In C++, `int` is a fundamental data type used to declare variables that hold integer values, which can be either positive or negative whole numbers.
Here’s a code snippet demonstrating the usage of `int` in C++:
#include <iostream>
int main() {
int age = 25; // Declare an integer variable named 'age' and assign it a value
std::cout << "Age: " << age << std::endl; // Output the value of 'age'
return 0;
}
Understanding Data Types in C++
What are Data Types?
In programming, data types define the type of data that can be stored and manipulated within a program. They inform the compiler about how much memory to allocate for a variable and how to interpret the data. Data types play a crucial role in operations—whether you're performing arithmetic, logical comparisons, or storing user input.
Categories of Data Types
C++ categorizes data types mainly into two groups:
- Fundamental types: These are built-in data types, including `int`, `char`, `float`, and `double`.
- User-defined types: These encompass types created by programmers, such as structures, classes, and unions.
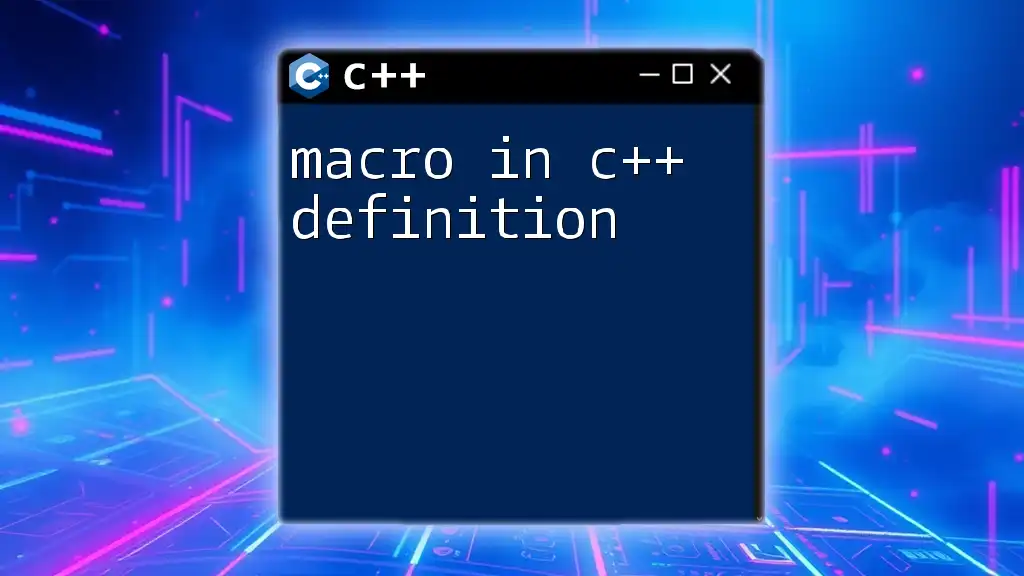
The `int` Data Type
Definition of `int`
The `int` type in C++ is one of the fundamental data types designed to represent integer values. Integers are whole numbers that can be both positive and negative, but they do not accommodate decimal points.
Characteristics of `int`
Size and Range
Typically, the size of an `int` is either 2 bytes or 4 bytes, depending on the system and the compiler used. While the C++ standard doesn’t define the exact range, a common range for a 4-byte `int` is -2,147,483,648 to 2,147,483,647.
Signed vs Unsigned
The `int` type can be declared as signed or unsigned. A `signed int` can hold both negative and positive integers, while an `unsigned int` can only store positive integers, effectively doubling the upper limit of values it can hold.
Code Snippet for Size and Range
#include <iostream>
#include <limits>
int main() {
std::cout << "Size of int: " << sizeof(int) << " bytes" << std::endl;
std::cout << "Min value of int: " << std::numeric_limits<int>::min() << std::endl;
std::cout << "Max value of int: " << std::numeric_limits<int>::max() << std::endl;
return 0;
}
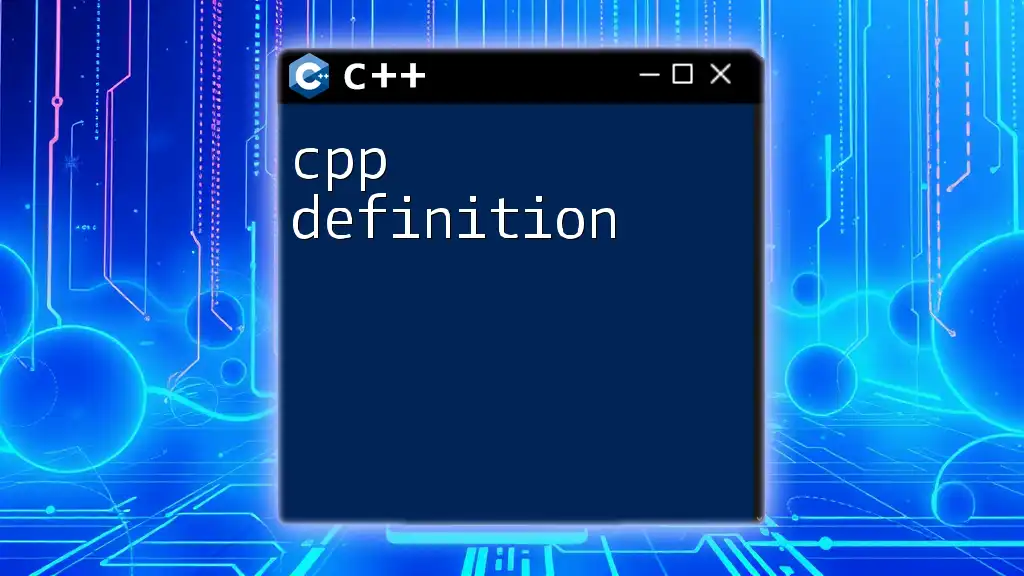
How to Declare and Initialize an `int` Variable
Declaration Syntax
Declaring an `int` variable is straightforward. You simply specify `int` followed by the variable name.
int myNumber;
Initialization Options
In C++, you can initialize `int` variables in several ways:
- Direct Initialization: Assigning a value at the point of declaration.
- Copy Initialization: Initializing using the assignment operator.
Code Examples of Initialization
int a; // Declaration
int b = 10; // Direct Initialization
int c(20); // Copy Initialization
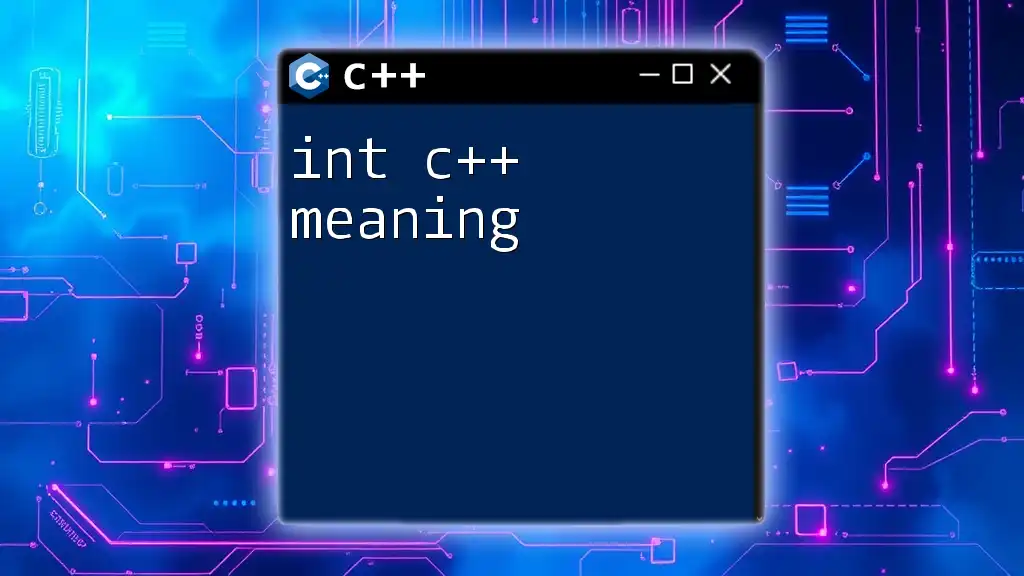
Using `int` in C++ Programs
Basic Arithmetic Operations
The `int` data type allows for various arithmetic operations, including addition, subtraction, multiplication, and division. These operations are performed using standard arithmetic operators.
Examples of Operations
#include <iostream>
int main() {
int a = 10;
int b = 5;
std::cout << "Addition: " << a + b << std::endl;
std::cout << "Subtraction: " << a - b << std::endl;
std::cout << "Multiplication: " << a * b << std::endl;
std::cout << "Division: " << a / b << std::endl;
return 0;
}
Casting and Type Conversion
You may encounter situations where you need to convert data types. C++ allows you to cast between types, including from other types to `int`. This is often necessary when working with mixed types.
Code Snippet for Type Casting
double d = 9.8;
int i = static_cast<int>(d); // Conversion from double to int
std::cout << "Casted value: " << i << std::endl; // Outputs 9
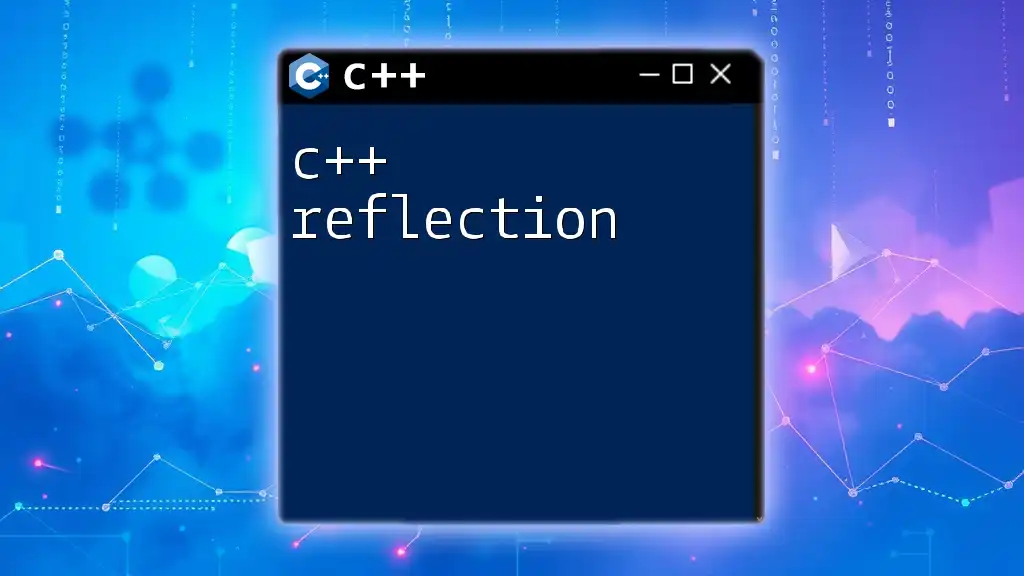
Common Mistakes with `int`
Overflow and Underflow
One of the most frequent mistakes made with the `int` type is integer overflow or underflow. This occurs when you exceed the maximum or minimum value an `int` can hold, leading to unpredictable behavior.
Code Snippet for Overflow
#include <iostream>
#include <limits>
int main() {
int max_int = std::numeric_limits<int>::max();
std::cout << "Max int: " << max_int << std::endl;
std::cout << "Overflow: " << max_int + 1 << std::endl; // Undefined Behavior
return 0;
}
Using `int` in Loops and Conditions
When using `int` in control structures like loops and conditional statements, be cautious. Incorrect assumptions about the range or value of the integer can lead to infinite loops or logical errors.
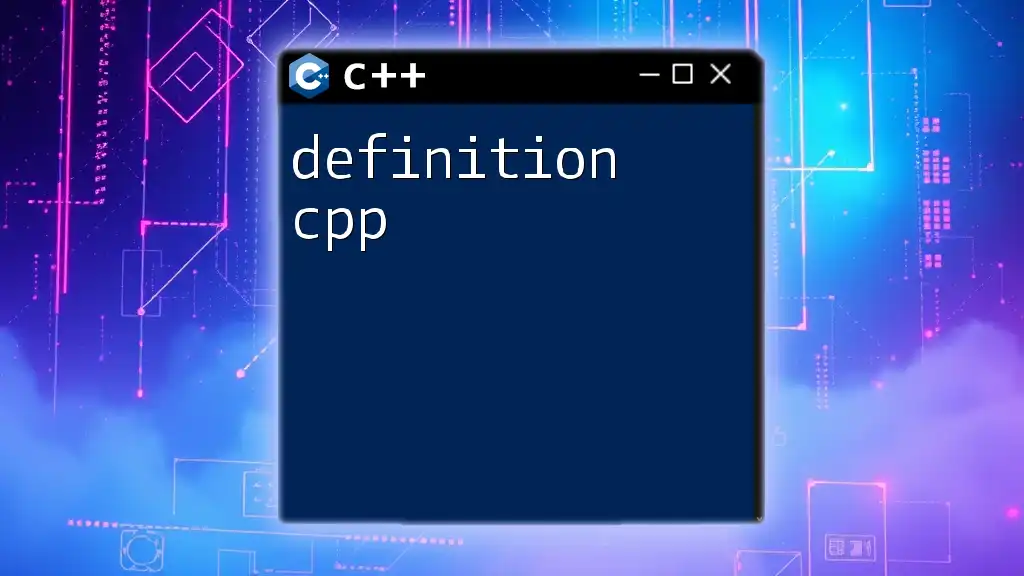
Advanced Topics
`int` in Functions
The use of `int` also extends to functions. You can pass `int` variables to functions either by value (copying the actual value) or by reference (passing the address) for more efficient memory usage.
Code Snippet for Function Example
int add(int a, int b) {
return a + b;
}
int main() {
int result = add(5, 10);
std::cout << "Result: " << result << std::endl;
return 0;
}
`int` in Standard Template Library (STL)
The `int` data type is frequently used in STL containers, such as vectors and lists, to represent collections of integer values. This showcases the `int` type's versatility within the C++ programming ecosystem.
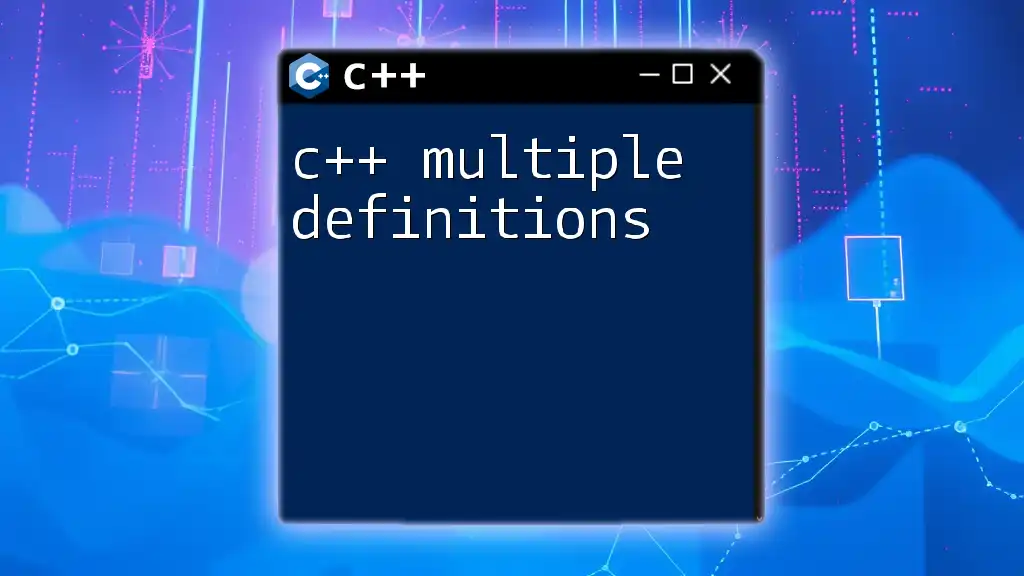
Conclusion
Understanding the int C++ definition is crucial for anyone looking to utilize this powerful programming language efficiently. The `int` type serves as a foundational building block in C++, enabling you to perform arithmetic operations, manage data, and create robust applications. Experimentation is key—try incorporating `int` types in your projects to enhance your grasp of their functionality and potential.
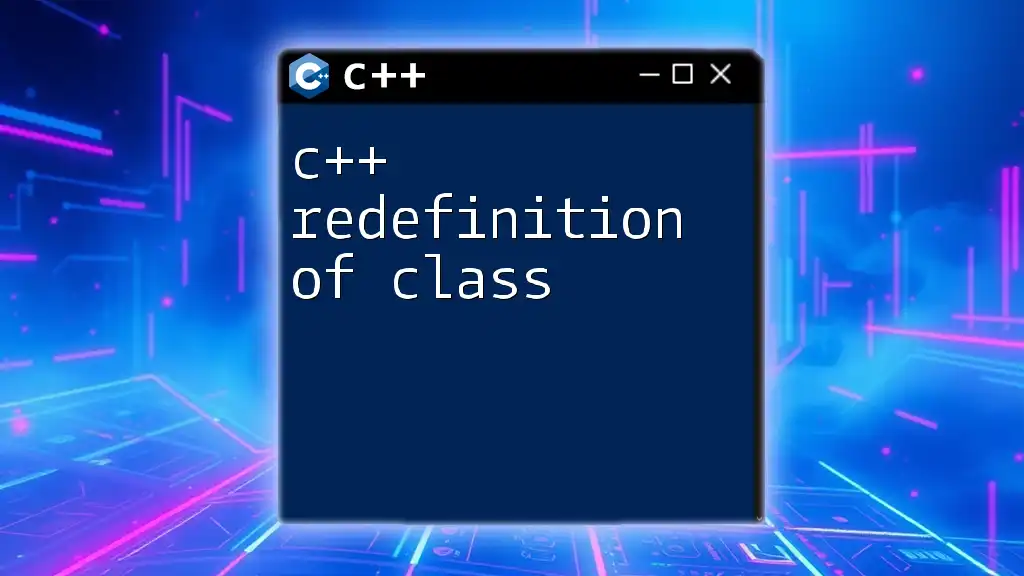
Additional Resources
To deepen your understanding of `int` and data types in C++, consider diving into reputable resources such as advanced textbooks, online courses, or official C++ documentation. Participating in community forums can also provide valuable insights and support as you hone your programming skills.