The `getline` function in C++ is used to read an entire line of text from an input stream, storing it in a string variable.
#include <iostream>
#include <string>
int main() {
std::string line;
std::cout << "Enter a line: ";
std::getline(std::cin, line);
std::cout << "You entered: " << line << std::endl;
return 0;
}
Understanding the Basics of `getline` in C++
What is `getline` in C++?
The `getline` function in C++ is a powerful tool used for reading entire lines of text input. Unlike the standard `cin` method, which reads input until a whitespace character, `getline` captures all characters in a line, including spaces. This makes it particularly useful for applications requiring complete user input, such as names, addresses, or any structured data formatted across lines.
The Importance of `getline` in C++ Programming
Using `getline` is often preferable to other input methods, especially for reading strings containing spaces. It provides a safer and more flexible way to handle user input, ensuring that full lines are captured without truncation. For instance, when asking a user to enter their full name, `getline` will capture both first and last names, unlike `cin`, which would stop at the first space.
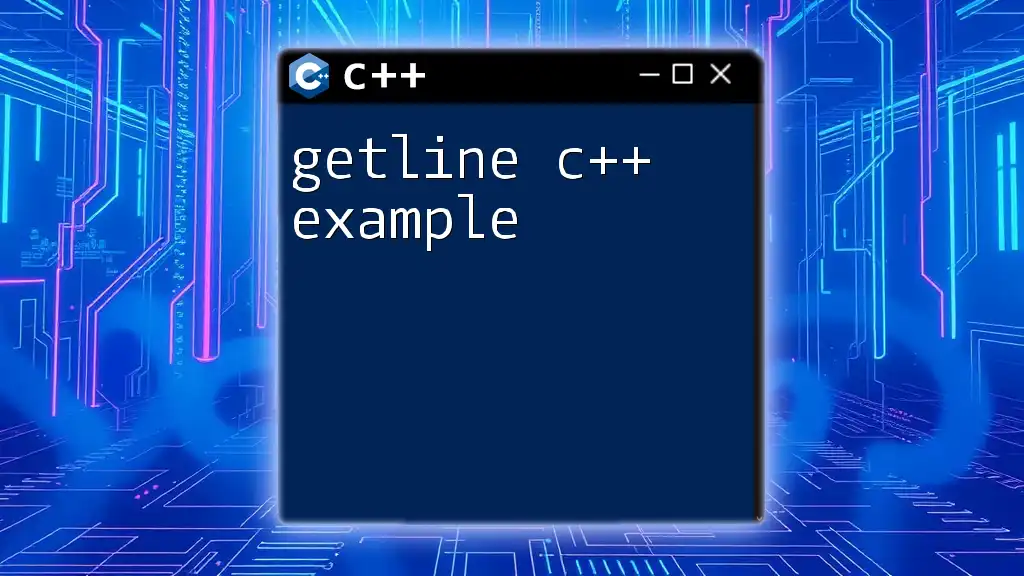
The C++ `getline` Function
Syntax of `getline`
The standard syntax of the `getline` function is simple yet effective:
std::getline(std::cin, string_variable);
In this line, `std::cin` is the input stream, and `string_variable` is the `std::string` where the line will be stored.
How Does `getline` Work in C++?
`getline` operates by reading characters from the input stream until a newline character is encountered, which signals the end of the line. Upon capturing the line, it automatically discards the newline character, allowing developers to work with clean inputs.
An important aspect to understand is the buffering that occurs: `getline` buffers input until the user signifies the end of their input with the `Enter` key. This mechanism allows for smooth and uninterrupted input processes.
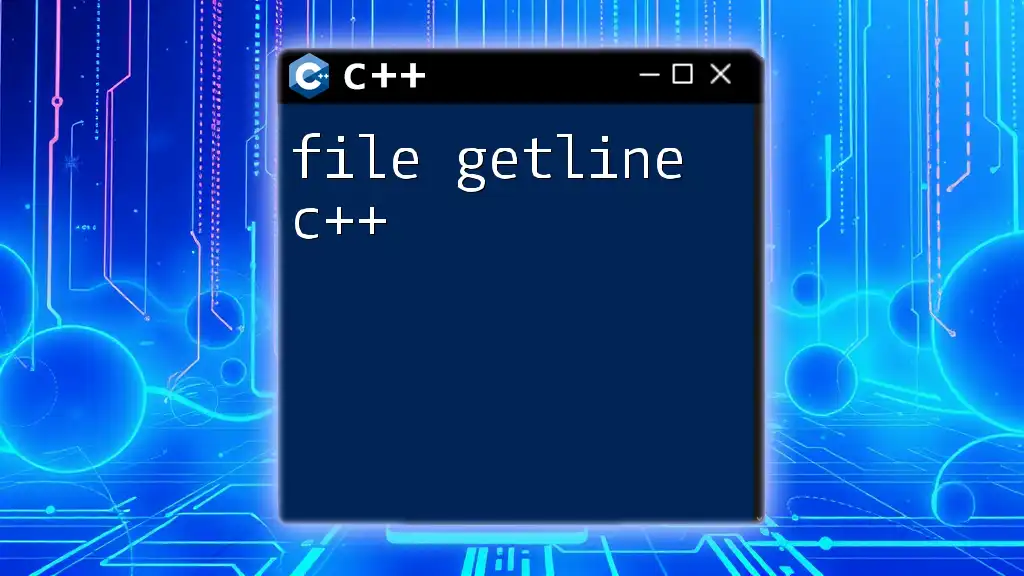
Using `getline` with Different Data Types
Read Strings into `std::string`
The most common usage of `getline` is to read data into a `std::string` variable. Here's a basic example:
std::string name;
std::cout << "Enter your name: ";
std::getline(std::cin, name);
std::cout << "Hello, " << name << "!";
In this code, the user is prompted to enter their name, and the program captures the entire line of input.
Read Lines and Store in `char` Arrays
Though `std::string` is preferred for its convenience, you can also use `getline` with `char` arrays. However, caution is needed regarding array size to avoid buffer overflows. Here's how you might do it:
char buffer[100];
std::cout << "Enter text: ";
std::cin.getline(buffer, 100);
In this example, `getline` reads input into the `buffer`, stopping after 100 characters, and ensuring that there are no overflow issues.
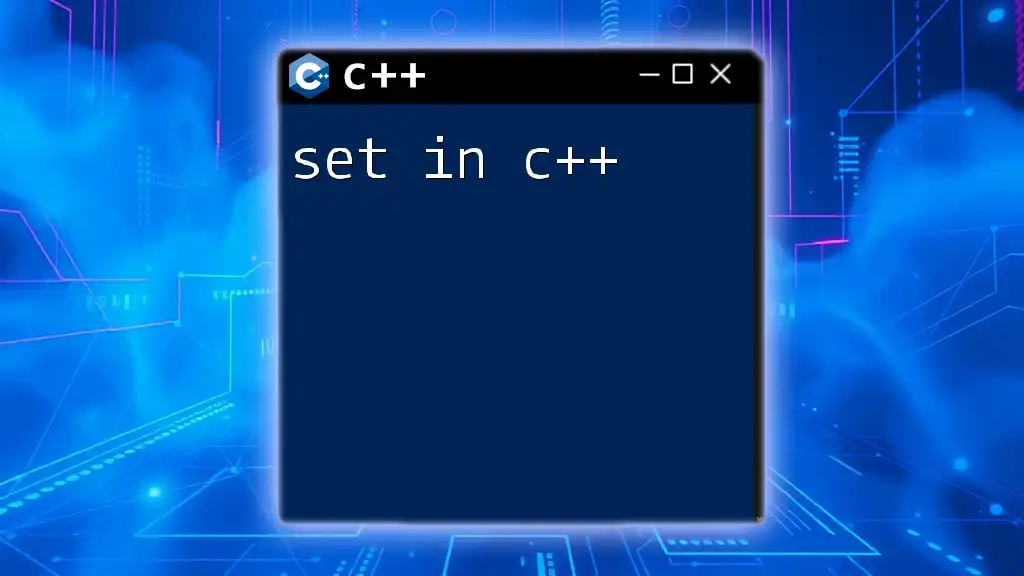
Error Handling with `getline`
Understanding Input Failures
While `getline` is robust, there are occasions when it might fail, such as reaching the end of the input stream or encountering an error. It's essential to know how to handle these cases gracefully.
Example of Error Handling with `getline`
Here's an example that demonstrates how to manage errors effectively:
std::string line;
if (std::getline(std::cin, line)) {
// Process line
} else {
std::cerr << "Error reading line.";
}
In this snippet, the program checks if `getline` successfully captures the input. If it fails, it outputs an error message.
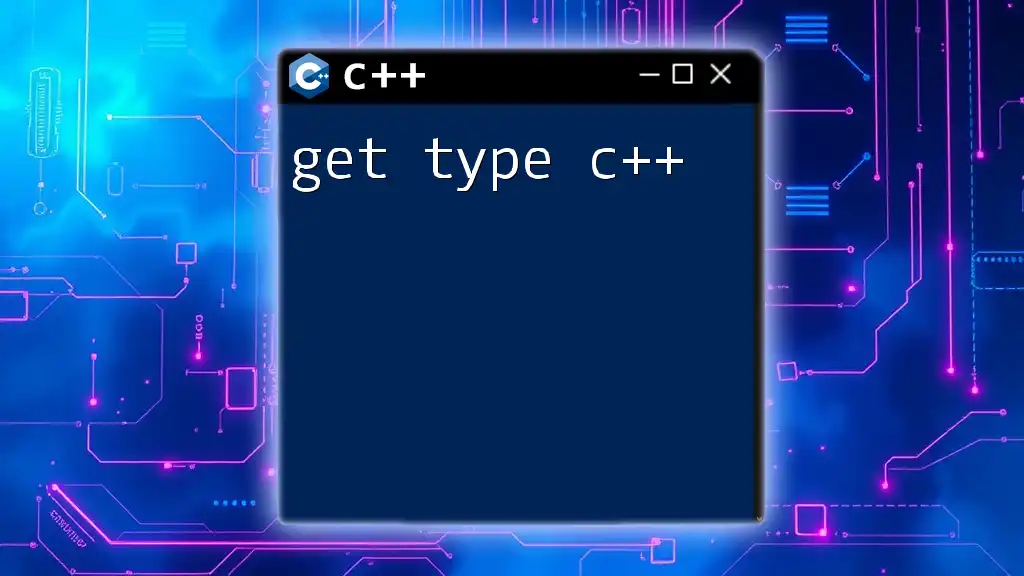
Advanced Features of `getline`
Using `getline` with Delimiters
`getline` also allows for customization through delimiters. By default, it uses the newline character, but you can change this to any character. For example:
std::string data;
std::cout << "Enter comma-separated values: ";
std::getline(std::cin, data, ',');
In this case, `getline` will read input up to the first comma, making it useful for parsing CSV data.
Combining Multiple Inputs with `getline`
You can combine `getline` with other input methods, allowing for more complex input scenarios:
int age;
std::string name;
std::cout << "Enter your name: ";
std::getline(std::cin, name);
std::cout << "Enter your age: ";
std::cin >> age;
This approach facilitates reading structured data where some fields can require full lines, while others may be singular values.
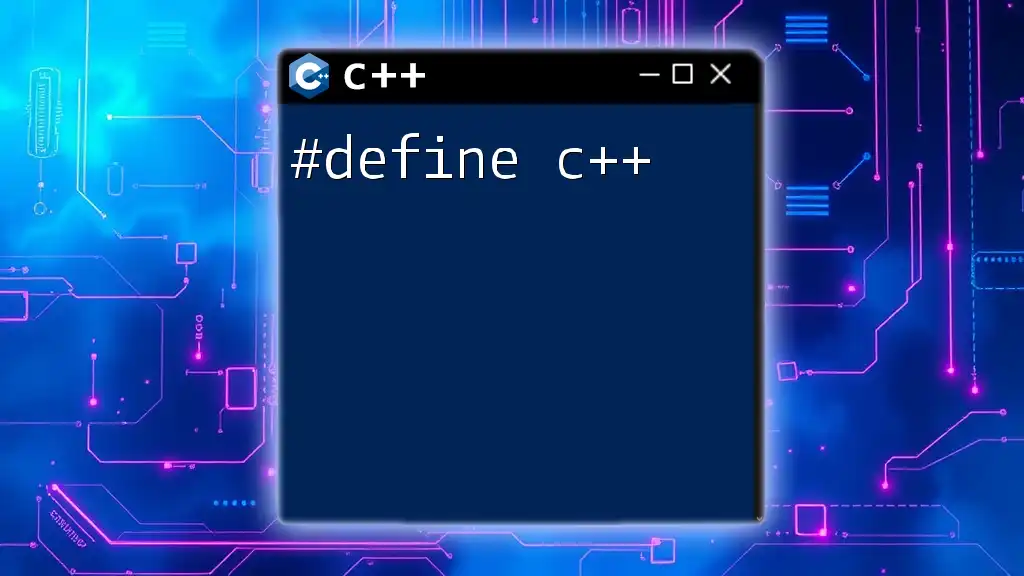
Common Use Cases for `getline` in C++
Reading from Files
`getline` is frequently used to read lines from text files, enabling applications to process file content line by line. Here's an example:
std::ifstream infile("example.txt");
std::string line;
while (std::getline(infile, line)) {
// Process each line
}
This pattern reads each line from the file and allows for further processing, making it ideal for data extraction and transformation tasks.
User Input and Command-Line Arguments
Combining `getline` with command-line arguments provides enhanced flexibility in parsing user-defined inputs and configurations. This empowers your applications to adapt to various input scenarios seamlessly.
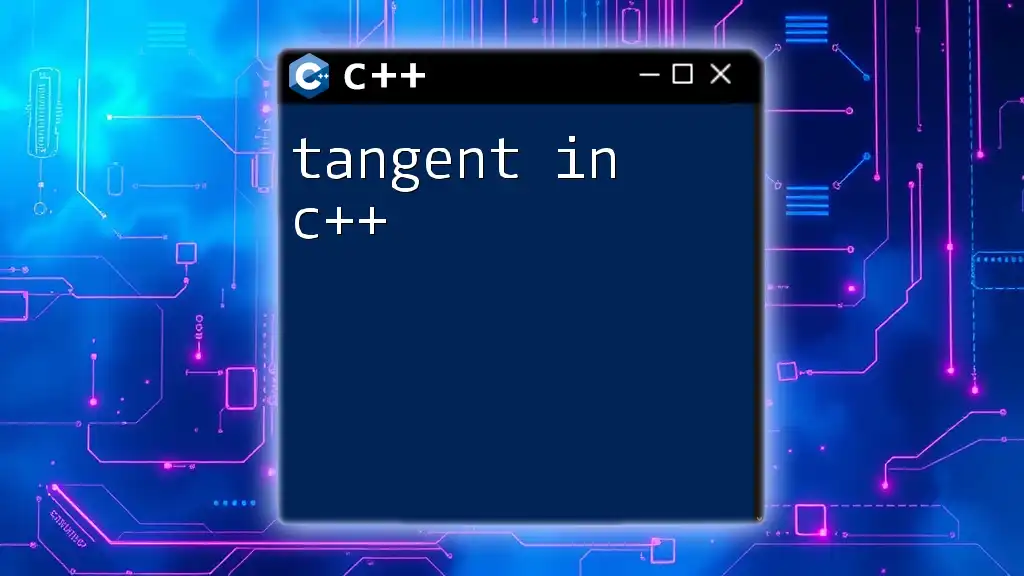
Performance Considerations with `getline`
Memory Management
When utilizing `getline` with `std::string`, memory allocation is dynamically handled, which is generally safe. However, when using `char` arrays, you must ensure that the array size is sufficient to hold the expected input without leading to buffers being overflowed.
Efficiency Tips
To optimize the performance when using `getline`, consider using it in loops to manage batch inputs efficiently instead of processing line-by-line interactively every time user input is required. This helps reduce lag times and makes your application more responsive.
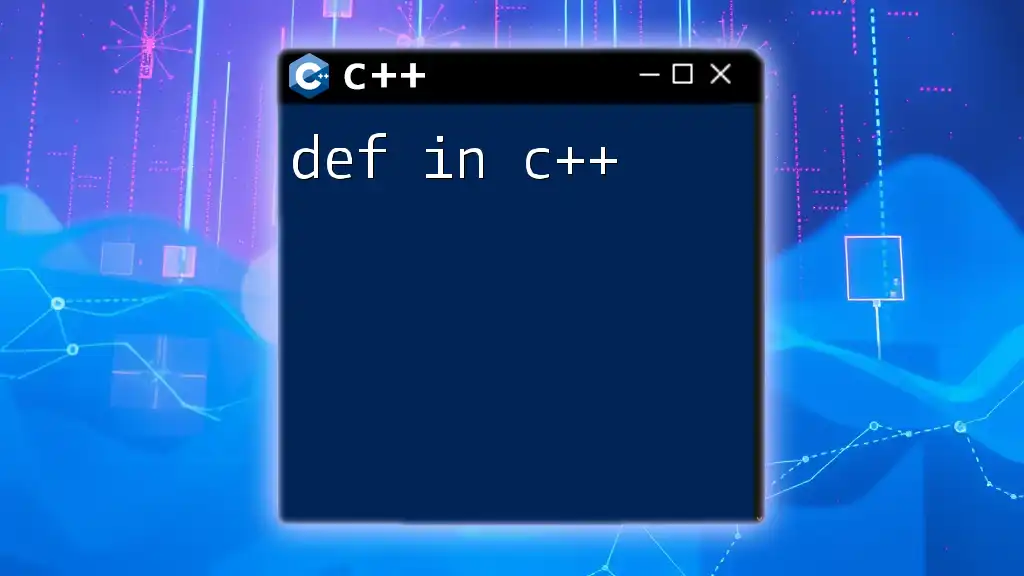
Conclusion
Recap of Key Points
In sum, the `getline` function in C++ is an essential tool for efficiently managing user and file input, allowing for complete line captures without losing space or notable characters. Understanding its syntax, use cases, and advanced features can significantly improve the way you handle string input in your applications.
Call to Action
Now that you are equipped with a comprehensive understanding of `getline`, practice using it in your projects! Feel free to explore its advanced features and share your experiences or questions regarding the implementation of `getline` in C++.