Clang is a compiler for C++ (and C) that provides a modern framework for developing efficient code, offering features like fast compilation and excellent diagnostics.
Here’s a simple example of a C++ program that utilizes clang for compilation:
#include <iostream>
int main() {
std::cout << "Hello, Clang C++!" << std::endl;
return 0;
}
To compile this code using Clang, you would run the following command in your terminal:
clang++ -o hello hello.cpp
What is Clang C++?
Clang C++ is a powerful and flexible compiler for the C++ programming language, which is part of the LLVM project. Developed to provide a modern alternative to traditional C++ compilers, Clang emphasizes performance, modularity, and comfort for both developers and end users. Its development was prompted by the need for a compiler that not only compiles efficiently but also offers better diagnostics than its predecessors.
Historically, Clang began development in 2007 and has quickly become a favorite in the programming community due to its impressive architecture and features. Notably, it fully supports various C++ standards, making it a prudent choice for developers looking to leverage the newest functionality in the language.
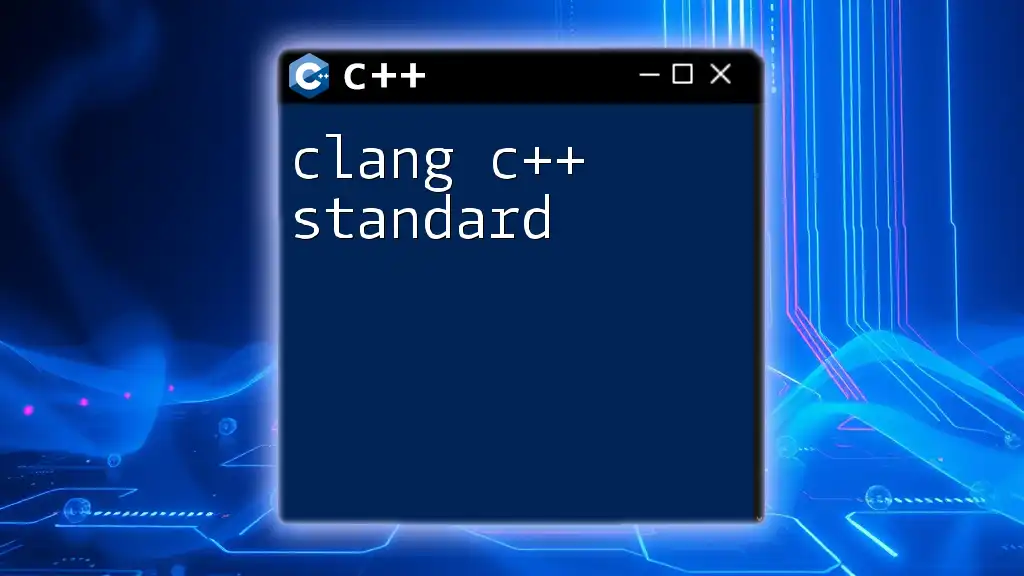
Setting Up Clang C++
Installing Clang
Installing Clang C++ varies slightly depending on your operating system:
-
Windows: Use the LLVM installer, which you can download from the official LLVM website. The installer makes it easy to set up Clang along with other LLVM tools.
-
macOS: Clang can be installed via Homebrew. Run the command:
brew install llvm
-
Linux: Use your package manager, for example, on Ubuntu:
sudo apt install clang
After installation, you should verify Clang is correctly installed by executing:
clang --version
This command should return the installed Clang version, confirming a successful installation.
Configuring Your Environment
To get the most out of Clang, configuring your development environment is critical. If you use Visual Studio Code, you can install the C/C++ extension, which provides IntelliSense, debugging, and code navigation capabilities. For CLion, Clang is already integrated, allowing you to configure it through the IDE settings.
Basic configurations include setting the path to your Clang installation and defining your build system (Makefile, CMake, etc.). Ensure your IDE is set to use the correct version of Clang for optimal performance and compatibility.
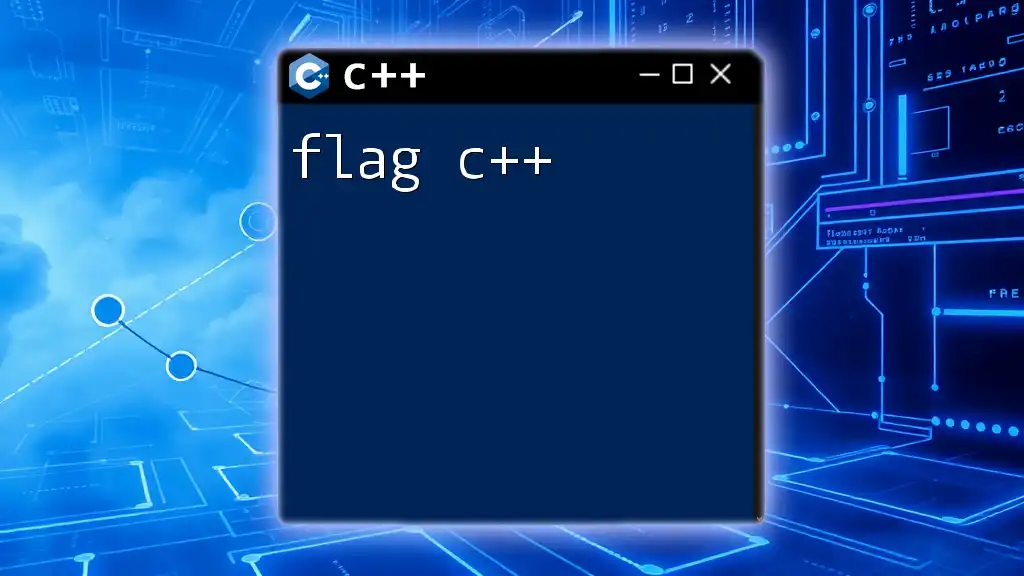
Key Features of Clang C++
Fast Compilation
One of Clang's standout features is its rapid compilation speed. Clang significantly reduces the time it takes to compile C++ programs compared to many traditional compilers. This speed is particularly valuable in larger projects where lengthy compile times can slow down development.
For instance, a comparative speed test could be illustrated simply:
clang++ main.cpp -o main
g++ main.cpp -o main
In many cases, the Clang command will execute faster, allowing developers to iterate quickly through their changes.
Static Analysis Tools
Clang includes powerful static analysis tools that analyze code before it executes. This functionality helps catch potential bugs or issues early in the development cycle, which can save time and resources down the line. For instance, Clang can detect memory leaks or resource management issues, which are common pitfalls in C++ development. It is not just about compiling code; it’s about compiling safe code.
Excellent Error Messaging
One of Clang's major advantages is its intuitive error messaging. Unlike many compilers that provide cryptic error messages, Clang aims to provide clear and actionable diagnostics. For example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl
return 0;
}
If the line above were compiled, Clang would provide an error like:
error: expected ';' at end of declaration
This type of feedback helps minimize debugging time and accelerates your development workflow.
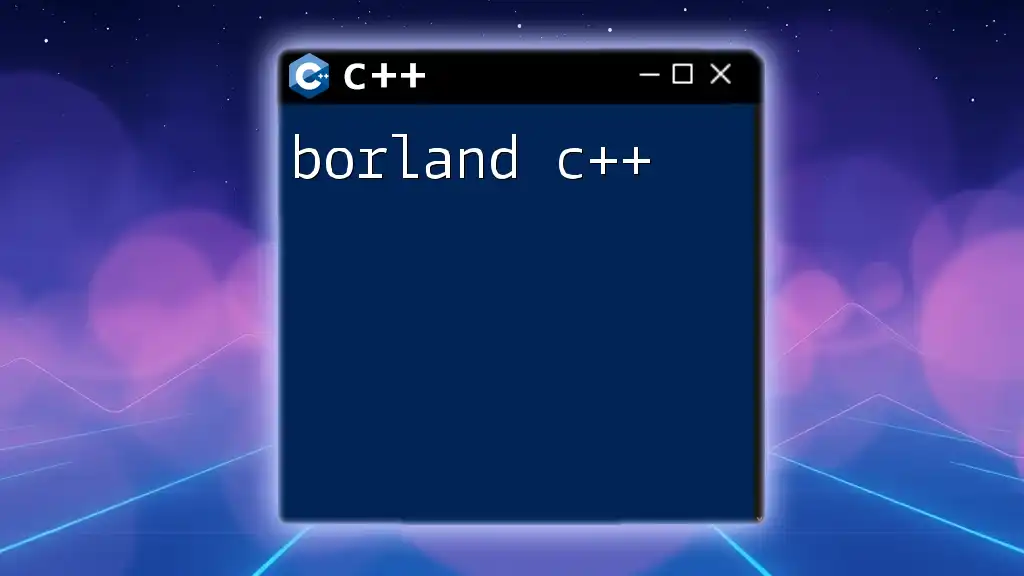
Writing Your First Clang C++ Program
Creating a Simple C++ Program
Getting started with Clang C++ is as straightforward as writing a simple program. Here’s how to create a classic “Hello, World!” application:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Compiling and Running Your Program
To compile the above program using Clang, navigate to the directory where your file is saved (let's say it's named `hello.cpp`) and execute:
clang++ hello.cpp -o hello
This command compiles the program and generates an executable named `hello`. To run your program, simply enter:
./hello
You should see the output:
Hello, World!
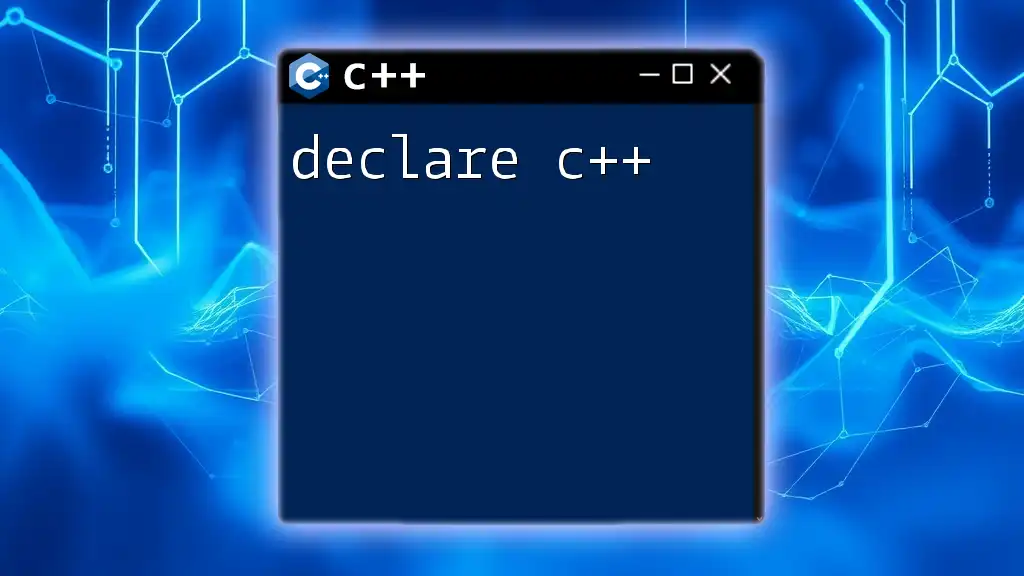
Advanced Features of Clang C++
C++ Standards Support
Clang supports several C++ standards, including C++11, C++14, C++17, and the latest, C++20. Using modern C++ features allows developers to write cleaner, more efficient, and safer code. Specifying the standard during compilation can be done with a flag:
clang++ -std=c++20 hello.cpp -o hello
Template Metaprogramming
C++ templates are powerful tools that allow you to write generic and reusable code. Clang provides full support for such features, enabling developers to build sophisticated algorithms. For example:
template <typename T>
T add(T a, T b) {
return a + b;
}
This template function can be used with any data type that supports the `+` operator, showcasing the versatility that comes with Clang's support for templates.
Optimizations
Clang's optimization capabilities are robust. When building production code, using optimization flags can significantly enhance performance. Common flags include `-O1`, `-O2`, and `-O3`, each providing a different level of optimization. For example, to compile with optimizations, you would use:
clang++ -O2 example.cpp -o example
Note: Higher levels of optimization may increase compile times, so it’s worth profiling to find the best balance for your specific project.
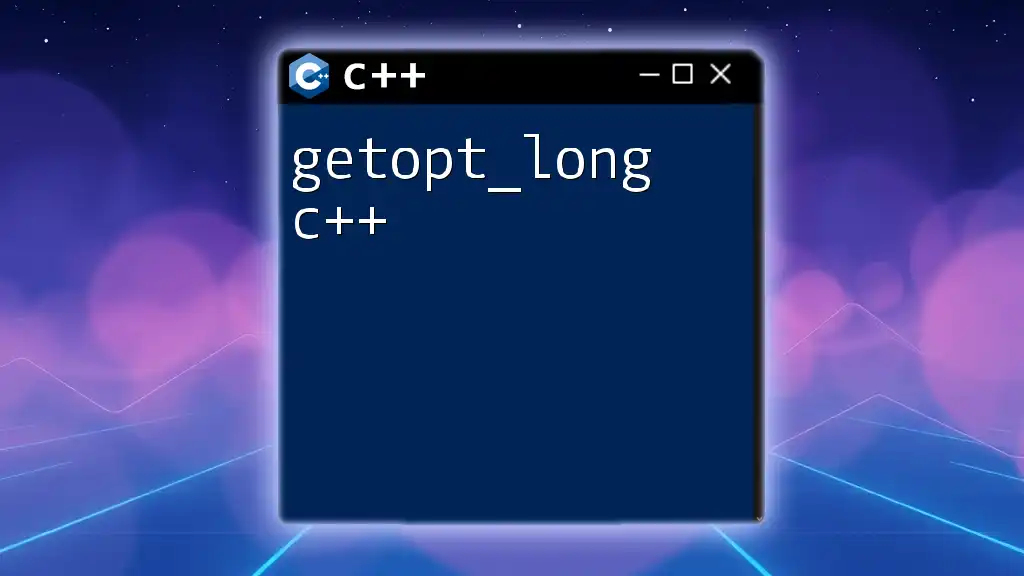
Clang Tools and Ecosystem
Clang-Tidy
Clang-Tidy is a valuable tool for maintaining code quality and improving overall code style. It works as a linter, suggesting fixes for common errors and stylistic issues. For example:
clang-tidy myfile.cpp -- -std=c++17
This command analyzes `myfile.cpp` and reports any detected issues, making it easier to enforce coding standards across your project.
Clang-Format
Code formatting is crucial for maintaining readability and consistency. Clang-Format automates this process, allowing you to define a set of formatting rules. To format your code, simply run:
clang-format -i myfile.cpp
This command modifies `myfile.cpp` in place to conform to the defined formatting guidelines.
Other Tools in the Clang Ecosystem
Beyond Clang-Tidy and Clang-Format, the Clang ecosystem includes several other tools such as the Clang Static Analyzer and libclang. These tools enhance code quality and provide advanced features for developers, creating a comprehensive suite to aid in efficient C++ development.
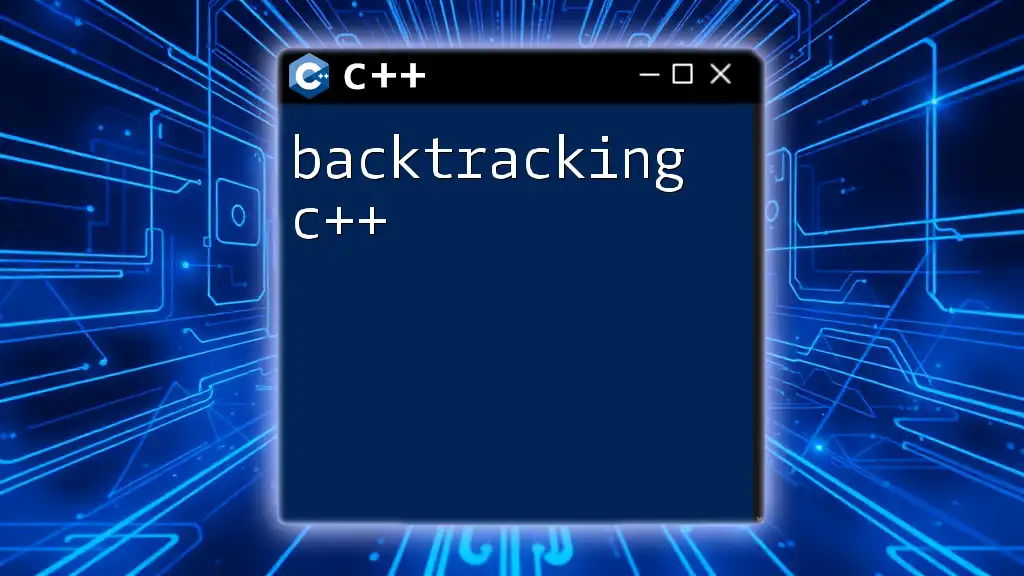
Common Issues and Troubleshooting
Compilation Errors
With any compiler, newcomers might encounter a variety of compilation errors. It's essential to interpret these errors accurately and learn how to address them. Common issues include syntax errors, missing headers, or incorrect data types. Familiarizing yourself with Clang’s error messages will greatly assist you in resolving these quickly.
Optimization Issues
When working with optimizations, developers may experience unforeseen behaviors in their code. Optimizations might alter how code executes, impacting performance. To mitigate any risks related to potential bugs introduced by optimizations, it’s wise to test thoroughly and consider adjusting optimization levels based on your findings.
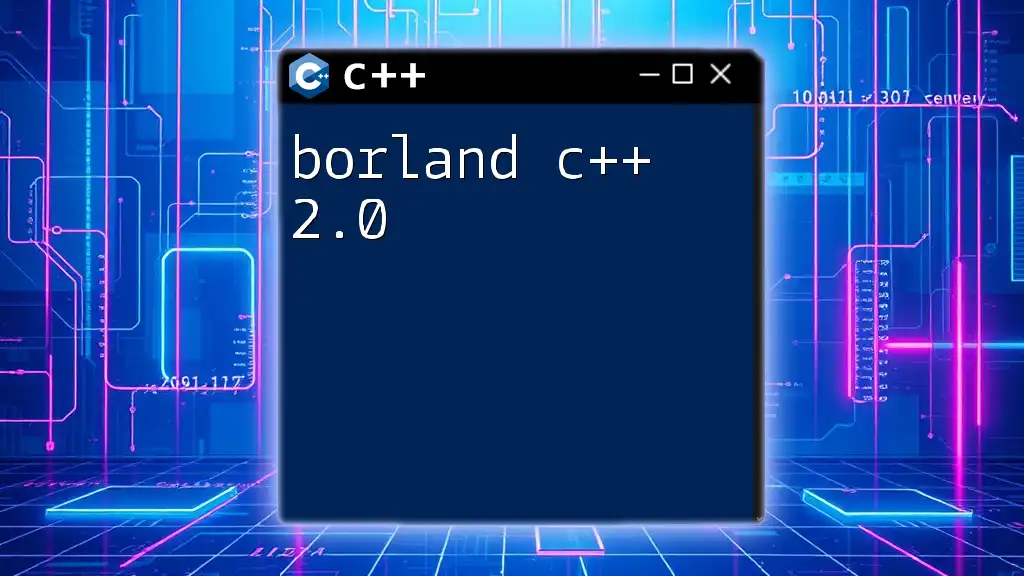
Conclusion
Clang C++ is an impressive compiler that focuses on speed, usability, and modern C++ features. Its robust error messaging, static analysis tools, and ecosystem support make it a strong choice for C++ developers. By setting up Clang correctly and leveraging its advanced tools, developers can streamline their workflows, enhance code quality, and ultimately become more productive in their C++ programming endeavors.
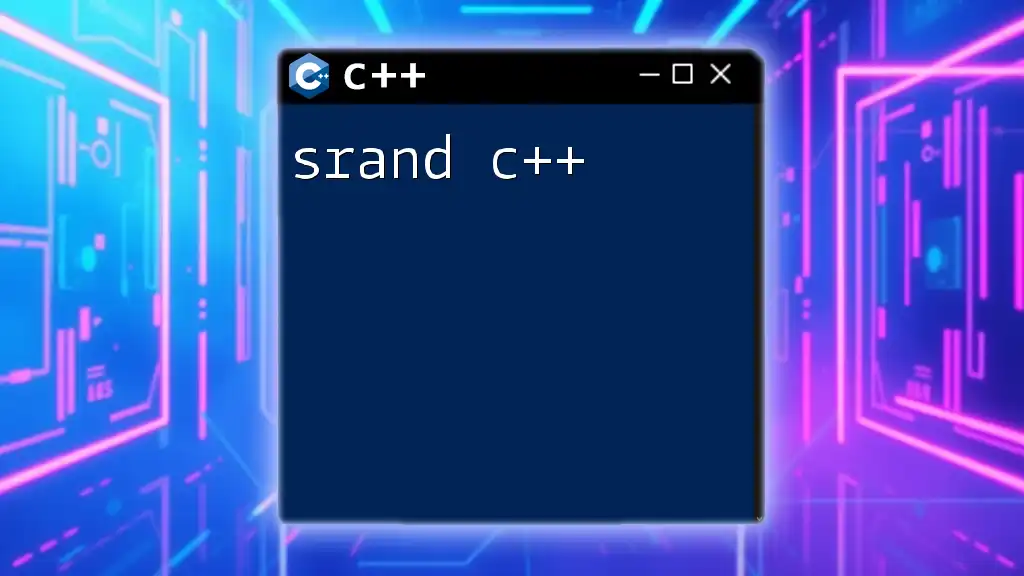
Additional Resources
For those looking to delve deeper into Clang C++, several resources can provide further insights:
- [Clang Official Documentation](https://clang.llvm.org/docs/)
- Books: "C++ Primer" by Lippman, Lajoie, and Moo, "Effective Modern C++" by Scott Meyers
- Online forums and community support available on sites like Stack Overflow and Reddit where you can share issues and seek advice from fellow developers.