C++ reflection is a feature that allows a program to inspect and modify its own structure and behavior at runtime, enhancing flexibility and dynamism.
#include <iostream>
#include <typeinfo>
class MyClass {
public:
void hello() {
std::cout << "Hello, C++ Reflection!" << std::endl;
}
};
int main() {
MyClass obj;
std::cout << "Class Name: " << typeid(obj).name() << std::endl; // Inspect class type
return 0;
}
Introduction to C++ Reflection
Reflection in programming refers to the ability of a program to examine and modify its own structure and behavior at runtime. In the context of C++ reflection, this concept plays a significant role in enabling dynamic type identification, object serialization, and creating flexible plugin architectures. Understanding how to leverage reflection can empower developers to write more dynamic and adaptable code.
Historical Context of C++ Reflection
Since its inception, C++ has undergone significant evolution, driven by advancements in programming needs and paradigms. Traditionally, C++ offered limited support for reflection, primarily through mechanisms like Run-Time Type Information (RTTI). However, as the demands for more dynamic programming practices grew, so did the need for robust reflection capabilities.
In modern programming, reflection is vital for scenarios like game development, where game engines rely on runtime versatility to handle complex interactions, or in modern software frameworks that require flexible component architectures. The rise of dynamic languages highlighted the capabilities of reflection, pushing C++ to evolve and embrace similar functionalities.
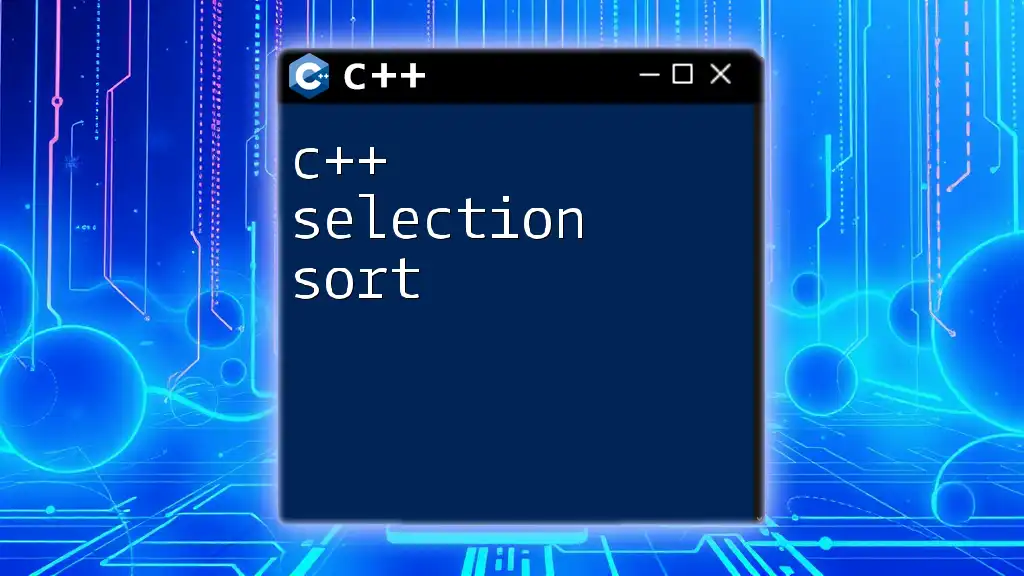
Understanding Reflection in C++
At its core, C++ reflection is about gaining access to type information and properties of classes at runtime. This capability allows developers to manipulate objects and invoke methods dynamically, enhancing the flexibility of their code.
Key Concepts of Reflection
Type Information is a crucial aspect of reflection, enabling programs to retrieve metadata about types, such as their names, attributes, and methods. This discovery is vital for serialization frameworks, testing libraries, and other utilities that rely on understanding object structure without prior compile-time knowledge.
Property Access refers to the ability to read or modify object properties dynamically. This functionality is particularly useful for data-binding applications, where changes to an object should reflect automatically in the user interface.
Method Invocation enables dynamic calls to methods of an object, depending on the context or state of the program at runtime. This is invaluable for implementing command patterns, callbacks, and event handlers in your code.
Common Uses of Reflection
In practice, reflection in C++ can be applied in various scenarios. Dynamic serialization of objects for data storage or network transmission often relies on reflection to convert attributes to a format like JSON or XML without hardcoding field names.
Dependency injection frameworks thrive on reflection as they use it to inject the required dependencies at runtime based on the declared types in class constructors.
Reflection can also simplify plugin architectures by allowing the main application to discover and instantiate plugins dynamically based on type metadata.
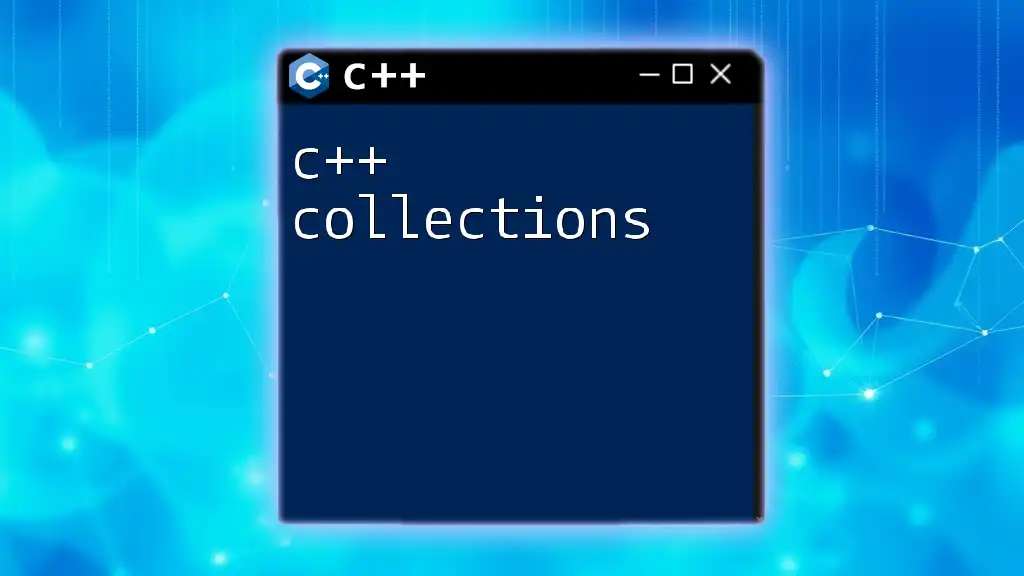
C++ Reflection Mechanisms
C++ offers a few built-in reflection capabilities, but these are limited. The primary built-in mechanism is RTTI, which provides type information through the `typeid` operator and dynamic_cast. However, for more advanced reflection capabilities, developers typically turn to third-party libraries.
Built-in Reflection Capabilities
RTTI allows for basic type identification and safely casting pointer types at runtime, but it doesn't provide comprehensive reflection abilities, such as accessing properties or invoking methods dynamically.
Third-Party Libraries for Reflection
Developers can utilize third-party libraries that offer more robust reflection capabilities:
Boost.Hana
Boost.Hana is a modern C++ metaprogramming library that can facilitate compile-time reflection. By leveraging template metaprogramming, developers can gain access to type information during compilation. This can lead to performance benefits, as reflection is resolved at compile time rather than at runtime.
RTTR (Run-Time Type Reflection)
RTTR is a comprehensive reflection library. It provides extensive functionalities, including property access, method invocation, and type registration. Here’s a basic example demonstrating how to register a class and its property:
#include <rttr/registration>
struct MyStruct {
int value;
};
RTTR_REGISTRATION
{
rttr::registration::class_<MyStruct>("MyStruct")
.property("value", &MyStruct::value);
}
This code snippet illustrates how to define a class with a registered property, allowing for runtime access and manipulation.
magic_enum
The magic_enum library provides a simple way to integrate reflection for C++ enumerations. It allows you to retrieve the names of enum values at runtime, enhancing the usability of enums in applications.
enum class Color { Red, Green, Blue };
std::string color_name = magic_enum::enum_name(Color::Red);
This powerful utility not only simplifies enumerations, but also makes your code cleaner and easier to maintain.
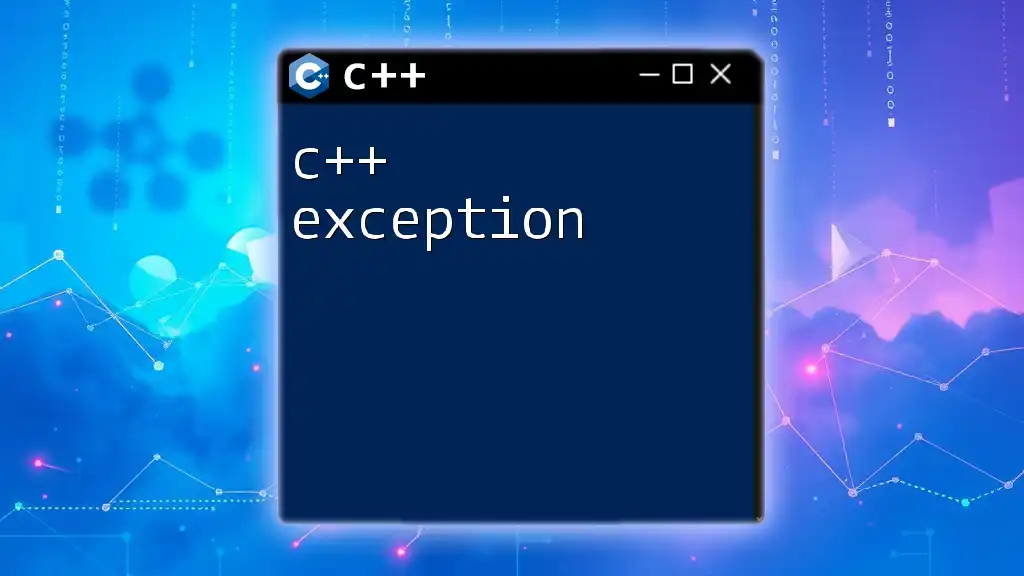
Implementing Reflection in C++
Creating your reflection mechanism in C++ can seem daunting, but with the right approach, it can be achieved elegantly. Using macros can streamline the creation of reflective functionalities.
How to Create a Basic Reflection Mechanism
You can create a simple reflection mechanism using macros to automate metadata retrieval:
#define REFLECT(...) \
static const char* reflect() { return #__VA_ARGS__; }
class Person {
REFLECT(name, age)
std::string name;
int age;
};
In this example, the `REFLECT` macro captures the names of the member variables, making them accessible at runtime through the `reflect()` method.
Example of a Simple Reflective Class
Let’s consider an example where we define a class that utilizes our custom reflection implementation. This class can represent a simple person object.
class Person {
REFLECT(name, age)
std::string name;
int age;
public:
Person(std::string name, int age) : name(name), age(age) {}
};
With this straightforward implementation, you can easily access the metadata of the `Person` class and dynamically manipulate its properties.
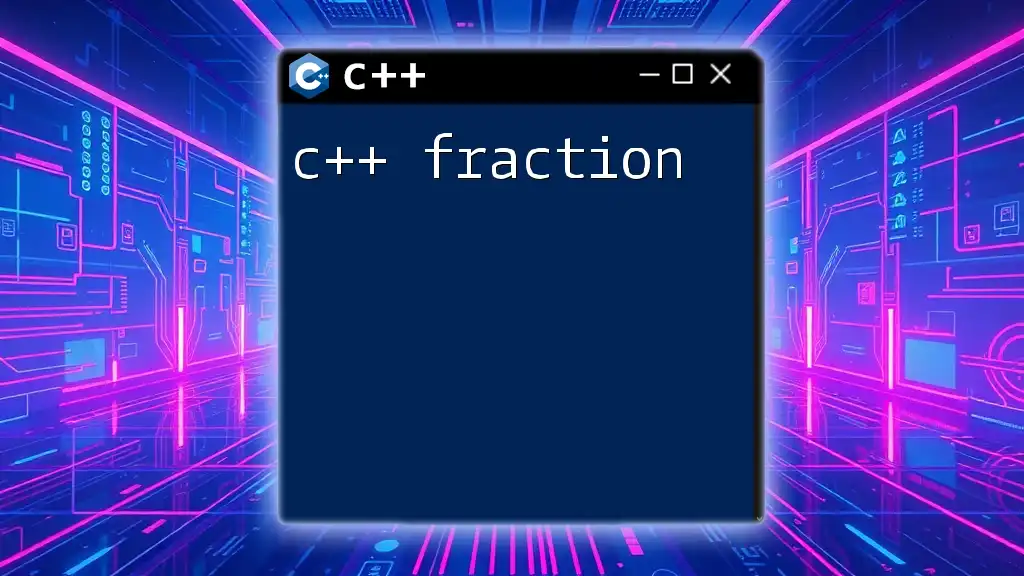
Limitations and Challenges of Reflection in C++
Despite its advantages, C++ reflection comes with limitations. Performance overhead is a significant concern, as reflection operations can be slower compared to direct access, especially in performance-critical applications. Developers must weigh the benefits of dynamic behavior against potential execution speed impacts.
Additionally, the lack of standardization in C++ means that not all compilers support the same reflection capabilities, creating inconsistencies and potential portability issues. As such, developers often rely on external libraries, which can introduce dependencies into projects.
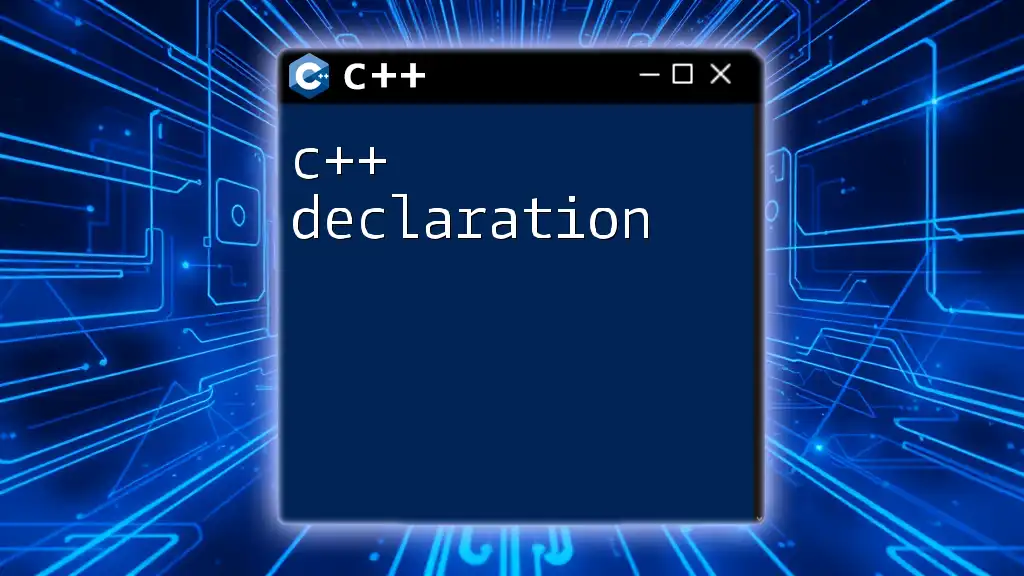
Future of Reflection in C++
The future of C++ reflection looks promising, as proposals for new C++ standards hint at enhanced built-in reflection features. Continuous discussions in the community spotlight the importance of robust and standardized reflection capabilities.
Having reflection built into the language could lead to more uniform and effective applications across different platforms. It’s crucial for developers to remain engaged with ongoing proposals and champion reflection advancements within the C++ community.
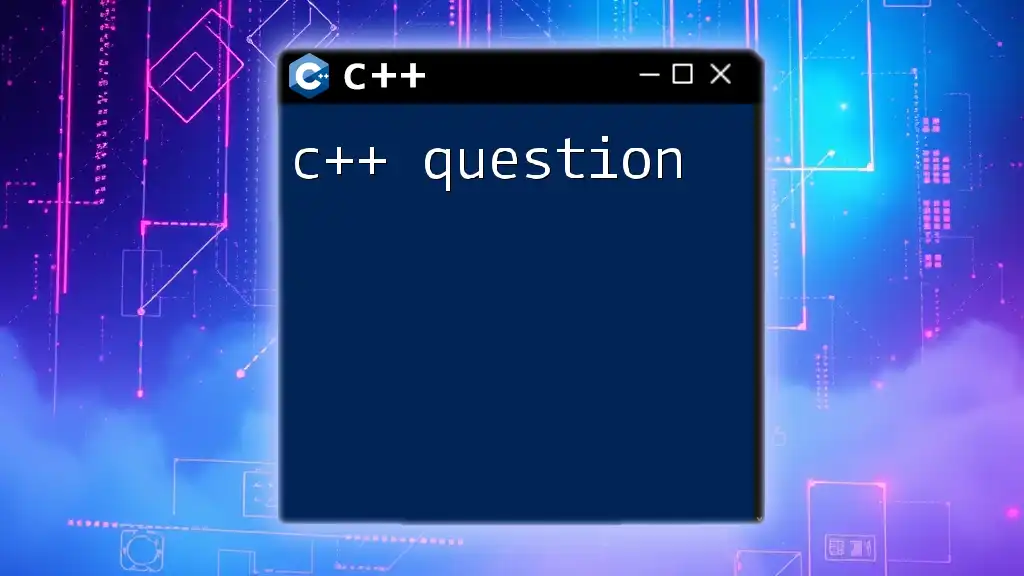
Conclusion
In conclusion, C++ reflection is a powerful tool for developers that broadens the flexibility of their applications. Understanding its mechanisms, limitations, and future potential allows programmers to harness its capabilities effectively.
Experimenting with reflection implementations and exploring third-party libraries can significantly enhance your coding practices. With a focus on maintaining performance while embracing dynamic behavior, reflection stands as a vital component of modern C++.
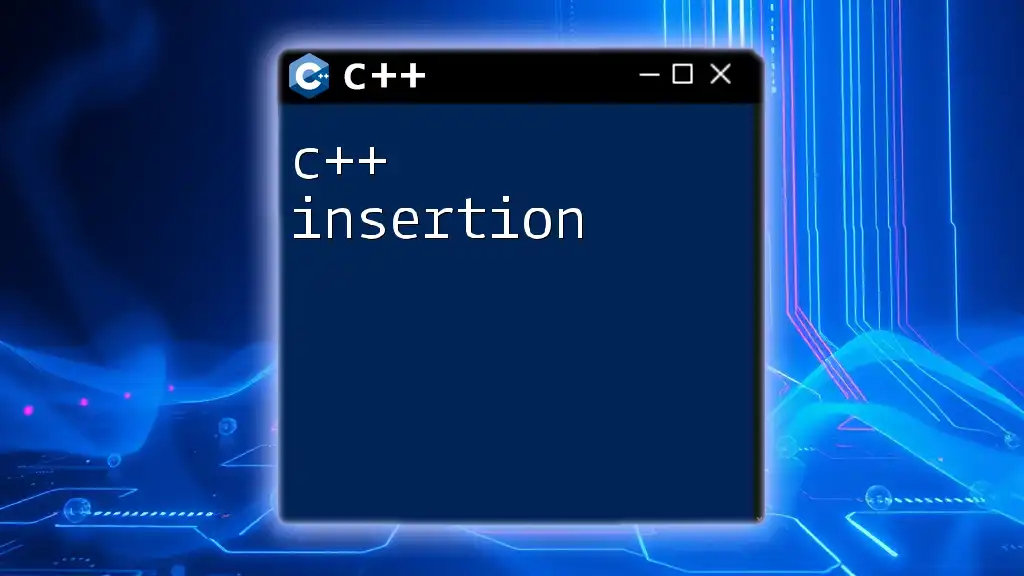
Additional Resources
To further enhance your understanding of C++ reflection, consider diving into recommended books, online articles, and community forums that discuss reflection practices, C++ best practices, and updates in language standards. Engaging with experienced developers in such environments can unlock new insights and techniques.