In C++, you cannot directly use a `switch` statement with `std::string` types, but you can achieve similar functionality by using if-else statements or a map for cleaner code.
Here’s an example using if-else statements:
#include <iostream>
#include <string>
int main() {
std::string str = "apple";
if (str == "apple") {
std::cout << "It's an apple!" << std::endl;
} else if (str == "banana") {
std::cout << "It's a banana!" << std::endl;
} else {
std::cout << "Unknown fruit." << std::endl;
}
return 0;
}
Understanding C++ Switch Statements
What is a Switch Statement in C++?
A switch statement in C++ is a control structure that allows you to execute specific blocks of code based on the value of a variable. It offers a cleaner alternative to multiple `if-else` conditions, making the code easier to read and maintain. Switch statements are particularly useful when you have a variable that can take on numerous discrete values.
While a switch statement can simplify decision-making in your code, it's essential to note that they only work with discrete data types, such as integers and enums.
Syntax of C++ Switch Statement
Here’s the basic syntax of a switch statement in C++:
switch (variable) {
case value1:
// code to be executed
break;
case value2:
// code to be executed
break;
default:
// code to be executed if no cases match
}
In this syntax:
- `variable` is evaluated once.
- The value is compared with the values in the different `case` statements.
- If a match is found, the code associated with that case is executed.
- The `break` statement prevents the execution from falling through to subsequent cases.
- The `default` case is executed if none of the specified cases match the switch variable.
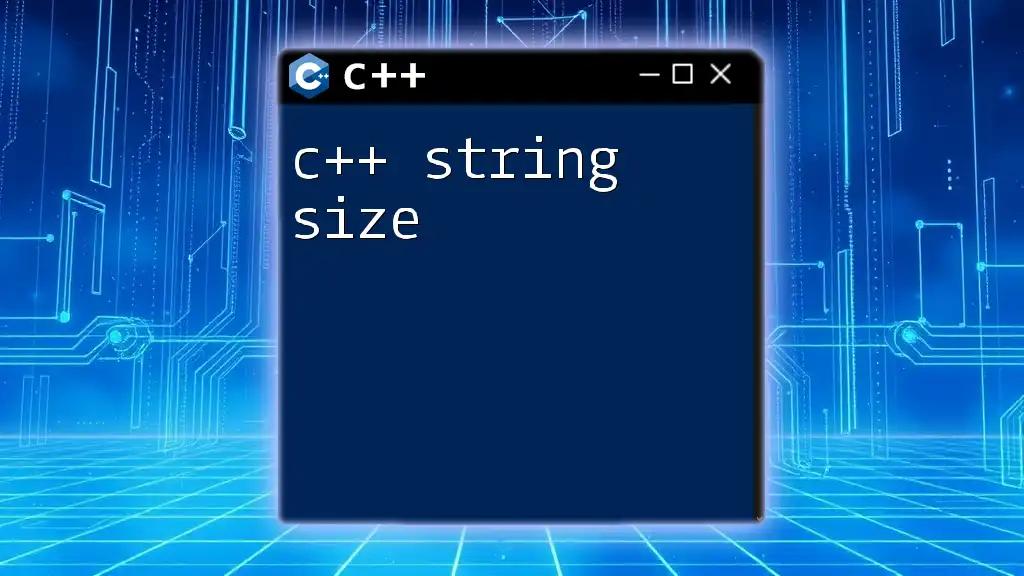
Why Strings Can't Be Directly Used in Switch Statements
Limitations of C++ Switch Statements
One crucial limitation of switch statements is that they only support integral types, such as integers, characters, and enums. Strings are not supported due to their complexity in terms of memory management and performance.
C++ manages strings dynamically and doesn't allow switch statements to operate on them directly because that would involve handling a lot of behind-the-scenes work to compare values. This complexity makes it inefficient for the compiler to handle true string comparisons in switch statements like it does with simple integral types.
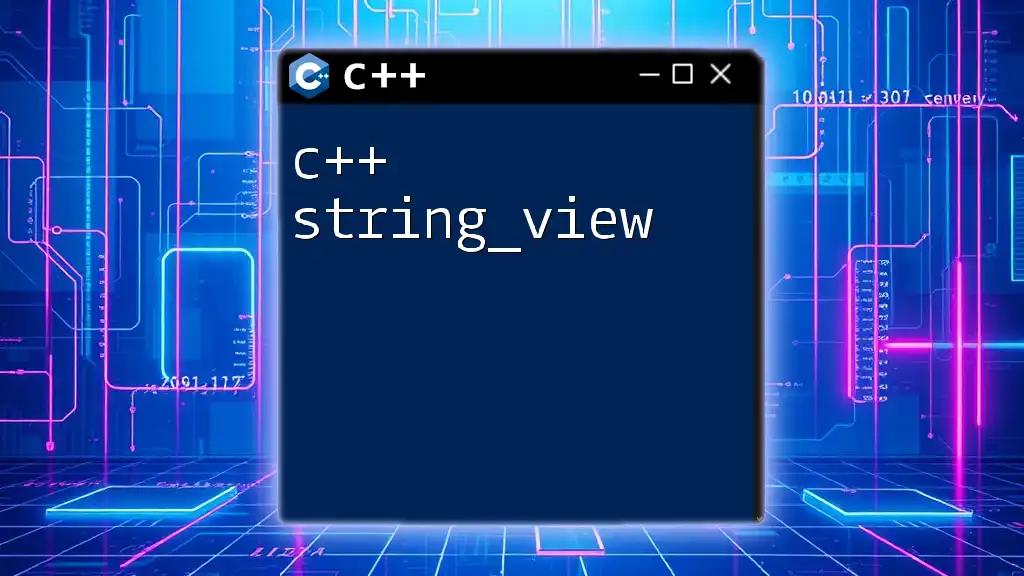
Alternatives to Switch Statements for Strings
Using If-Else Statements
If you want to check string values in a more straightforward way, using `if-else` statements is a common alternative.
Consider this example:
std::string input;
std::cout << "Enter a command: ";
std::cin >> input;
if (input == "case1") {
std::cout << "You selected case 1." << std::endl;
} else if (input == "case2") {
std::cout << "You selected case 2." << std::endl;
} else {
std::cout << "Unrecognized command." << std::endl;
}
This code snippet provides a simple way to handle string comparisons without the constraints of a switch statement. It offers direct control over each case, allowing for flexible coding.
Using Maps as an Alternative
Another effective method is utilizing `std::map` or `std::unordered_map` for string-based "switch-like" behavior. Here’s how it works:
#include <iostream>
#include <unordered_map>
#include <functional>
void case1() {
std::cout << "You selected case 1." << std::endl;
}
void case2() {
std::cout << "You selected case 2." << std::endl;
}
void handleDefault() {
std::cout << "Unrecognized command." << std::endl;
}
int main() {
std::string input;
std::cout << "Enter a command: ";
std::cin >> input;
std::unordered_map<std::string, std::function<void()>> actions;
actions["case1"] = case1;
actions["case2"] = case2;
actions["default"] = handleDefault;
if (actions.find(input) != actions.end()) {
actions[input](); // Executes the corresponding function
} else {
actions["default"](); // Calls the default case
}
return 0;
}
In this example, string values map directly to assigned functions. When a user input matches a key in the map, the corresponding function executes, providing a neat and scalable solution.
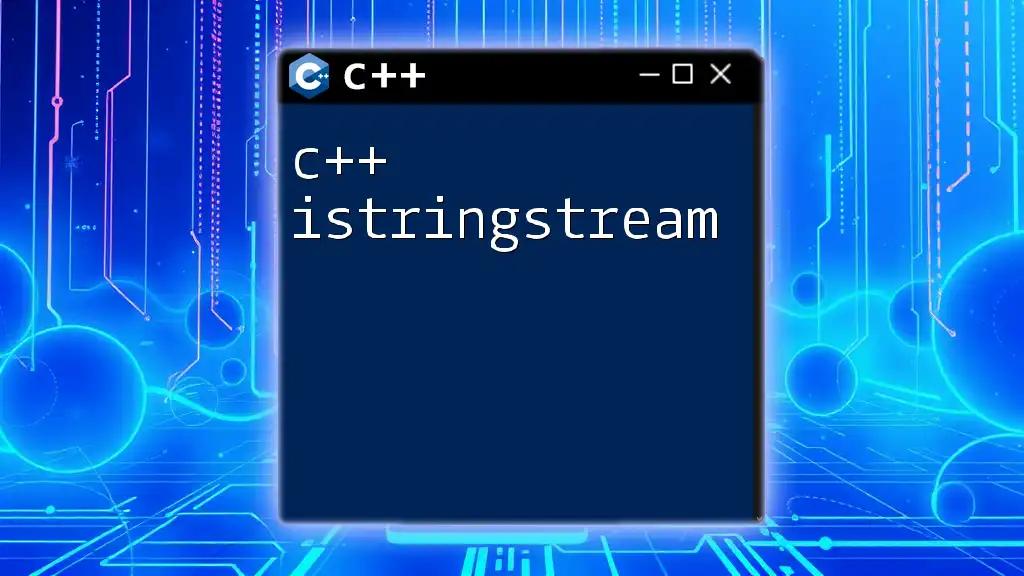
Implementing a Switch-Case-Like Structure with Strings
Using the `std::variant` and `std::visit`
With C++17, you can use `std::variant` to create a type-safe union that can hold different data types.
Using `std::visit`, you can implement a switch-case-like behavior for strings, like so:
#include <iostream>
#include <variant>
#include <string>
int main() {
std::variant<std::string, int> v = "case1";
std::visit([](auto&& arg) {
if (arg == "case1") {
std::cout << "You selected case 1." << std::endl;
} else if (arg == "case2") {
std::cout << "You selected case 2." << std::endl;
} else {
std::cout << "Unrecognized command." << std::endl;
}
}, v);
return 0;
}
This approach provides a flexible and type-safe way to handle multiple cases. However, it might still lack some clarity compared to a traditional switch statement.
Exploring Boost Library Alternatives
For those looking for an enriched library experience, the Boost library offers `Boost.Variant`, which allows for more advanced data handling and can simulate switch-case behavior using strings.
By leveraging Boost, you gain powerful features for variable types which facilitate easier manipulation of strings and other types, providing clearer semantics around type interactions within your switch-like structures.

Best Practices for String Handling in C++
Using Enumerations with Strings
A more structured approach is to convert string values into enums, which allows for more efficient comparisons while maintaining type safety. For instance:
#include <iostream>
#include <string>
enum Command {
CASE1,
CASE2,
UNRECOGNIZED
};
Command getCommandEnum(const std::string& input) {
if (input == "case1") return CASE1;
if (input == "case2") return CASE2;
return UNRECOGNIZED;
}
int main() {
std::string input;
std::cout << "Enter a command: ";
std::cin >> input;
switch (getCommandEnum(input)) {
case CASE1:
std::cout << "You selected case 1." << std::endl;
break;
case CASE2:
std::cout << "You selected case 2." << std::endl;
break;
default:
std::cout << "Unrecognized command." << std::endl;
break;
}
return 0;
}
In this example, string commands are converted into enumerated types, thereby allowing you to use a switch statement efficiently and safely.
Performance Considerations
Efficiency is critical when processing numerous comparisons. By utilizing if-else statements or maps for small sets of conditions, any performance hits become negligible. However, if you’re working with larger datasets, using enums is optimal due to its straightforward comparison inherent in switch statements.
When performance is crucial, favor strategies that balance readability and execution speed—often using enumerations when possible for high-frequency operations.

Conclusion
In summary, while a C++ string switch may not exist in its traditional form, there are several alternatives worth exploring. This guide covered why strings are not valid in switch statements and offered various robust methods such as `if-else` chains, using maps, `std::variant`, and enumerations. Each method has its pros and cons, and understanding them allows you to choose the best option for your specific scenario.
Experimenting with these methods in real projects will deepen your understanding and versatility as a C++ programmer. Embrace the flexibility within these alternatives, and always keep performance considerations in mind.