In C++, `int` is a fundamental data type used to declare variables that can store integer values.
int main() {
int number = 5; // Declares an integer variable named 'number' and initializes it to 5
return 0;
}
Understanding Data Types in C++
What are Data Types?
Data types are essential concepts in programming languages, serving as classifications for defining the type of data a variable can hold. They dictate the operations that can be performed on the data and determine how much space it occupies in memory. Understanding data types is crucial for developing efficient and bug-free applications.
Categories of Data Types in C++
When we categorize data types in C++, they are commonly divided into two main groups:
- Primitive Data Types: These include fundamental types like `int`, `char`, `float`, and `double`. Each serves a specific function and has defined characteristics.
- User-defined Data Types: This category includes more complex types like structs, unions, and enums, allowing developers to create types that suit their specific needs.
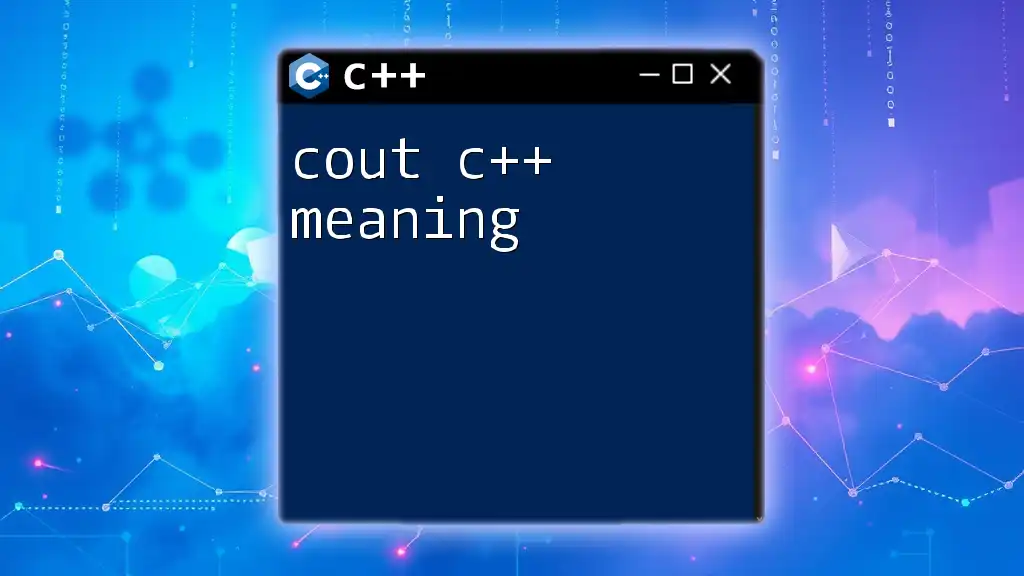
The int Data Type: A Closer Look
Definition of int
The `int` data type is one of the most commonly used data types in C++. It represents integer values, which are whole numbers without any fractional component. The use of `int` allows for the representation of positive and negative numbers as well as zero.
Characteristics of int
The `int` data type comes with specific characteristics that define its behavior in your programs:
- Storage Size: An `int` typically occupies 4 bytes (32 bits) on most systems.
- Value Range: Standard `int` can represent values from -2,147,483,648 to 2,147,483,647. This range may vary on different architectures.
- Signed vs Unsigned int: An `int` can be signed (default) or unsigned, where an unsigned `int` can represent only non-negative integers, effectively doubling the maximum positive range.
Variance in int Data Type Across Systems
The range of the `int` data type is specified by the C++ standard but can still vary based on the system architecture. It is essential to understand the specific limits defined in the `<limits>` header. For example, on a 16-bit system, an `int` may only range from -32,768 to 32,767, rather than the standard range found in 32-bit systems.
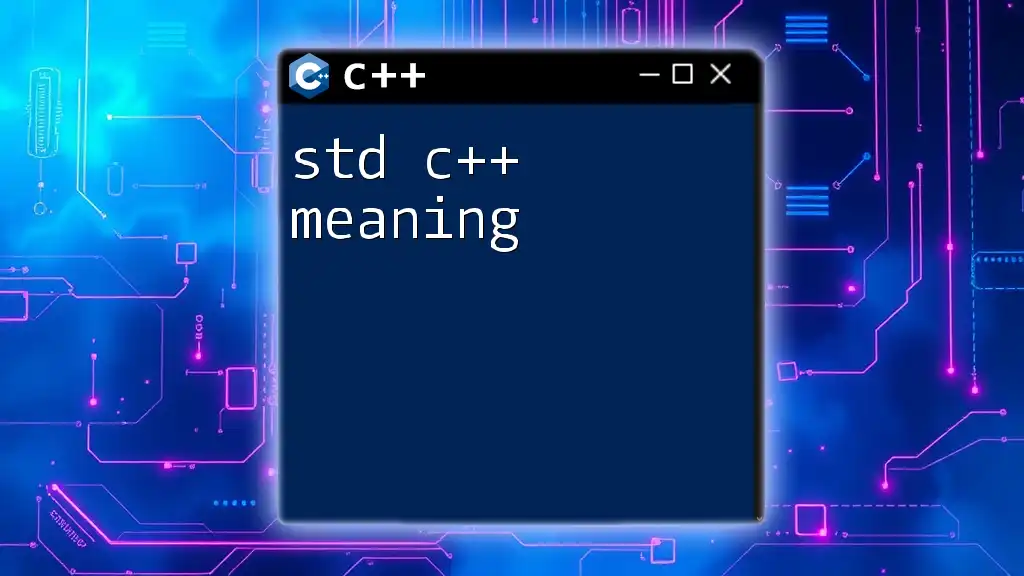
Declaring and Using int in C++
Declaring an int Variable
In C++, you can declare an integer variable using simple syntax. The general format is:
int myVariable;
It’s straightforward and allows you to create integer variables where `myVariable` is now ready to store an integer value.
Initializing an int Variable
Initializing an `int` variable means giving it an initial value at the time of declaration. This can be done in several ways:
int myVariable = 10;
This example assigns the value `10` to `myVariable`. It’s always a good practice to initialize your variables to prevent undefined behavior.
Example: Simple int Operations
Using `int` in calculations is straightforward. Here’s a simple example demonstrating basic arithmetic operations:
int a = 5;
int b = 10;
int sum = a + b; // sum will be 15
In this example, we defined two integers, `a` and `b`, and computed their sum, demonstrating how `int` can be used in common operations.

Common Uses of int in C++
Counting and Iterations
One of the most common uses of the `int` data type is in loop constructs. For example, you can utilize `int` in a `for` loop to run a set of instructions multiple times:
for(int i = 0; i < 10; i++) {
// Loop body with operations using i
}
In this example, `i` is an integer variable controlling the loop iteration, starting from `0` and incrementing until it reaches `10`.
Example: Simple Number Comparison
Another common scenario for `int` is in conditional statements. Below is an example where we use `int` to compare two numbers:
int x = 10;
int y = 5;
if (x > y) {
// This block executes if x is greater than y
}
This snippet demonstrates how `int` values can be used in logical comparisons, allowing for decision-making in code execution.

Best Practices when Using int
Choosing Between int, short, and long
When working with integer values, understanding when to use `int`, `short`, or `long` is critical for optimizing your program. Generally, `int` is suitable for most use cases. However, if memory usage is a concern, you may choose `short` for smaller numbers, while `long` is appropriate when you expect values exceeding the range of `int`.
Avoiding Overflow Issues
Integer overflow occurs when an arithmetic operation exceeds the range that can be stored within the variable. For example, adding `1` to the maximum `int` value results in an overflow, wrapping around to the minimum value of `int`.
To prevent such issues, you can:
- Use larger data types like `long` or `long long` when you expect big numbers.
- Implement checks before performing operations to ensure values stay within safe limits.
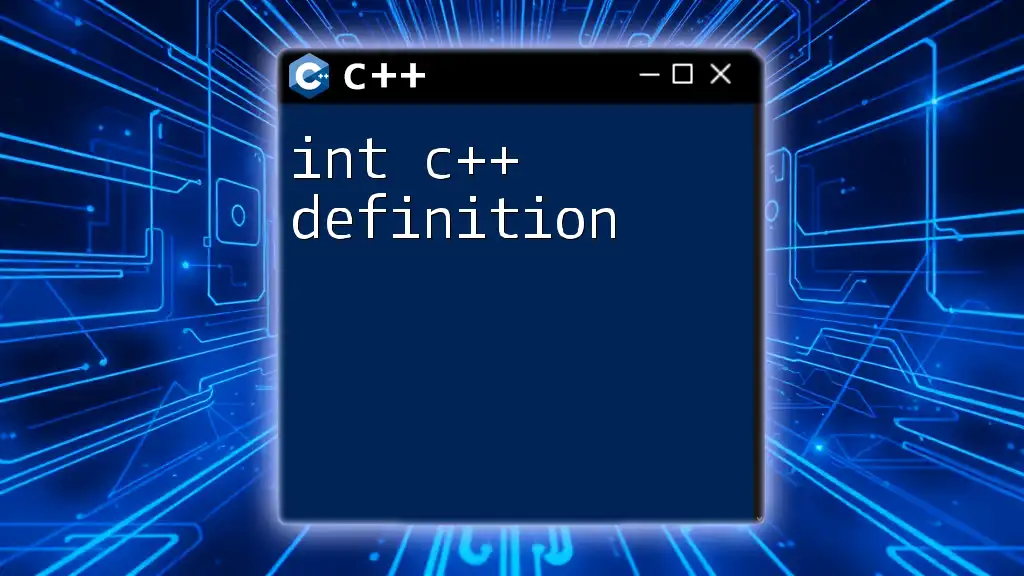
Conclusion
In summary, understanding the int C++ meaning is vital for effective programming. The `int` data type not only represents integers but also plays a crucial role in various operations, such as looping and conditionals. By getting familiar with its characteristics, usage, and best practices, you'll be equipped to harness the power of this fundamental data type efficiently. Always remember to keep practicing and experimenting with different `int` applications to deepen your knowledge and skills in C++ programming.