In C++, a `const` array is an array whose elements cannot be modified after initialization, providing a way to ensure the integrity of the data throughout its scope.
const int myArray[5] = {1, 2, 3, 4, 5};
// myArray[0] = 10; // This line would cause a compilation error since myArray is const.
Understanding C++ Arrays
In C++, an array is a collection of elements of the same type, stored in contiguous memory locations. Using arrays in your programs can lead to significant improvements in performance and memory management. The syntax for declaring an array is as follows:
int myArray[5];
This declaration creates an integer array named `myArray` capable of storing five integer values. Memory is allocated for these values sequentially, which allows for efficient access. One of the primary benefits of using arrays is that they allow you to store multiple values under one variable name, leading to cleaner and more understandable code.

What is a Constant Array in C++?
A constant array, or const array, is an array whose elements cannot be modified after their initialization. The `const` keyword is used to define such arrays, ensuring that their values remain constant throughout the program. This is particularly useful when you have data that you do not want to change accidentally.
Here’s how you can declare a constant array:
const int myConstArray[5] = {1, 2, 3, 4, 5};
In this example, `myConstArray` is declared as a constant array that holds five integer values.
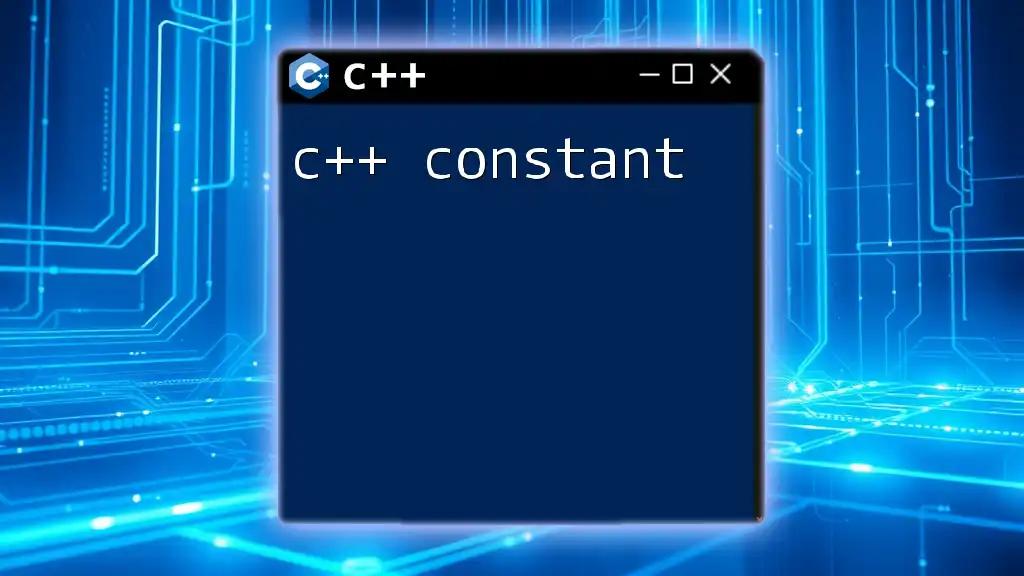
Differences Between Array and Constant Array in C++
Modifiability
One of the most significant differences between a regular array and a constant array is the ability to modify its elements. A regular array can be altered at any time after its declaration:
myArray[0] = 10; // This is allowed
However, if you attempt to change the value of an element in a constant array, it leads to a compilation error:
myConstArray[0] = 10; // This will cause a compilation error
Initialization
Constant arrays must be initialized at the time of declaration. They do not allow for late initialization or modification. For example, you can do this:
const float piValues[3] = {3.14f, 3.14159f, 3.141592f};
In this case, `piValues` is assigned values upon declaration, and these values cannot be modified later in the code.
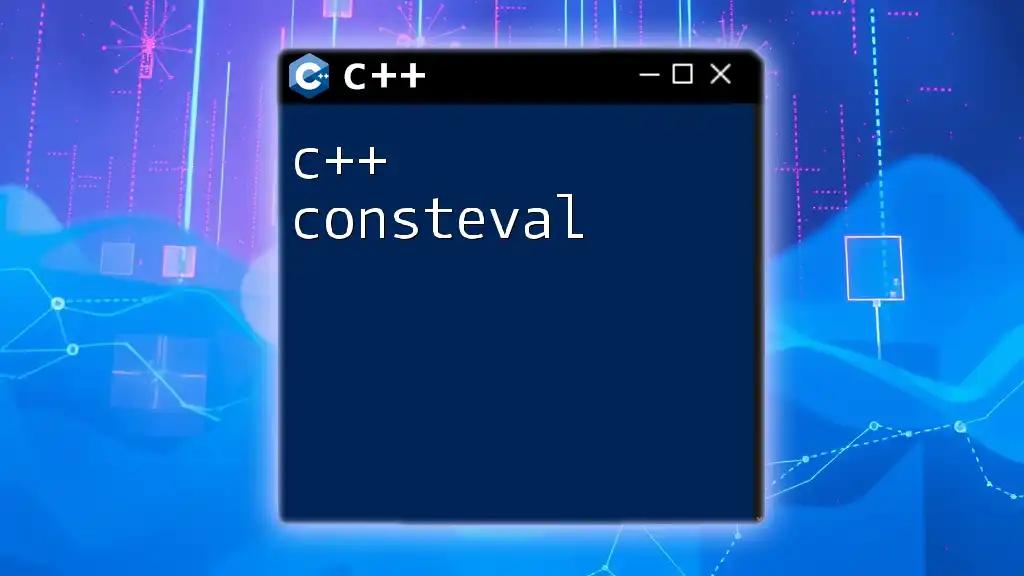
Benefits of Using Constant Arrays in C++
Performance, Safety, Readability, and Optimization are the key benefits of using constant arrays in C++.
- Performance: By knowing that the data in a constant array will not change, compilers can optimize memory usage, leading to better performance.
- Safety: They prevent accidental modifications to data that should remain constant, reducing the risk of bugs.
- Readability: Declaring an array as constant helps communicate your intentions to other developers and anyone who reads your code later.
- Optimization: The impossibility of changes helps compilers make more informed optimizations, which may lead to faster execution times.
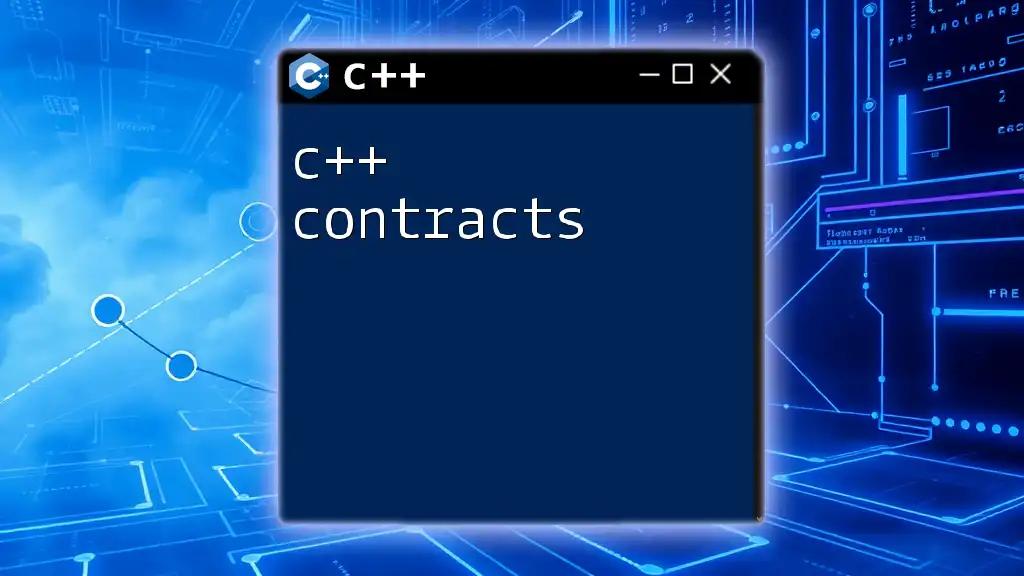
Declaring and Using C++ Const Arrays
Syntax and Declaration
The syntax for declaring constant arrays mirrors that of regular arrays, but includes the `const` keyword. Here’s an example:
const char nameList[3] = {'A', 'B', 'C'};
In this declaration, you create an array called `nameList` that holds three characters.
Accessing Elements
You can access elements of a constant array using the same methods as regular arrays. However, keep in mind that you cannot modify the elements. Here’s how to access an element of `myConstArray`:
cout << "First element: " << myConstArray[0] << endl;
This line of code will output the first element of the constant array without any modifications.
Iterating Over Constant Arrays
When working with constant arrays, you can also iterate through the elements using loops. Here’s an example using a `for` loop:
for (int i = 0; i < 5; ++i) {
cout << myConstArray[i] << " ";
}
This loop will print all elements of `myConstArray`, demonstrating how you can efficiently access all items without changing their values.

Common Use Cases for Constant Arrays
In Functions
Constant arrays can be passed as parameters to functions. This ensures that the function does not modify the original data. Here’s an example of how to declare such a function:
void printArray(const int arr[], int size);
The `const` keyword here indicates that the function promises not to change the elements of the array it receives.
In Constants
Constant arrays are often used for pre-defined configurations or settings in a program. Here’s an example demonstrating this usage:
const string apiKeys[3] = {"key1", "key2", "key3"};
This constant array holds API keys that you can easily reference throughout your program, without any risk of accidental modification.
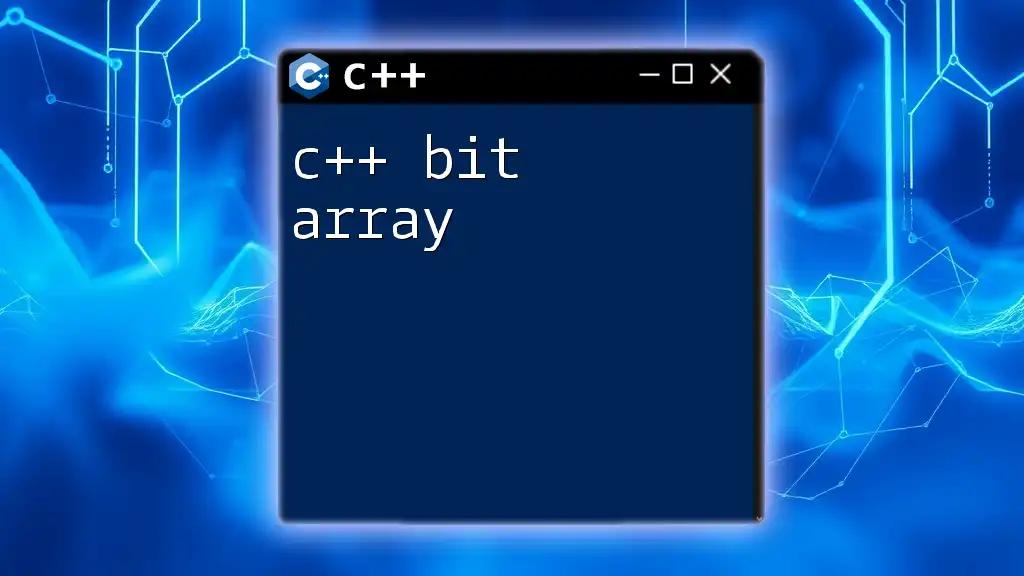
Limitations of Constant Arrays
Despite their many advantages, constant arrays can present specific limitations, especially when you require mutable data. For example, algorithms that depend on modifying the array elements may not work effectively with constant arrays. It’s essential to evaluate your requirements carefully when deciding whether to use a constant array or a regular one.
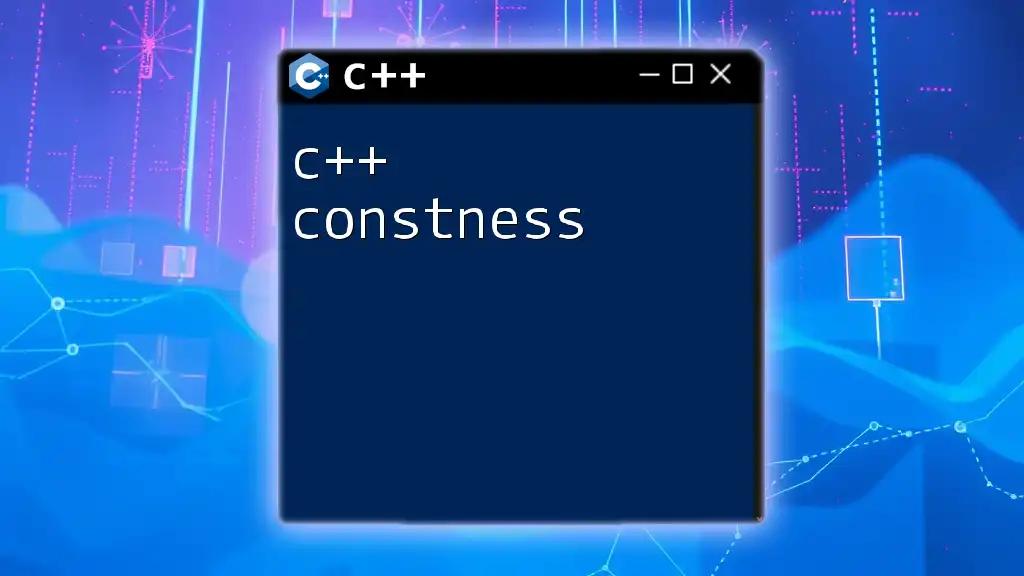
Best Practices for Working with C++ Const Arrays
When working with C++ const arrays, consider the following best practices:
- Naming Conventions: Use clear and descriptive names that indicate the array is constant, such as `kNameList` for a constant array of names. This helps underscore its immutable nature.
- Documentation: Documenting your code, including the purpose and immutability of constant arrays, can guide future developers and your future self in understanding the codebase.
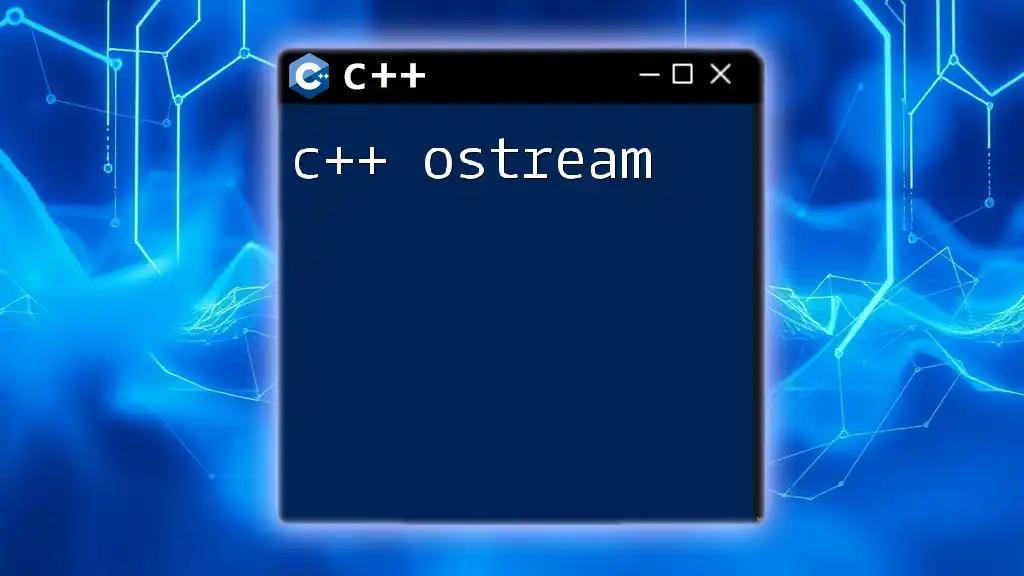
Conclusion
C++ const arrays are a fundamental yet powerful tool in programming, providing numerous benefits such as performance improvement, safety, and clarity in code. By correctly implementing these arrays, you can enhance your coding practices and ensure robust, error-free applications.
Engage with your community to share your experiences, ask questions, and continue mastering C++ commands!