C++ libraries provide a collection of pre-written code that can be used to perform common programming tasks, enhancing productivity and efficiency in software development.
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 2, 8, 1, 4};
std::sort(numbers.begin(), numbers.end());
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Introduction to C++ Libraries
C++ libraries are a fundamental part of C++ programming, providing reusable code that simplifies the development process. They offer pre-written functions and classes that can be used to perform specific tasks, enabling developers to write efficient and effective code without having to reinvent the wheel. The use of libraries not only enhances productivity but also encourages best practices, allowing developers to focus on building unique features rather than common functionalities.
When categorizing C++ libraries, they can typically be divided into two broad types: static and dynamic libraries. Static libraries are linked into the executable at compile time, making the final program larger but more self-contained. Dynamic libraries, on the other hand, are linked at runtime and can be shared among multiple programs, which can help conserve memory and storage.
Additionally, we have standard libraries and third-party libraries. Standard libraries, like the Standard Template Library (STL), come built-in with the C++ environment, while third-party libraries are developed by individuals or organizations outside the standard libraries.
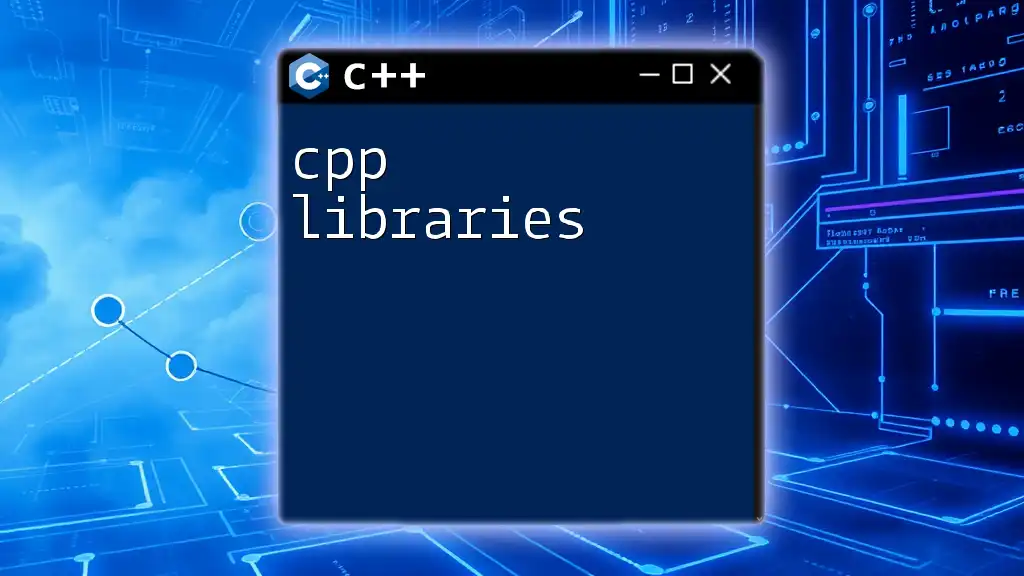
Understanding the Standard Template Library (STL)
Overview of STL
The Standard Template Library (STL) is a powerful set of C++ template classes and functions that provide data structures and algorithms. Its primary components are containers, algorithms, and iterators.
The importance of STL cannot be overstated, as it streamlines programming and allows for the reuse of complex data structures and algorithms without the need for detailed implementations. By leveraging STL, developers can write cleaner and more maintainable code.
Commonly Used STL Components
Containers
STL provides several types of containers, including:
- Vector: A dynamic array capable of resizing itself automatically.
- List: A doubly linked list allowing for efficient insertions and deletions.
- Map: A sorted associative container that stores key-value pairs.
Here’s an example of how to use a vector in C++:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4};
for(int num : vec) {
std::cout << num << " ";
}
return 0;
}
Algorithms
STL algorithms facilitate common operations such as sorting and searching. These algorithms work directly with STL containers and utilize iterators for traversing the data. For instance, using the `std::sort` function on a vector can be performed as follows:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {4, 1, 3, 2};
std::sort(vec.begin(), vec.end());
for(int num : vec) {
std::cout << num << " ";
}
return 0;
}
Iterators
Iterators are pointers that enable traversal through the elements of a container. They abstract the underlying data structures, letting developers use the same interface regardless of the container type.
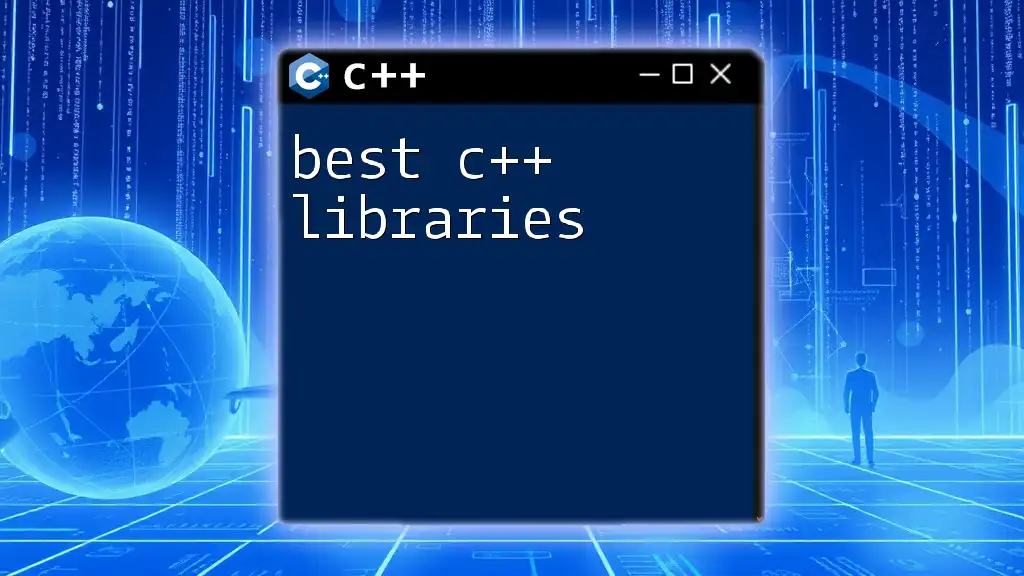
Popular C++ Libraries for Different Domains
General Purpose Libraries
Boost is among the most well-known C++ libraries, providing a wide range of functionalities. It includes smart pointers for automatic memory management and tools for asynchronous input/output operations.
An example of a Boost implementation can show how to use smart pointers:
#include <iostream>
#include <memory>
class MyClass {
public:
MyClass() { std::cout << "Constructor" << std::endl; }
~MyClass() { std::cout << "Destructor" << std::endl; }
};
int main() {
std::shared_ptr<MyClass> ptr = std::make_shared<MyClass>();
return 0;
}
Graphics and Game Development Libraries
SFML (Simple and Fast Multimedia Library) provides a simple interface for multimedia programming with support for 2D graphics, audio, and input management. Here’s how to create a basic SFML window:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "SFML window");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.display();
}
return 0;
}
Networking and Protocol Libraries
POCO (Portable C++ Libraries) is designed for building networked applications. It provides various features like handling HTTP requests and FTP connections, making it an excellent choice for server-side applications. An example of using POCO for creating an HTTP client might look like this:
#include <Poco/Net/HttpClientSession.h>
#include <Poco/Net/HttpRequest.h>
#include <Poco/Net/HttpResponse.h>
#include <Poco/Stream.h>
#include <iostream>
int main() {
Poco::Net::HttpClientSession session("www.example.com");
Poco::Net::HttpRequest request(Poco::Net::HttpRequest::HTTP_GET, "/");
session.sendRequest(request);
Poco::Net::HttpResponse response;
std::istream& rs = session.receiveResponse(response);
std::cout << response.getStatus() << " " << response.getReason() << std::endl;
return 0;
}
Numerical Libraries
Eigen is a popular C++ template library for linear algebra. It facilitates operations such as matrix and vector computations, essential for scientific computing. Here's an example demonstrating matrix multiplication:
#include <Eigen/Dense>
#include <iostream>
int main() {
Eigen::MatrixXd m(2,2);
m(0,0) = 3; m(0,1) = 2.5;
m(1,0) = -1; m(1,1) = m(0,0) + m(0,1);
std::cout << m << std::endl;
return 0;
}
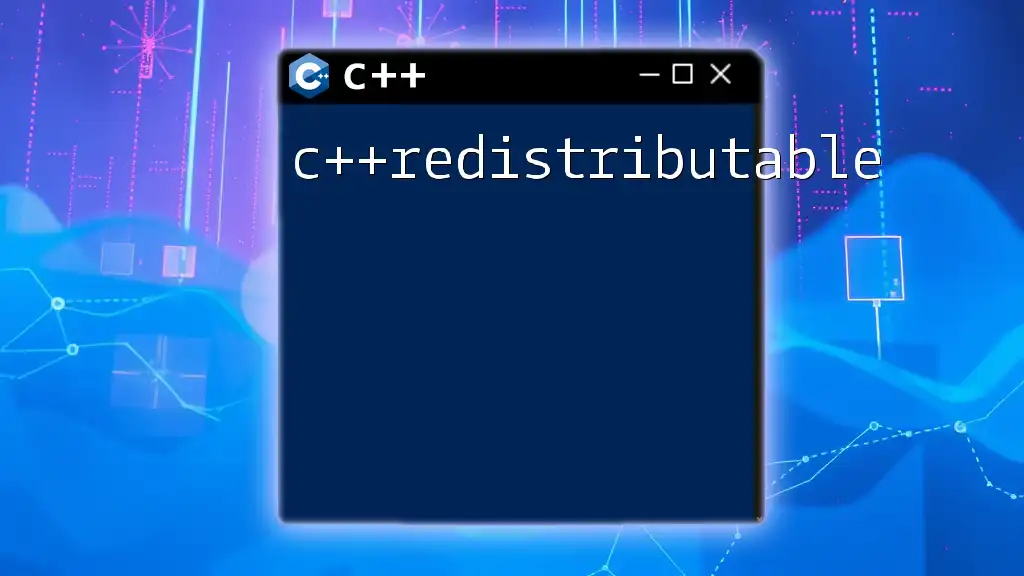
Specialized Libraries
Scientific Computing Libraries
Armadillo stands out for numerical linear algebra tasks. It simplifies tasks like matrix operations, making it easy to implement complex algorithms without deep mathematical intricacies. In practice, Armadillo allows rapid prototyping and development of scientific applications.
Image Processing Libraries
OpenCV (Open Source Computer Vision Library) excels in image processing and computer vision tasks. It includes functionalities for image manipulation, object detection, and even facial recognition. Loading and displaying an image in OpenCV can be achieved with the following code:
#include <opencv2/opencv.hpp>
int main() {
cv::Mat image = cv::imread("image.jpg", cv::IMREAD_COLOR);
if(image.empty()) {
std::cerr << "Could not open or find the image!" << std::endl;
return -1;
}
cv::imshow("Display Image", image);
cv::waitKey(0);
return 0;
}
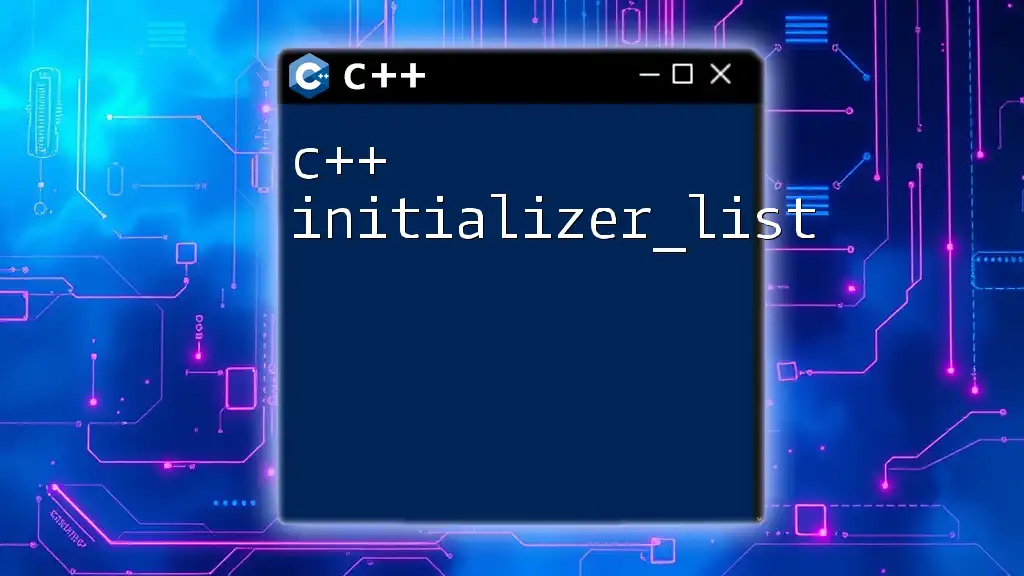
Advantages of Using C++ Libraries
Using C++ libraries presents numerous advantages. First and foremost, code reusability saves time and reduces the potential for bugs. By utilizing pre-existing libraries, developers can ensure that a large portion of the functionality they need is already tested and optimized.
Additionally, libraries can lead to significant time-saving benefits. Whether it’s implementing a complex algorithm or managing memory efficiently, leveraging libraries allows developers to concentrate on creating unique solutions instead of addressing common problems.
Lastly, community support and documentation are abundantly available, especially for popular libraries. This support network can be invaluable for beginners and experienced developers alike, providing resources to overcome challenges and learn best practices in C++ development.
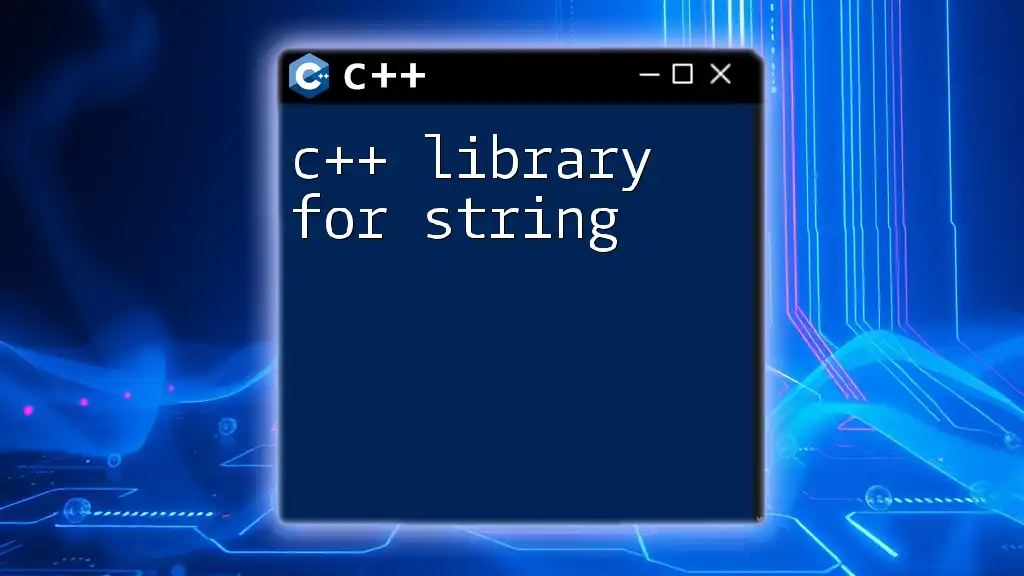
How to Choose the Right C++ Library for Your Project
Selecting the right C++ library for your project can drastically impact your development process. Here are some considerations:
-
Project Requirements: Always start by clearly defining what your project needs. Some libraries excel in specific domains, so aligning your choice with your goals is essential.
-
Performance Considerations: Some libraries prioritize performance, especially in critical applications. Investigate the efficiency of the libraries in question to ensure they meet your application's standards.
-
Community and Support: Libraries backed by active communities often provide better support and resources, making it easier to resolve issues and keep pace with updates.
-
Licensing Issues: Be aware of the licensing agreements for libraries, especially if you plan to distribute your software commercially. Some libraries come with restrictions that you need to comply with.
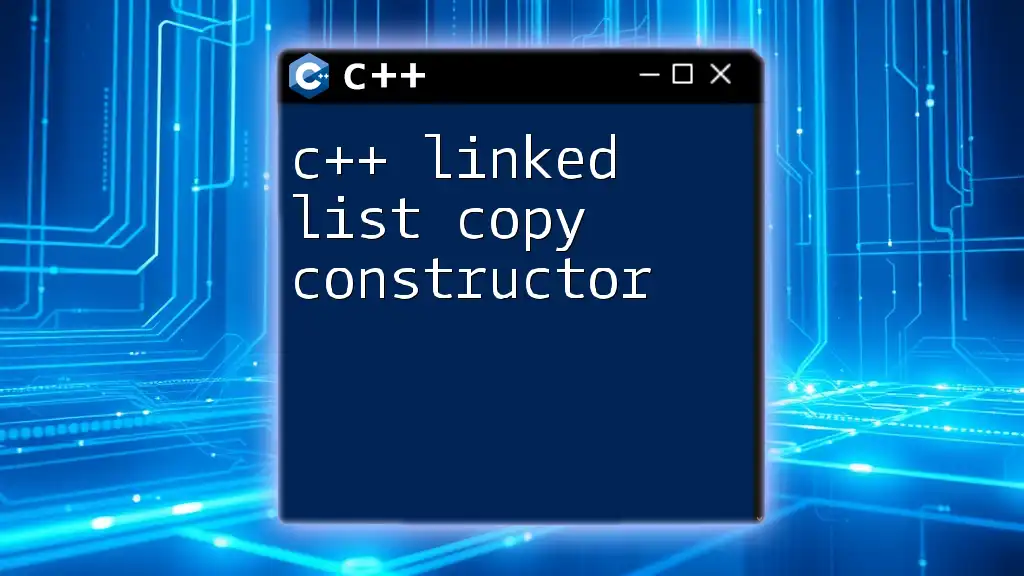
Conclusion
Understanding and utilizing a comprehensive C++ libraries list is crucial for enhancing your programming skills and streamlining your development projects. The rich variety of libraries available, including STL, Boost, SFML, POCO, Eigen, Armadillo, and OpenCV, provides robust solutions across various domains.
As you explore these libraries and integrate them into your projects, you'll find that they significantly improve your efficiency and code quality. Each library brings unique functionalities and optimizations to the table, ultimately enriching your programming experience. Embrace the possibilities that come with leveraging C++ libraries and elevate your development skills to new heights.
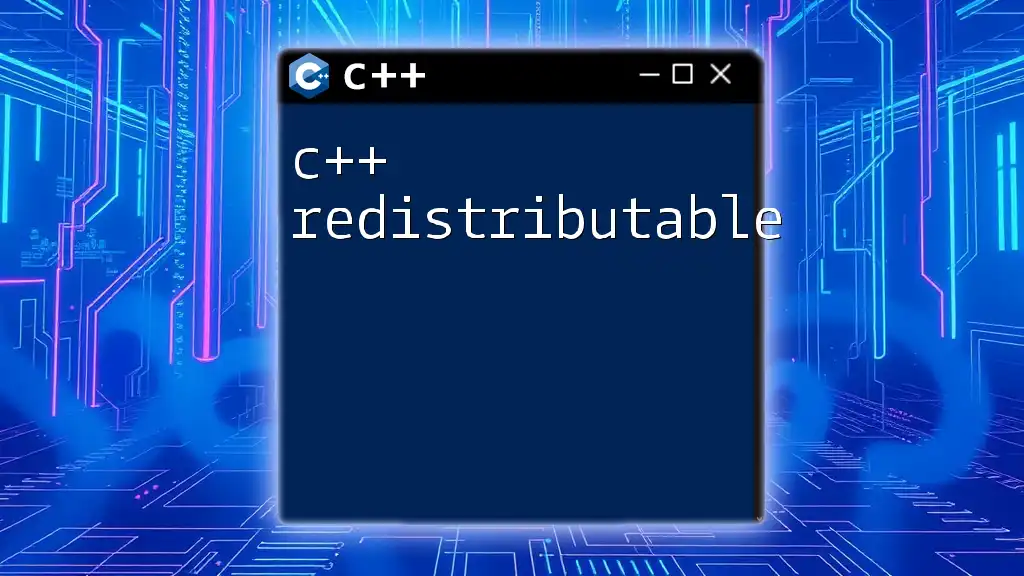
Further Resources
For those looking to dive deeper into the world of C++ libraries, numerous resources are available. Comprehensive documentation, tutorials, and community forums are key in your learning journey. Books and online courses can provide structured learning paths, while engaging with community forums can offer valuable insights and support as you navigate the complexities of C++.