A C++ stopwatch can be created using the `<chrono>` library to measure elapsed time accurately in a program. Here's a simple code snippet demonstrating how to implement a stopwatch in C++:
#include <iostream>
#include <chrono>
#include <thread>
int main() {
auto start = std::chrono::high_resolution_clock::now(); // Start the stopwatch
std::this_thread::sleep_for(std::chrono::seconds(3)); // Simulate some work (3 seconds)
auto end = std::chrono::high_resolution_clock::now(); // End the stopwatch
std::chrono::duration<double> duration = end - start; // Calculate the duration
std::cout << "Elapsed time: " << duration.count() << " seconds." << std::endl; // Output the elapsed time
return 0;
}
Understanding Time Measurement in C++
When programming, accurate timing measurements are crucial, especially for performance evaluation, benchmarking, and even in games. In C++, the <chrono> library provides a robust and intuitive approach for timekeeping, including functions that enable you to move beyond simple clock readings to implementing functional time-tracking features like a C++ stopwatch.
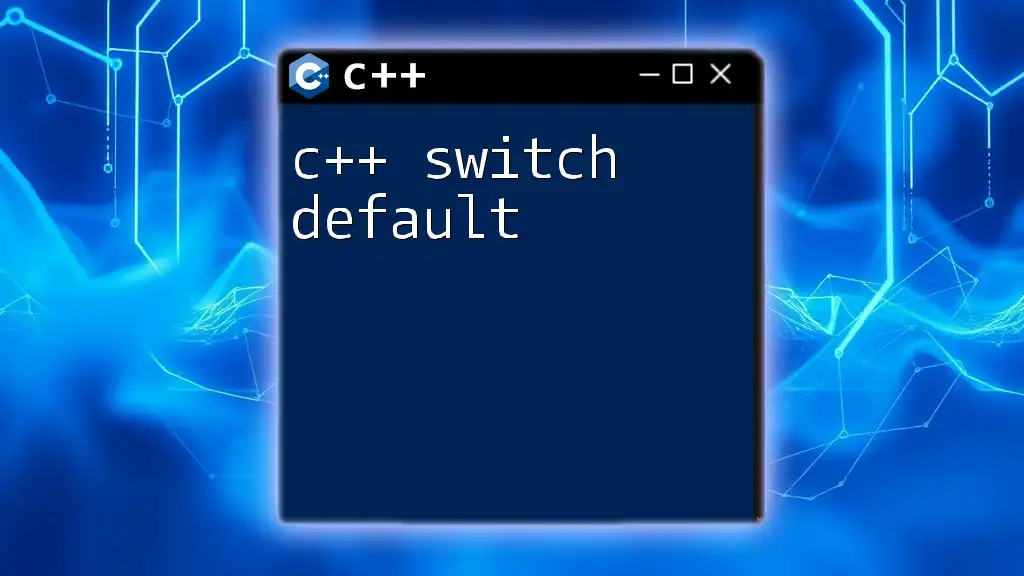
Setting Up Your C++ Stopwatch
Including Necessary Libraries
To get started, you'll need to include the appropriate C++ libraries in your code. The `<chrono>` library is essential for high-resolution timing.
#include <iostream>
#include <chrono>
- iostream is used for input and output operations, while chrono allows you to track time in high precision.
Defining Your Stopwatch Class
Creating a stopwatch entails designing a class that encapsulates timer behavior. This class will manage time tracking internally, organizing your code logically and efficiently.
class Stopwatch {
// Class members and methods go here
};
In this class, you’ll define several important features, such as the start time, end time, and elapsed time, helping you maintain the stopwatch state.
Stopwatch Class Members
Private Members
- start_time: A variable to store the moment the stopwatch begins.
- end_time: A variable to store the moment the stopwatch stops.
- elapsed_time: A variable to hold the time difference between these points.
Public Methods
- start(): This method initializes the stopwatch by capturing the current time.
- stop(): This method captures the time when you want the stopwatch to stop.
- getElapsed(): This retrieves the time elapsed between the start and stop events.
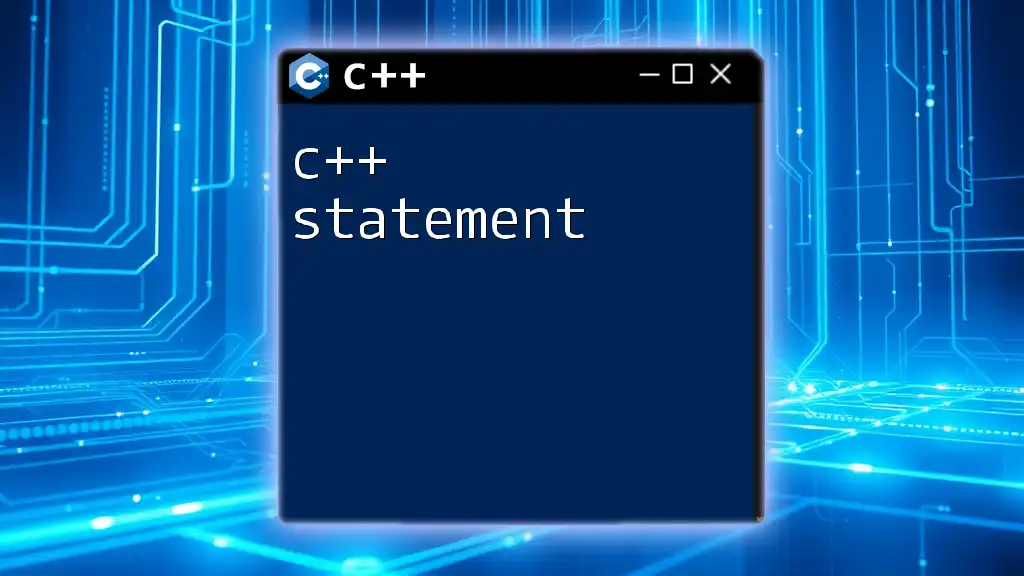
Implementing Basic Stopwatch Functionality
Writing the Start Method
The `start` method captures the current high-resolution time when the stopwatch is activated. This data will serve as your starting point.
void start() {
start_time = std::chrono::high_resolution_clock::now();
}
By invoking `std::chrono::high_resolution_clock::now()`, you're ensuring maximum precision for tracking elapsed time.
Writing the Stop Method
To stop the stopwatch, you'll create a method that captures the ending time.
void stop() {
end_time = std::chrono::high_resolution_clock::now();
}
This function is critical as it provides the endpoint necessary to calculate the elapsed duration.
Calculating Elapsed Time
The elapsed time can be calculated by subtracting the start time from the end time. This is where the `getElapsed` method comes into play.
double getElapsed() {
std::chrono::duration<double> elapsed = end_time - start_time;
return elapsed.count();
}
The `elapsed.count()` method returns the duration in seconds, providing an easy-to-read format.
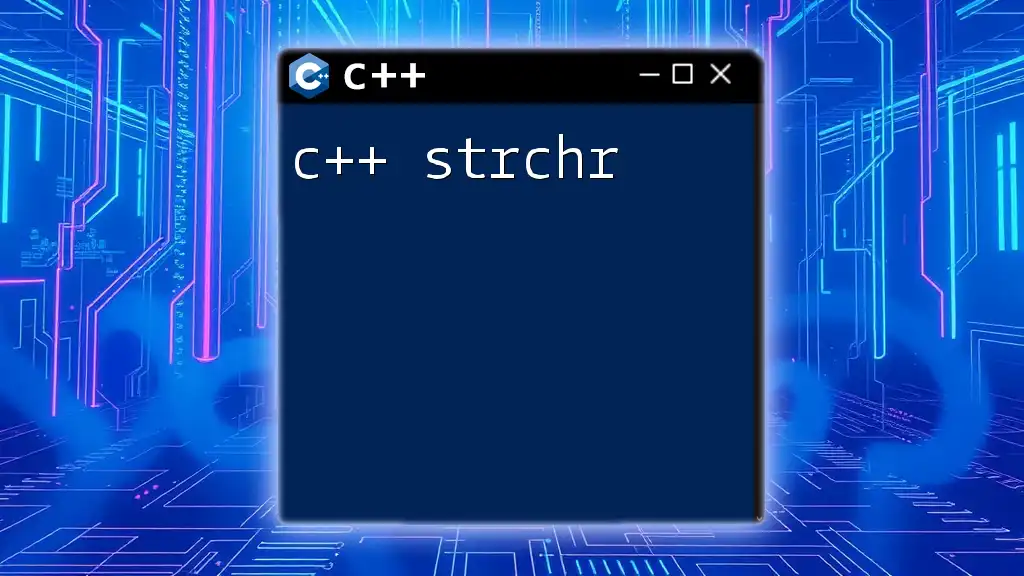
Complete C++ Stopwatch Example
Now that you’ve defined the class and implemented the essential methods, here is how it all fits together in a complete example:
#include <iostream>
#include <chrono>
class Stopwatch {
private:
std::chrono::high_resolution_clock::time_point start_time;
std::chrono::high_resolution_clock::time_point end_time;
public:
void start() {
start_time = std::chrono::high_resolution_clock::now();
}
void stop() {
end_time = std::chrono::high_resolution_clock::now();
}
double getElapsed() {
std::chrono::duration<double> elapsed = end_time - start_time;
return elapsed.count();
}
};
int main() {
Stopwatch stopwatch;
stopwatch.start();
// Simulate a long task
for (volatile int i = 0; i < 1e8; ++i);
stopwatch.stop();
std::cout << "Elapsed time: " << stopwatch.getElapsed() << " seconds\n";
return 0;
}
In this example, the `main()` function creates an instance of `Stopwatch`, starts it, simulates a delay using a loop, and then stops the timer. The elapsed time is displayed in seconds, demonstrating the stopwatch's effectiveness.
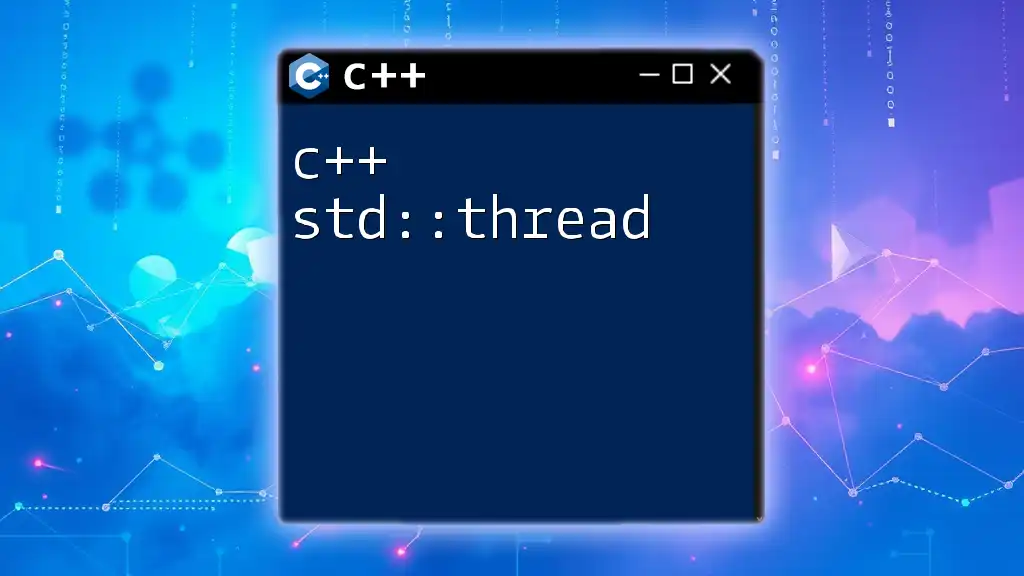
Enhancements for Your Stopwatch
Adding Lap Timing
To enhance the functionality of your C++ stopwatch, you might consider implementing a feature that allows for lap timing. This function records the time at various intervals without resetting the stopwatch.
void lap() {
// Implementation goes here
}
By storing the lap times in a collection, you can maintain a history of intervals, providing valuable insights for performance measurement.
Format Elapsed Time
Moreover, customizing the display of elapsed time can increase its usability, especially when dealing with larger intervals.
void formatElapsed() {
// Convert seconds to minutes and seconds
}
By breaking down the elapsed time into more digestible formats, such as minutes and seconds, you enhance the user experience.
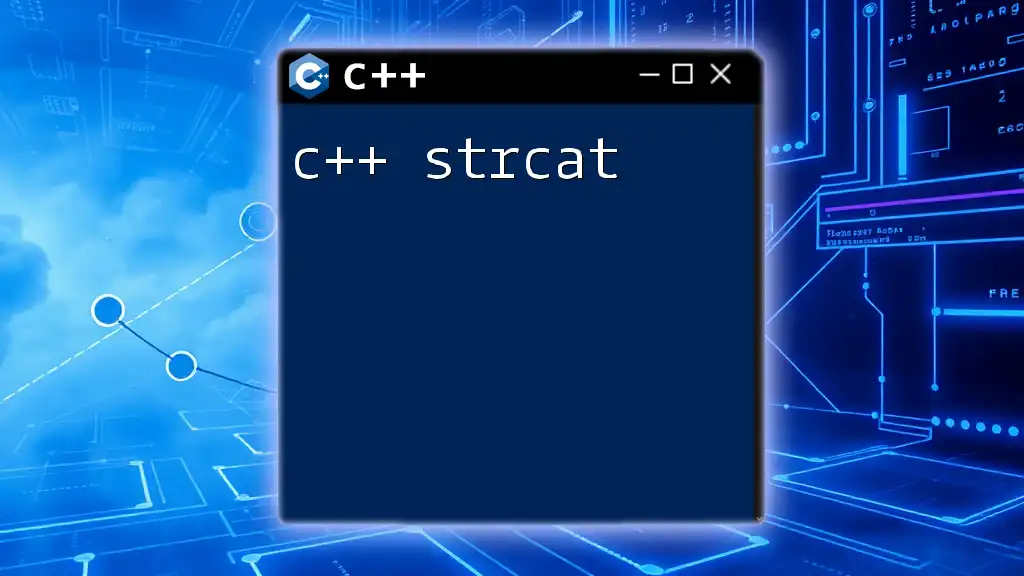
Conclusion
In this guide, we explored the critical aspects of implementing a C++ stopwatch, outlining how to use the powerful `<chrono>` library effectively. By following the steps outlined here, you can create a functional and efficient stopwatch for your C++ applications.
Understanding how to measure time accurately is not just about coding; it’s about honing your programming skills to develop robust and efficient applications. As you practice building your stopwatch, consider implementing additional features and experimenting with different time measurements.
Now it’s your turn—take what you’ve learned and create your custom C++ stopwatch!