A C++ static array is a fixed-size data structure that allows storage of multiple elements of the same type, where the size is determined at compile time and cannot be changed at runtime.
#include <iostream>
int main() {
int staticArray[5] = {1, 2, 3, 4, 5}; // Declaration of a static array of integers
for (int i = 0; i < 5; ++i) {
std::cout << staticArray[i] << " ";
}
return 0;
}
Understanding Static Arrays
What is an Array?
An array is a collection of elements of the same type stored in contiguous memory locations. It is essentially a data structure that allows you to store multiple values under a single variable name. Arrays enable efficient management of data, especially when dealing with collections of items, like a list of scores, user IDs, or configurations settings.
Distinction Between Static and Dynamic Arrays
Static arrays have a fixed size, determined at compile time. This means that once you declare a static array, its size cannot be changed during program execution. On the other hand, dynamic arrays can resize themselves during runtime, providing more flexibility when handling unknown or varying data sizes. However, this flexibility comes at the cost of more complex memory management.

Declaring Static Arrays in C++
Syntax of Static Array Declaration
Declaring a static array in C++ is quite straightforward. The general syntax is:
type arrayName[size];
For example, if you want to declare an integer array named `myArray` that can hold five elements, you would write:
int myArray[5];
This snippet creates an integer array capable of holding five values.
Initializing Static Arrays
Static arrays can be initialized in various ways. An array can be initialized at the time of declaration, which is often considered a good practice as it provides immediate clarity about the array's intended content.
For instance:
int myArray[5] = {1, 2, 3, 4, 5};
In this case, all five elements are explicitly initialized.
If you only partially initialize an array, the remaining elements will be automatically set to zero:
int myArray[5] = {1, 2}; // The third, fourth, and fifth elements are initialized to 0.

Accessing and Modifying Static Array Elements
Accessing Array Elements
Each element in a static array can be accessed using its index. Remember that C++ uses zero-based indexing, meaning that the first element is at index 0, the second at index 1, and so on.
To access the third element in `myArray`, you would write:
int value = myArray[2]; // Accesses the third element (value will be 3).
Understanding how to properly access array elements is critical for manipulating data effectively.
Modifying Array Elements
Modifying the values of an array is just as simple as accessing them. If you want to change the fourth element of `myArray` to 10, you can do so with:
myArray[3] = 10; // Changes the fourth element to 10.
This flexibility allows developers to easily update data held within a static array.

Common Operations with Static Arrays
Iterating Through Static Arrays
One of the most common operations performed on arrays is iteration. By using loops, such as `for` or `while`, you can efficiently traverse the elements of a static array.
Here’s an example that prints each element in `myArray`:
for (int i = 0; i < 5; i++) {
std::cout << myArray[i] << " ";
}
This loop will output the values stored in the array, enabling you to process data in bulk.
Finding the Length of a Static Array
Knowing the size of a static array is vital when performing operations on it. You can easily determine the number of elements in an array using the `sizeof` operator. It allows you to compute the byte size of the array and then divide it by the size of one element:
size_t length = sizeof(myArray) / sizeof(myArray[0]);
This line of code effectively gives you the number of elements in `myArray`, which is essential for safe iteration.

Applications of Static Arrays in C++
Storing Fixed Data
Static arrays are particularly useful for storing fixed collections of data. For example, if you are developing a game, you might use a static array to track player scores that won’t change in size:
int playerScores[10] = {0}; // Initializes array with 10 players, all scores set to 0.
Multi-Dimensional Arrays
C++ also supports multi-dimensional static arrays, allowing you to create arrays with more than one dimension, much like a math matrix. You can declare a two-dimensional array as follows:
int matrix[3][3] = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
This represents a 3x3 grid of integers, making it perfect for applications like image processing or game maps.
Use Cases and Best Practices
When deciding whether to use a static array, consider its practical applications. They are ideal in scenarios where the size of the dataset is known beforehand or remains constant throughout the execution of the application. For instance, if you’re storing a fixed number of user permissions or status flags, static arrays would be an excellent fit due to their efficiency and simplicity.
However, be cautious of drawbacks such as wasted memory if the array is not fully utilized, and the inability to resize once declared.
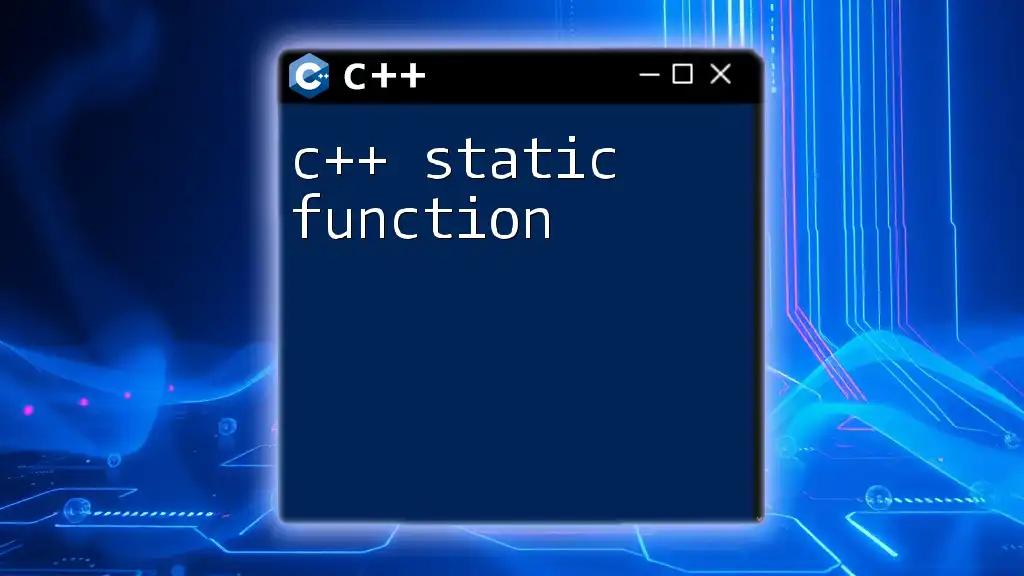
Advantages and Disadvantages of Static Arrays
Advantages
Static arrays are known for their speed and efficiency. Because their size is fixed, accessing elements is very fast, as it simply requires calculating a memory address. Moreover, static arrays incur less overhead in terms of memory management since they do not require dynamic allocation.
Disadvantages
On the flip side, static arrays lack flexibility. Once you declare one, you cannot change its size, which can lead to wasted memory if the full capacity is not utilized. They are not suited for applications where the size of the dataset can fluctuate dynamically. You also risk buffer overflow errors if you try to access an index outside the declared range.

Conclusion
Recap
In summary, this comprehensive guide has covered the fundamental concepts, operations, and applications of C++ static arrays. You learned how to declare, initialize, access, and modify elements within static arrays, along with common operations like iterating through elements.
When to Use Static Arrays
Static arrays are best employed in scenarios where the size of the data set is known and unchanging. They offer efficiency and simplicity, making them a go-to choice for many fixed data situations. On the other hand, if you find yourself needing to change the size of your dataset dynamically, consider exploring the realm of dynamic arrays or other data structures.
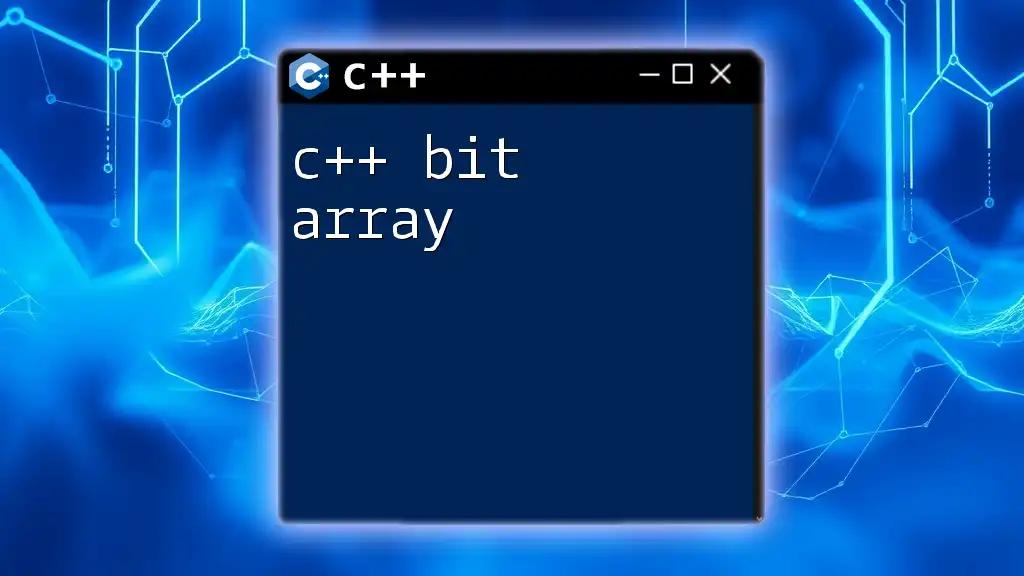
Additional Resources
For those who wish to deepen their understanding of C++ static arrays, consider seeking out recommended texts on data structures and algorithms, along with various online forums where you can engage with the community. These resources will further enhance your knowledge and practical skills in effectively utilizing C++ arrays.