In C++, you can declare a string by including the `<string>` header and using the `std::string` type, as shown in the following example:
#include <string>
std::string myString = "Hello, World!";
Understanding Strings in C++
What is a String?
In C++, a string is a sequence of characters used to store text. Unlike character arrays, which are fixed in size, `std::string` objects offer dynamic sizing and ease of manipulation. They manage memory automatically, reducing the risk of buffer overflows and making string operations more intuitive.
Why Use Strings?
Strings are integral to human-computer interaction, as they allow for user input, text processing, and storing textual data. They provide a versatile and safe way to handle text, making C++ development more efficient. Some advantages include:
- Flexibility: Strings can grow and shrink as needed.
- Safety: Automatic memory management helps to avoid common pitfalls associated with raw character arrays.
- Rich functionality: C++ strings come with numerous built-in methods to manipulate text easily.
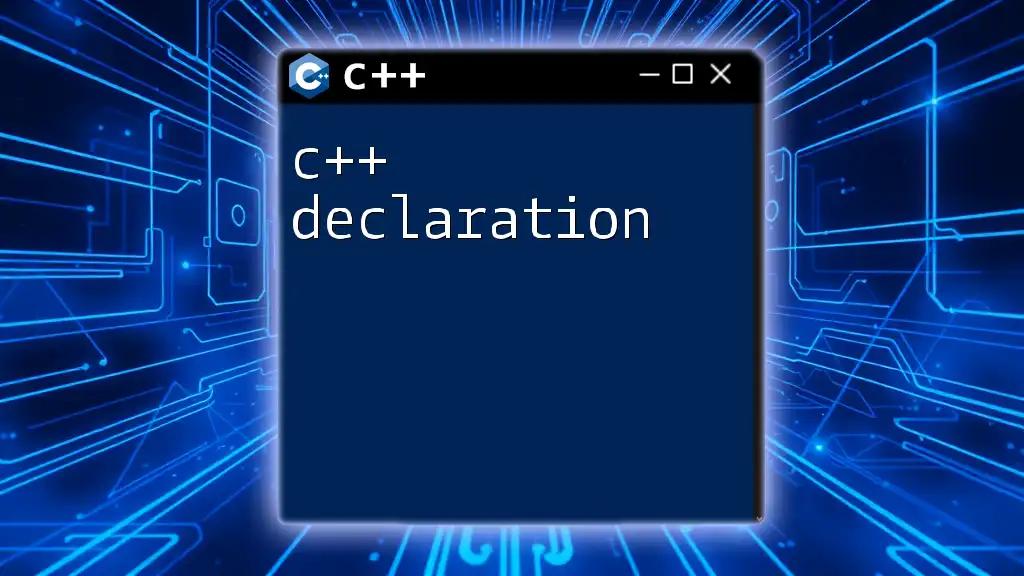
C++ String Declaration Basics
C++ Declare a String
Declaring a string in C++ is straightforward. The standard way to declare a string is by using the `std::string` type from the `<string>` header. This can be done without initialization or with an initialization value, depending on your needs.
Declaring Strings in Different Ways
Standard String Declaration
To declare a string without initializing it, you can simply do:
#include <iostream>
#include <string>
int main() {
std::string myString; // Declaration
myString = "Hello, World!"; // Assignment
std::cout << myString << std::endl; // Output
return 0;
}
Declare a String C++ with Initialization
If you want to declare and initialize a string in one step, it is as simple as:
std::string myString = "Sample Text"; // Declaration and initialization
Multiple String Declarations
You can also declare multiple strings in a single statement, which helps you manage related text variables smoothly:
std::string str1 = "First", str2 = "Second"; // Multiple declarations

Advanced String Declaration Techniques
Declaring a String Variable C++
When declaring a string variable, it's essential to choose meaningful names to improve code clarity. For instance:
std::string userInput; // Declaring string variable for user input
Constant Strings
You may sometimes want to declare a string that should not change throughout the program. To do so, use the `const` keyword. This ensures that the string's value remains constant:
const std::string greeting = "Welcome!"; // Constant string declaration
Declaring Strings as Function Parameters
Strings can be passed to functions, allowing you to manipulate text more efficiently. Here’s an example of how to pass strings to a function:
#include <iostream>
#include <string>
void printString(const std::string &str) {
std::cout << str << std::endl; // Printing a string
}
int main() {
std::string message = "Hello, Functions!";
printString(message); // Calling the function with a string
return 0;
}
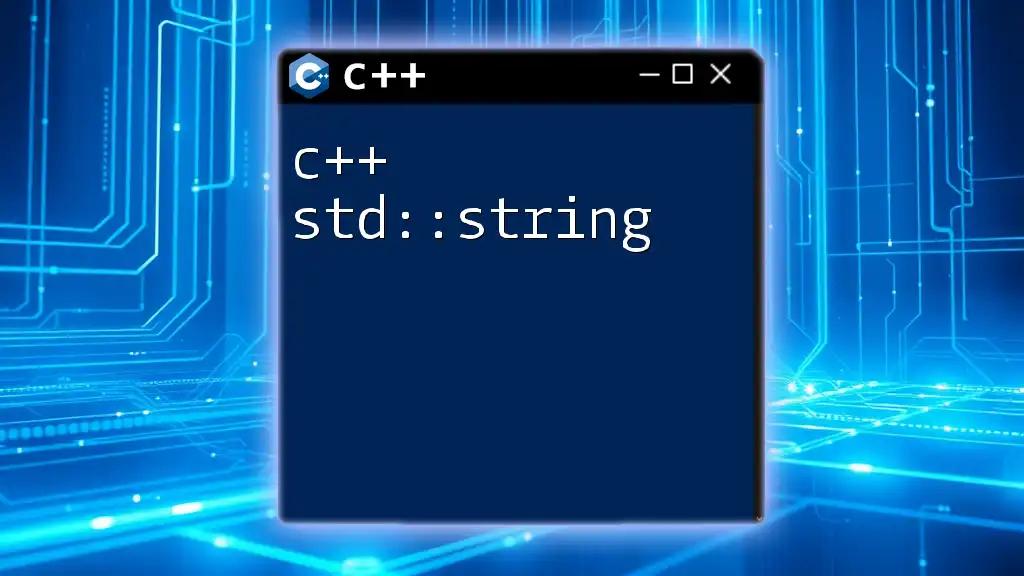
Defining a String in C++
Using String Literals
String literals are fixed sequences of characters that can be assigned to string variables. When working with string literals, you need to ensure they are correctly referenced:
const char* str = "Literal String"; // String literal declaration
Dynamic String Declaration
C++ allows for dynamic memory allocation for strings. If you need a string whose size isn't known at compile time, you can use the `new` keyword. However, be mindful of memory management:
std::string* dynamicStr = new std::string("Dynamic String");
// Don't forget to release allocated memory
delete dynamicStr;

Working with Declared Strings
Modifying Strings
Once you have declared a string, you can modify it using several built-in methods. For instance, the `append` method allows you to add text to the end of a string:
std::string myString = "Hello";
myString.append(", welcome to C++!"); // Modifying the string
Common String Operations
Concatenation
Joining strings can be done easily with the `+` operator:
std::string fullStr = myString + " Let's learn!";
Length and Size
To get the length of a string, you can use the `length()` method, which returns the number of characters in the string:
size_t length = myString.length(); // Getting the length of the string
Input and Output with Strings
User interactions often involve string inputs. You can use `std::cin` to accept user input effectively:
std::string input;
std::cout << "Enter a string: ";
std::cin >> input; // Capturing user input
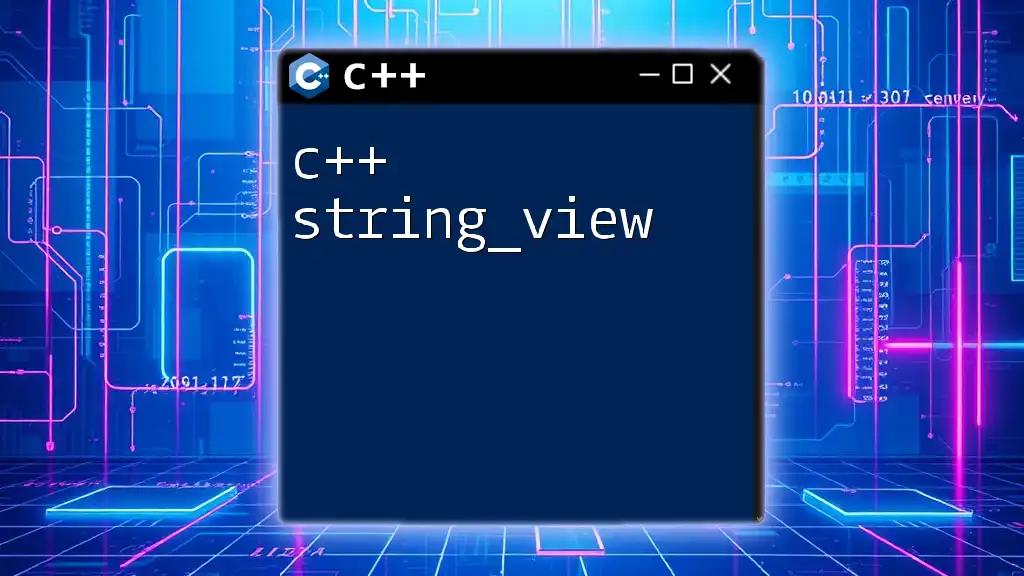
Best Practices for Declaring Strings in C++
Avoiding Memory Leaks
Effective memory management is crucial in C++. Always ensure to release any dynamically allocated strings with `delete` to avoid memory leaks. Using standard library strings (`std::string`) helps mitigate many memory-related issues due to automatic management.
Using the Right String Type
C++ provides various string types, including `std::string` for narrow characters and `std::wstring` for wide characters used in multilingual applications. Understanding when to use each type can significantly impact your program's functionality and performance. Generally, prefer `std::string` unless you have specific needs for wide character handling.
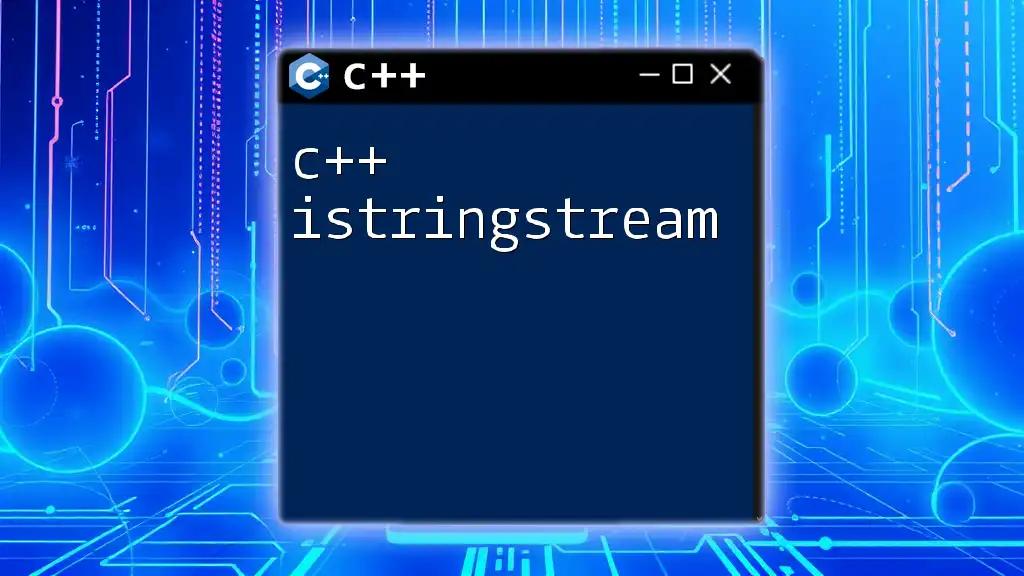
Conclusion
Understanding how to declare a string in C++ is foundational for effective text manipulation and user interaction. From basic declarations to advanced usage, C++ offers diverse capabilities for string handling. By practicing these techniques and adhering to best practices, you can write more robust and efficient C++ programs. Now, it's time to dive deeper and explore additional string concepts to elevate your programming skills!
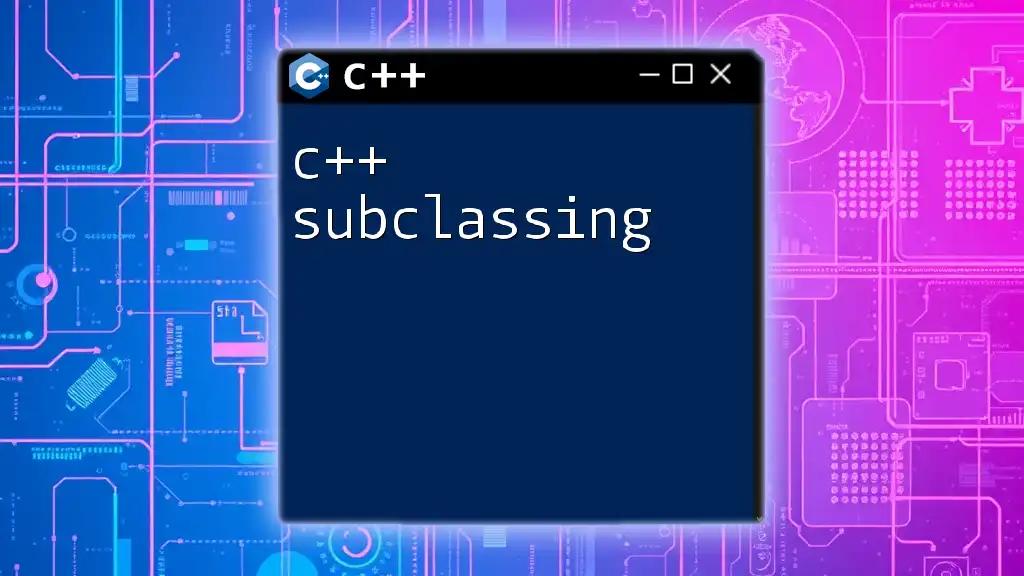
Additional Resources
To enhance your understanding of C++ string operations, consider exploring recommended books, online courses, sample code repositories, and community forums for real-world examples and discussions. Happy coding!