C++ literals are fixed values that represent data of various types such as integers, floating-point numbers, characters, and strings, and can be directly used in your code.
Here's a code snippet demonstrating different types of literals in C++:
#include <iostream>
int main() {
// Integer literal
int intLiteral = 42;
// Floating-point literal
double floatLiteral = 3.14;
// Character literal
char charLiteral = 'A';
// String literal
const char* stringLiteral = "Hello, World!";
std::cout << intLiteral << ", " << floatLiteral << ", " << charLiteral << ", " << stringLiteral << std::endl;
return 0;
}
Understanding C++ Literals
What is a Literal?
A literal in programming refers to a fixed value that is written directly in the code. They serve as the fundamental building blocks of data in C++. In the context of C++, literals are a way to express constant values that can be used throughout your programs, such as numbers or characters. Understanding literals is crucial because they help communicate intent and meaning clearly within the code, enhancing readability and maintainability.
Types of Literals in C++
C++ offers a diverse array of literals that fall into several categories, including character literals, string literals, integer literals, floating-point literals, boolean literals, pointer literals, and user-defined literals. Each type serves different purposes and adheres to specific syntax rules, which are essential for the programmer to grasp.
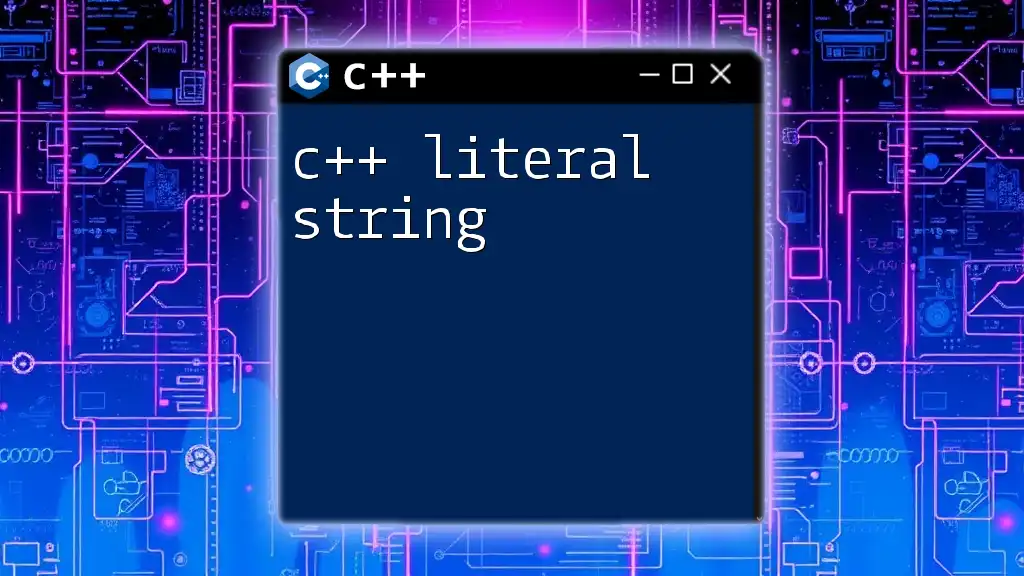
Character Literals
Introduction to Character Literals
Character literals represent single characters in C++. They are enclosed in single quotes, making it easy to distinguish them from other data types. Each character literal corresponds to a specific integer value defined by the ASCII character-set standards, and can include escape sequences, which are crucial for representing special characters.
Example of Character Literals
char letter = 'A';
char newline = '\n'; // Escape sequence for new line
In the example above, `letter` is assigned the character 'A', while `newline` is an escape sequence that represents a new line. This highlights how character literals can be practical in controlling output format.
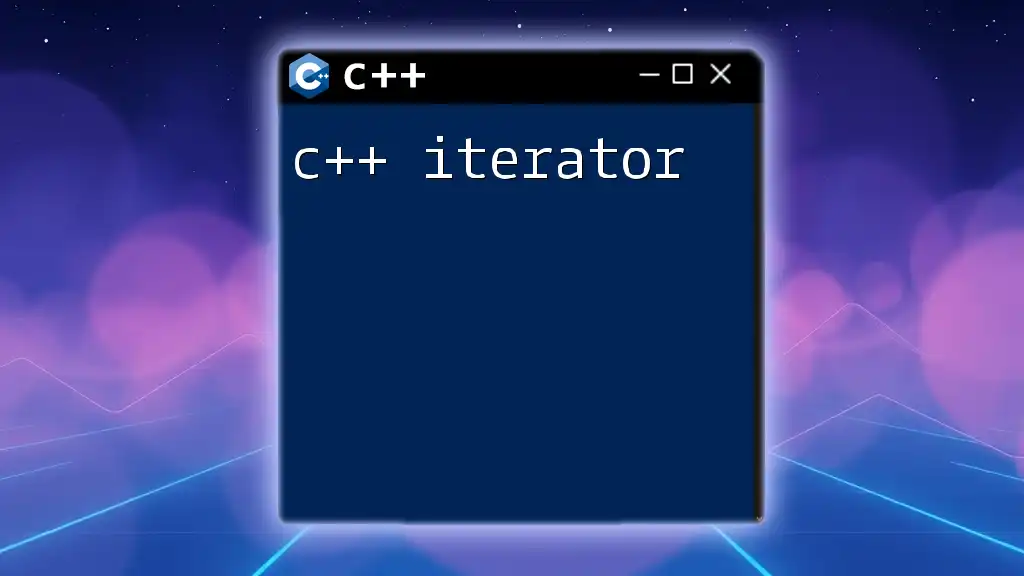
String Literals
Understanding String Literals
String literals are essentially sequences of characters enclosed in double quotes. They can represent text and can be manipulated or printed in various ways. String literals are crucial for user interaction and output in many applications.
Raw String Literals
Raw string literals allow developers to define strings without escaping special characters. Enclosed in `R"( ... )"`, they are particularly useful for paths or regular expressions where backslashes could lead to errors.
const char* rawString = R"(C:\Users\Name\Documents)";
In this case, `rawString` is defined as a path string, and the use of raw string literals avoids confusion with escape sequences, making it cleaner and easier to read.
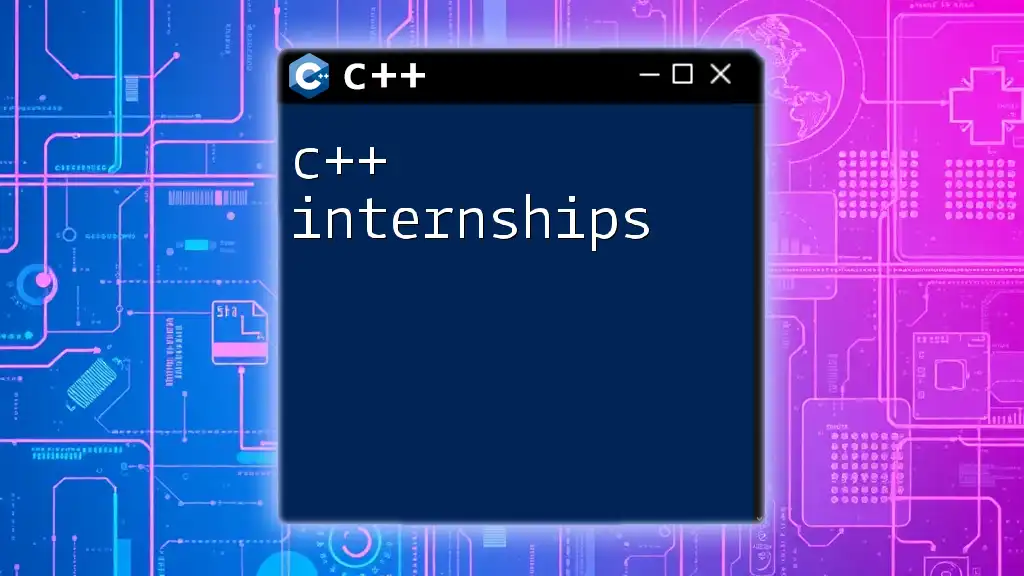
Integer Literals
Overview of Integer Literals
Integer literals in C++ can be expressed in several numeral systems: decimal (base 10), octal (base 8), hexadecimal (base 16), and binary (base 2). Each numeral type has its own specific syntax, allowing programmers to select the most suitable representation for their needs.
Examples of Integer Literals
int decimal = 42; // Decimal literal
int octal = 052; // Octal literal (equivalent to 42)
int hexadecimal = 0x2A; // Hexadecimal literal (equivalent to 42)
int binary = 0b101010; // Binary literal (C++14 and later)
In this section of code, `decimal`, `octal`, `hexadecimal`, and `binary` each demonstrate how integer literals can represent the same value in different forms, emphasizing C++’s flexibility with numeral systems.
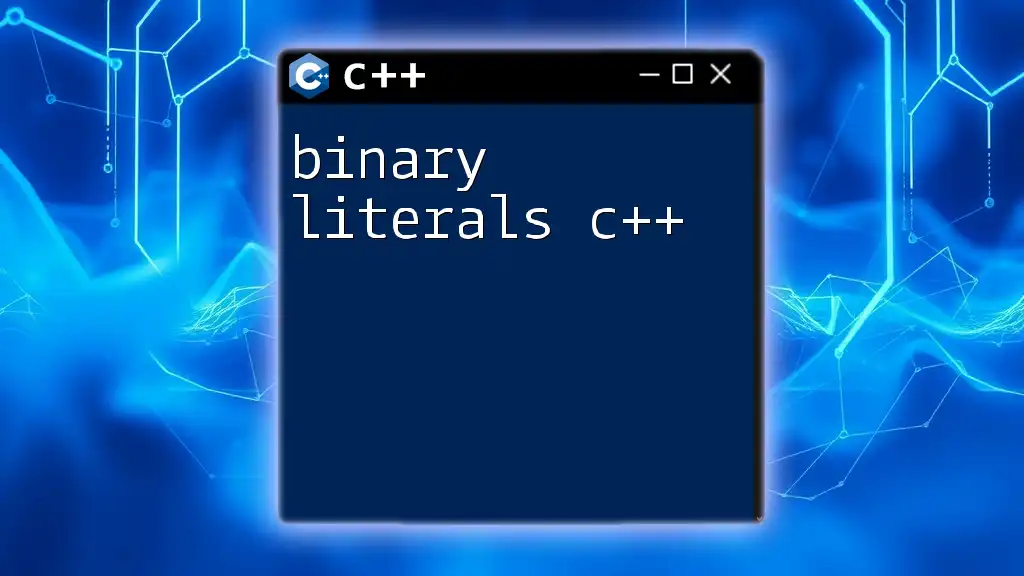
Floating-Point Literals
Understanding Floating-Point Literals
Floating-point literals represent non-integer numbers and are classified as either `float` or `double`. They can be expressed using decimal notation or in scientific notation, allowing for ease of representation in various scales.
Code Examples of Floating-Point Literals
float pi = 3.14f; // Float literal
double e = 2.71828; // Double literal
double scientific = 1.5e2; // Scientific notation
In this code snippet, `pi` is a float literal, while `e` is a standard double literal. The variable `scientific` illustrates how scientific notation can compactly represent large or small values.
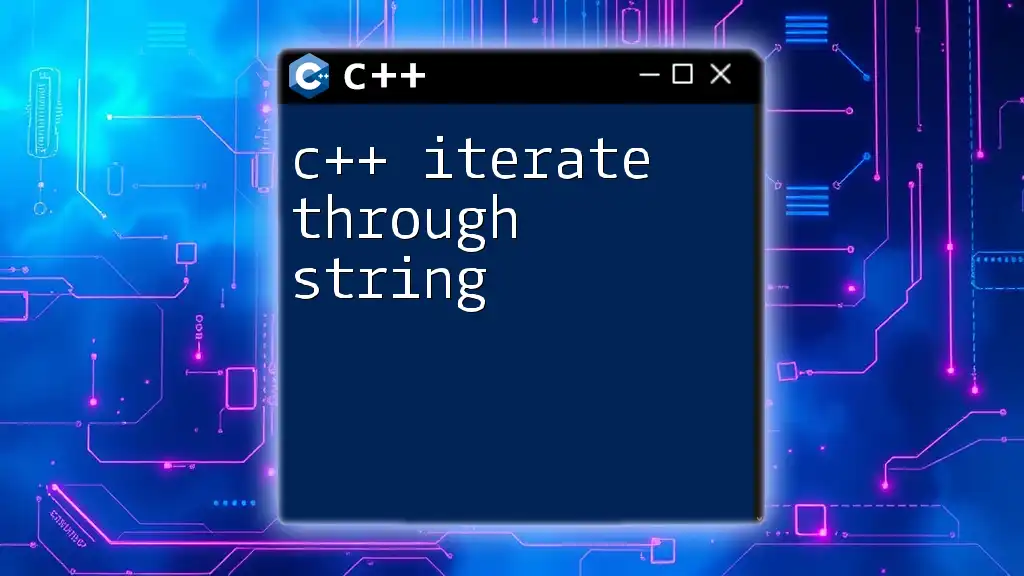
Boolean Literals
Introduction to Boolean Literals
Boolean literals consist of two distinct values: `true` and `false`. They are pivotal in control flow within programs, allowing developers to leverage logical conditions in decision-making processes.
Example of Boolean Literals
bool isActive = true;
bool isFinished = false;
In this code, `isActive` and `isFinished` are assigned boolean literals, illustrating how simple binary choices can dictate the flow of an application, such as in if-statements and loops.
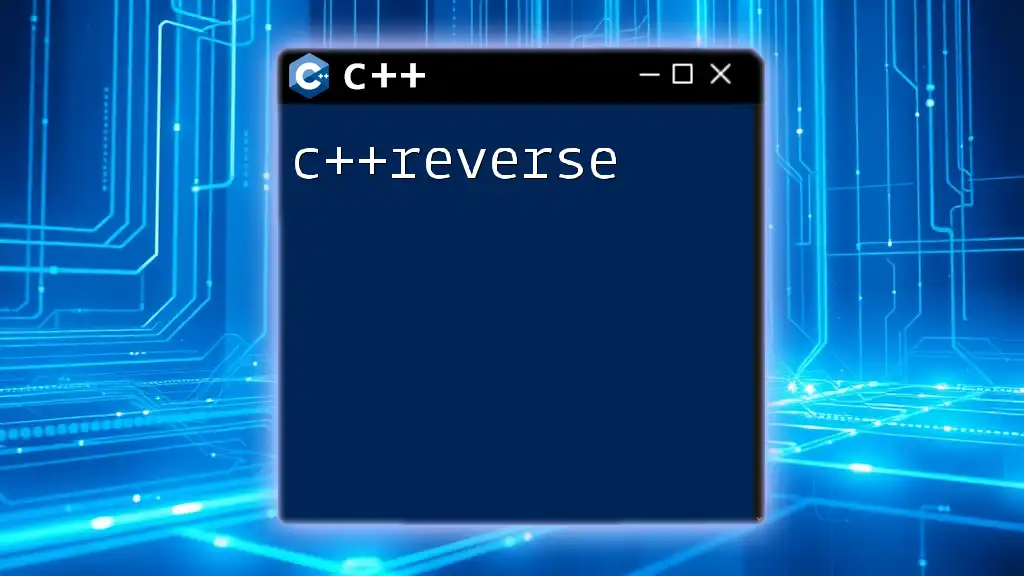
Pointer Literals
Understanding Pointer Literals
Pointer literals, especially the null pointer literal, play a fundamental role in memory management in C++. A null pointer represents the absence of an object or value.
Example of Pointer Literals
int* ptr = nullptr; // C++11 null pointer literal
In this case, `ptr` is initialized with `nullptr`, indicating that it doesn’t point to any valid memory location. Using null pointers helps prevent dereferencing invalid memory, thereby improving program stability.
![Understanding Literals in C++ [A Quick Guide]](/images/posts/l/literals-in-cpp.webp)
User-Defined Literals
What are User-Defined Literals?
C++ allows developers to create user-defined literals, empowering them to define custom types of literals based on their specific needs. This feature enhances code expressiveness and clarity.
Syntax for User-Defined Literals
User-defined literals follow the syntax that consists of a suffix attached to a base literal. The suffix can be a single alphabetic character or more, depending on the desired effect.
Example of User-Defined Literals
long double operator"" _km(long double distance) {
return distance * 1000; // Convert kilometers to meters
}
long double distance = 1.5_km; // Using the user-defined literal
In the example above, a custom user-defined literal `_km` converts kilometers to meters when the literal `1.5_km` is encountered, showing how user-defined literals can make related operations more intuitive.
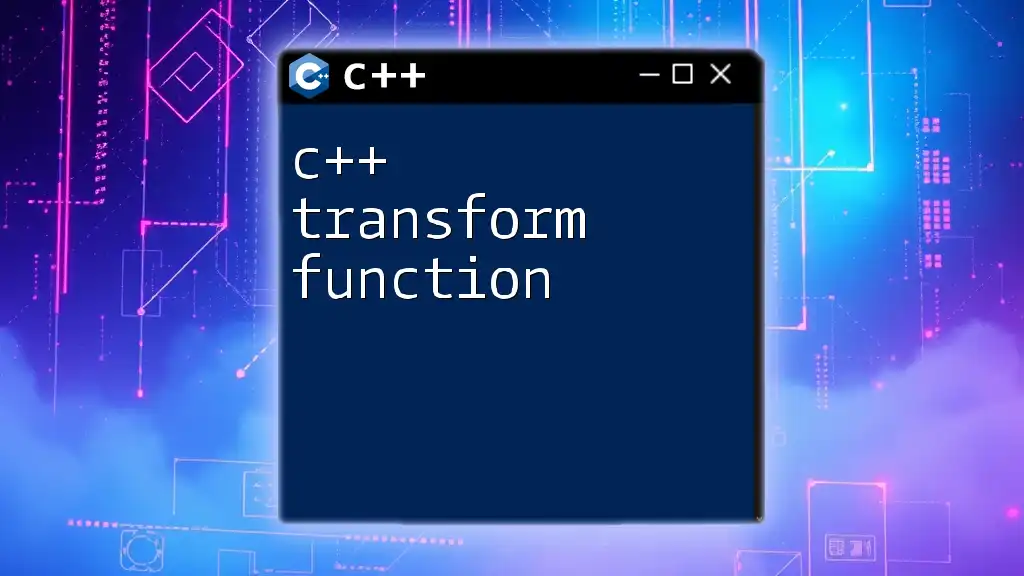
Summary and Key Takeaways
Recap of C++ Literals
C++ literals come in various forms, including character, string, integer, floating-point, boolean, pointer, and user-defined literals. Understanding these types is essential for effective programming, as they form the foundation upon which more complex code is built.
Importance of Mastering Literals
Mastering C++ literals not only simplifies the coding experience but also enhances the efficiency and clarity of your programs. By practicing the usage of these literals, developers can write better, more manageable code that clearly conveys its intent.
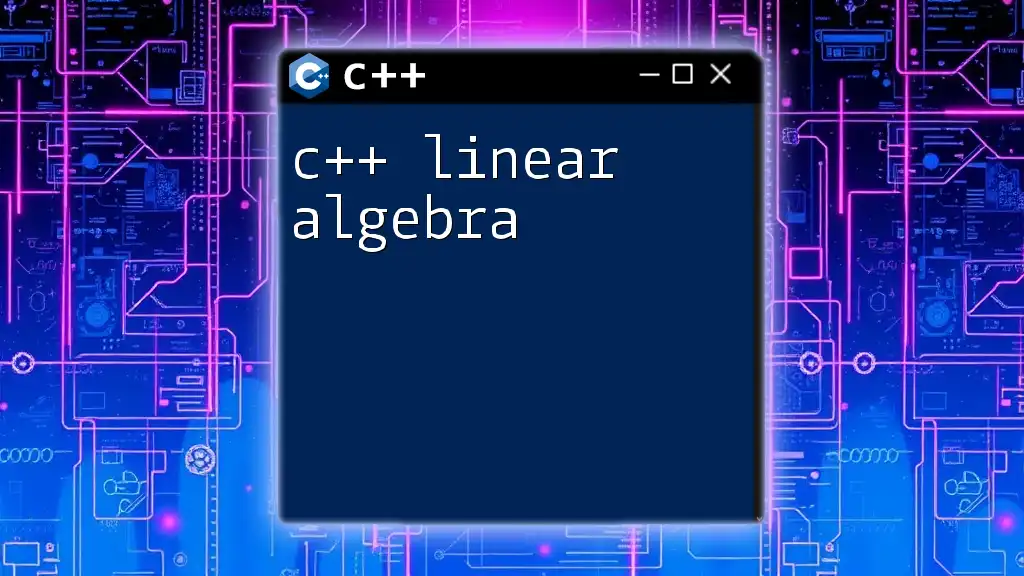
Additional Resources
References for Further Learning
Consider exploring books, online courses, or C++ reference documentation to deepen your understanding of literals and other programming concepts.
Community and Support
Joining C++ forums and online communities can provide additional support and resources, helping you stay updated on best practices and trends in C++ programming.
By absorbing this comprehensive information on C++ literals, readers can enhance their coding skills and foster a greater appreciation for the subtleties that C++ offers.