C++ in Unreal Engine is used to create gameplay functionalities and interactions, allowing developers to leverage the powerful features of the engine for high-performance game development.
Here’s a simple example of a C++ class in Unreal Engine that defines a basic actor:
#include "GameFramework/Actor.h"
#include "MyActor.generated.h"
UCLASS()
class MYPROJECT_API AMyActor : public AActor
{
GENERATED_BODY()
public:
AMyActor();
protected:
virtual void BeginPlay() override;
public:
virtual void Tick(float DeltaTime) override;
};
Introduction to C++ in Unreal Engine
What is Unreal Engine?
Unreal Engine is a powerful and widely used game engine developed by Epic Games. It allows developers to create high-quality 3D games and experiences, and it comes with a robust set of tools, including an advanced editor, asset management, and a powerful rendering engine. Unreal Engine 4 and its latest iteration, Unreal Engine 5, support both visual scripting through Blueprints and programming using C++, making it a versatile choice for developers at all experience levels.
Why Choose C++ for Game Development?
Using C++ in Unreal Engine offers several significant advantages.
- Performance advantages: C++ is known for its speed and efficiency, leading to better performance in memory management and execution time.
- Versatility and control: C++ provides developers with granular control over game mechanics and system resources, enabling them to optimize their games for specific hardware.
- Access to Unreal Engine's advanced features: Many of Unreal Engine's powerful features are exposed through C++, allowing for deeper integration and customization of gameplay mechanics.
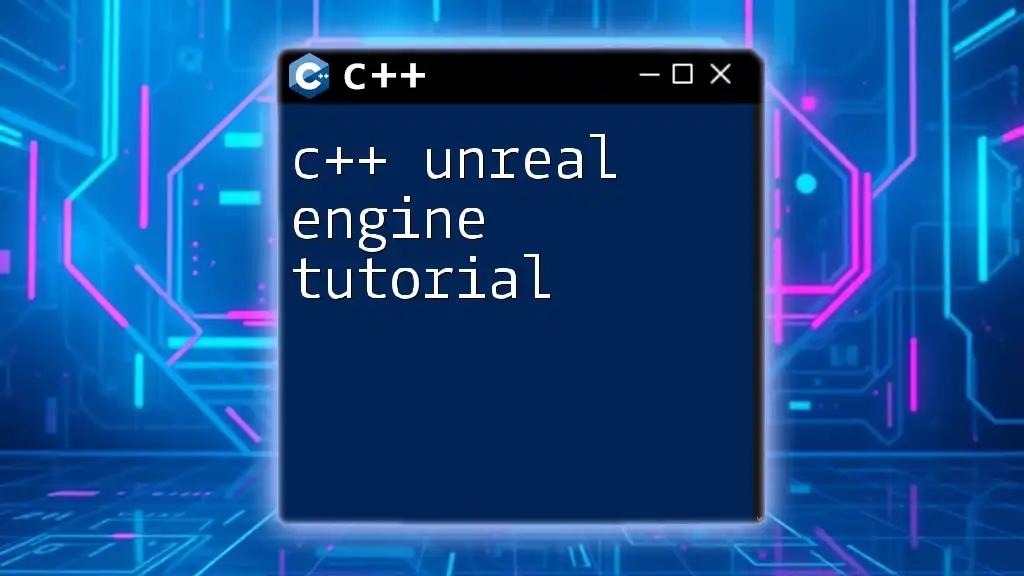
Getting Started with Unreal Engine and C++
Setting Up Your Development Environment
To get started with C++ in Unreal Engine, you'll need to set up your development environment properly.
- Required software and tools: Before you begin, ensure that you have the latest version of Unreal Engine installed alongside Visual Studio, which is the recommended IDE for C++ development in Unreal.
- Creating your first project: Launch Unreal Engine, select "New Project," choose "Games," and select a template that suits your needs. Ensure you select C++ as the project type and give your project a name.
Basic Concepts of C++ in Unreal
To effectively utilize C++ in Unreal, it's crucial to understand its foundational concepts.
- Overview of classes and objects: C++ uses classes as blueprints for creating objects. Each class can contain data members (variables) and member functions (methods) that define the behavior and attributes of the objects.
- Understanding Blueprint vs C++: Blueprints offer a visual scripting alternative, which is easier for non-programmers. However, C++ provides greater flexibility, performance, and control, making it essential for complex gameplay mechanics.
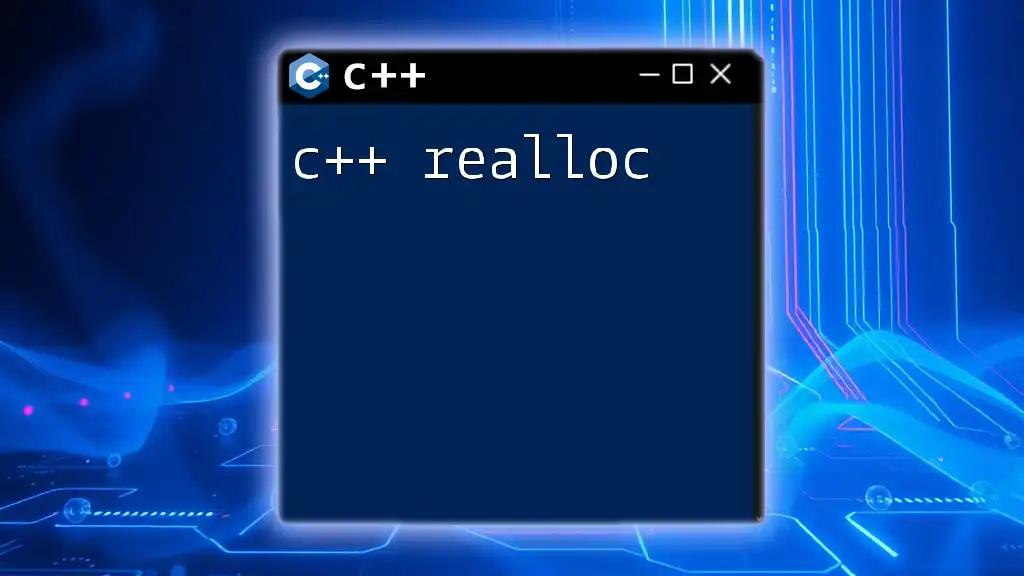
C++ Scripting in Unreal Engine
Understanding UObject and AActor
In Unreal Engine, all classes that you create can derive from `UObject` or `AActor`.
- What is UObject?: `UObject` serves as the base class for most Unreal Engine classes. It handles memory management and serialization.
- What is AActor?: `AActor` is a subclass of `UObject` that represents an object in the game world. It includes properties and methods essential for gameplay elements.
Creating Your First C++ Class
Creating a custom C++ class is straightforward. Here's how to create a simple Actor class:
#include "GameFramework/Actor.h"
#include "MyActor.generated.h"
UCLASS()
class MYPROJECT_API AMyActor : public AActor
{
GENERATED_BODY()
public:
// Sets default values for this actor's properties
AMyActor();
protected:
// Called when the game starts or when spawned
virtual void BeginPlay() override;
public:
// Called every frame
virtual void Tick(float DeltaTime) override;
};
In this example, we declared a new class called `AMyActor`. The `UCLASS()` macro signifies that it's a UObject class, and `GENERATED_BODY()` generates necessary boilerplate code.
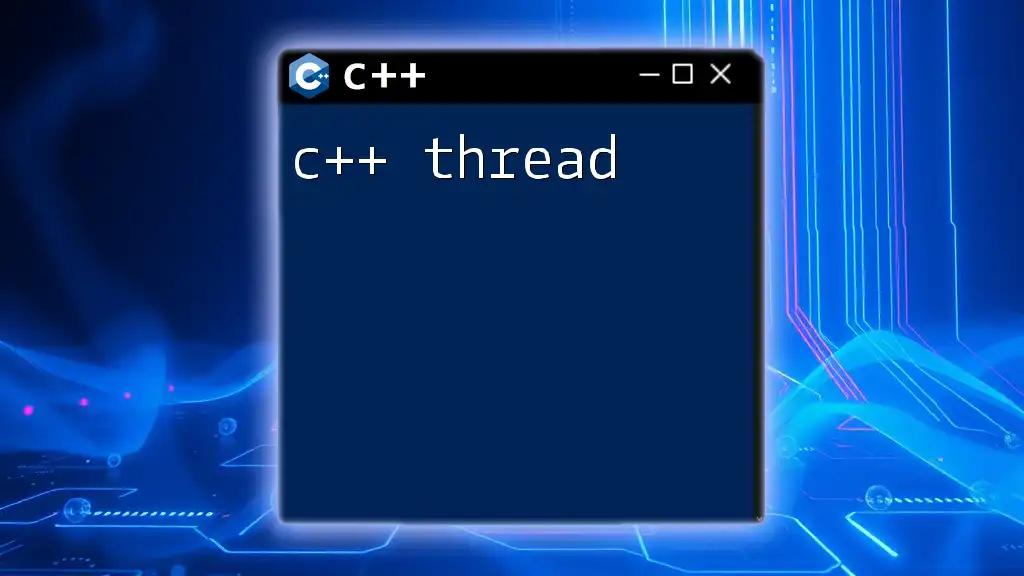
Essential C++ Concepts for Unreal Engine
Properties and Access Specifiers
In Unreal Engine C++, you can define properties using the `UPROPERTY()` macro. This macro also provides various specifier options to control the behavior of these properties.
Functions and Event Handlers
Functions in C++ allow you to define code that can be executed at various points in the game, such as responding to events. You can use the `UFUNCTION()` macro to expose functions to Blueprints. For example:
UFUNCTION(BlueprintCallable, Category="Gameplay")
void MyFunction();
Inheritance and Polymorphism in Unreal C++
C++ supports inheritance, allowing you to create base classes from which other classes can inherit. This is essential for creating complex systems within Unreal. Virtual functions enable you to override behavior in derived classes, promoting code reuse and flexibility.
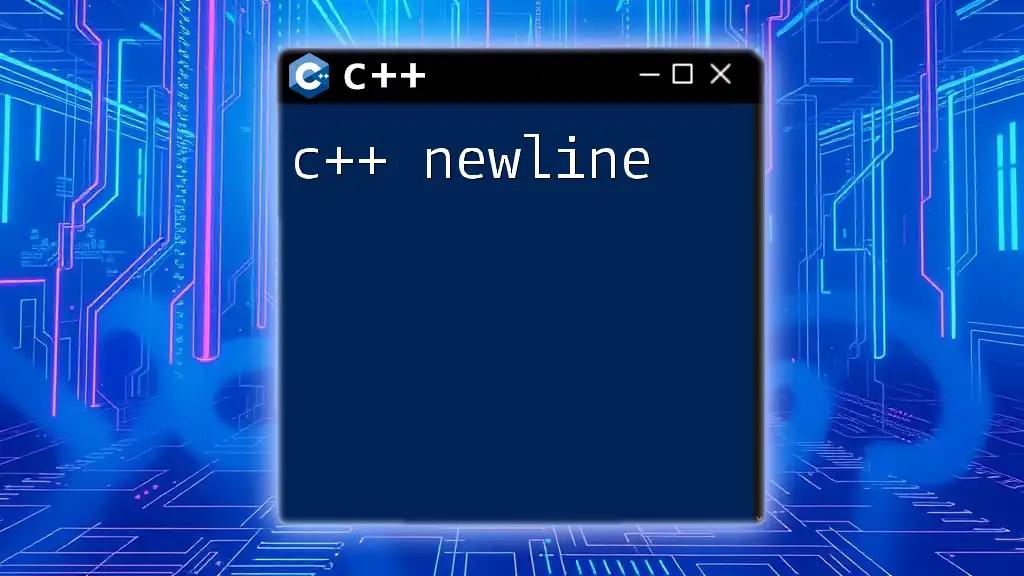
Working with Unreal Engine APIs
Introduction to Unreal Engine APIs
Unreal Engine provides a rich set of APIs to interact with game objects, manage scenes, and handle gameplay logic, enabling you to greenlight innovative gameplay features effectively.
Manipulating Game Objects
You can spawn and manage game objects programmatically. For instance, here is how to spawn an actor at runtime:
AMyActor* MyActor = GetWorld()->SpawnActor<AMyActor>(MyActorClass, SpawnLocation, SpawnRotation);
Handling Input in Unreal
Setting up input handling is crucial for responsive gameplay. Configure input bindings in the Project Settings and listen for input in your C++ classes:
void AMyActor::SetupPlayerInputComponent(UInputComponent* PlayerInputComponent)
{
PlayerInputComponent->BindAction("Jump", IE_Pressed, this, &AMyActor::Jump);
}
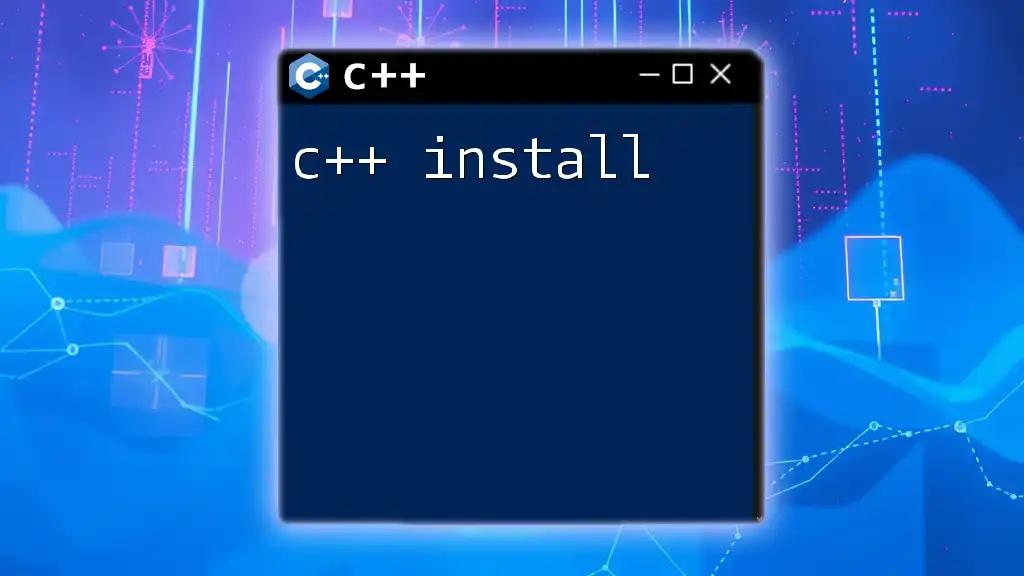
Creating and Managing Game States
Understanding Game States and Game Modes
In Unreal Engine, the Game Mode dictates the rules of the game, while Game State holds information about the current state of the game. Understanding these classes is vital for effective game management.
Implementing a Custom Game Mode
You can create a custom game mode by deriving from `AGameModeBase`. Below is an example of defining your own game mode class:
class MYPROJECT_API AMyGameMode : public AGameModeBase
{
GENERATED_BODY()
public:
AMyGameMode();
};
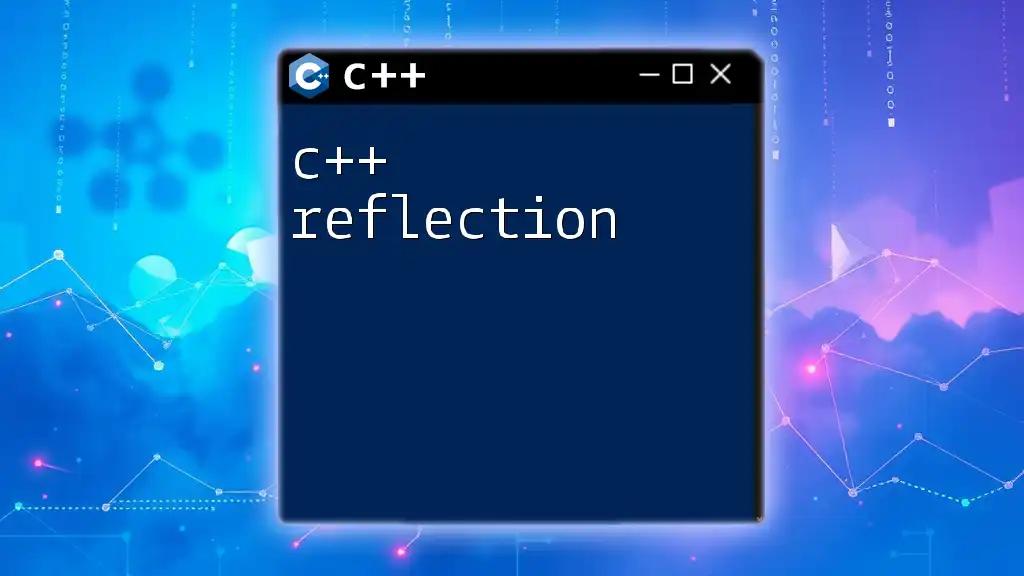
Debugging and Optimizing C++ Code in Unreal
Debugging Techniques
Effective debugging allows you to identify issues in your code efficiently. Utilize breakpoints in Visual Studio to inspect variables and control execution flow. Familiarize yourself with Unreal Engine's debugging tools, such as the Output Log and Visual Studio's debugger.
Performance Optimization Tips
Optimization is key to ensuring your game runs smoothly:
- Profiling your game: Use the built-in profiling tools to analyze performance bottlenecks and identify areas needing improvement.
- Best practices for writing efficient C++ code: Consider memory usage, minimize object creation in frequently called functions, and prefer using raw pointers judiciously.
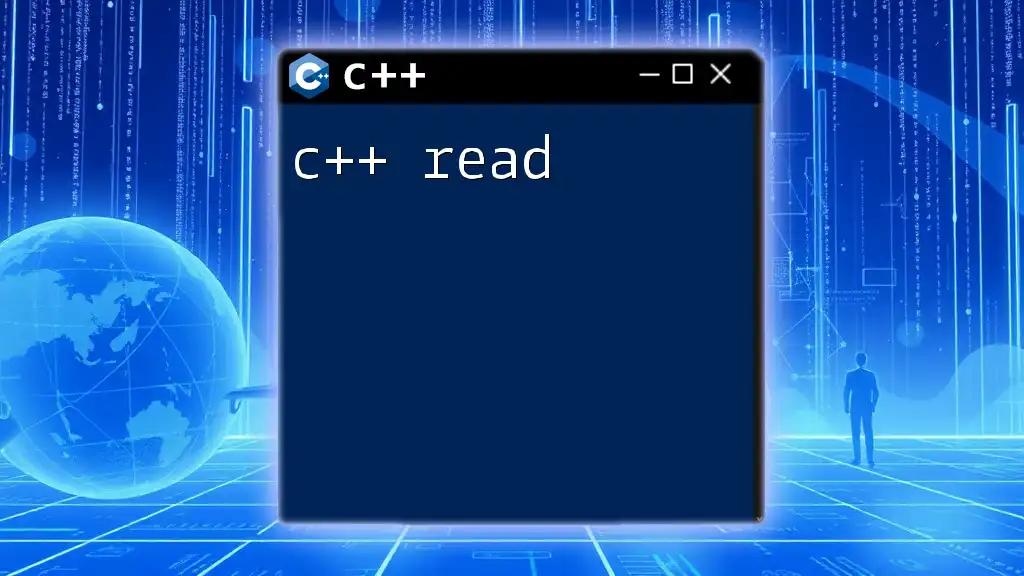
Advanced C++ Concepts in Unreal Engine
Multithreading in Unreal
Multi-threading can enhance performance, particularly for extensive calculations or large-scale simulations by distributing workloads across available processor cores.
Networking and Replication
For multiplayer games, understanding networking concepts and replication is essential. The basics include setting up server-client architecture, managing player connections, and ensuring state consistency across clients.
Using Third-Party Libraries
Integrating third-party libraries can expand the capabilities of your Unreal project. Ensure compatibility and follow proper guidelines for including and linking against external libraries.
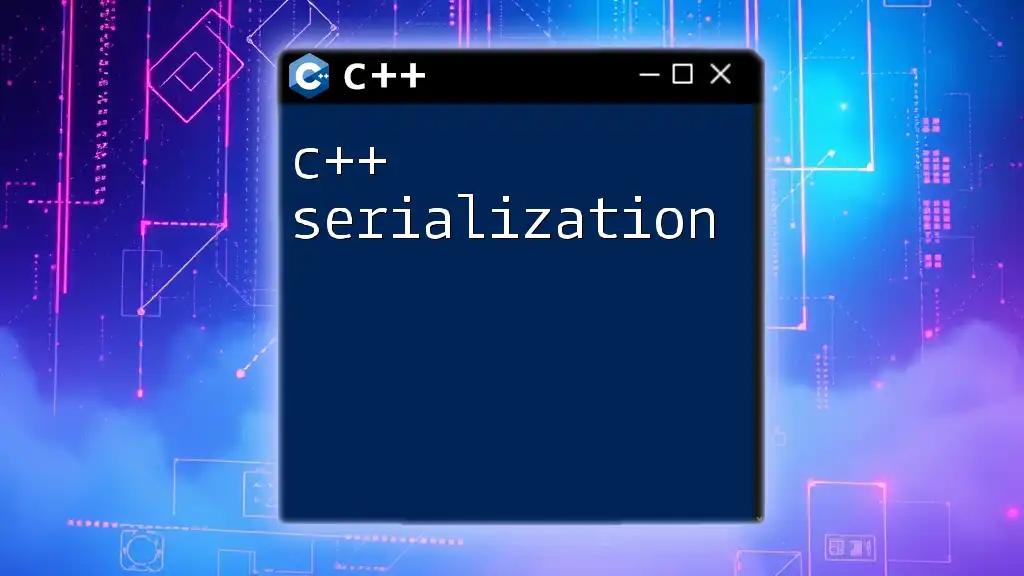
Resources for Learning C++ in Unreal Engine
Official Documentation and Tutorials
Epic Games provides extensive documentation and tutorials to assist developers in mastering Unreal and C++. Utilize these resources to deepen your understanding of the engine's features.
Community Forums and Groups
Join active community forums, such as Unreal Engine forums and Reddit groups, where you can ask questions, share knowledge, and collaborate with other developers who are also learning C++ in Unreal.
Recommended Books and Online Courses
Various books and online courses cover Unreal Engine and C++ specifically. Invest time to find materials that suit your learning style and pace.
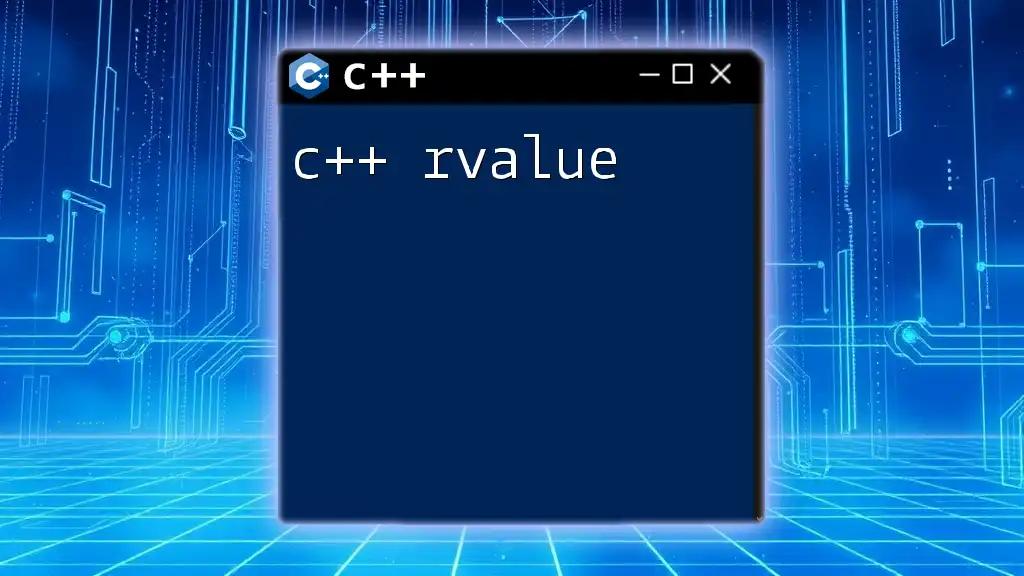
Conclusion
In summary, C++ is an essential language for developers wanting to maximize their skills in Unreal Engine. With its performance advantages and deep access to Unreal's features, becoming proficient in C++ will significantly enhance your game development abilities. Explore the resources mentioned, experiment with your projects, and join our community to take advantage of collective learning and support.