In C++, `cin` is an object of the `istream` class that is used to read input from the standard input device (usually the keyboard).
Here's a code snippet demonstrating how to use `cin`:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
cout << "You entered: " << number << endl;
return 0;
}
What Does `cin` Mean in C++?
`cin`, short for "character input," is an essential part of the C++ programming language, specifically designed to handle input from the user. It is a predefined object of class `istream` and is used to read data from the standard input stream, typically the keyboard.
Understanding Standard Input in C++
In any programming language, handling input is a crucial functionality. C++ provides the `cin` object to facilitate this. Input handling allows a program to dynamically respond to user data, making applications interactive. Without user input, programs become static and lose their practicality.
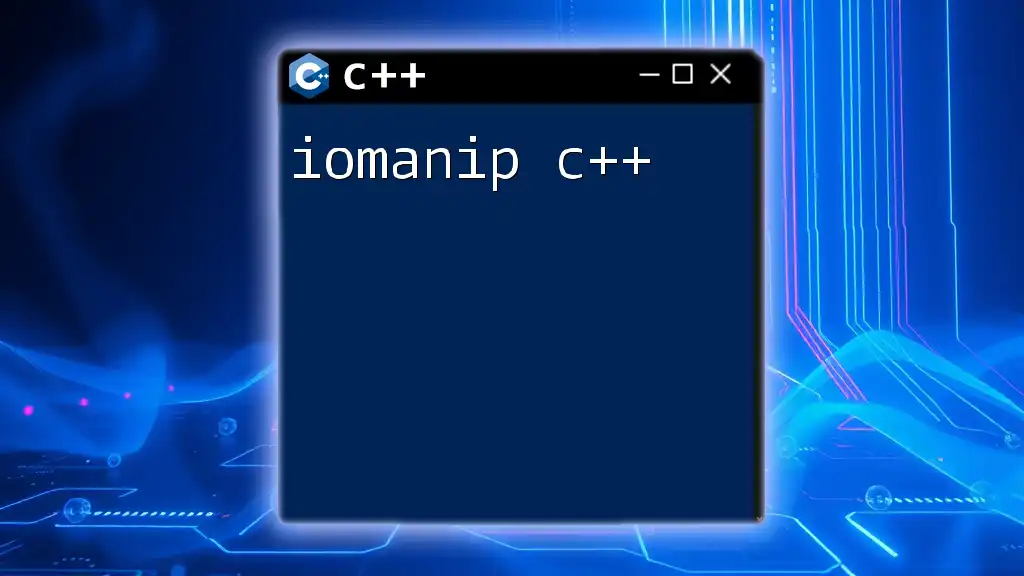
How to Use `cin` in C++
Basic Syntax of `cin`
The syntax for using `cin` is quite straightforward. You utilize the extraction operator (`>>`) to read data from the input stream into a variable.
Here’s a simple example of how to use `cin`:
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
cout << "You are " << age << " years old." << endl;
return 0;
}
In this snippet, the program prompts the user to enter their age. The input is then stored in the variable `age`, which is subsequently displayed.
Data Types with `cin`
`cin` can handle various data types, making it versatile for different input requirements. Here are some common types alongside example usages:
-
Integer:
int number; cout << "Enter an integer: "; cin >> number;
-
Float:
float weight; cout << "Enter your weight: "; cin >> weight;
-
String: For strings, you typically use the `cin` object directly:
string name; cout << "Enter your name: "; cin >> name;
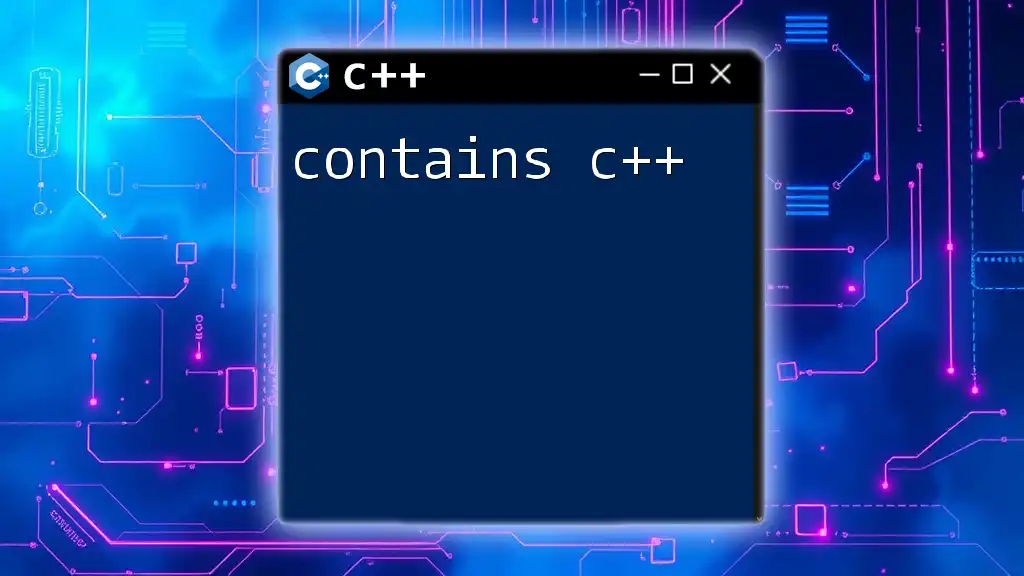
Common Use Cases for `cin`
Reading User Input
`cin` is most commonly used when you want to gather data from users, such as their names, ages, or preferences. This interaction is what makes applications user-friendly.
Taking Multiple Inputs
You can also use `cin` to accept multiple inputs simultaneously. This feature is particularly useful when you want to collect related data at once. Here’s how to do it:
int x, y;
cout << "Enter two numbers: ";
cin >> x >> y;
cout << "Sum: " << (x + y) << endl;
In this example, users are prompted to enter two numbers, which are then summed together and displayed.
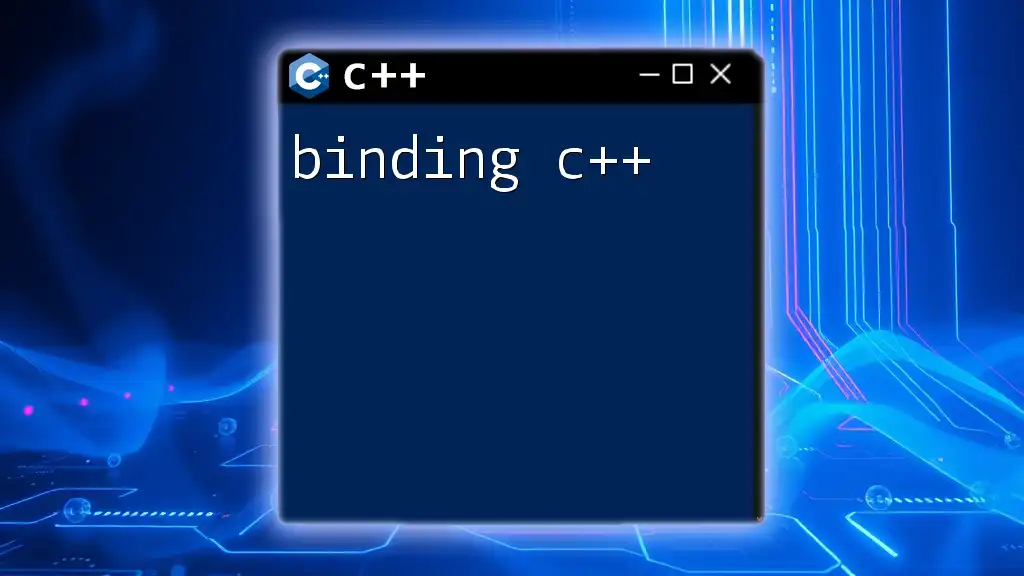
Error Handling with `cin`
What Happens on Invalid Input?
One challenge of using `cin` is handling scenarios where the user does not provide valid input. When such an event occurs, the program may enter an error state, leading to undesirable behavior.
Using `cin.fail()` to Check for Errors
To manage errors effectively, you can use the `cin.fail()` function. Here’s a simple way to implement error handling:
int value;
cout << "Enter a number: ";
cin >> value;
if (cin.fail()) {
cin.clear(); // clear error state
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // discard invalid input
cout << "Invalid input! Please enter a number." << endl;
}
In this code, if the input is invalid, the error state is cleared, and also any remaining invalid input is discarded, prompting the user to try again.
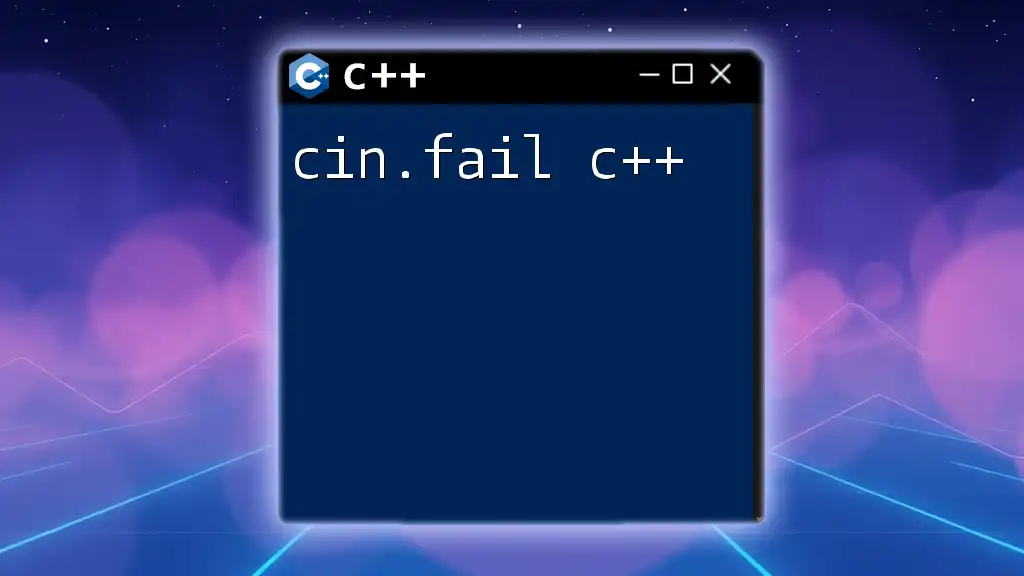
Working with `cin` and Loops
Using `cin` in Loops for Continuous Input
You can also use `cin` in loops, allowing for repeated user input until a condition is met. This is particularly useful in scenarios where you need users to input multiple values continuously.
Here’s how you can do it:
int number;
while (true) {
cout << "Enter a positive number (0 to quit): ";
cin >> number;
if (number == 0) break;
cout << "You entered: " << number << endl;
}
In this example, the program will continue to prompt for input until the user enters `0`, allowing a continual interaction.
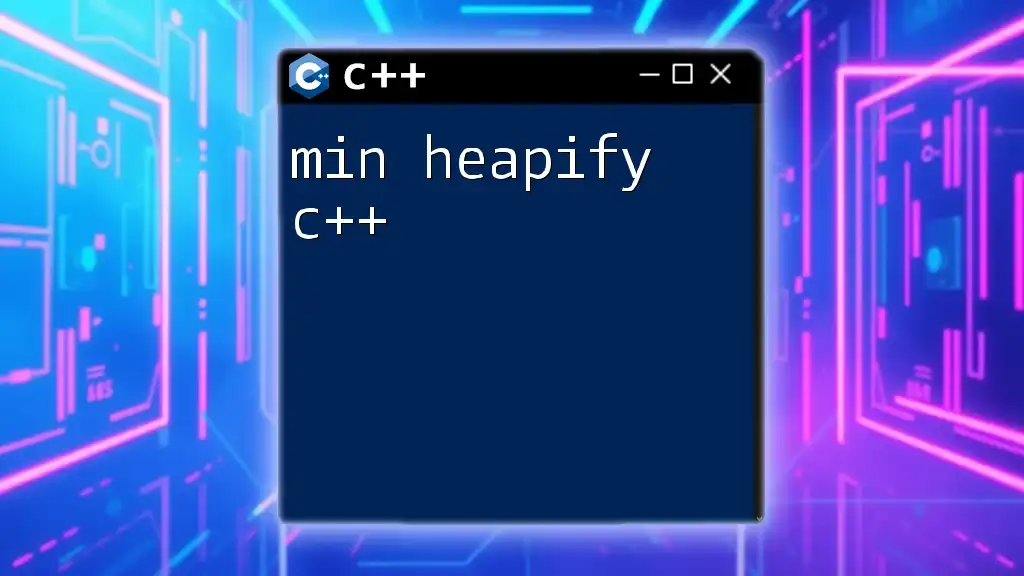
Advanced Features of `cin`
Using `cin.get()` for Character Input
Beyond the extraction operator (`>>`), C++ provides `cin.get()` for reading character input. This method provides an alternative way to gather input and is especially useful when you need to read a single character.
Here’s a simple example:
char ch;
cout << "Enter a character: ";
cin.get(ch);
cout << "You entered: " << ch << endl;
This code takes a single character as input and displays it back to the user.
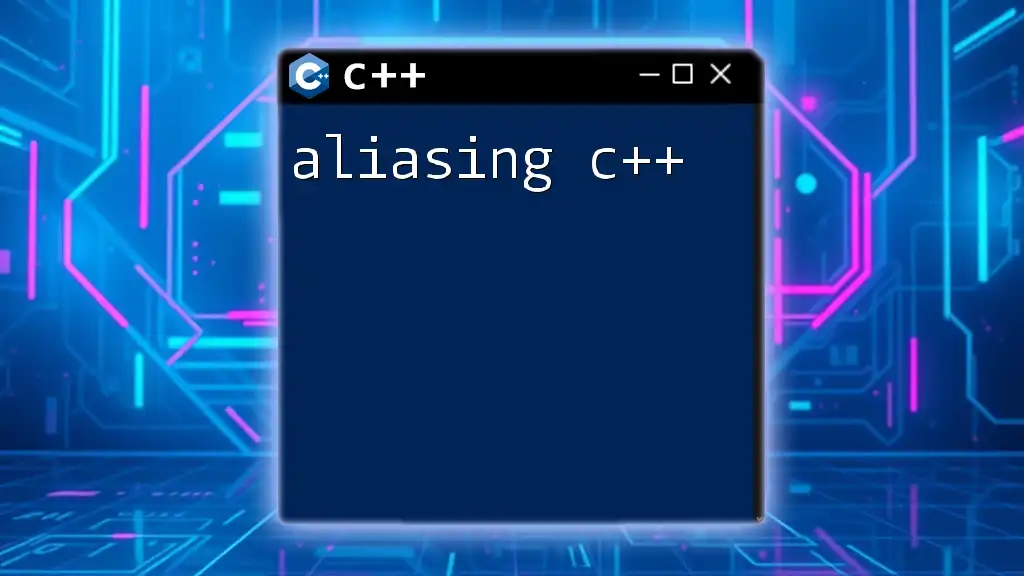
Conclusion
In summary, understanding the `cin` meaning in C++ is vital for effective programming in the language. By mastering `cin`, users can create dynamic applications that interactively gather input, handle errors gracefully, and respond appropriately to user data.
I encourage you to experiment with `cin` in your C++ programs. Practice handling different data types, incorporate error handling, and utilize loops to enhance user interaction. As you gain proficiency, you’ll find that handling input is a fundamental skill that contributes significantly to writing effective C++ applications.
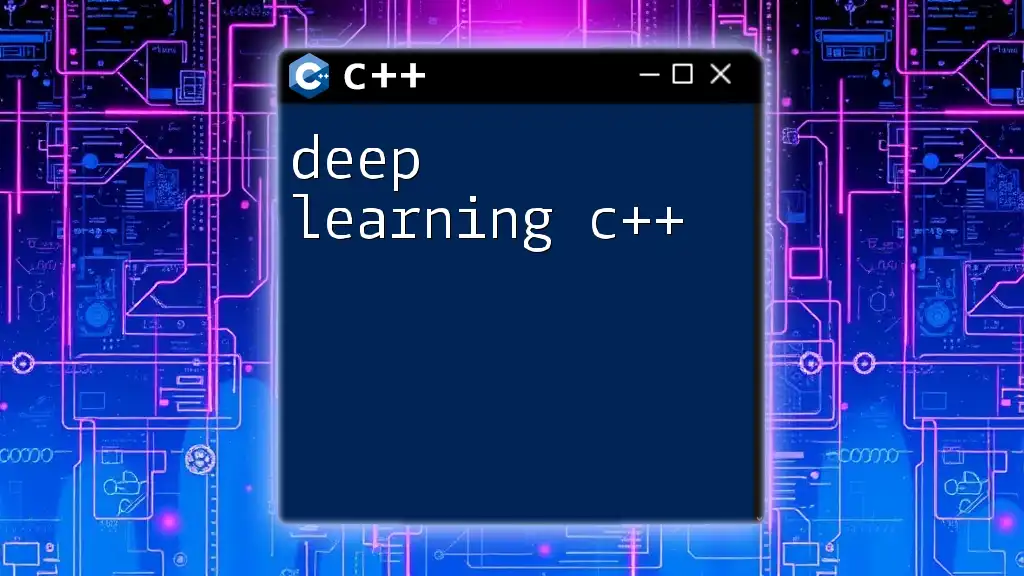
Additional Resources
To further your learning, you might explore the C++ documentation on input and output or consider enrolling in online courses that provide comprehensive tutorials on C++.