasks and expand your capabilities as a developer.
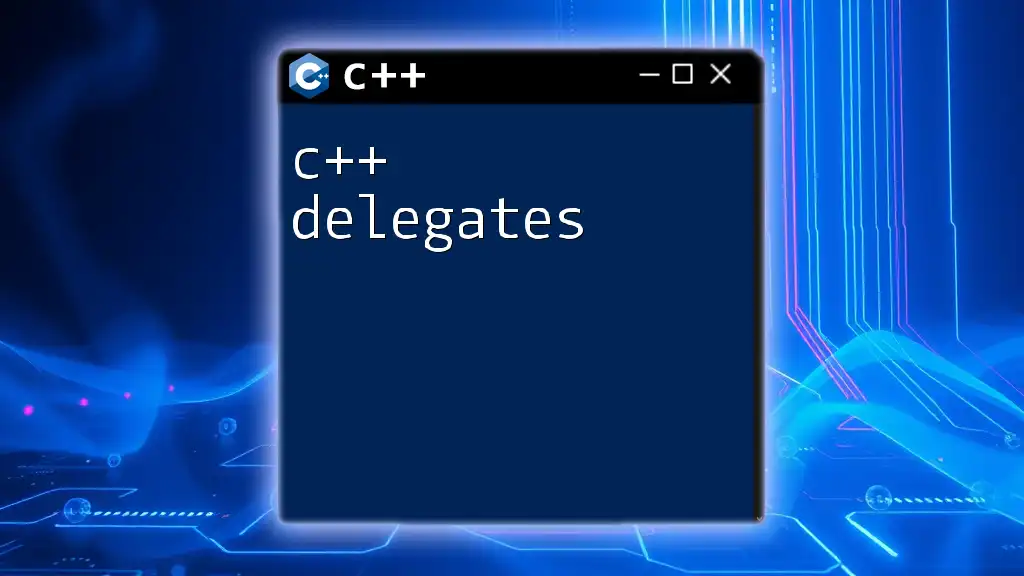
Further Resources
Books and Online Materials
For those looking to deepen their knowledge of C++, I recommend checking out classics like "Effective Modern C++" by Scott Meyers as well as online resources like the C++ reference documentation.
Community and Forums
Engaging with communities on platforms like Stack Overflow, Reddit’s r/cpp, or specialized C++ forums can help you overcome challenges, share insights, and grow your understanding of advanced C++ features, including `std::generate_n`.