A C++ editor is a software application that provides a platform for writing, editing, and compiling C++ code efficiently, often featuring syntax highlighting and debugging tools.
Here's an example of the basic syntax of a C++ program that outputs "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Editors
What is a C++ Editor?
A C++ editor is a specialized software tool designed to assist programmers in writing, editing, and managing C++ code more efficiently. These editors facilitate various aspects of coding, such as enhancing readability, reducing errors, and simplifying the debugging process. The use of an effective C++ editor can significantly improve productivity and code quality, making it an essential tool for both beginners and experienced developers.
The Difference Between an IDE and a Code Editor
While a C++ editor can sometimes refer to any tool that allows for editing C++ code, Integrated Development Environments (IDEs) serve a broad and enhanced function. An IDE typically includes not just the editor but also a compiler, debugger, and other useful tools encapsulated in a single application.
In contrast, a code editor may simply be a lightweight text editor that provides basic syntax highlighting and code editing features without the multiple integrated functionalities of an IDE.
Key features of a C++ IDE might include:
- Project management: Organizing files and resources easily.
- Debugging tools: Streamlining the process of identifying code errors.
- Build automation: Simplifying compilation and execution of code.
Comparison of IDEs vs. Text-Based Code Editors
While text-based code editors like Notepad++ are useful for quick edits, IDEs like Visual Studio or CLion offer powerful built-in features that enhance the coding experience. IDEs often include features such as code completion and sophisticated debugging tools, which may not be present in basic text editors.
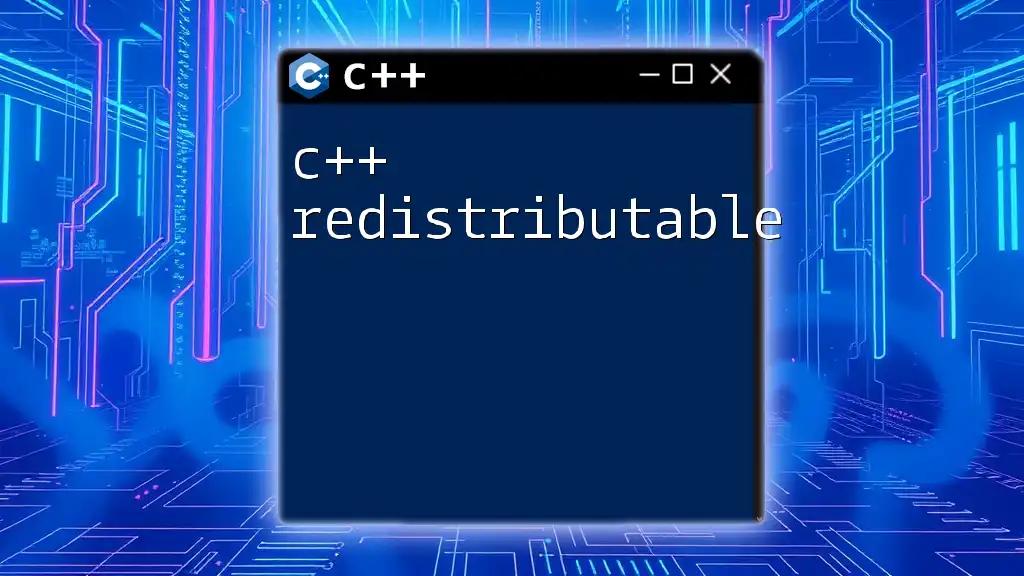
Key Features to Look for in a C++ Editor
Syntax Highlighting
Syntax highlighting is a crucial feature that differentiates code elements visually, making it easier to read and understand the code structure. Highlighting keywords, variables, and comments helps programmers quickly identify issues within their code.
For example, in C++, keywords like `int`, `return`, and `if` would be highlighted in bold, making it clear where these elements reside in the code.
Code Completion and Intellisense
Code completion and Intellisense features predict what a programmer is likely to type next, offering suggestions that can improve coding speed and accuracy. This is beneficial in avoiding typographical errors and enhances productivity by allowing the programmer to focus on logic instead of syntax.
Here's how you may use code completion in a C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // Start typing std::count and see suggestions
return 0;
}
Debugging Tools
An effective C++ editor or IDE will often include integrated debugging tools that allow users to step through code line by line, examine variable values, and monitor the execution flow. This feature is crucial for troubleshooting errors in your programs.
For example, using breakpoints, developers can halt program execution at specific lines to investigate the state of the application:
int main() {
int x = 10; // Set a breakpoint here
int y = 0;
std::cout << "Enter a number: ";
std::cin >> y; // Debugging can show values of x and y
return 0;
}
Integrated Terminal
Having an integrated terminal within your C++ editor simplifies the process of compiling and running your C++ code without switching to a separate command line interface. This seamless approach minimizes distractions and fosters a more fluid development experience.
For example, after writing a simple C++ program, a typical workflow would be:
g++ -o hello hello.cpp # Compile the program
./hello # Execute the compiled program
Customization Options
Every programmer works differently, and a good C++ editor allows for a high degree of customization. Whether it’s changing themes to reduce eye strain, adjusting font sizes for better readability, or defining custom shortcuts, personalizing your development environment can lead to enhanced productivity.
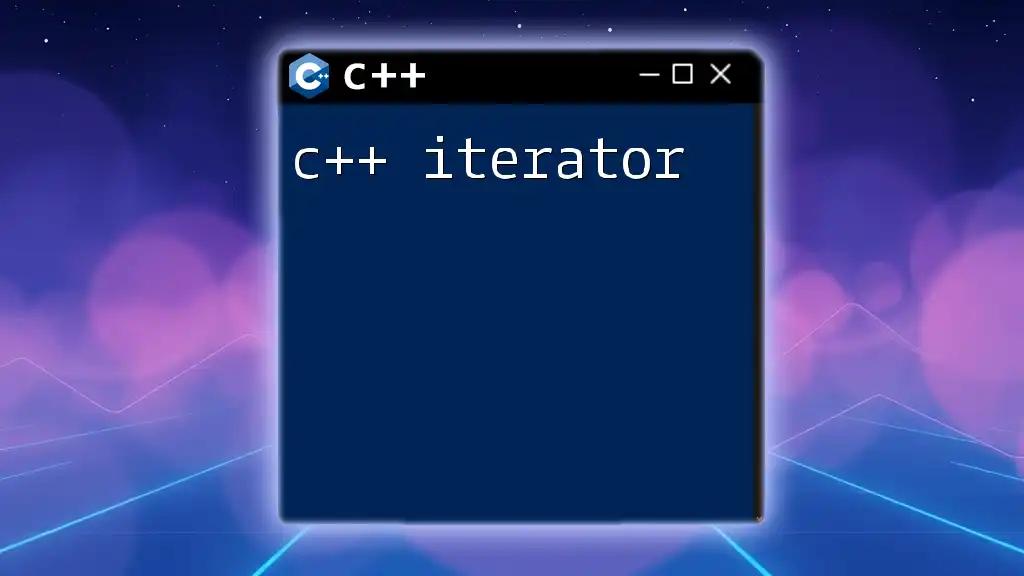
Popular C++ Editors and IDEs
Visual Studio
Visual Studio is a robust IDE that offers a comprehensive suite of tools tailored for C++ developers. With features like IntelliSense, integrated debugging, and a user-friendly interface, it is perfect for both novice and advanced programmers.
Setting up a simple project in Visual Studio includes choosing the C++ project template and following the guided steps to write your first program.
Code::Blocks
Code::Blocks is an open-source C++ IDE known for being lightweight yet versatile. It supports multiple compilers and offers various plugins to extend its capabilities.
To set up Code::Blocks:
- Download the IDE and install it.
- Create a new project and set up your development environment.
- Start coding, and enjoy features like syntax highlighting and debugging.
Example of starting your first program:
#include <iostream>
int main() {
std::cout << "Hello from Code::Blocks!" << std::endl;
return 0;
}
CLion
Developed by JetBrains, CLion is a cross-platform IDE that excels at automatically managing CMake projects, which is ideal for C++ development. Its code analysis tools help identify potential bugs even while you write code.
Eclipse with CDT (C/C++ Development Tooling)
Eclipse is a popular open-source IDE that can be configured with the C/C++ Development Tooling (CDT). This allows developers to use familiar Eclipse features while working with C++. You can create a C++ project similar to how you would with Java, making it a versatile editor for polyglot developers.
Qt Creator
Qt Creator stands out as an IDE designed specifically for developing applications using the Qt library. It offers robust tools for C++ development with an emphasis on GUI application design.
Using Qt Creator, you can easily compile and run your applications while taking advantage of its user-friendly debugging tools and integrated documentation.
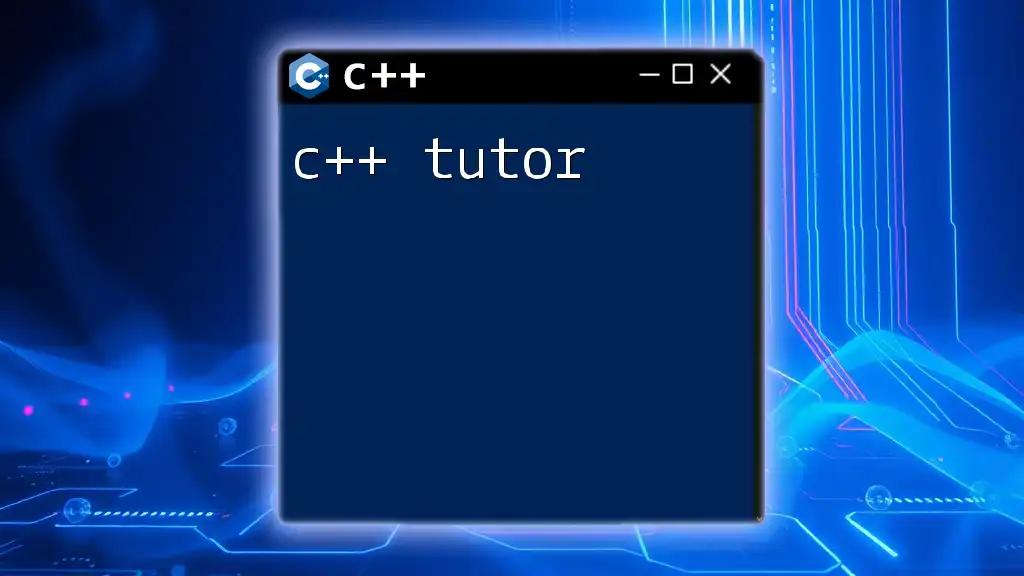
Setting Up Your C++ Editor
Installation Guides
To install your editor of choice, visiting the official website and following the related setup instructions is the best path. Most editors provide step-by-step guides that cover installation details for various operating systems.
Basic Configuration
Once installed, configuring the editor for C++ development involves setting compiler paths, adjusting editor preferences for tab and newline settings, and possibly adjusting the theme for a better visual experience. These adjustments can greatly enhance your overall coding experience by making tasks smoother and more intuitive.
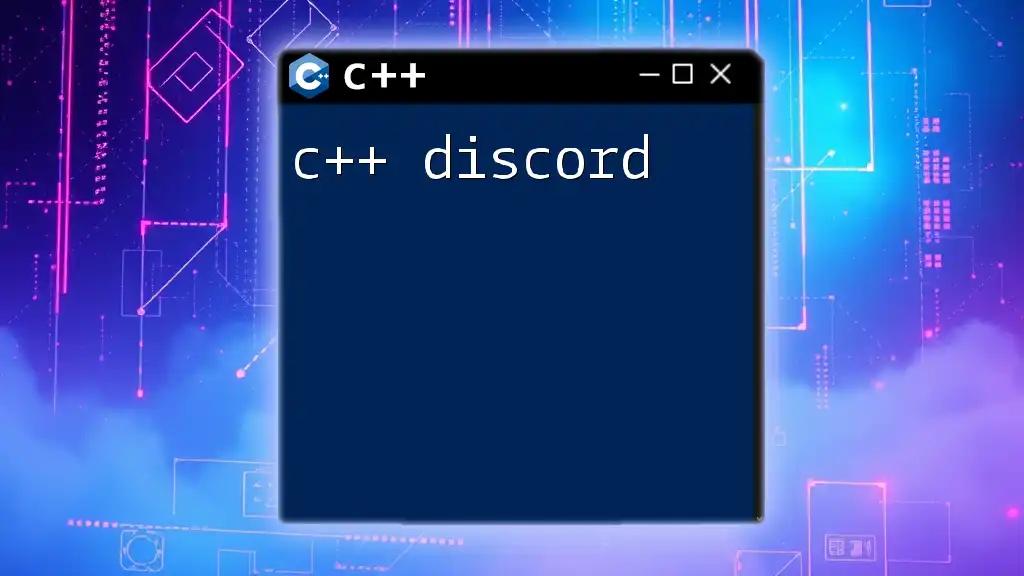
Best Practices for Using C++ Editors
Keyboard Shortcuts
Mastering keyboard shortcuts can lead to significant improvements in productivity. Many editors provide a list of common shortcuts which can speed up navigation, file management, and debugging tasks. Familiarize yourself with shortcuts specific to the editor you choose.
Organizing Your Workspace
A well-organized workspace improves efficiency. Creating a logical file structure for your projects can ease the burden of managing multiple files and related assets. Following conventions for naming files and directories can save time in the long run.
Version Control Integration
Integrating a version control system like Git into your C++ editor adds an essential layer of code management. It allows you to track changes, collaborate with others, and roll back unwanted modifications.
Most IDEs have built-in support or plugins that facilitate easy connection to your version control repositories, streamlining your development process.
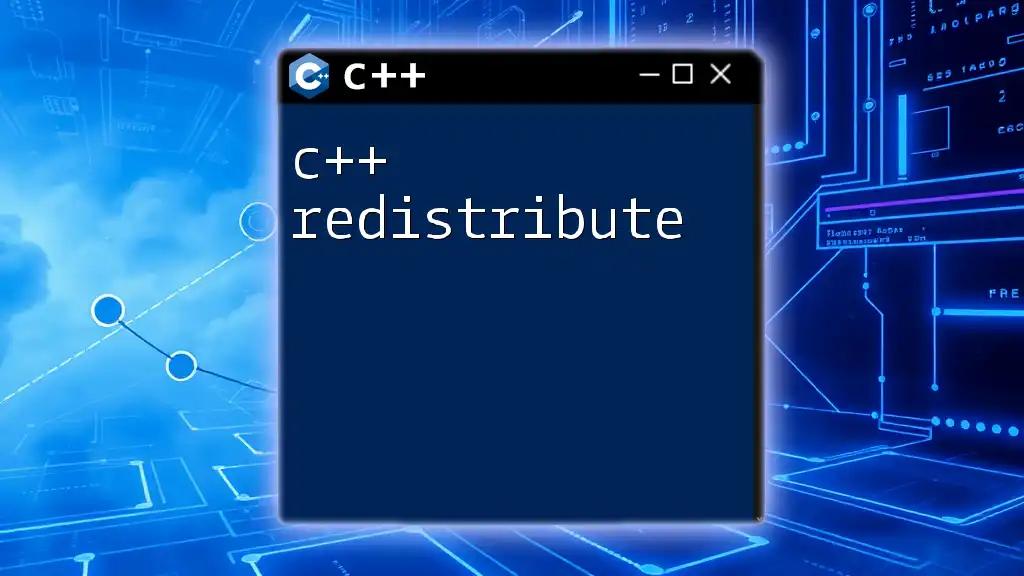
Troubleshooting Common Issues
Compile Errors
Compile errors are common when writing C++ code, especially for beginners. Focusing on the error messages provided by the IDE can help identify issues quickly. An example could be a common syntax error when you forget a semicolon:
#include <iostream>
int main() {
std::cout << "Missing semicolon" // Error: expected ';'
return 0;
}
Resolving these issues often involves reviewing the code line by line or checking against C++ syntax rules.
Configuration Problems
Common configuration problems, such as incorrect paths to your compiler or missing libraries, can disrupt your workflow. Conducting a thorough setup review and referencing IDE documentation can assist in resolving these roadblocks.
For example, ensuring that the paths to necessary compiler tools are correctly set in your IDE's settings can alleviate configuration problems.
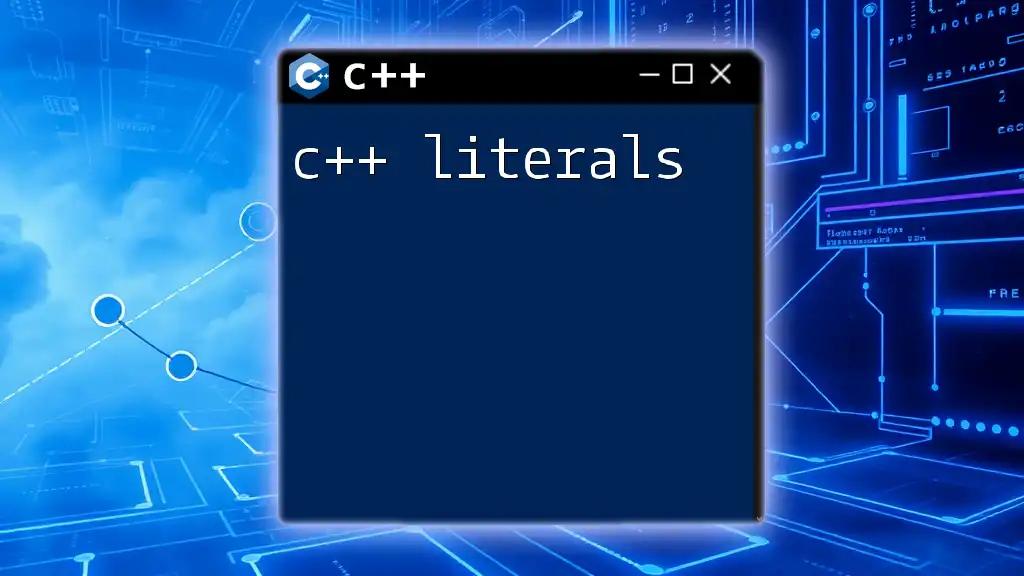
Conclusion
Choosing the Right C++ Editor for You
When choosing a C++ editor, it’s essential to consider your specific needs and workflow preferences. Whether you opt for a powerful IDE like Visual Studio or a lightweight solution like Code::Blocks, ensuring it includes features that boost productivity is key.
Final Thoughts
Ultimately, the goal is continual practice and improvement in your coding journey. An appropriate C++ editor can serve as an invaluable ally, guiding you through the complexities of C++ development while enhancing your coding experience.