A C++ dynamic array is a resizable array allocated on the heap that allows for flexible memory usage during runtime.
#include <iostream>
int main() {
int size;
std::cout << "Enter the number of elements: ";
std::cin >> size;
// Create a dynamic array
int* dynamicArray = new int[size];
// Fill the dynamic array
for (int i = 0; i < size; i++) {
dynamicArray[i] = i + 1; // Assign values
}
// Output the dynamic array
std::cout << "Dynamic Array Elements: ";
for (int i = 0; i < size; i++) {
std::cout << dynamicArray[i] << " ";
}
// Deallocate memory
delete[] dynamicArray;
return 0;
}
Basics of C++ Arrays
What is an Array in C++?
In C++, an array is a collection of elements that are stored in contiguous memory locations. The elements must be of the same data type, allowing for efficient access and manipulation of data. Typically, arrays are declared with a fixed size, which is known at compile time during static allocation.
Static Arrays vs. Dynamic Arrays
Static arrays have a predetermined size, which can lead to limitations, particularly in situations where the size of the required array isn't known at compile time. This rigidity can lead to wasted memory if the array is overly large or inadequate space if it is too small. In contrast, dynamic arrays provide flexibility, allowing you to allocate memory during runtime based on user input or other conditions.
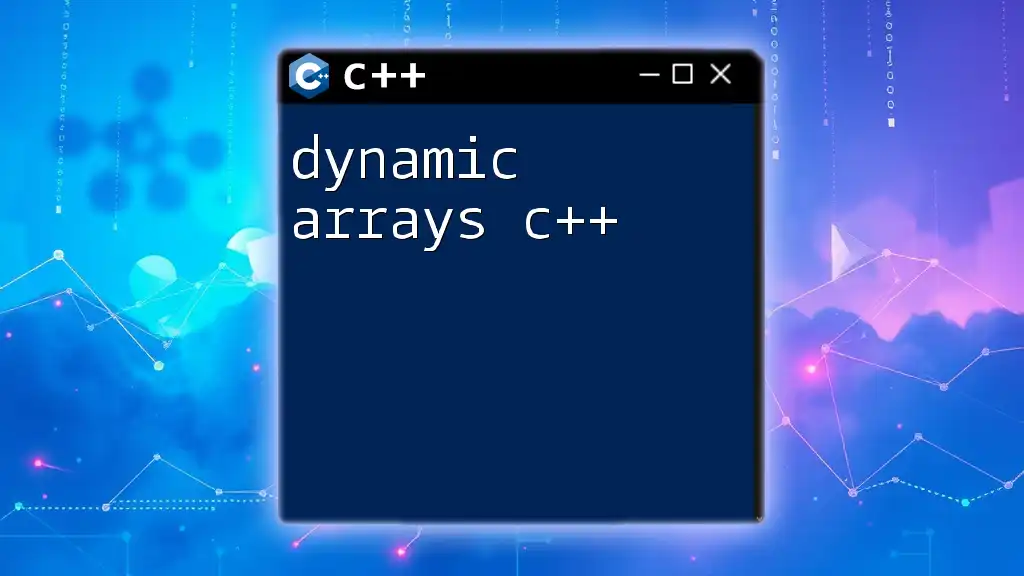
Understanding Dynamic Arrays in C++
What is a Dynamic Array in C++?
A dynamic array in C++ is an array whose size can be determined during runtime. This means that you can create an array size based on the requirements of your program without the limitations imposed by static arrays. The primary benefit of dynamic arrays is that they allow you to use memory more efficiently and adaptively.
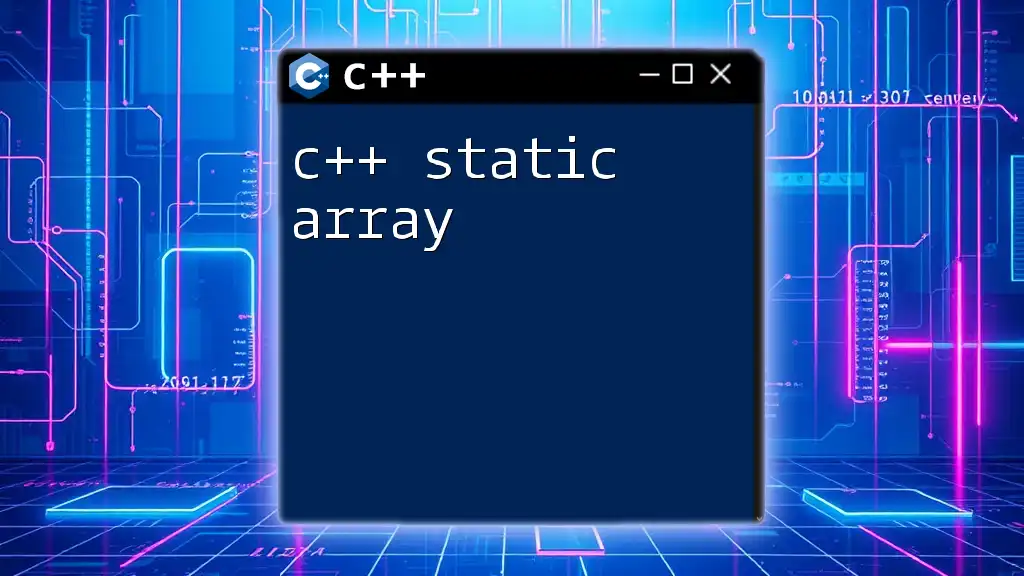
How to Create Dynamic Arrays in C++
Dynamic Array Creation Syntax
To create a dynamic array in C++, you will generally use the `new` keyword followed by the data type of the array, and the size of the array you would like to create.
Using `new` Keyword for Dynamic Allocation
Here's a simple example of how to create a dynamic array:
int size = 5; // Example size
int* arr = new int[size]; // Allocating memory for a dynamic array
In this code, `arr` is a pointer to the first element of the dynamically allocated array of integers. The `new` keyword allocates the specified amount of memory on the heap.
Initialising Dynamic Arrays
After creating a dynamic array, you may want to initialize it. You can do this using loops:
for(int i = 0; i < size; i++) {
arr[i] = i * 2; // Assign values to the array
}
Here, we are populating the array with even numbers based on the index. This initialization allows for immediate usability of the array data.
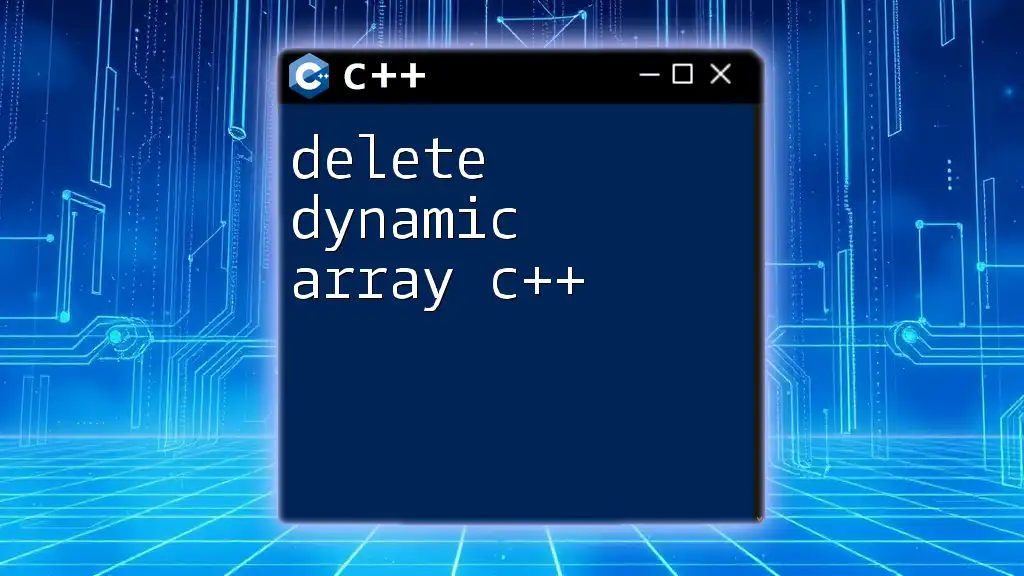
Managing Dynamic Arrays
Accessing Elements in a Dynamic Array
Accessing elements in a dynamic array is done similarly to static arrays, using the index:
std::cout << arr[0]; // Accessing the first element
This provides a clear and quick way to retrieve data stored in your dynamic array.
Resizing a Dynamic Array
One challenge with dynamic arrays is resizing them. Since they have a fixed size once allocated, you cannot simply change their size. To resize a dynamic array, follow these steps:
- Create a new array of the desired new size.
- Copy existing elements from the old array to the new array.
- Deallocate the memory of the old array and reassign the pointer.
Here’s an example:
int *newArr = new int[newSize];
for(int i = 0; i < oldSize; i++) {
newArr[i] = arr[i]; // Copying elements to new array
}
delete[] arr; // Deallocate old memory
arr = newArr; // Reassigning pointer to the new array
This method ensures that you carry over the existing data while adapting to new size requirements.
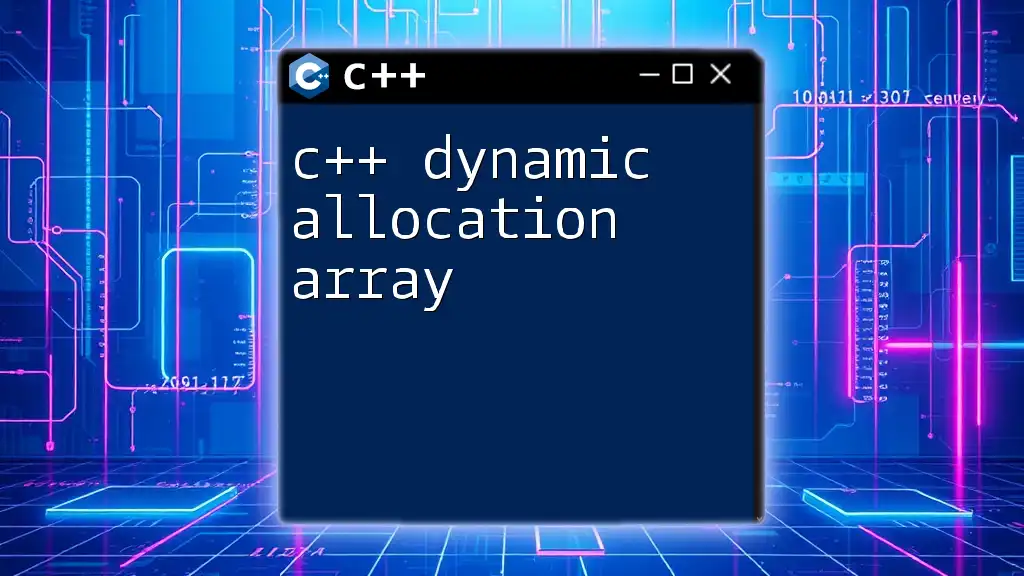
Deallocating Memory for Dynamic Arrays
Importance of Memory Management
Managing memory is crucial in C++ programming, especially when using dynamic arrays. Failing to properly deallocate memory can lead to memory leaks, which can degrade performance or lead to crashes.
Using `delete` Keyword
When you are finished using a dynamic array, you must release the memory back to the system using the `delete` keyword:
delete[] arr; // Freeing dynamic array memory
This code ensures that the resources allocated for the dynamic array are returned, preventing memory leaks.
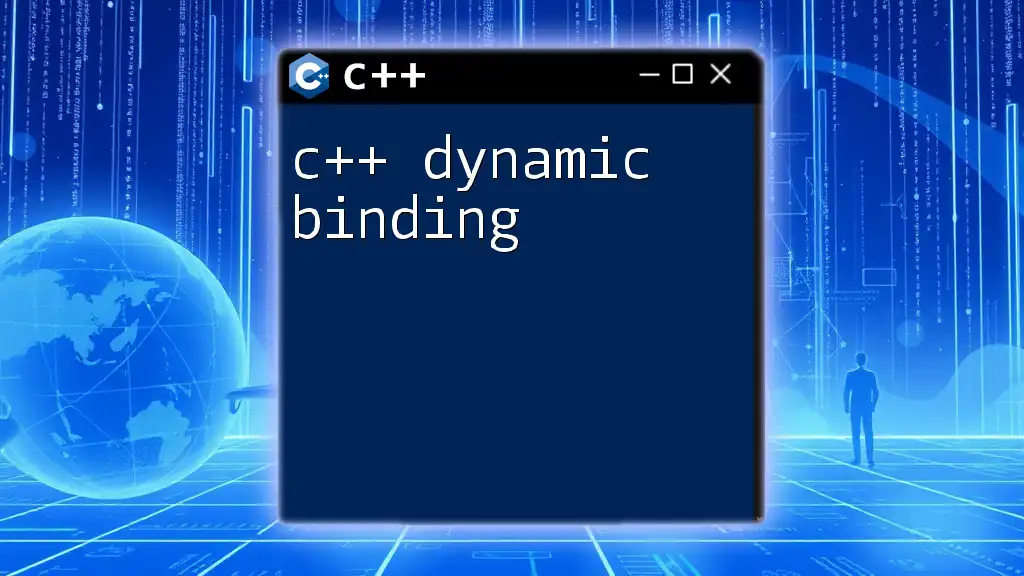
Best Practices for Using Dynamic Arrays
Avoiding Memory Leaks
To avoid memory leaks, ensure that every `new` operation has a corresponding `delete`. Utilize smart pointers whenever feasible, as they automatically manage memory for you.
Using Smart Pointers
In modern C++, using smart pointers like `std::unique_ptr` and `std::shared_ptr` makes memory management simpler and safer. Smart pointers automatically deallocate memory when they go out of scope, reducing the risk of memory leaks. For example:
std::unique_ptr<int[]> arr(new int[size]); // Creating a dynamic array with unique_ptr
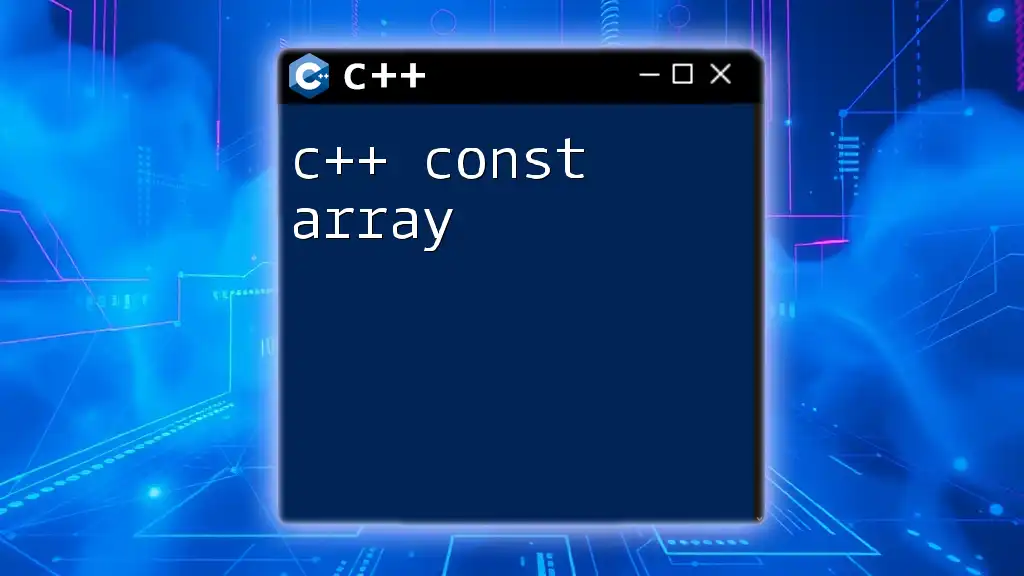
Advanced Topics in C++ Dynamic Arrays
Dynamic Arrays of Objects
It’s also possible to create dynamic arrays of user-defined objects in C++. Here is an example:
class MyClass {
public:
int data;
MyClass(int val) : data(val) {}
};
MyClass* objArr = new MyClass[size]; // Allocating dynamic array of objects
In this case, `size` determines how many `MyClass` objects you allocate, adapting to your program's current needs.
Using Standard Template Library (STL)
While dynamic arrays are useful, C++ provides more user-friendly alternatives, such as `std::vector`. Vectors automatically manage memory, resizing as needed, and provide numerous benefits over traditional dynamic arrays. For instance:
#include <vector>
std::vector<int> vec(size); // Creating a vector of integers
Vectors handle resizing and memory management for you, making them a recommended choice for most scenarios.
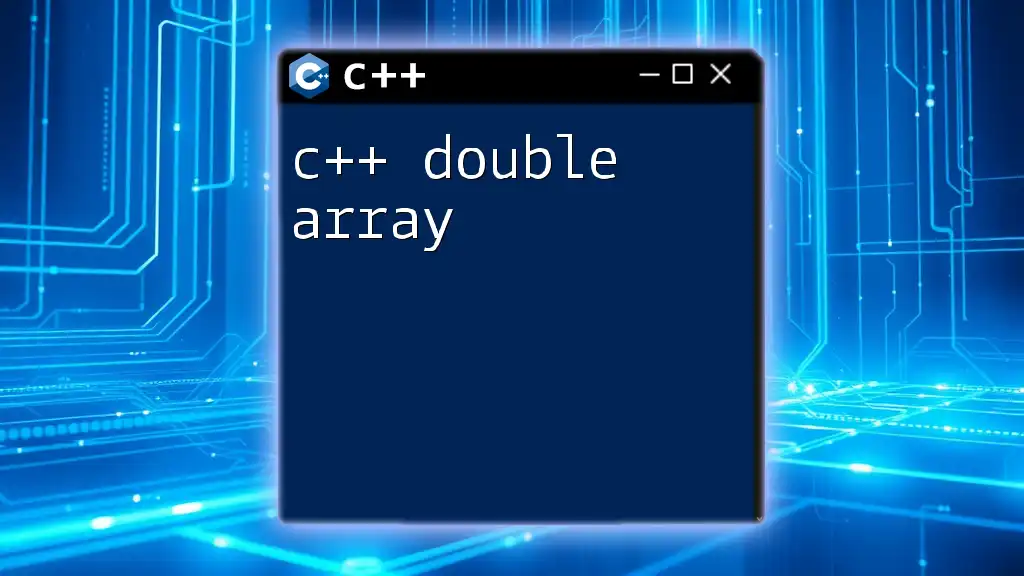
Conclusion
In summary, C++ dynamic arrays empower programmers with the flexibility to manage memory efficiently at runtime. While they offer considerable advantages over static arrays, careful memory management and understanding their use cases are critical in avoiding common pitfalls. By considering smart pointers and STL alternatives, you can enhance your programming experience, creating efficient and reliable software solutions.
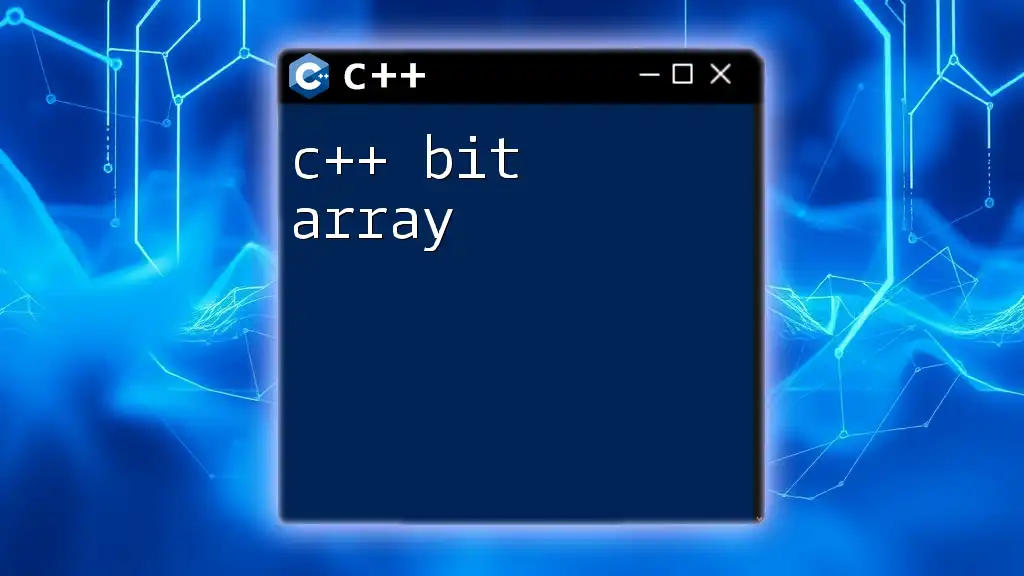
Additional Resources
For those eager to delve deeper into the world of C++ dynamic arrays, numerous tutorials, books, and official documentation options are available. Engaging in hands-on projects and exercises will also solidify your understanding and skills.
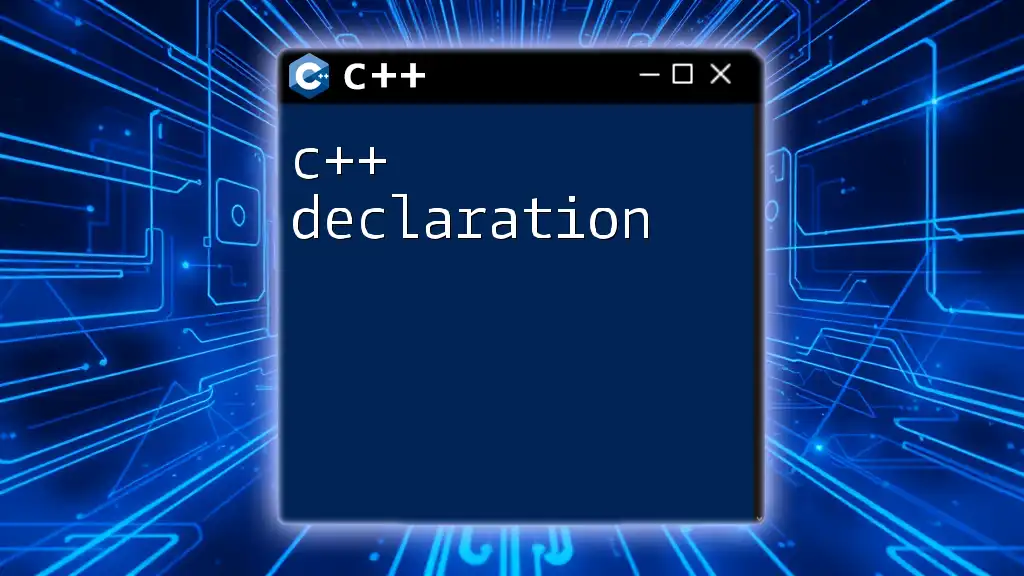
FAQs about C++ Dynamic Arrays
What are the key differences between dynamic arrays and vectors in C++?
Dynamic arrays require manual management of memory, while vectors handle memory allocation and resizing automatically, making them a safer and more convenient option.
Can a dynamic array be resized?
A dynamic array cannot be resized directly; instead, you must allocate a new array, copy elements, and deallocate the old one.
How do I handle memory leaks with dynamic arrays?
To minimize memory leaks, always pair every `new` allocation with a `delete` operation. Consider using smart pointers for automatic memory management.
When should I use a dynamic array over other data structures?
Use dynamic arrays when you need an array structure with flexible size that cannot be determined at compile time, but consider using `std::vector` for most applications due to its added safety and convenience features.