A C++ double array, also known as a two-dimensional array, allows you to store and manage data in a tabular format with rows and columns. Here's an example of how to declare and initialize a double array in C++:
#include <iostream>
int main() {
// Declare and initialize a 2D array
int doubleArray[3][4] = {
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12}
};
// Print the array
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 4; j++) {
std::cout << doubleArray[i][j] << " ";
}
std::cout << std::endl;
}
return 0;
}
What is a Double Array in C++?
A double array in C++ is a multi-dimensional array that allows you to store data in a matrix format, where data is organized in rows and columns. This structure is particularly useful when dealing with table-like data, such as matrices or grids. Unlike a single-dimensional array, which stores elements in a linear sequence, a double array can hold a collection of elements categorized by two indices: one for the row and one for the column.
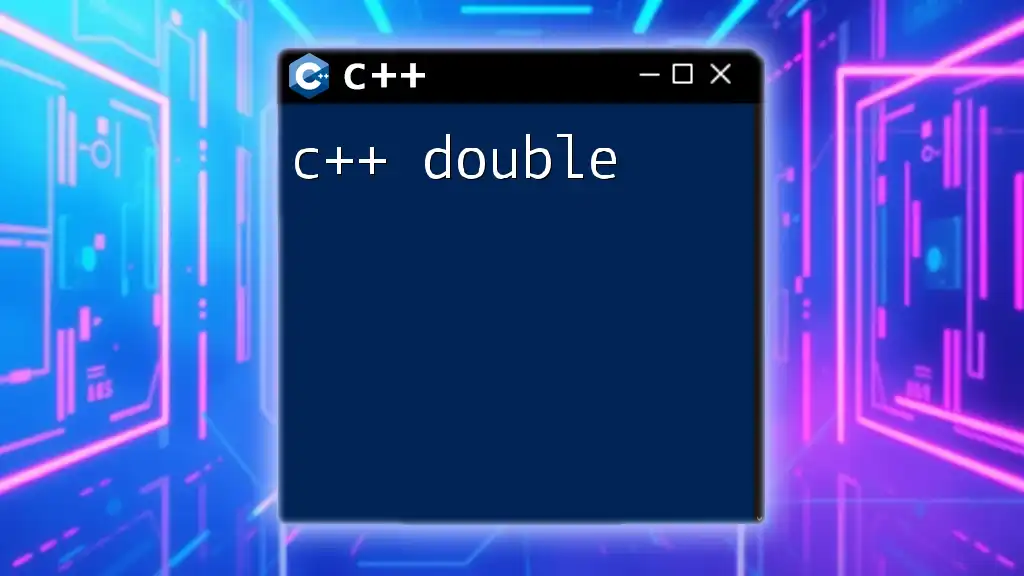
Declaring and Initializing a Double Array in C++
Syntax for Declaring Double Arrays
To declare a double array in C++, the syntax is straightforward. You specify the type of elements (in this case, `double`) followed by the name of the array and the dimensions enclosed in brackets.
double arrayName[rows][columns];
For example, to declare a double array named `mat` with 3 rows and 4 columns, you would write:
double mat[3][4];
Initialization of Double Arrays
Initializing a double array can be done at the time of declaration. This can be achieved in several ways:
-
Static Initialization: You can assign values in a structured manner.
double numbers[2][3] = { {1.1, 2.2, 3.3}, {4.4, 5.5, 6.6} };
-
Default Initialization: If no values are provided, all elements will default to zero.
double zeros[3][2] = { 0 };
This initialization not only helps in memory management but also lays down a clear model for how your data is structured from the get-go.
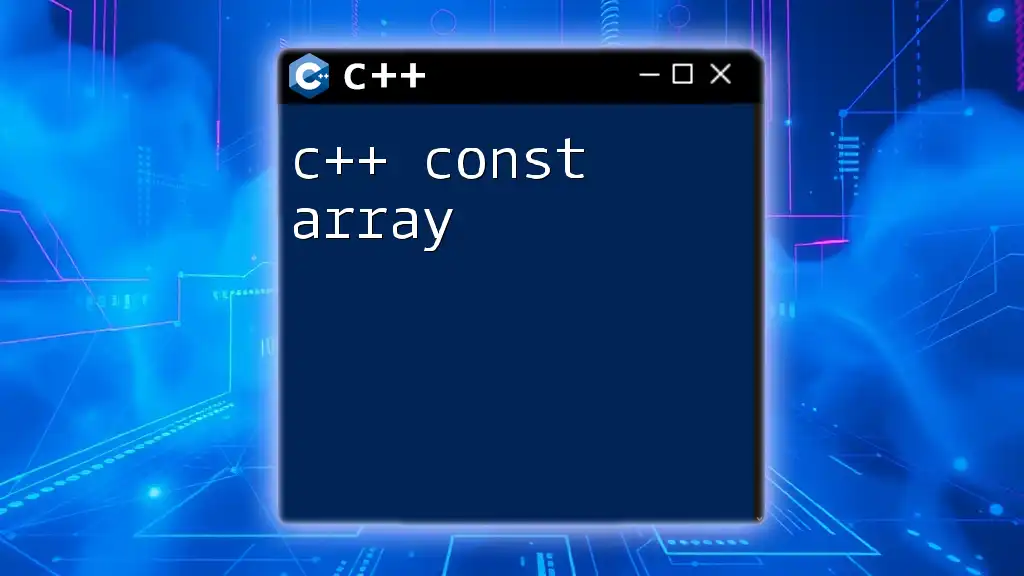
Accessing and Modifying Double Arrays
Accessing Elements in a Double Array
To access elements within a double array, you utilize two indices: one for the row and one for the column. The syntax is as follows:
double value = arrayName[rowIndex][columnIndex];
For example, to access the element in the second row and first column of the `numbers` array declared earlier, the code would be:
double value = numbers[1][0]; // Accessing the value 4.4
Modifying Elements in a Double Array
Modifying elements in a double array follows a similar approach. You can assign a new value to a specific element using its indices:
numbers[0][2] = 7.7; // Updating the first row, third column to 7.7
It’s crucial to remember that in C++, arrays are reference types. This means that when you pass an array to a function, the function can modify the original array.
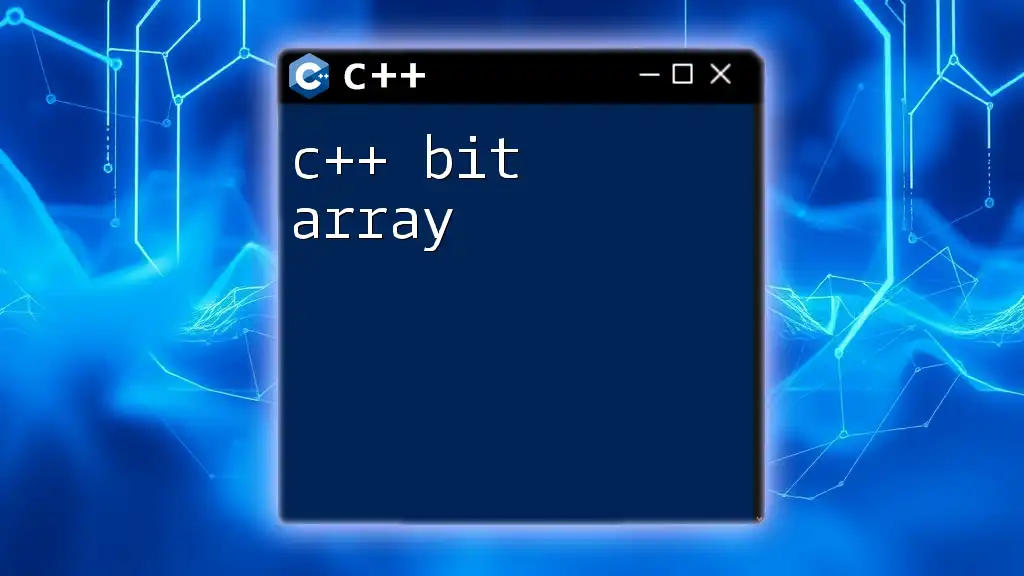
Common Operations on Double Arrays
Iterating Through Double Arrays
To perform operations like displaying or modifying each element of a c++ double array, you typically employ nested loops. The outer loop goes through each row, while the inner loop traverses through each column:
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 3; j++) {
std::cout << numbers[i][j] << " ";
}
}
With this code, you can print all the elements of the `numbers` array in a structured format, allowing you to visualize how the data is organized.
Summing Elements of a Double Array
Summing all the elements in a double array can also be quickly achieved by using nested loops to traverse it:
double sum = 0.0;
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 3; j++) {
sum += numbers[i][j];
}
}
std::cout << "Total Sum: " << sum;
This code snippet finds the sum of all numbers in the `numbers` array, showcasing how easily you can manipulate data stored in an array structure.
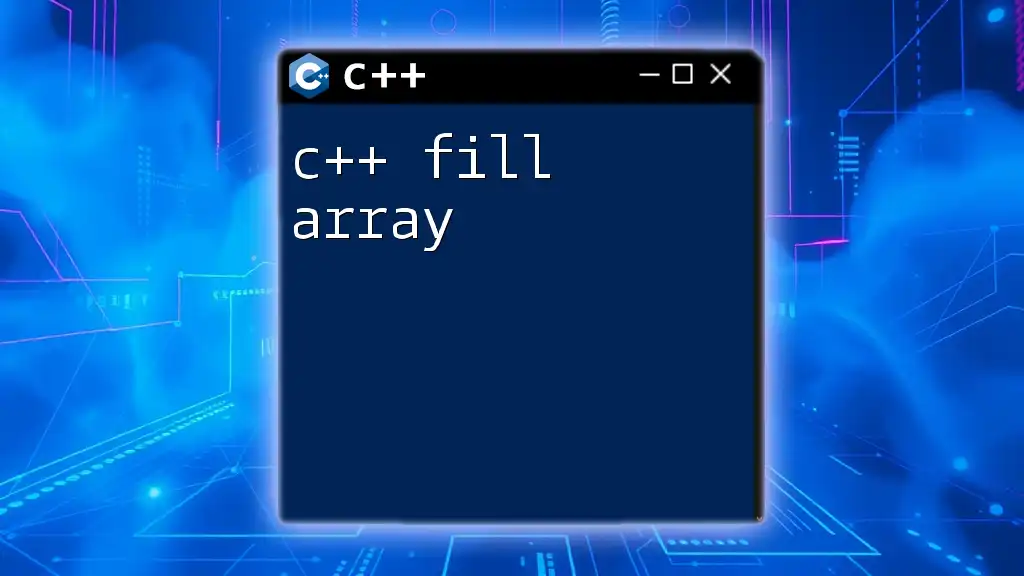
Advanced Topics in Double Arrays
Dynamic Allocation of Double Arrays
In certain situations, the size of your array may not be known at compile time. For such cases, dynamic allocation lets you create double arrays using pointers and the `new` keyword:
double** dynamicArray = new double*[rows];
for (int i = 0; i < rows; i++) {
dynamicArray[i] = new double[columns];
}
Remember to delete any allocated memory to avoid memory leaks:
for (int i = 0; i < rows; i++) {
delete[] dynamicArray[i];
}
delete[] dynamicArray;
This method engages with heap memory, allowing greater flexibility compared to stack-allocated arrays.
Using std::vector for 2D Arrays in C++
In modern C++, using `std::vector` simplifies the creation and management of double arrays. Unlike raw arrays, `std::vector` automatically handles memory allocation and deallocation, which minimizes the risk of memory leaks. The syntax looks like this:
#include <vector>
std::vector<std::vector<double>> vec2D(3, std::vector<double>(4));
This code initializes a 2D vector `vec2D` with 3 rows and 4 columns filled with default values of `0.0`. You can then access or modify elements in a manner similar to regular arrays:
vec2D[0][1] = 5.5; // Assigning 5.5 to the first row, second column
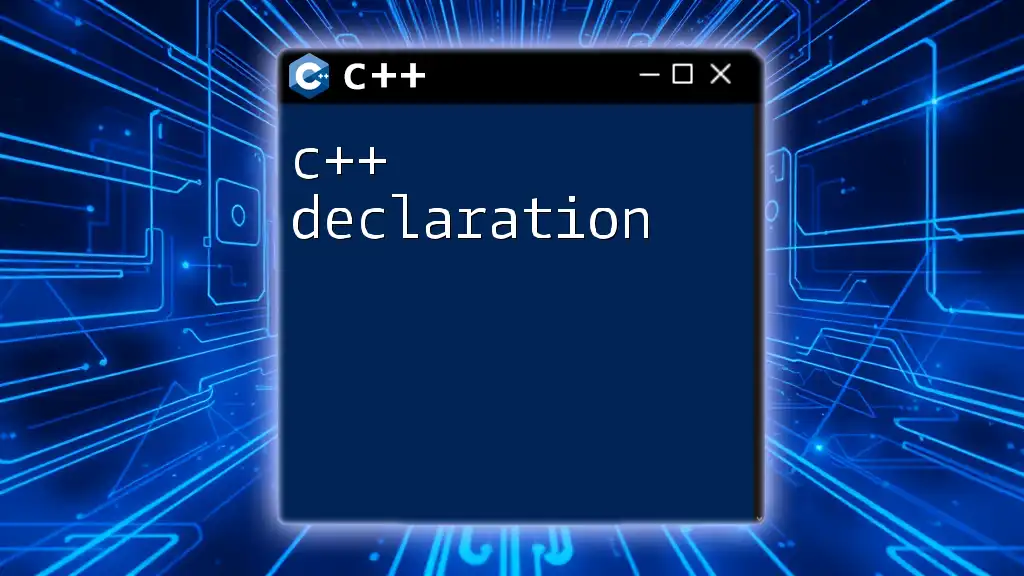
Real-world Applications of Double Arrays
Double arrays have a multitude of practical applications in programming. They are frequently encountered in fields that require handling two-dimensional data, such as:
- Matrices and Linear Algebra: Used in mathematical computations, simulations, and graphics processing.
- Game Development: Storing game state information, such as maps or grids that define spaces, player positions, etc.
- Image Processing: Representing pixels in two-dimensional arrays where each pixel can be defined by its RGB values.
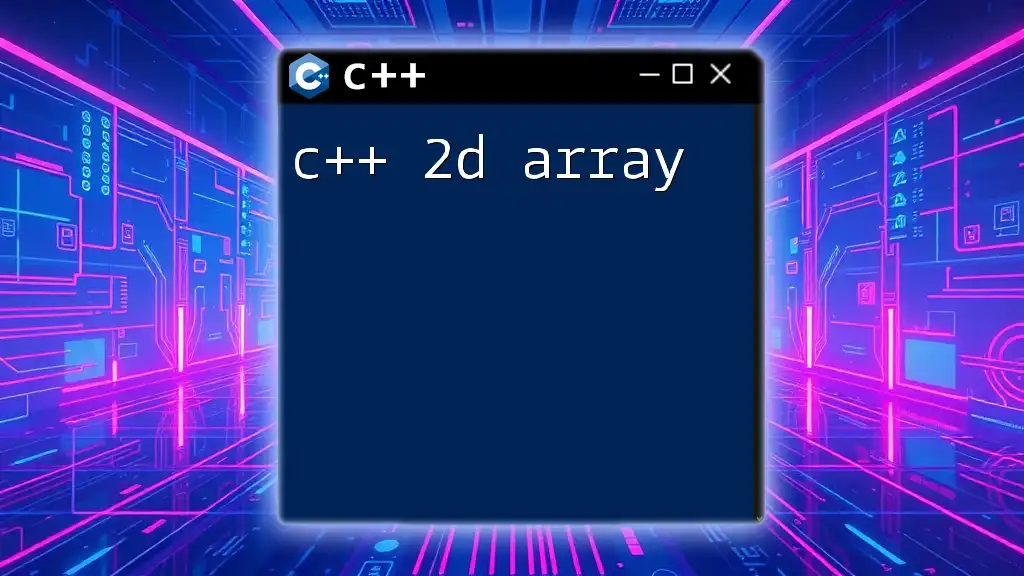
Conclusion
Understanding how to effectively implement and manipulate c++ double arrays equips you with the tools to handle complex data structures with ease. By mastering the definitions, syntax, operations, and advanced topics surrounding double arrays, you can enhance your programming skills significantly. Transition into more complex data structures will be smooth as you gain experience with this fundamental concept.
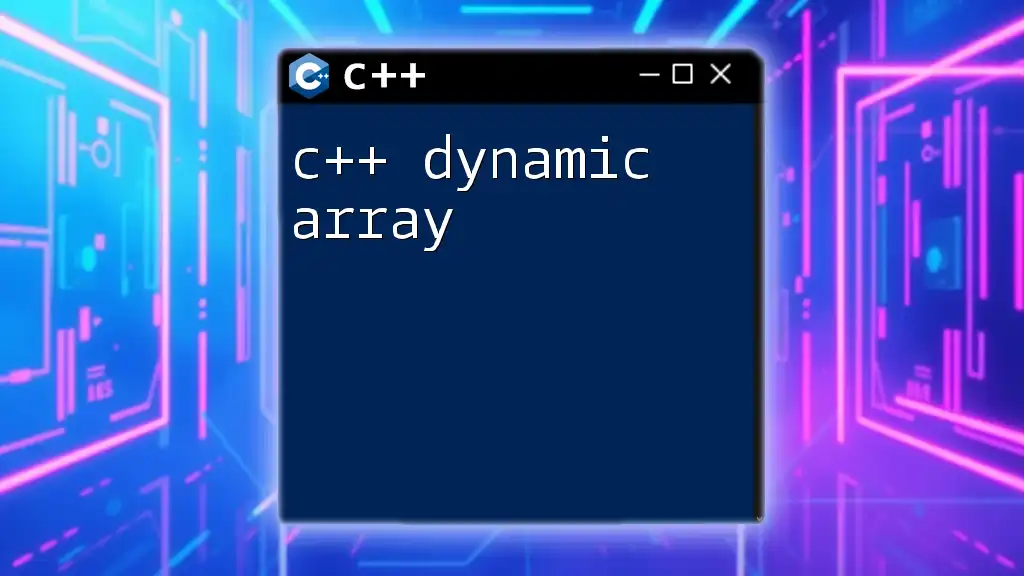
Additional Resources
To further your knowledge, explore more about C++ double arrays through the following resources, which provide in-depth tutorials and examples to broaden your understanding:
- C++ documentation on arrays
- Books focused on C++ programming
- Online courses that dive deeper into data structures and algorithms
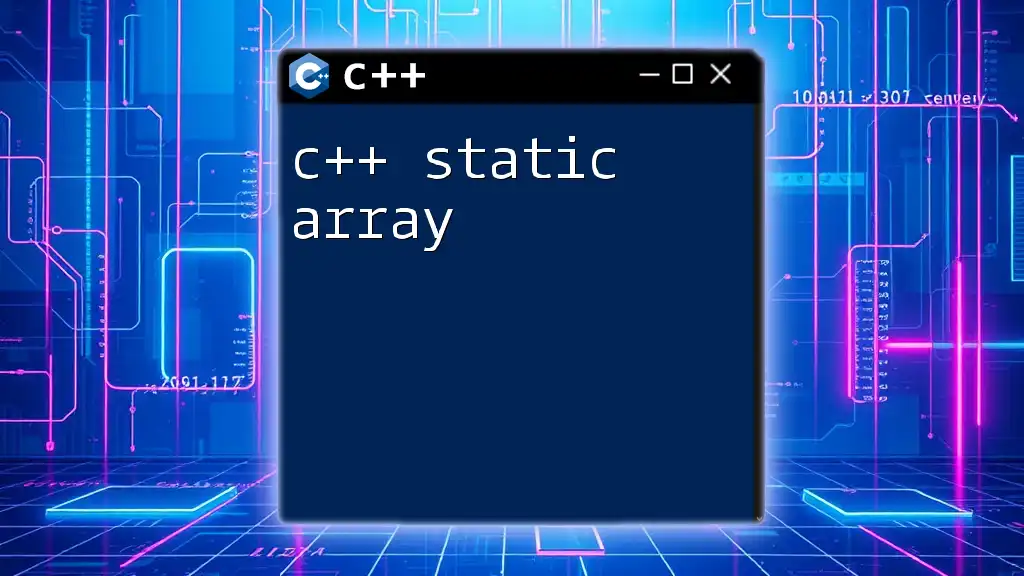
Frequently Asked Questions (FAQs)
In this section, we address common concerns and queries about the concept of double arrays, helping to clarify and reinforce your learning experience.