In C++, `consteval` is a keyword that specifies that a function is evaluated at compile time, enforcing its usage in constant expressions.
Here’s a simple example:
consteval int square(int x) {
return x * x;
}
int main() {
constexpr int result = square(5); // evaluated at compile time
}
What is `consteval`?
`consteval` is a keyword introduced in C++20 that signifies a function or variable must be evaluated at compile time. Its primary purpose is to enforce compile-time evaluation, distinguishing it from the `constexpr` keyword, which allows for both compile-time and run-time evaluations. This ensures that any result produced by `consteval` is guaranteed to be calculated when the program is being compiled, as opposed to when it runs.
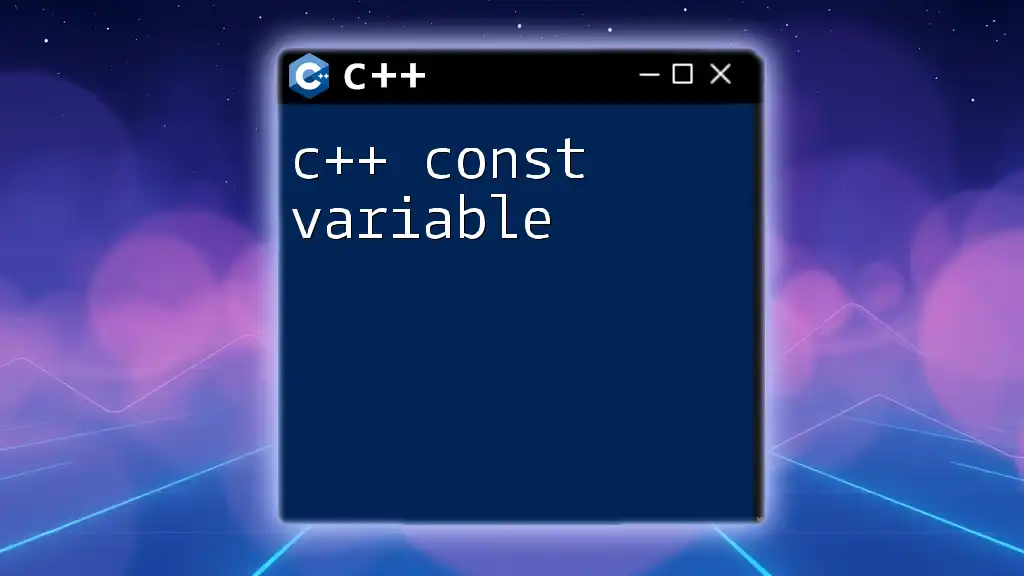
The Purpose of `consteval`
The introduction of `consteval` addresses critical performance requirements in C++ programming. By ensuring that specific expressions are evaluated at compile time, developers can create more efficient code, reducing run-time overhead. This feature is particularly useful in scenarios where immediate results are required, such as defining constants or performing computations that benefit from early evaluation.
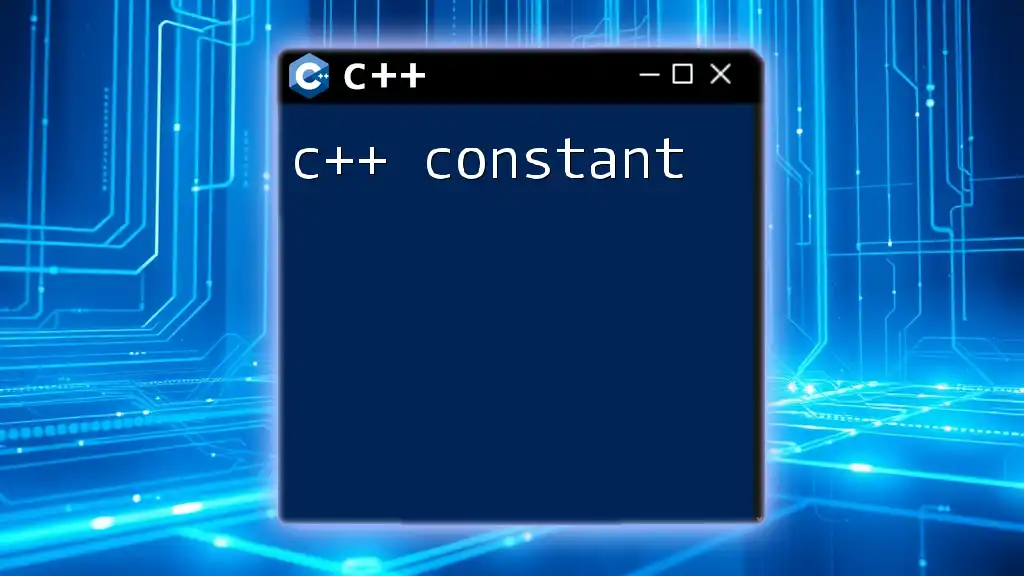
Understanding Compile-Time Evaluation
The Importance of Compile-Time Evaluation
Compile-time evaluation has significant advantages for performance-oriented applications. When computations are performed during compilation, the resultant values can be utilized as constants throughout the code. This reduces the amount of computation that must occur during execution, ultimately leading to a faster program.
Example: Consider a complex mathematical function that needs to be executed multiple times. If the result can be precomputed at compile time, the program can access this precomputed value whenever needed, rather than recalculating it each time.
How `consteval` Works
When a function is declared with `consteval`, the compiler is mandated to compute its result before running the program. This transforms the way constants and values are defined in C++, providing a strong guarantee that any usage of `consteval` must yield an evaluated expression during compilation.
This mechanism allows C++ to be more expressive in defining compile-time constants, which can lead to optimization opportunities and fewer errors related to run-time conditions.
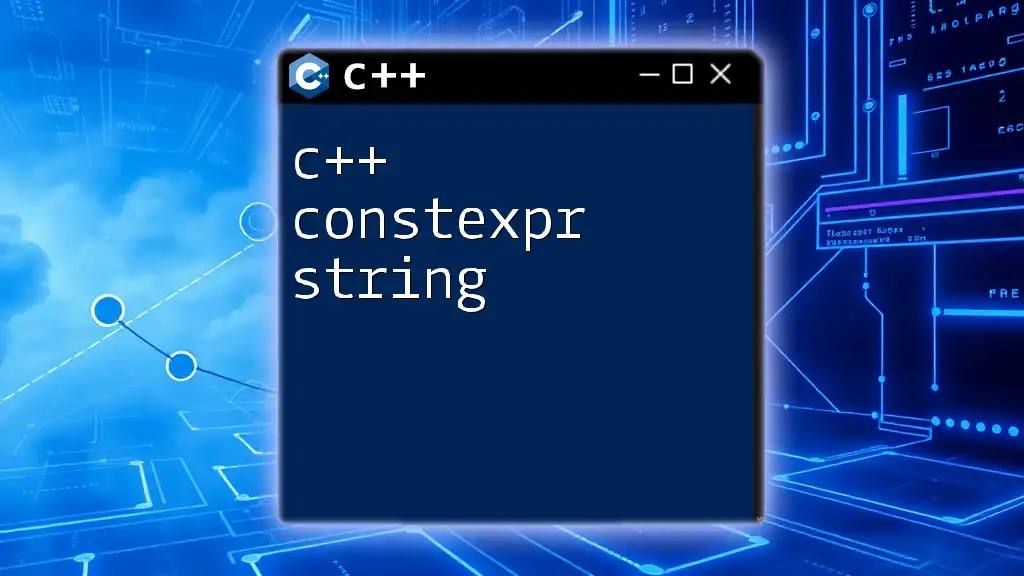
Defining `consteval` in Your Code
Syntax of `consteval`
The `consteval` keyword is straightforward to implement. Here’s a basic example illustrating its usage:
consteval int square(int x) {
return x * x;
}
In this example, `square` computes the square of the value `x`. Since it is defined with `consteval`, any invocation of `square` must provide a constant argument, ensuring that the result is computed during compilation.
When to Use `consteval`
You should use `consteval` when defining values or functions that are inherently constant. Common use cases include:
- Mathematical constants: Complex computations that require validated inputs.
- Fixed sizes: Arrays or data structures that depend on constants.
- Configuration settings: Defaults that should remain unchanged.
Using `consteval` in these situations allows for cleaner, more efficient code, as these values can be trusted to not change, thus enabling optimizations.
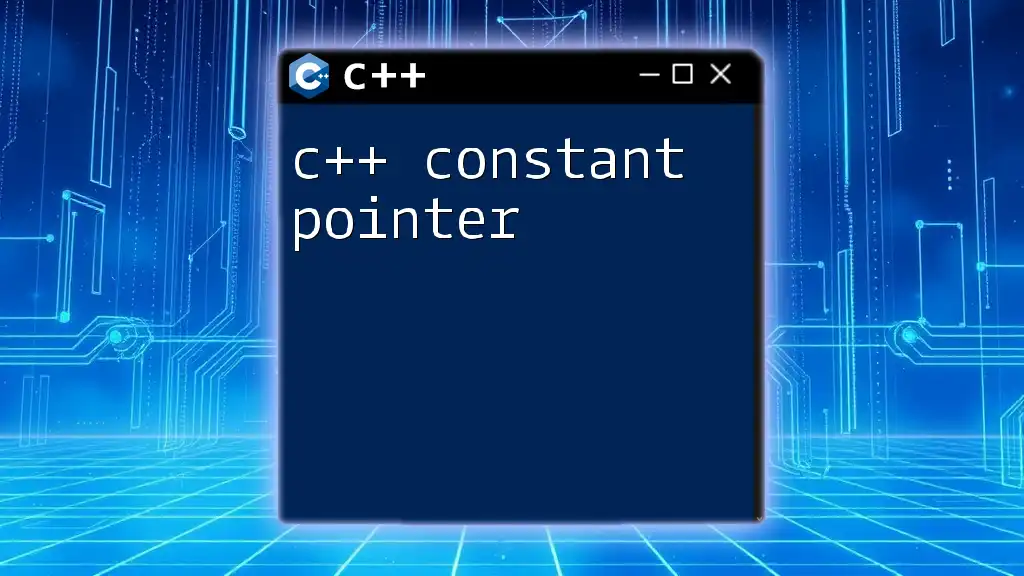
Differences Between `consteval` and `constexpr`
`consteval` vs. `constexpr`: Key Differences
While both `consteval` and `constexpr` promote compile-time computations, there are fundamental differences in how they operate.
-
Evaluation Guarantee:
- `consteval` guarantees that the function's result is strictly computed at compile time, whereas `constexpr` allows functions to be invoked at run-time.
- Example with `constexpr`:
constexpr int cube(int x) { return x * x * x; // Could be evaluated at runtime }
-
Usage Restrictions:
- `consteval` does not allow dynamic arguments or conditions that could evaluate at run-time, while `constexpr` can be used in broader contexts.
- Example with `consteval`:
consteval int cube_consteval(int x) { return x * x * x; // Must be evaluated at compile time }
Practical Implications of These Differences
The implications of these differences stretch into the realm of performance and debugging. Functions defined with `consteval` are typically more efficient since they eliminate all run-time overhead. This rigid structure helps avoid bugs related to unexpected variable states at run-time, thus providing stronger guarantees on the correctness of compile-time computations.
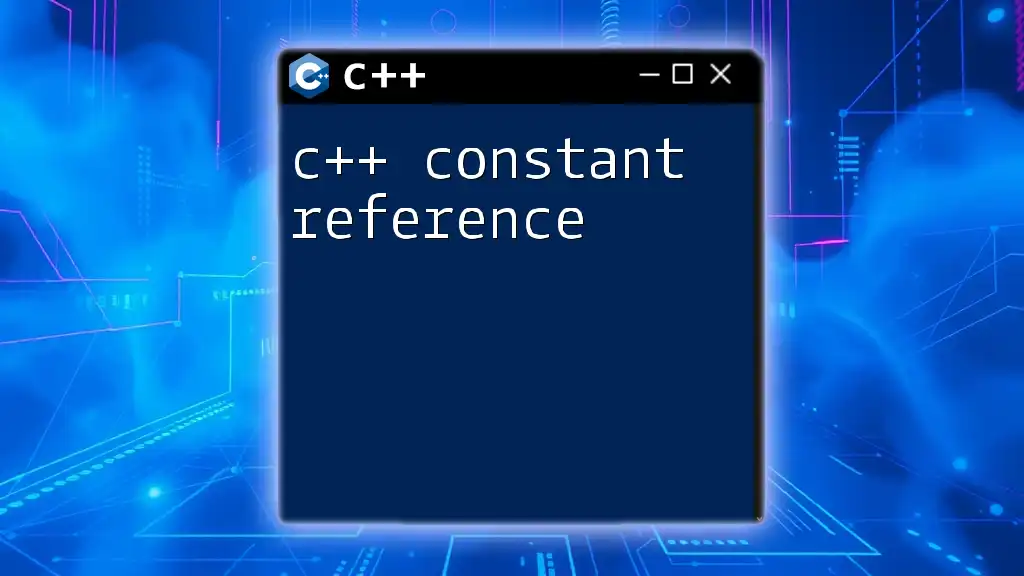
Error Handling in `consteval`
Common Errors with `consteval`
Because `consteval` enforces strict compile-time evaluation, it can lead to various pitfalls for developers. One common error occurs when using conditions that aren't constant expressions. For example:
consteval int badExample(int x) {
if (x > 0) return x; // This is not valid since it depends on runtime condition
}
This function will result in a compile-time error because the condition `x > 0` is not a constant expression, violating the rules of `consteval`.
Debugging Compile-Time Errors
Debugging compile-time errors requires a different approach than run-time issues. To effectively identify problems, consider the following strategies:
- Review compiler error messages carefully, as they provide insights on where the violation occurred.
- Isolate problematic sections of code to see if they comply with the `consteval` rules.
- Leverage tools and utilities that can assist you in analyzing compile-time evaluations.
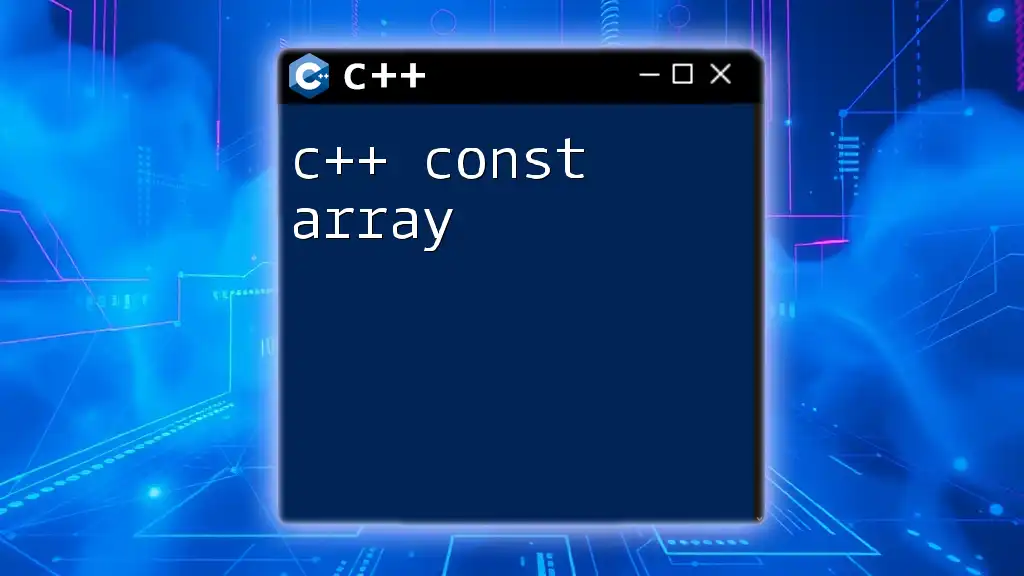
Practical Examples of Using `consteval`
Example 1: Computing Factorials
A classic use case for `consteval` is to compute factorials at compile time. Here’s how it can be implemented:
consteval int factorial(int n) {
return (n == 0) ? 1 : n * factorial(n - 1);
}
In this example, any call to `factorial(n)` requires `n` to be a constant expression, thus guaranteeing that the factorial value is calculated at compile time.
Example 2: Creating Compile-Time Constants
You can also use `consteval` to define constants that need to remain fixed throughout the program's lifecycle:
consteval int getMaxSize() {
return 1024; // Fixed size determined at compile time
}
This method creates a constant returned by `getMaxSize` that can be used wherever needed without incurring run-time costs.
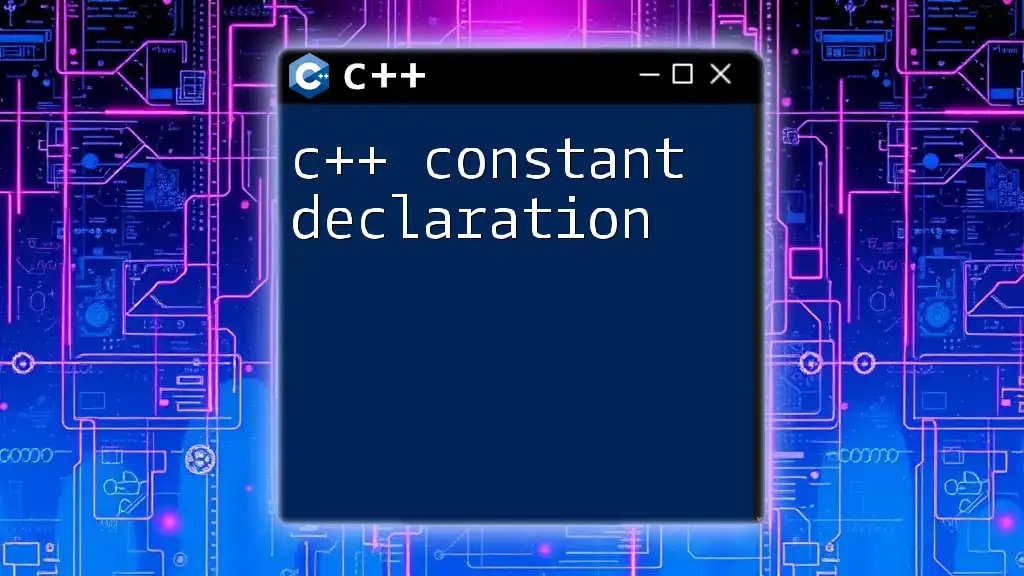
Best Practices for Using `consteval`
Tips for Effective Use of `consteval`
To make the most of `consteval`, consider these best practices:
- Code Organization: Keep your `consteval` functions organized in a way that promotes readability, such as grouping mathematical calculations in one file.
- Combine with Other Features: You can use `consteval` alongside `constexpr` judiciously, capitalizing on their strengths without overcomplicating your design.
Potential Pitfalls to Avoid
While `consteval` can enhance your code’s efficiency, overusing it can lead to complications. Be wary of applying `consteval` in scenarios where run-time evaluation is required or beneficial. Additionally, recognize that `consteval` cannot accept dynamic inputs, leading to compilation errors if used incorrectly.
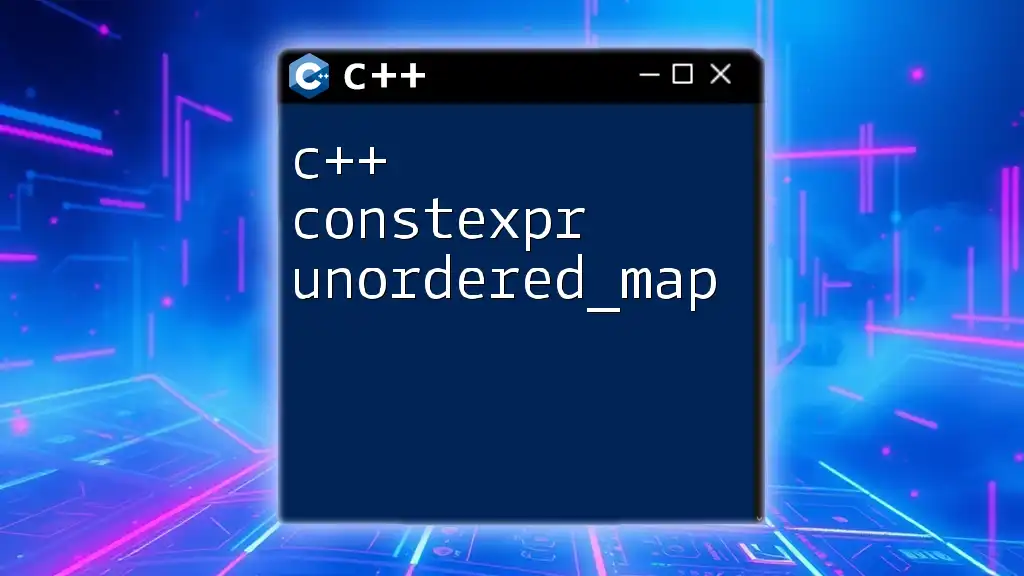
Conclusion
The `c++ consteval` keyword is a powerful addition to the language, enabling developers to enforce compile-time evaluation of functions and values. By ensuring that certain computations are done during compilation, it significantly contributes to performance optimization and error reduction in C++ programs. As C++ continues to evolve, understanding and implementing `consteval` allows you to write more efficient and reliable code.
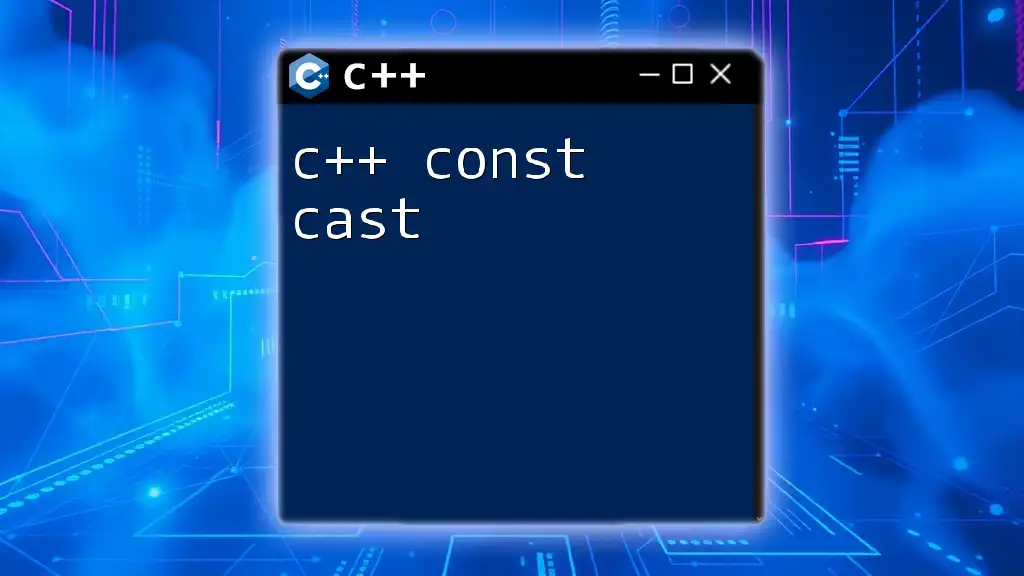
Additional Resources
For deeper exploration into `consteval` and its applications, consider the following resources:
- Books on modern C++ programming that cover features from C++20 and beyond.
- Online forums and communities dedicated to C++, providing a platform for discussion and support as you learn.