C++ raw string literals allow you to include special characters and multi-line strings without the need for escaping, creating more readable and maintainable code.
#include <iostream>
int main() {
const char* rawString = R"(
This is a raw string literal.
Special characters like \n, \t, and " are included as-is.
)";
std::cout << rawString << std::endl;
return 0;
}
What are Raw String Literals?
C++ raw string literals are a special feature that allows you to define string values without the need to escape special characters, such as quotation marks or backslashes. Unlike regular string literals, which require escape sequences for these characters, raw string literals enable clearer and more readable code. This is particularly useful when dealing with complex strings, such as regex patterns, JSON data, or multiline text.
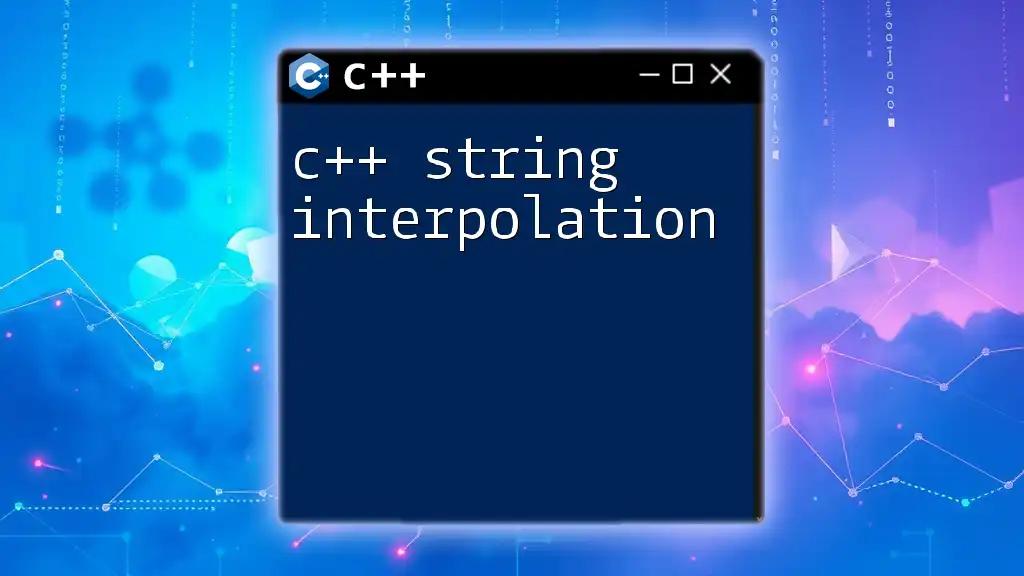
Why Use Raw String Literals in C++?
The primary advantage of using C++ raw string literals is the improvement in code readability. You can write strings as they are intended to appear without worrying about the syntactical constraints of escape sequences. Additionally, it significantly reduces the chance of bugs related to incorrectly escaped characters, which can often be a source of confusion in regular strings.
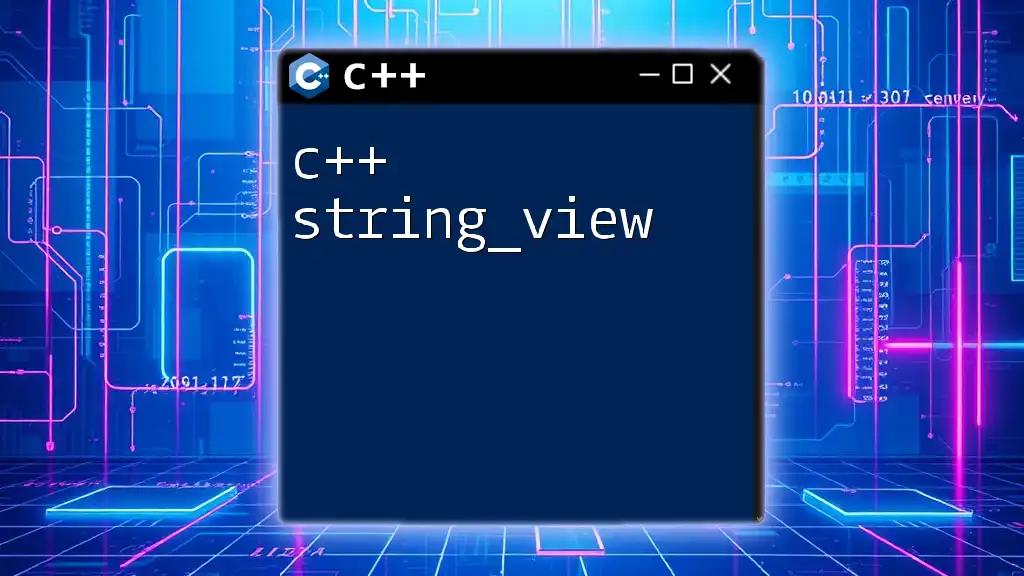
The Syntax of Raw String Literals
The syntax for creating a raw string literal in C++ is as follows:
R"delimiter(raw_string)delimiter"
The `delimiter` is optional but serves as a way to define a boundary for the string, particularly useful if your string contains parentheses or other delimiters. Notably, the delimiter can be any sequence of characters, so you have flexibility in naming it.
Basic Example of a Raw String Literal
Here’s a simple example demonstrating how to declare a raw string literal:
const char* raw_string = R"(Hello, World! This is a raw string.)";
In this example, the entire contents between the parentheses are treated as a literal string without any interpretation of escape sequences.
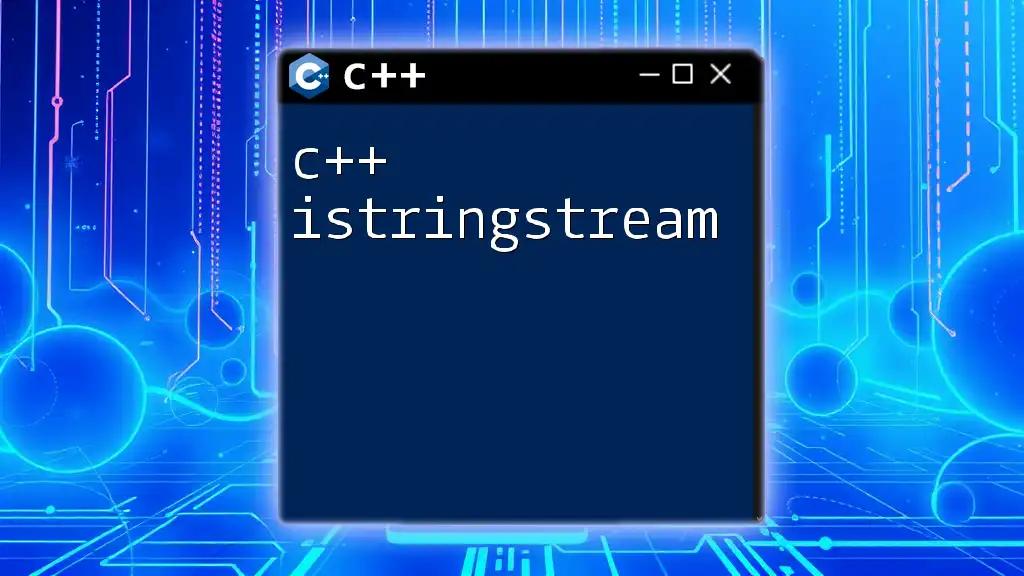
Clarity in Complex Strings
One of the most significant benefits of raw string literals is their usability when working with complex, multiline strings. For instance, consider the scenario where you want to create a multiline string. With regular string literals, it often becomes messy with escape sequences.
Example of a Multi-Line String
Using raw string literals, you can straightforwardly write:
std::string multi_line_string = R"(Line 1
Line 2
Line 3)";
This clarity allows for easier maintenance and better understanding of the string's structure.
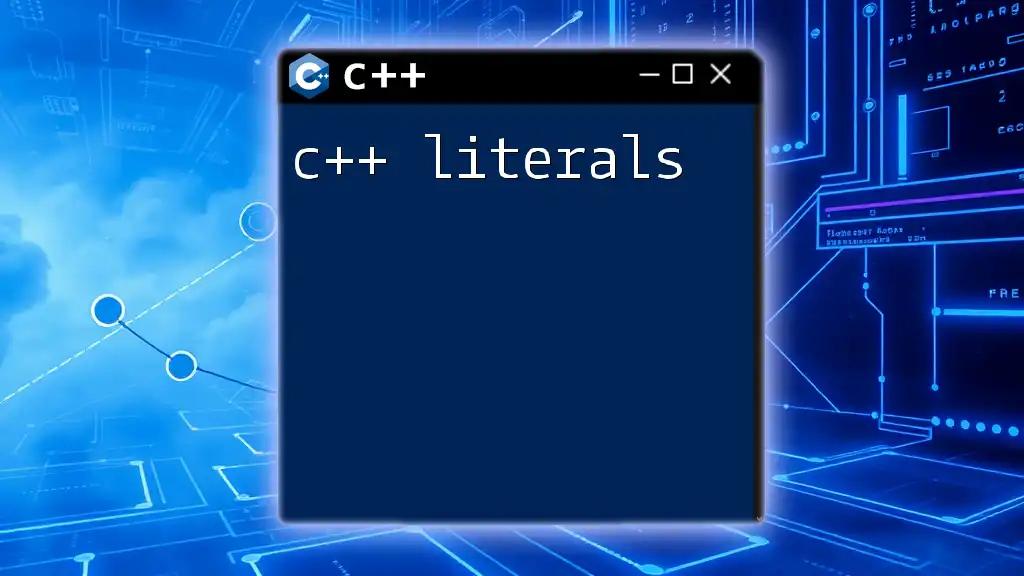
Embedding Special Characters
Another noteworthy feature of C++ raw string literals is the ability to include various special characters without needing escape sequences. This is especially beneficial when dealing with file paths, URLs, or any string containing backslashes or quotes.
Example of Including Special Characters
Here’s how you can include a Windows file path within a raw string:
std::string sample = R"(Path: C:\Users\Name\Documents)";
In this case, the backslashes do not require escaping, making the string more intuitive and less error-prone.
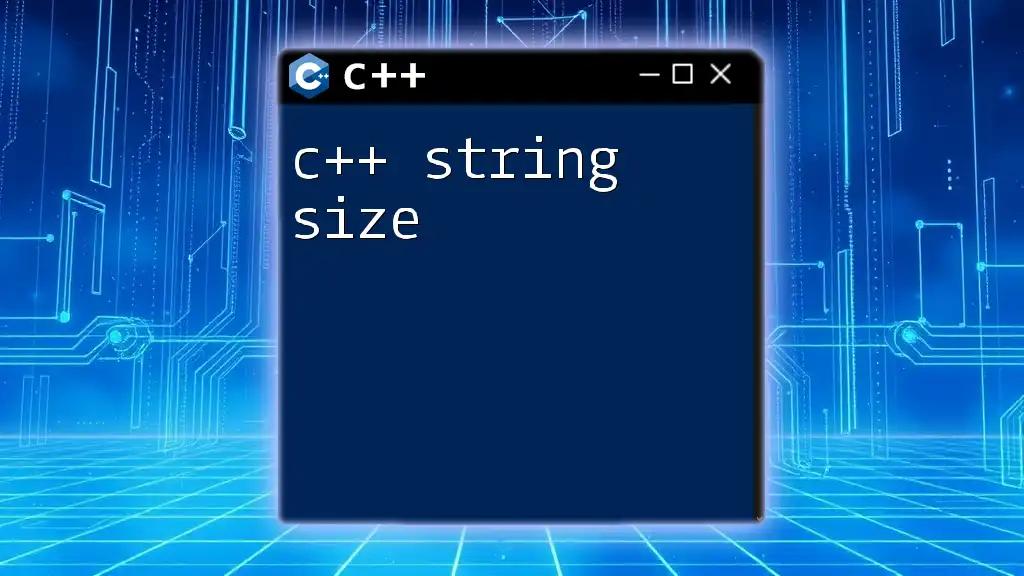
Use Cases in Regular Expressions
Raw string literals shine when it comes to regular expressions. The readability of regex patterns is often compromised by numerous escape characters. With raw string literals, a regex can be declared cleanly.
Regular Expressions Example
Take the following code snippet, which uses a raw string literal for a regex pattern matching a Social Security number format:
std::regex pattern(R"(\d{3}-\d{2}-\d{4})"); // Matches a Social Security number format
In this example, the regex pattern is much clearer and easier to understand as it appears unchanged from its intended format.
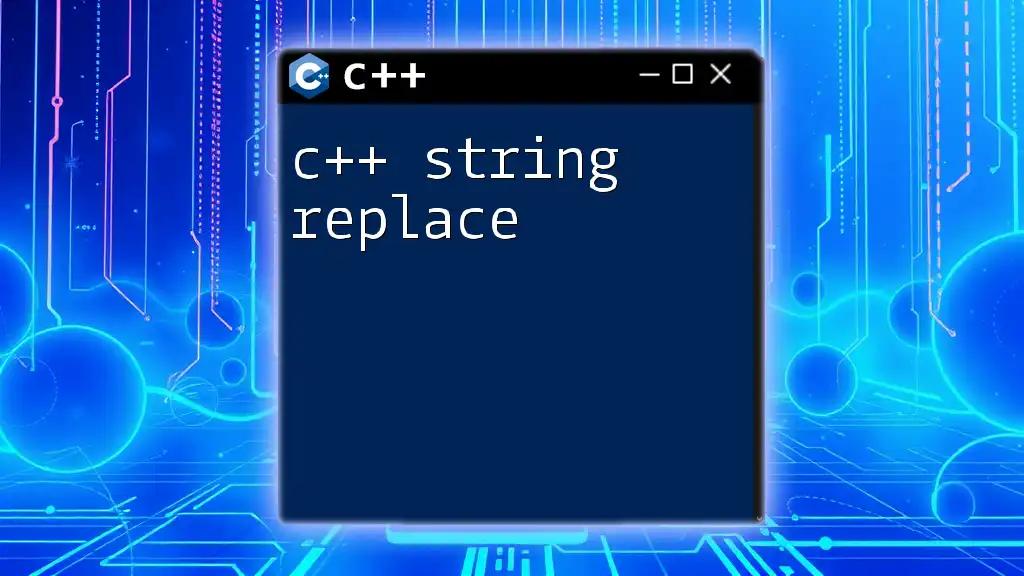
Working with JSON and XML Data
C++ raw string literals are particularly useful when dealing with JSON or XML data. Both formats often contain special characters, making it cumbersome to keep track of escape sequences.
JSON Data Example
Here's how you might write a JSON object as a raw string literal:
std::string json_data = R"({"name": "John", "age": 30})";
This method provides a straightforward way to represent data structures without the headache of escaping quotes.

Limitations of Raw String Literals
While raw string literals offer substantial benefits, they come with certain limitations. One key point to note is the use of delimiters. If the raw string contains the same delimiter used in its declaration, it may cause confusion. This requires careful selection of delimiters.
Best Practices for Choosing Delimiters
Select a unique sequence for delimiters that does not appear in the string content itself. This is crucial for maintaining clarity and avoiding syntax errors.
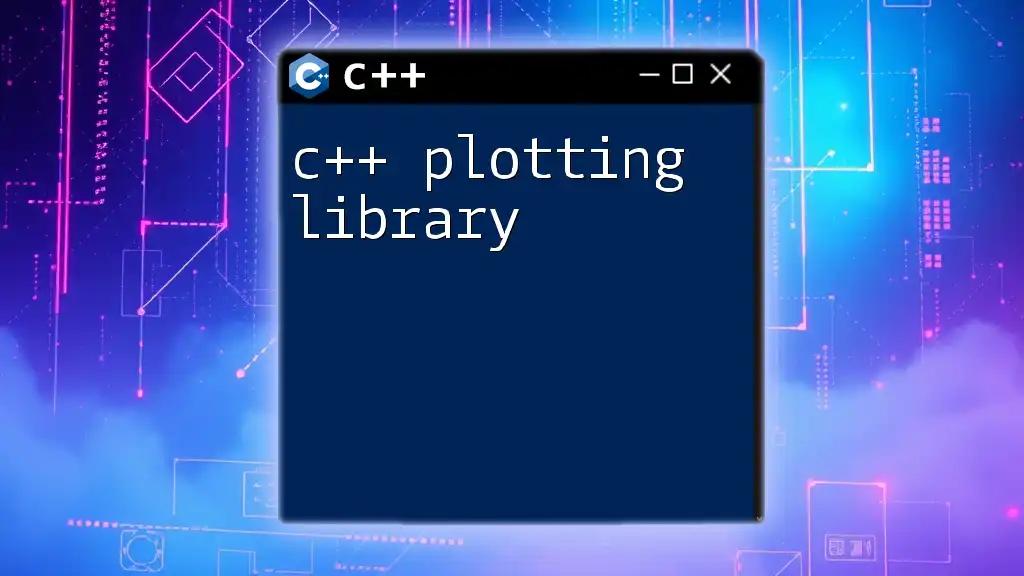
Combining Raw String Literals with Other C++ Features
Raw string literals can seamlessly be combined with other C++ features, which significantly enhances their flexibility.
Concatenating Raw Strings with Regular Strings
You can easily concatenate raw strings with traditional strings. Here’s an example:
std::string combined = std::string(R"(Hello, )") + "World!";
In this scenario, the use of both types of strings is comfortable and practical, maintaining clarity throughout.
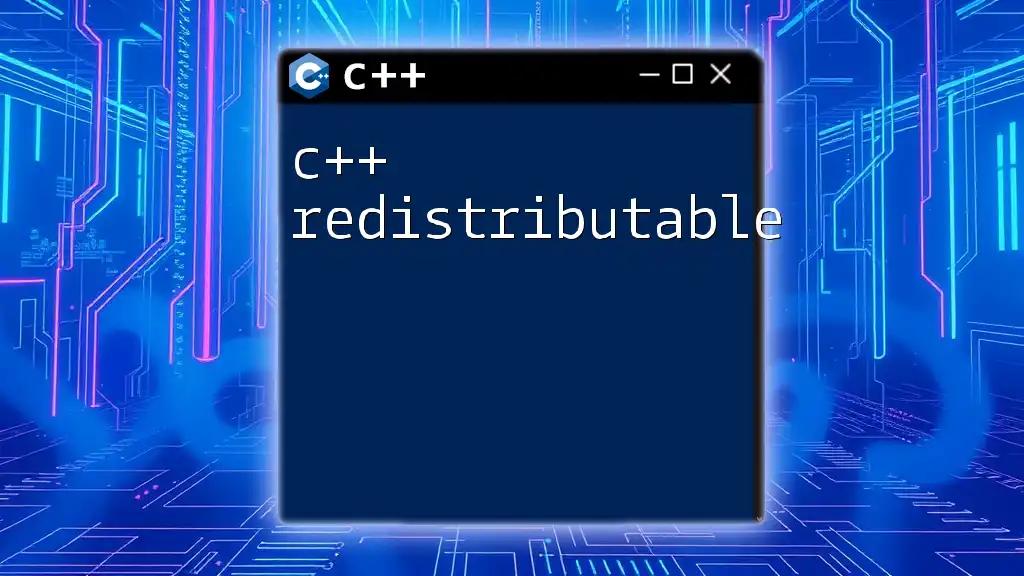
Recap of the Importance of Raw String Literals
In summary, C++ raw string literals provide an excellent way to handle strings in a more readable and maintainable manner. They remove the burden of escape sequences, making your code cleaner, especially when dealing with complex or special characters.
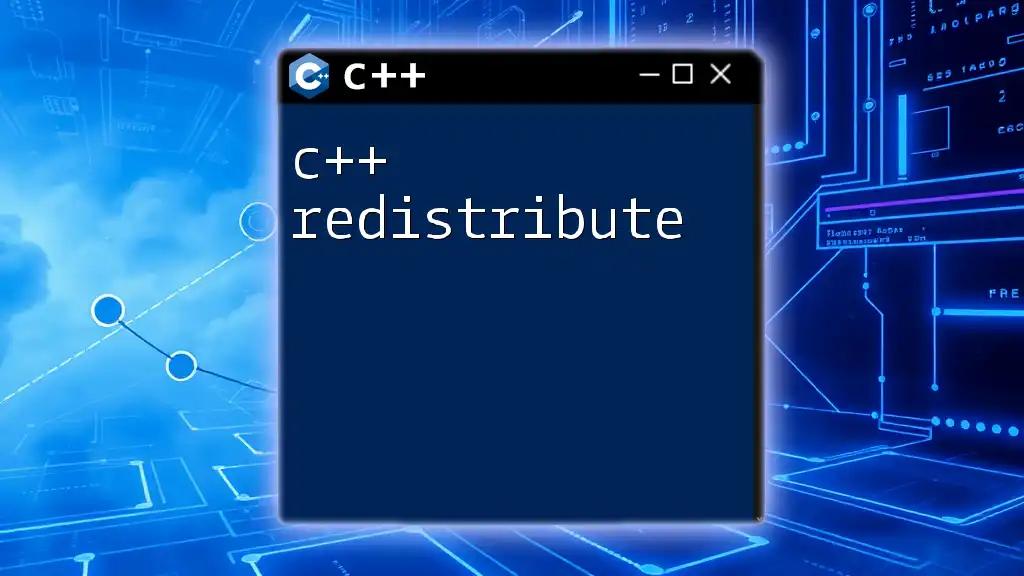
Encouragement to Practice Using C++ Raw String Literals
I encourage you to begin experimenting with C++ raw string literals in your projects. Understanding how to effectively leverage this feature can enhance your coding skills and improve your overall programming experience.
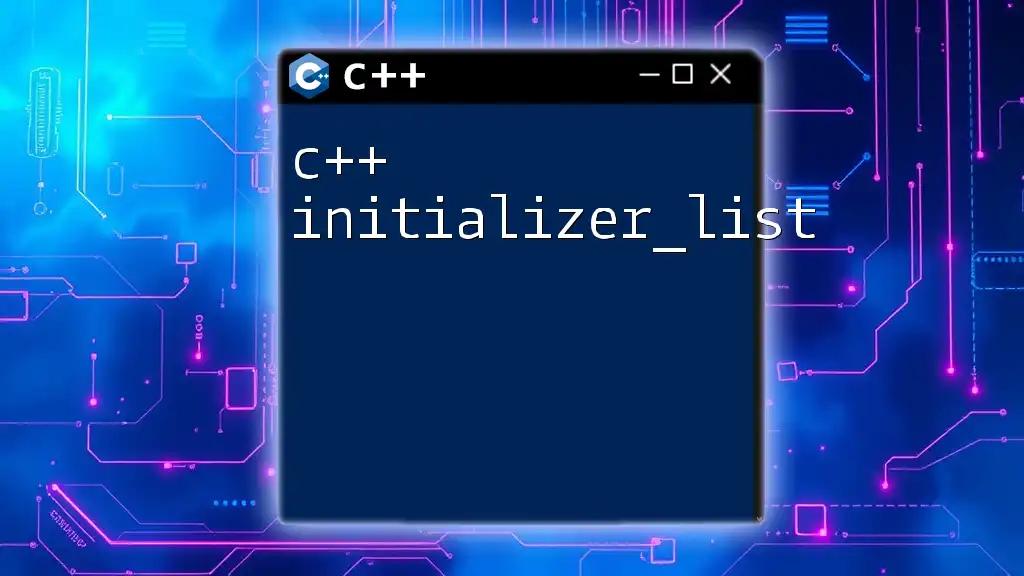
Further Reading and References
For those who wish to delve deeper into C++ programming, consider exploring the official C++ documentation or other recommended books and articles focused on string manipulation and C++ best practices.

Join Our Community
We invite you to join our community to share your experiences and questions regarding C++ raw string literals. Engaging with fellow learners can enrich your knowledge and provide further insights into mastering C++.