The `getopt_long` function in C++ is used to parse command-line options in a more flexible manner than `getopt`, allowing for long options in addition to short ones.
#include <iostream>
#include <getopt.h>
int main(int argc, char *argv[]) {
int option_index = 0;
struct option long_options[] = {
{"verbose", no_argument, nullptr, 'v'},
{"output", required_argument, nullptr, 'o'},
{nullptr, 0, nullptr, 0}
};
int c;
while ((c = getopt_long(argc, argv, "vo:", long_options, &option_index)) != -1) {
switch (c) {
case 'v':
std::cout << "Verbose mode activated.\n";
break;
case 'o':
std::cout << "Output set to: " << optarg << "\n";
break;
case '?':
// Error handling for unknown options
break;
default:
break;
}
}
return 0;
}
Understanding Command-Line Argument Parsing
What is Command-Line Argument Parsing?
Command-line argument parsing is essential for modern applications, allowing programs to receive input directly from users through the terminal. When users execute programs from a command line, they often need to provide options or parameters that modify the program's behavior. This is where parsing comes into play.
Overview of `getopt` and `getopt_long`
The `getopt` library, a staple in C and C++ programming, facilitates the process of parsing command-line options. While `getopt` handles short options (e.g., `-h`, `-v`), `getopt_long` extends this functionality, enabling support for long-form options (e.g., `--help`, `--verbose`). This flexibility enhances usability and user experience by offering more readable command-line interfaces.
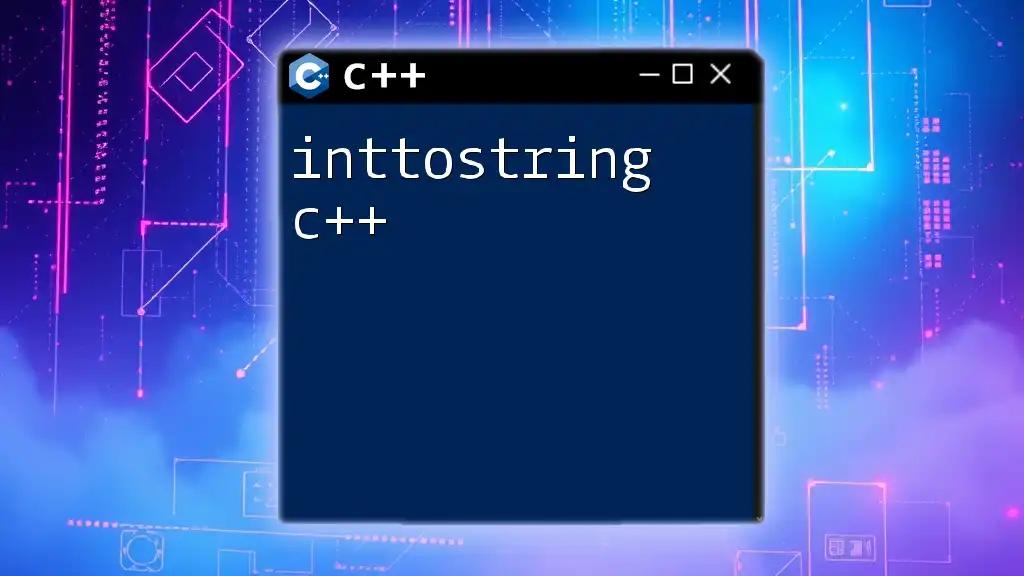
Understanding `getopt_long`
The Purpose of `getopt_long`
`getopt_long` is crucial for C++ applications that require command-line input. It allows developers to define both short and long options, thus making command-line interfaces intuitive for users familiar with different conventions. This is particularly useful in tools and utilities, where users expect options to be self-descriptive.
How `getopt_long` Works
The function signature for `getopt_long` is as follows:
int getopt_long(int argc, char *const argv[], const char *optstring,
const struct option *longopts, int *longindex);
- Parameters:
- `argc` and `argv` represent the argument count and argument vector, respectively.
- `optstring` is a string containing the valid short option characters.
- `longopts` is an array of `struct option` defining the long options.
- `longindex`, if specified, allows tracking the current index in the long options list.
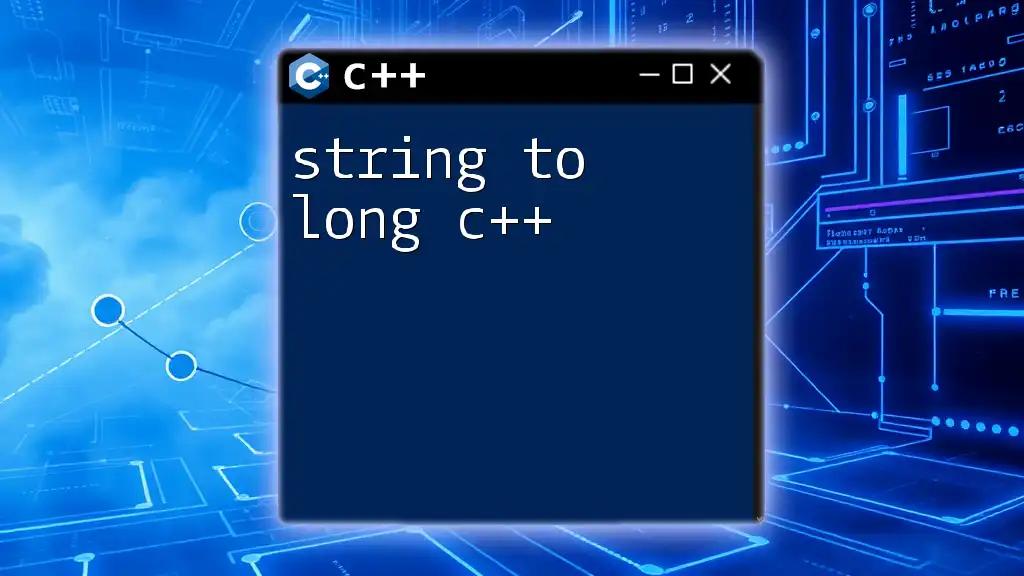
Setting Up Your Environment
Including the Required Header Files
To use `getopt_long`, include the following headers at the beginning of your program:
#include <unistd.h> // for getopt and other POSIX functions
#include <getopt.h> // for getopt_long
#include <iostream> // for input and output
These headers provide the necessary functions and data structures for command-line argument parsing.
Compiling Your Program with `getopt_long`
When compiling a program that utilizes `getopt_long`, use the following command in your terminal:
g++ your_program.cpp -o your_program
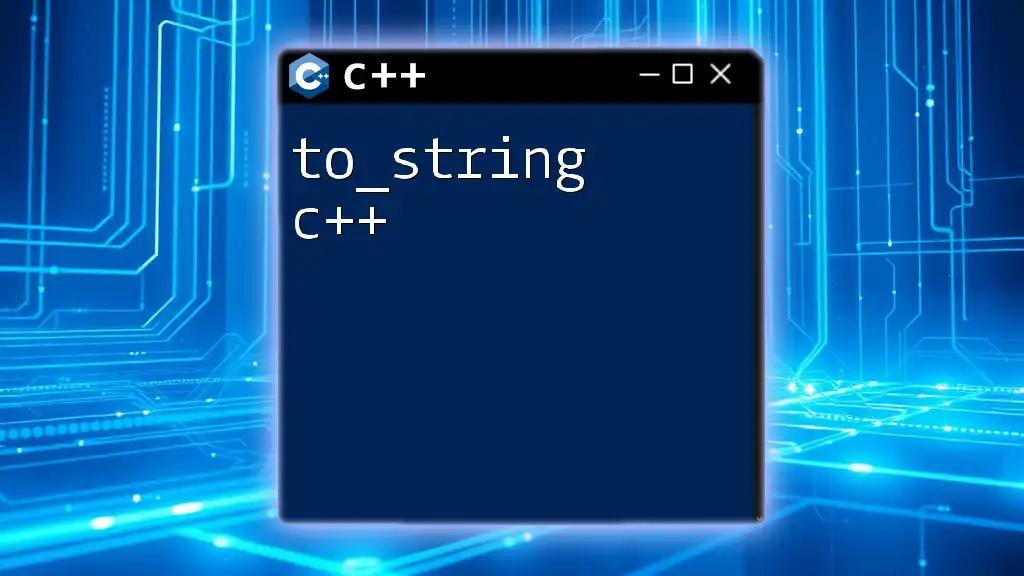
Implementing `getopt_long`
Basic Usage of `getopt_long`
With the groundwork laid, let's start implementing `getopt_long`. Here is a simple example illustrating its usage:
int main(int argc, char *argv[]) {
int option;
struct option long_options[] = {
{"help", no_argument, nullptr, 'h'},
{"verbose", no_argument, nullptr, 'v'},
{"size", required_argument, nullptr, 's'},
{nullptr, 0, nullptr, 0}
};
while ((option = getopt_long(argc, argv, "hvs:", long_options, nullptr)) != -1) {
switch (option) {
case 'h':
std::cout << "Help section..." << std::endl;
break;
case 'v':
std::cout << "Verbose mode enabled." << std::endl;
break;
case 's':
std::cout << "Size: " << optarg << std::endl;
break;
default:
std::cerr << "Unknown option." << std::endl;
return 1;
}
}
return 0;
}
In this example:
- Options are defined in the `long_options` structure. Each long option has a name, the type of argument it takes (if any), a pointer for its associated variable, and a character that represents the option.
- The `while` loop processes the command-line options until all are consumed, passing results to a `switch` statement to execute corresponding actions.
Handling Options and Arguments
Required vs. Optional Arguments
In the `long_options` structure, options like `--size` require an argument, specified with `required_argument`. To denote optional arguments, simply leave this parameter as `no_argument`. This clarity allows programs to convey expectations clearly to users.
Parsing Multiple Long Options
Developers can easily parse multiple long options in a single execution line. Consider the following command example that invokes the program with several options:
./your_program --help --verbose --size 10
The program will recognize and handle each of these options according to the cases defined in the `switch` statement within the loop.
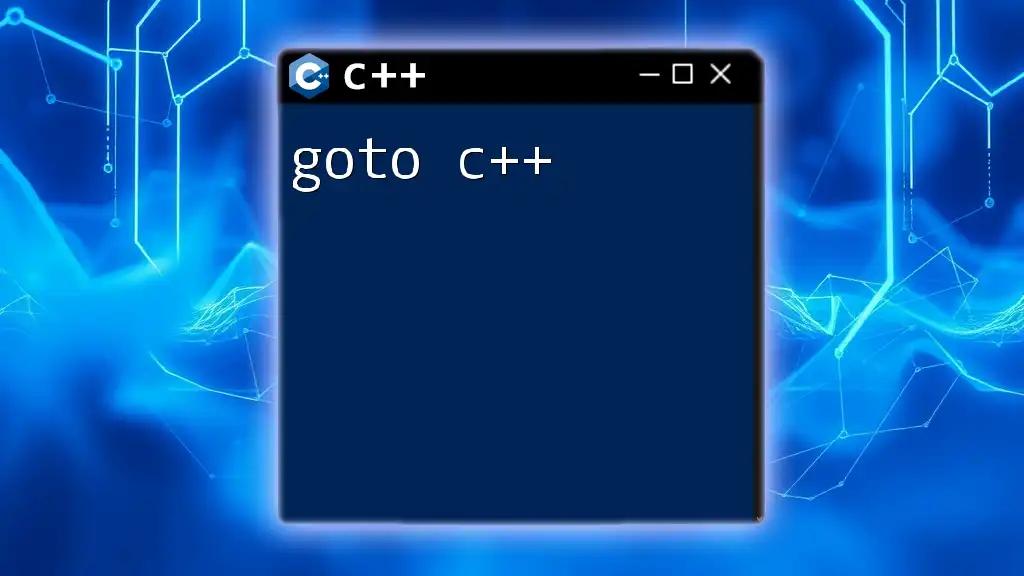
Error Handling
Common Errors with `getopt_long`
While `getopt_long` is a powerful function, developers can encounter errors, such as:
- Invalid option character: A character in `optstring` that is not handled in the `switch` case results in an error.
- Missing required argument: Users must provide an argument when prompted, or the program should handle this gracefully.
Debugging Tips
When debugging command-line argument parsing, using logging within your `switch` cases can help trace the flow of execution. Always ensure clear and user-friendly error messages are provided when options are misused, guiding users on proper usage patterns.
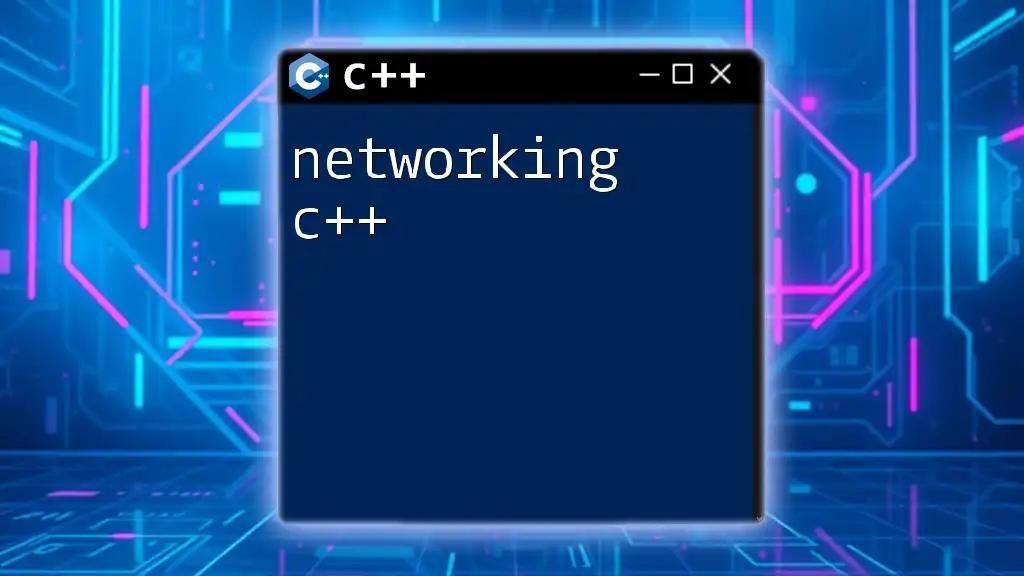
Practical Example
Complete Example Program
Here’s a more comprehensive application that utilizes `getopt_long` effectively:
#include <iostream>
#include <unistd.h>
#include <getopt.h>
int main(int argc, char *argv[]) {
int option;
struct option long_options[] = {
{"help", no_argument, nullptr, 'h'},
{"verbose", no_argument, nullptr, 'v'},
{"size", required_argument, nullptr, 's'},
{"output", required_argument, nullptr, 'o'},
{nullptr, 0, nullptr, 0}
};
while ((option = getopt_long(argc, argv, "hvs:o:", long_options, nullptr)) != -1) {
switch (option) {
case 'h':
std::cout << "Usage: program [options]\n"
<< "Options:\n"
<< "--help, -h Show help\n"
<< "--verbose, -v Enable verbose output\n"
<< "--size, -s SIZE Specify size\n"
<< "--output, -o FILE Specify output file\n";
return 0;
case 'v':
std::cout << "Verbose mode enabled." << std::endl;
break;
case 's':
std::cout << "Size set to: " << optarg << std::endl;
break;
case 'o':
std::cout << "Output file: " << optarg << std::endl;
break;
default:
std::cerr << "Unknown option." << std::endl;
return 1;
}
}
return 0;
}
Testing Your Program
Testing command-line options is critical for validating the functionality of your program. Use unit tests or manually execute command-line commands to ensure your program handles all expected inputs correctly.
For example:
./your_program --size 20 --output result.txt
Expected output:
Size set to: 20
Output file: result.txt

Conclusion
Summary of Key Points
Understanding `getopt_long c++` provides a way to create intuitive command-line interfaces that enhance usability. By examining its syntax, implementation, and the nuances of option handling, developers can create robust applications that cater to user needs.
Additional Resources
For further exploration of `getopt_long`, consider checking out the official GNU documentation or resources like Stack Overflow for community-driven solutions and examples.
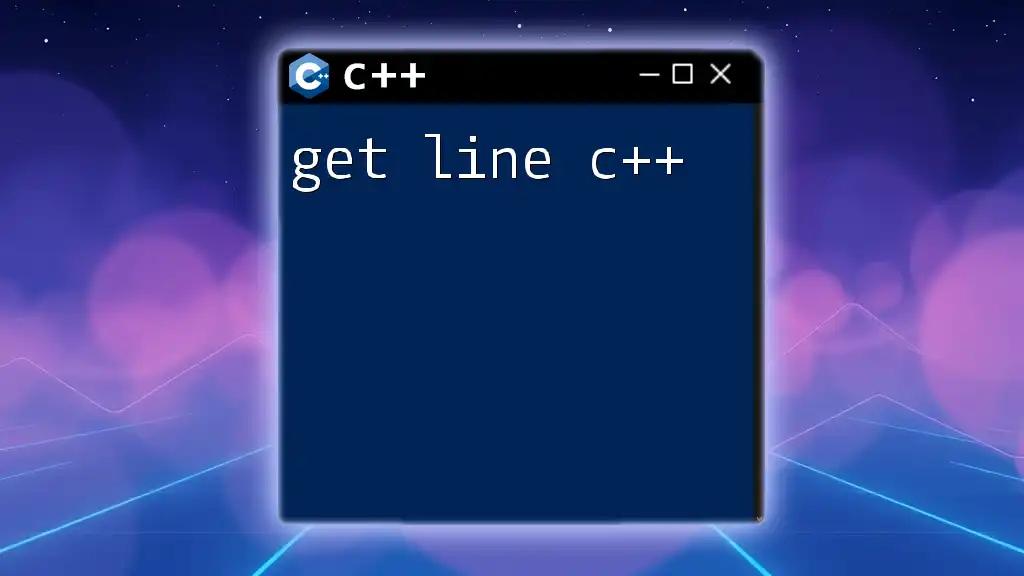
Call to Action
Join Our Community
If you found this guide helpful, stay connected with us for more programming tips and tutorials! Share your experiences using `getopt_long` in the comments or join discussions with fellow C++ enthusiasts.