In C++, you can replace a substring within a string using the `std::string::replace` method, which allows you to specify the position of the substring to be replaced, its length, and the new substring.
Here's a code snippet that demonstrates this:
#include <iostream>
#include <string>
int main() {
std::string text = "Hello, World!";
text.replace(7, 5, "C++"); // Replaces "World" with "C++"
std::cout << text << std::endl; // Outputs: Hello, C++!
return 0;
}
Understanding Strings in C++
In C++, strings are a fundamental data structure used to represent text. The string class from the C++ Standard Library provides a robust way to handle a sequence of characters. Working with strings frequently involves manipulation tasks, such as concatenation, substring extraction, and importantly, string replacement.
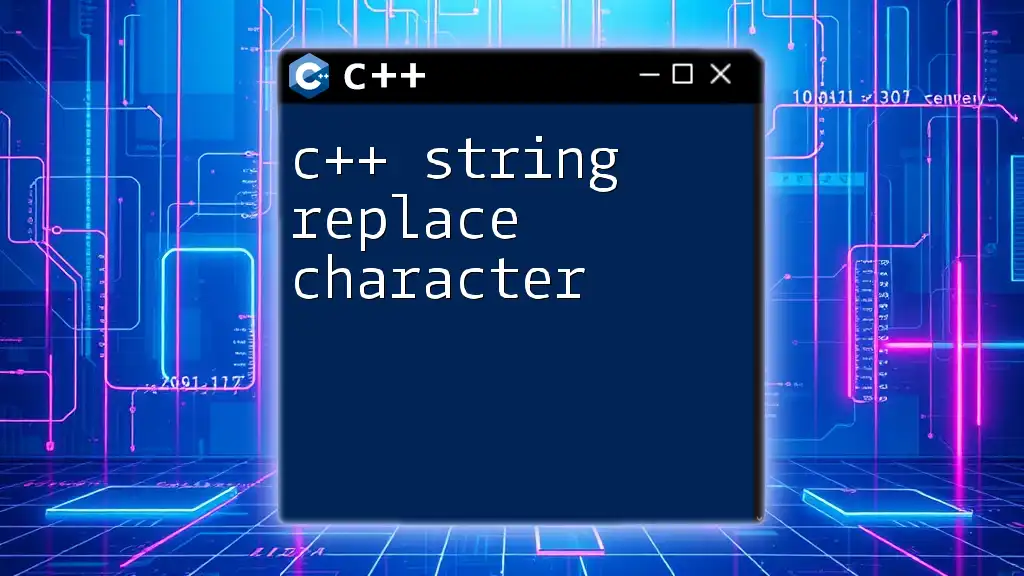
What is String Replacement?
String replacement refers to the process of substituting a portion of a string with a different substring. It’s a common operation in many applications, from simple text editing to complex data processing systems. Understanding how to correctly implement C++ string replace functionality is crucial for effective programming in C++.
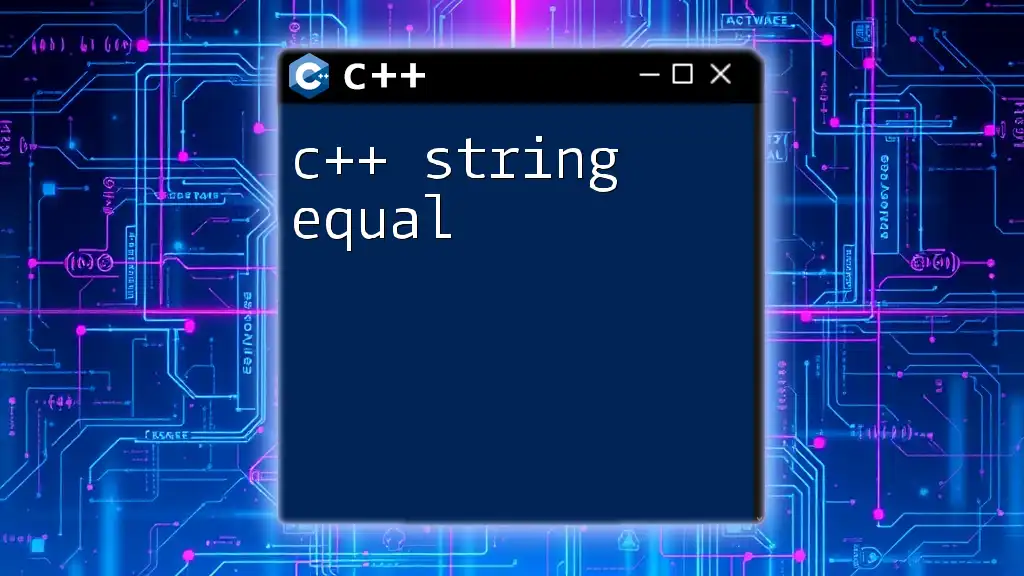
The String.replace() Method in C++
The string.replace() method is the built-in way to perform string replacements in C++. It allows you to target specific sections of a string and replace them with another string.
Overview of the String.replace() Method
The syntax of the `string.replace()` method is as follows:
string& string::replace(size_type pos, size_type len, const string& str);
Here, the parameters include:
- `pos`: The starting index from where the replacement occurs.
- `len`: The number of characters to replace.
- `str`: The string that will be inserted into the original string.
Basic Example of Using string.replace
Let's look at a simple implementation of the `string.replace()` method:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
str.replace(7, 5, "C++");
std::cout << str; // Output: Hello, C++!
return 0;
}
In this example, we replace the substring "World" (which starts at index 7 and has a length of 5) with "C++". The result is a new string: "Hello, C++!".
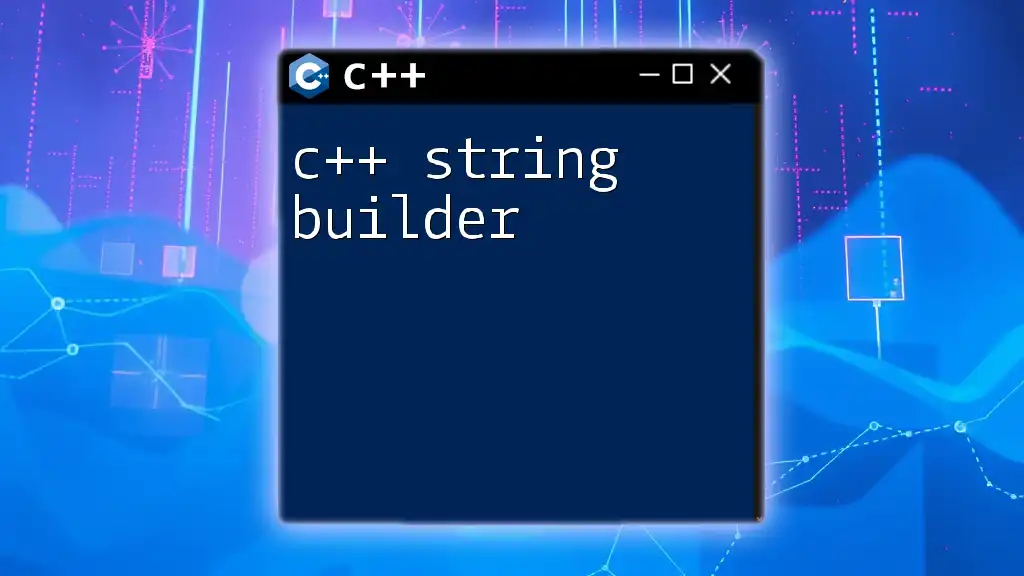
Variations of String Replacement Methods
Using the Replace Method with Different Data Types
The `string.replace()` method can also directly replace individual characters or be used with strings of varying lengths. For instance:
std::string str = "aaaaa";
str.replace(0, 3, "b"); // Output: bbaaa
In this case, we replace the first three characters ('aaa') with 'b', demonstrating how flexible the `replace()` method is when handling different types of replacement.
Replacing Only Part of a String
You may often find that you only need to replace part of a string without affecting the entire content. For example:
std::string str = "I love C++ programming.";
str.replace(7, 3, "C#");
Here, we replace "C++" (which spans from position 7 to 10) with "C#". Consequently, the output will now read: "I love C# programming."
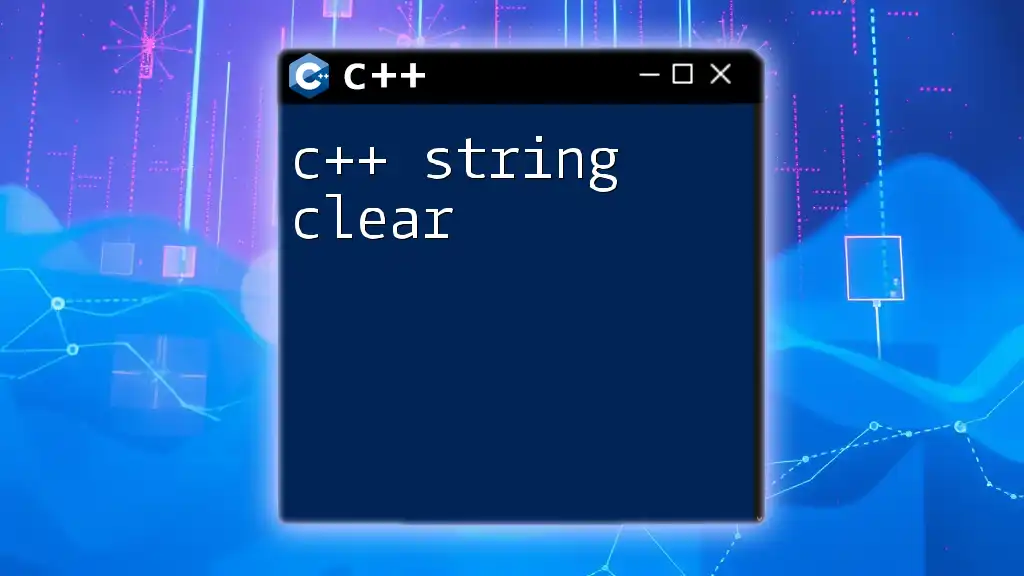
Advanced C++ String Replacement Techniques
Using Regular Expressions for Enhanced String Replacement
For more advanced scenarios, C++ provides the `<regex>` library, which enables powerful pattern-based replacements. This is especially useful when dealing with variable content.
Here is an example using regular expressions:
#include <regex>
#include <string>
int main() {
std::string text = "C++ is great. C++ is powerful.";
std::regex pattern("C\\+\\+");
std::string result = std::regex_replace(text, pattern, "C#");
std::cout << result; // Output: C# is great. C# is powerful.
return 0;
}
In this case, we created a regex pattern that matches "C++" and replaces all occurrences with "C#". Regular expressions are particularly useful when the text to be replaced may vary in format or when you need to match more complex patterns.
Handling Edge Cases in String Replacement
When working with string replacements, it’s important to consider potential edge cases. For instance, what happens if you attempt to replace a substring that doesn’t exist? The method will simply leave the original string unchanged.
Performance is another vital aspect to consider, particularly with large strings or multiple replacements. Efficient string manipulation typically involves minimizing the number of modifications to the original string to avoid overhead from reallocating memory.
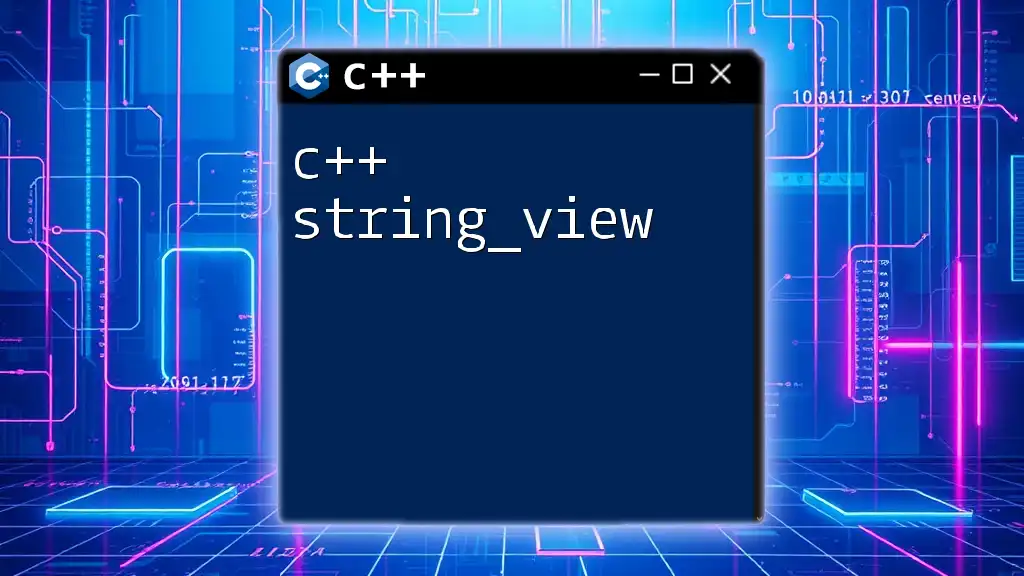
Custom String Replacement Functions
If you need more control or a specialized replacement mechanism, you can implement your custom replace function. Below is a step-by-step guide on how to create one:
- Define the function to accept a string, the substring to replace, and the new substring.
- Use the `find()` method to locate occurrences of the substring within the original string.
- Use the `replace()` method in a loop to perform the replacements.
Here’s a sample implementation:
#include <iostream>
#include <string>
std::string customReplace(const std::string& str, const std::string& from, const std::string& to) {
std::string result = str;
size_t start_pos = 0;
while ((start_pos = result.find(from, start_pos)) != std::string::npos) {
result.replace(start_pos, from.length(), to);
start_pos += to.length(); // Handle case where 'from' and 'to' are different lengths
}
return result;
}
int main() {
std::string original = "Hello, World! Welcome to the World of C++.";
std::string replaced = customReplace(original, "World", "Universe");
std::cout << replaced; // Output: Hello, Universe! Welcome to the Universe of C++.
return 0;
}
In this function, we iterate through the string as long as we can find occurrences of the specified substring, replacing each instance until there are none left. This approach gives you full control over the replacement process.
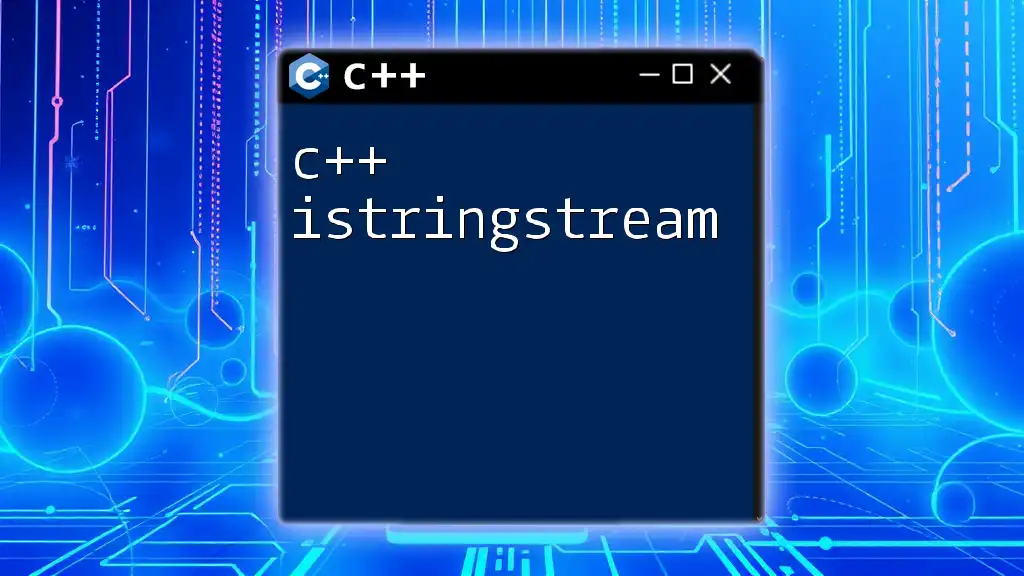
Conclusion
Mastering the C++ string replace functionality opens up various possibilities for text manipulation, enhancing your capability to work with data dynamically. From simple substitutions using `string.replace` to advanced regex techniques, the flexibility is vast. Understanding how to efficiently implement these methods will greatly improve your programming proficiency and contribute to building more robust applications. Exploring custom solutions further extends your toolset, allowing you to address specific needs as they arise. With these skills in hand, you are well on your way to becoming proficient in C++ string manipulation.