A C++ generator is a function that simplifies the process of producing sequences of values, enabling efficient iteration over potentially infinite data streams through the use of coroutines.
Here’s a simple example of a C++ generator using a coroutine:
#include <iostream>
#include <coroutine>
#include <optional>
template <typename T>
struct Generator {
struct promise_type {
T value;
std::experimental::coroutine_handle<> continuation;
auto get_return_object() {
return Generator{std::experimental::coroutine_handle<promise_type>::from_promise(*this)};
}
auto yield_value(T val) {
value = val;
continuation.resume();
return std::experimental::suspend_always{};
}
void unhandled_exception() {
std::exit(1); // Handle error
}
void return_void() {}
};
std::experimental::coroutine_handle<promise_type> coroutine_handle;
explicit Generator(std::experimental::coroutine_handle<promise_type> h) : coroutine_handle(h) {}
~Generator() { coroutine_handle.destroy(); }
bool move_next() {
if (coroutine_handle.done()) return false;
coroutine_handle.resume();
return !coroutine_handle.done();
}
T current() {
return coroutine_handle.promise().value;
}
};
Generator<int> count_up_to(int max) {
for (int i = 1; i <= max; ++i) {
co_yield i; // Yield the current value
}
}
int main() {
auto generator = count_up_to(5);
while (generator.move_next()) {
std::cout << generator.current() << std::endl; // Output the generated value
}
return 0;
}
Understanding C++ Generators
What is a Generator?
A generator in C++ is a special type of function that allows you to pause its execution and yield a series of values, one at a time, instead of returning a single value and terminating. This concept stands out because it preserves the state between calls, making it useful for producing sequences of data, managing large datasets, or implementing asynchronous computations.
History of Generators in C++
The introduction of coroutines in C++20 marked a significant milestone for implementing generators. Coroutines allow functions to suspend execution and resume later, enabling efficient writing of generator logic.
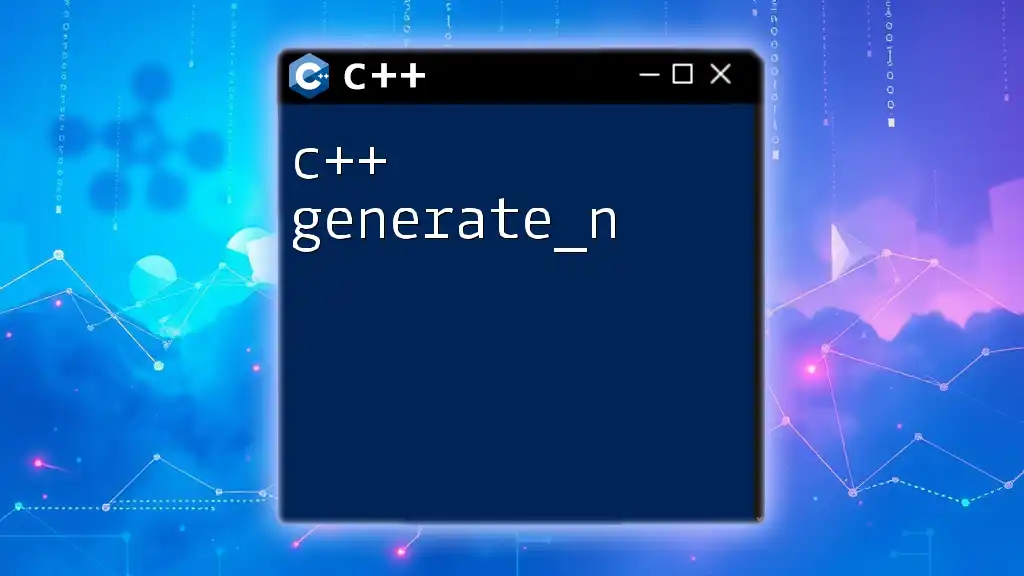
Key Concepts Behind Generators
Synchronous vs. Asynchronous Generators
Understanding the distinction between synchronous and asynchronous generators is essential:
-
Synchronous Generators: These generators produce values in a single thread, yielding them upon demand. They are straightforward and useful for most scenarios where the execution order matters.
-
Asynchronous Generators: These are capable of yielding values in a non-blocking manner, useful in applications where tasks can run concurrently, such as network requests or I/O operations.
Yielding Values
The `yield` keyword is central to the functioning of generators. Unlike a standard function that exits after returning a value, a generator can yield multiple values over time. Each time the generator is called, it resumes execution from where it last yielded.
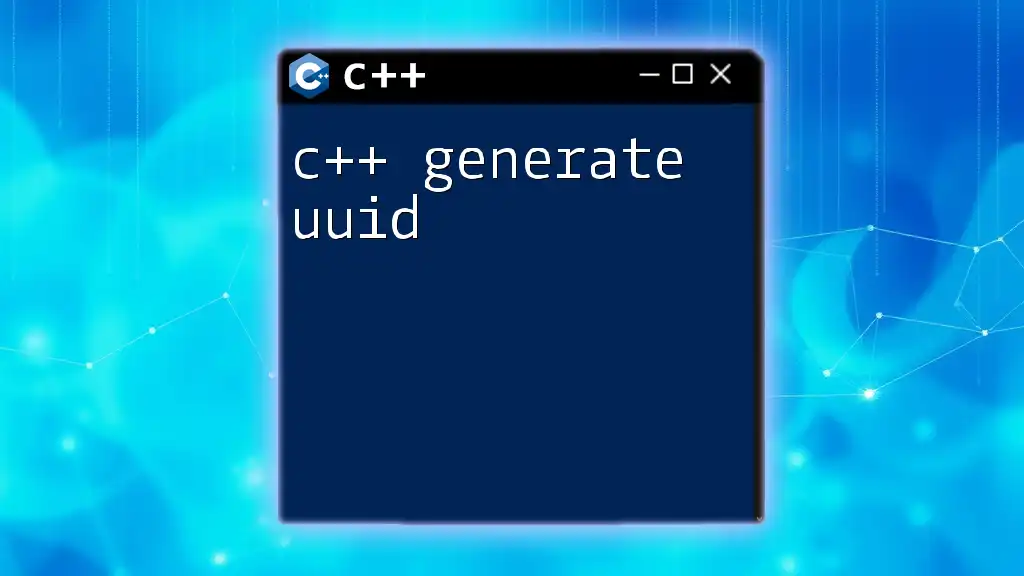
Implementing a Simple Generator in C++
Basic Structure of a Generator Function
To implement a generator, define a function using the coroutine syntax. The generator itself can be treated as an iterable object.
Code Snippet: Simple Counter Generator
Here’s a simple implementation of a generator that counts up to a specified number:
#include <iostream>
#include <coroutine>
struct Generator {
struct promise_type {
int current_value;
auto get_return_object() { return Generator{this}; }
auto initial_suspend() { return std::suspend_always{}; }
auto final_suspend() noexcept { return std::suspend_always{}; }
std::suspend_always yield_value(int value) {
current_value = value;
return {};
}
void return_void() {}
};
promise_type* promise;
Generator(promise_type* p) : promise(p) {}
int get_next() {
promise->current_value = 0; // Reset for next use
return promise->current_value;
}
};
Generator count_up_to(int n) {
for (int i = 1; i <= n; ++i) {
co_yield i;
}
}
int main() {
auto gen = count_up_to(5);
for (int i = 1; i <= 5; ++i) {
std::cout << gen.get_next() << std::endl;
}
return 0;
}
In this example, the generator `count_up_to` yields numbers from 1 to `n`. The `promise_type` manages the state of the generator, allowing it to hold the current value and control suspensions.
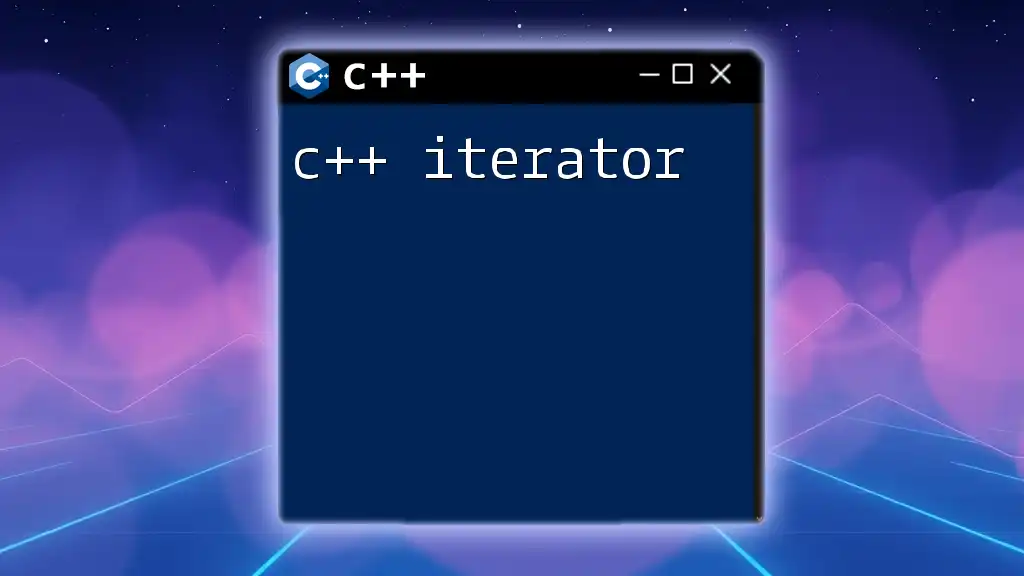
Advanced Generator Techniques
Generator State Management
Maintaining the state between yields is crucial. This ensures that the generator recalls its previous values and can continue from the last yielded state without starting over.
Handling Multiple Yields
Generators can yield different values at various points in time. You can design more complex generators that yield multiple times based on various conditions.
Code Example Demonstrating Multi-Yield Generators
Here’s how to create a generator that produces a range of values:
Generator range(int start, int end) {
for (int i = start; i <= end; ++i) {
co_yield i;
}
}
In this snippet, the `range` generator yields all integers between `start` and `end`, making it a versatile tool in data handling scenarios.
Generating Infinite Sequences
C++ generators can also produce infinite sequences, which can be very powerful in specific applications.
Code Example for Infinite Generator
Generator infinite_numbers() {
int i = 0;
while (true) {
co_yield i++;
}
}
This generator will endlessly yield incrementing integers. Usages include scenarios where a continual data stream is necessary, such as generating unique identifiers for records.
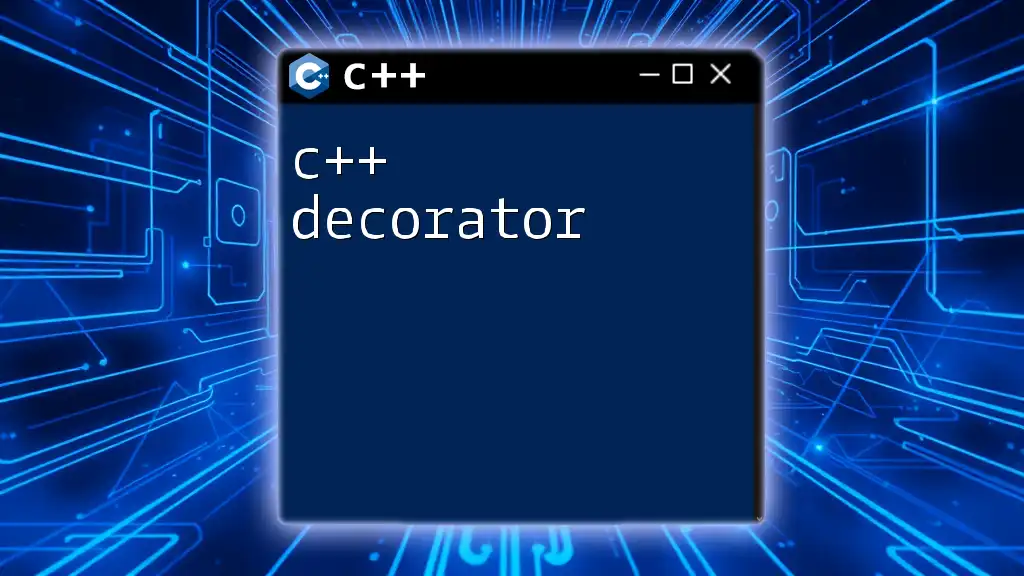
Practical Applications of C++ Generators
Generators in Data Streaming
Generators are incredibly useful in scenarios where data needs to be processed in a streaming fashion. Instead of loading the entire dataset into memory, you can utilize a generator to fetch and process values on-the-fly, leading to optimized memory usage and improved performance.
Performance Optimization with Generators
One of the primary benefits of using generators is their ability to help manage memory efficiently. By yielding values instead of storing extensive collections, your program can run faster and consume less memory.
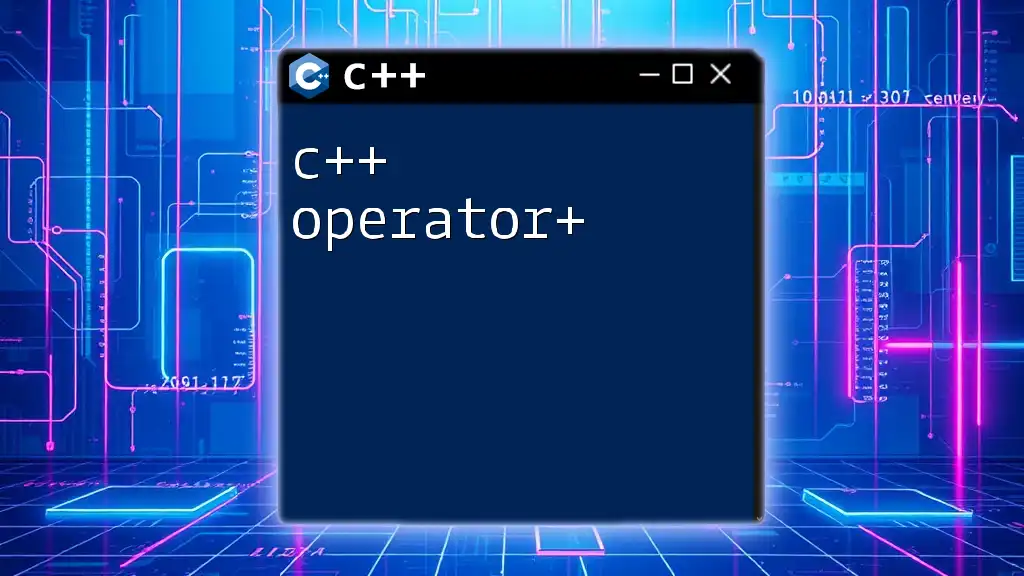
Best Practices for Using Generators
Error Handling with Generators
It’s vital to incorporate proper error handling into your generators. When a generator encounters an issue, it should manage the error gracefully rather than terminating abruptly.
When to Use Generators vs. Other Patterns
Deciding when to utilize a generator involves considering the context of your application. Generators are ideal where state maintenance across multiple calls is necessary, or when constructing potentially large data sets that would be impractical to hold all at once.
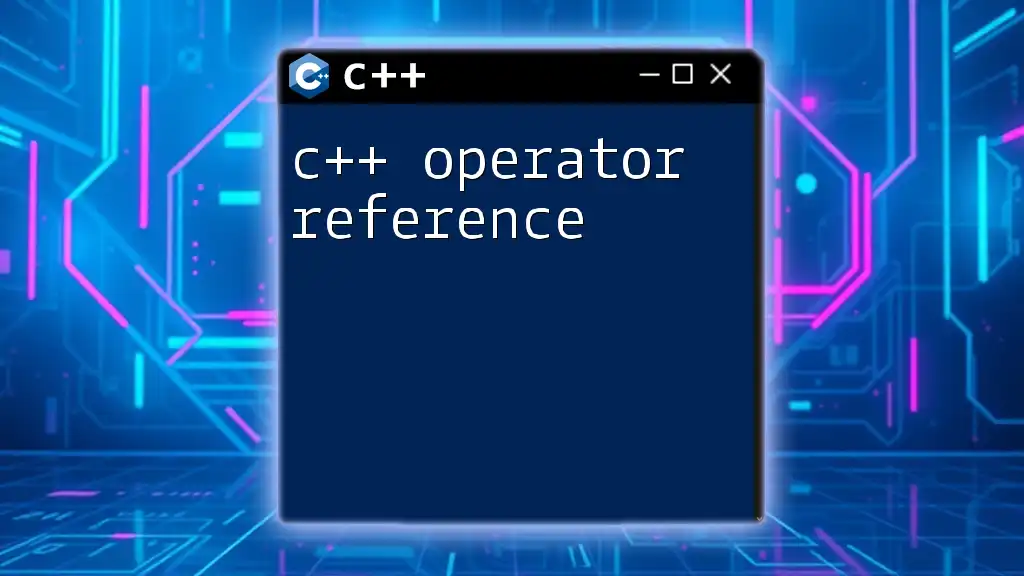
Common Misconceptions about C++ Generators
Myths vs. Facts
There are several common misunderstandings about C++ generators, such as the belief that they are inherently complex or only suitable for advanced programmers. In reality, they are powerful tools that can simplify many programming tasks, and their learning curve is manageable with practice.
Comparison with Iterators
While both generators and iterators navigate through collections of data, generators produce data on-the-fly and can maintain execution state without requiring the entire dataset to be present in memory. This makes generators a more flexible option for many applications.
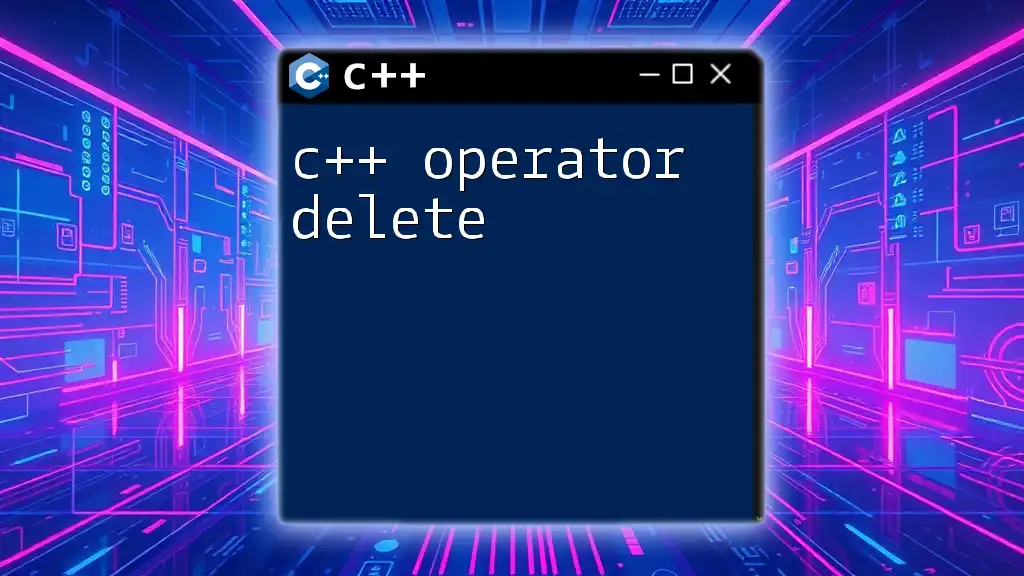
Conclusion
C++ generators are a powerful and efficient way to manage sequences of data and enhance the performance of C++ applications. With the introduction of coroutines in C++20, implementing generators has become more intuitive, allowing developers to create more readable and maintainable code. By exploring generators, you can harness their potential for data streaming, processing, and even asynchronous programming.
As you venture into utilizing C++ generators in your projects, consider implementing them in scenarios where managing state dynamically is paramount. The future of C++ programming is evolving with coroutines, and incorporating generators will position you as a forward-thinking developer.