In C++, `cstring` is a header file that provides functions for manipulating C-style strings, which are arrays of characters terminated by a null character.
Here's a simple example of using `cstring` to copy one string to another:
#include <iostream>
#include <cstring>
int main() {
const char* source = "Hello, World!";
char destination[50];
strcpy(destination, source);
std::cout << "Copied string: " << destination << std::endl;
return 0;
}
What is CString?
A CString refers to a character string used in C/C++ that is represented as an array of characters terminated by a null character (`'\0'`). Unlike the `std::string` class in C++, which is part of the C++ Standard Library, C-style strings require manual management of memory and string functions.
The difference between `cstring` and `string` lies in how they manage data. While `std::string` handles memory allocation and provides various features such as dynamic resizing and easy concatenation, `cstring` relies on simple character arrays combined with relevant functions from the C Standard Library. This simplicity can lead to performance gains in specific situations, especially where fine control over memory is needed.
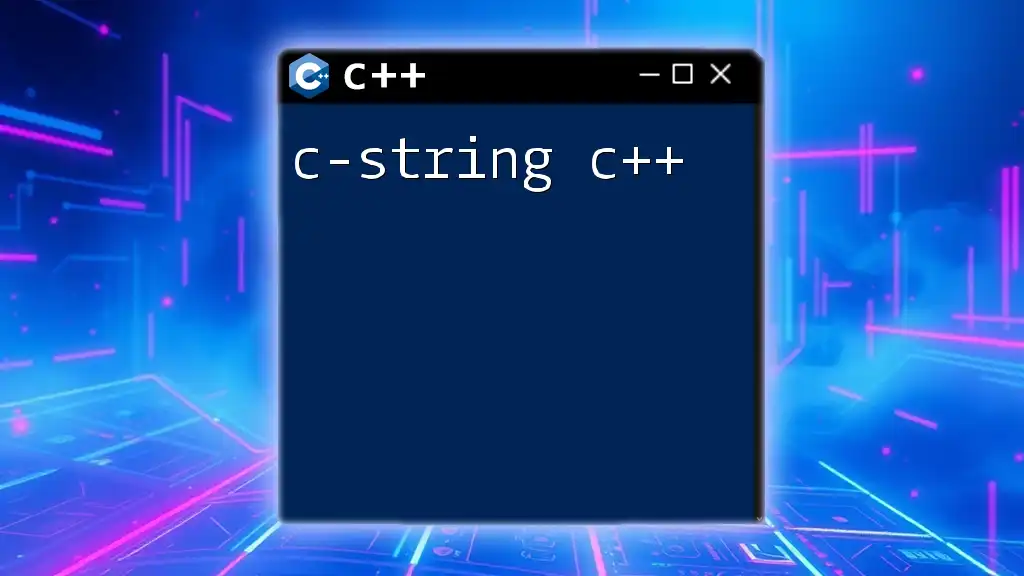
Importance of CString in C++
Understanding and effectively using cstring in C++ is crucial, as it allows developers to manage memory more directly. CString operations can be more efficient in performance-critical applications, such as embedded systems or real-time applications where every byte counts. Having knowledge of `cstring` enables a programmer to work with lower-level string manipulation when required.
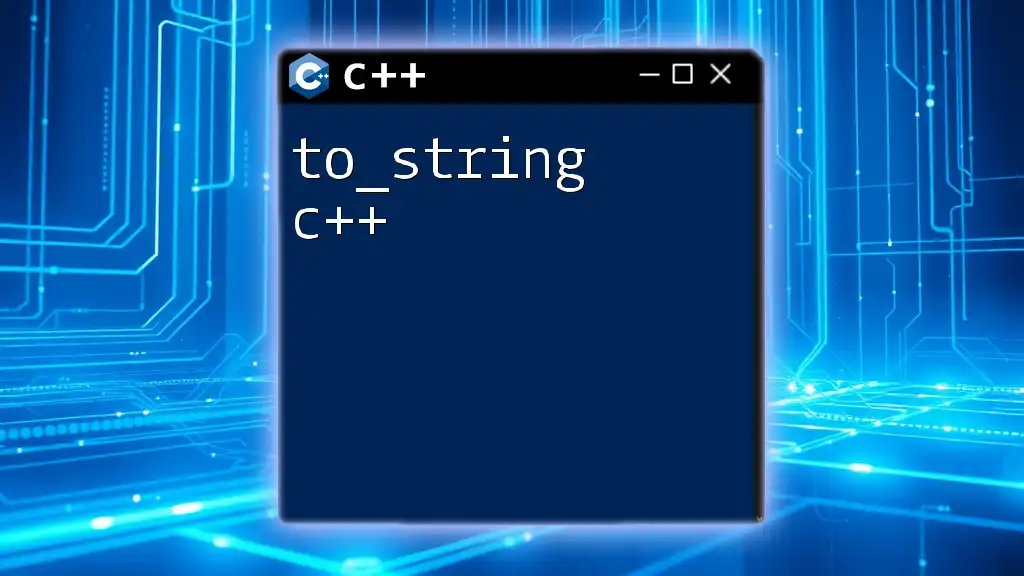
Understanding `cstring` Header
Overview of the `cstring` Library
To utilize `cstring` in your C++ programs, you must include the header file:
#include <cstring>
This inclusion allows access to various functions designed for manipulating C-style strings, including length calculations, copying, concatenation, and comparison.
Common `cstring` Functions
`strlen()`
The `strlen()` function computes the length of a given string (excluding the null terminator). Here's how it works:
#include <iostream>
#include <cstring>
int main() {
const char* str = "Hello World";
std::cout << "Length: " << strlen(str) << std::endl; // Outputs: Length: 11
return 0;
}
In this example, the output is `11`, representing the number of characters in "Hello World".
`strcpy()` and `strncpy()`
The `strcpy()` function copies a string from source to destination. However, care must be taken to ensure that the destination array is large enough to hold the copied string to avoid buffer overflows.
Here's how to use `strcpy()`:
char src[20] = "Hello";
char dest[20];
strcpy(dest, src); // Copying string src to dest
On the other hand, `strncpy()` allows copying with the ability to specify the number of characters to copy, which enhances security:
strncpy(dest, src, sizeof(dest) - 1);
dest[sizeof(dest) - 1] = '\0'; // Ensuring null termination
`strcat()` and `strncat()`
The `strcat()` function concatenates one string onto another. However, it’s critical to ensure that the destination string has enough space to accommodate the additional characters.
Here’s an example:
char str1[20] = "Hello";
char str2[20] = " World";
strcat(str1, str2); // str1 now contains "Hello World"
std::cout << str1 << std::endl; // Outputs: Hello World
`strncat()` is a safer alternative, allowing for bounds checking:
strncat(str1, str2, sizeof(str1) - strlen(str1) - 1);
`strcmp()` and `strncmp()`
The `strcmp()` function compares two strings and returns:
- `0` if they are equal,
- a negative value if the first string is less than the second,
- and a positive value if the first is greater.
Here’s an example of how to use `strcmp()`:
if (strcmp(str1, str2) == 0) {
std::cout << "Strings are equal" << std::endl;
}
Using `strncmp()`, you can limit the comparison to a specific number of characters, enhancing safety in certain contexts.
Memory Management in CString
Dynamic Memory Allocation
Using dynamic memory with C-style strings provides flexibility, but it also invites risks such as memory leaks if not managed correctly. Here’s how to allocate and deallocate memory for a C-string:
char* dynamicStr = new char[20];
strcpy(dynamicStr, "Dynamic String");
// Always remember to free the assigned memory
delete[] dynamicStr;
Avoiding Memory Leaks
To prevent memory leaks, ensure every call to `new` is paired with `delete`, and avoid storing pointers to dynamically allocated memory without proper management. Always perform checks before deleting memory.
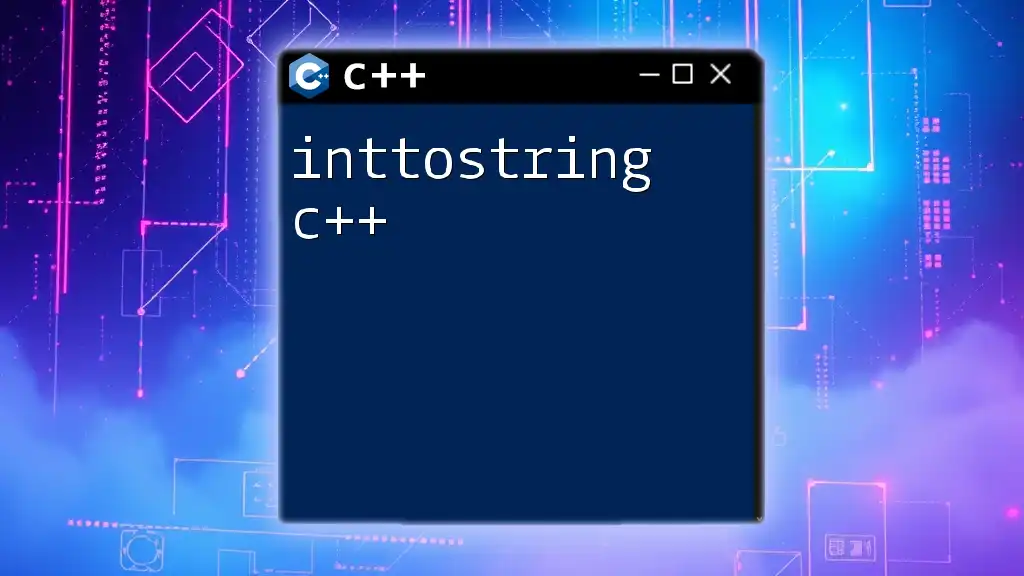
Working with C++ Strings and `cstring`
Transitioning from `string` to `cstring`
While `std::string` is safer and easier to use, there may be cases where C-style strings provide better performance or control. Working directly with `cstring` allows you to handle byte-level operations critical in systems programming.
Interoperability between `cstring` and `std::string`
You may find it necessary to convert between `std::string` and C-style strings. To convert a `std::string` to a C-string, you can use:
std::string stdStr = "Hello";
const char* cStr = stdStr.c_str();
Conversely, if you need to create a `std::string` from a C-style string, use:
const char* cStr = "Hello";
std::string stdStr(cStr);
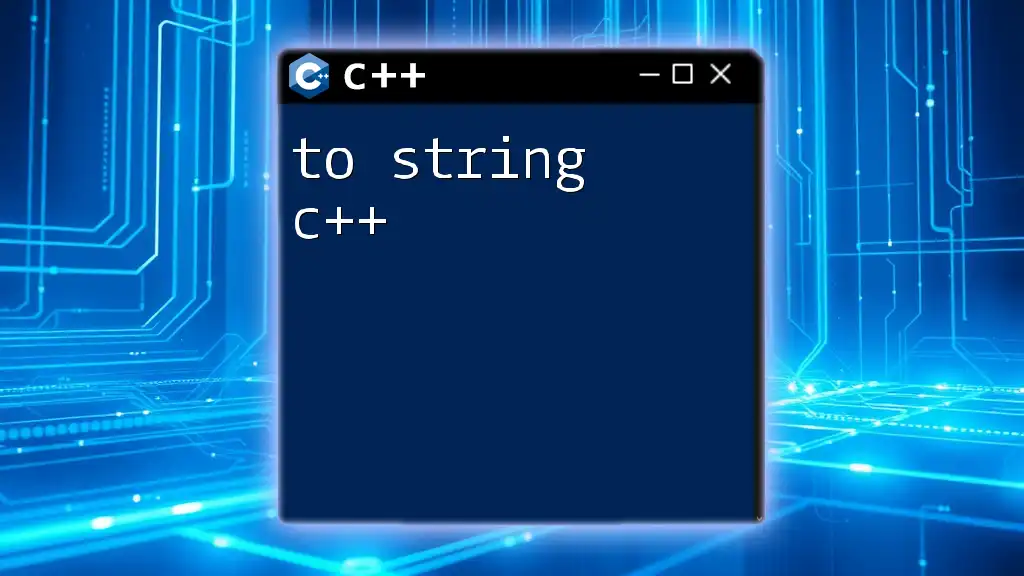
Common Problems and Solutions
Buffer Overflows
One of the most significant risks when using C-style strings is buffer overflow. Careless copying or concatenation can overwrite adjacent memory. Always ensure destination buffers are sufficiently sized to prevent overflow.
A common buffer overflow example:
char buffer[10];
strcpy(buffer, "This string is too long!"); // Dangerous!
To avoid this, use:
strncpy(buffer, "Safe", sizeof(buffer) - 1);
Null Terminators in C-Strings
Null terminators are crucial in defining the end of a C-string. Forgetting to include a null terminator can lead to errors, as functions such as `strlen()` and `strcpy()` rely on it for string length determination and secure copying.
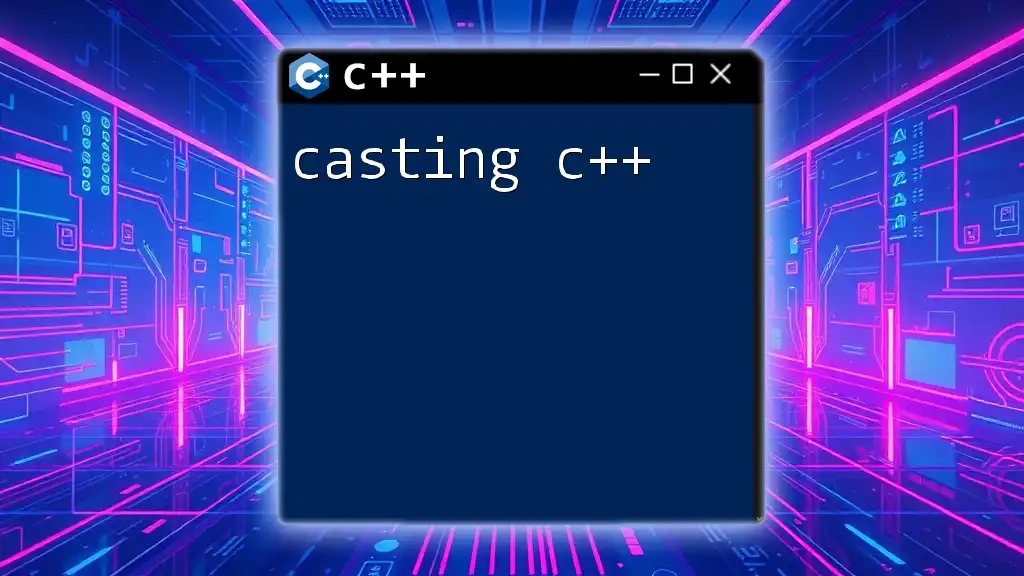
Best Practices for Using CString in C++
Tips for Efficient CString Usage
Utilize smart and safe coding practices when working with `cstring`. Always ensure sufficient buffer sizes and consider using `snprintf()` for safer formatted output. Additionally, validate inputs thoroughly when operating on character arrays.
Recognizing When to Move Away from C-Strings
As a general guideline, while C-style strings are efficient and minimal, they can be unwieldy compared to `std::string`. Utilize `std::string` for routine string manipulation and reserve `cstring` for memory-constrained or performance-critical situations.
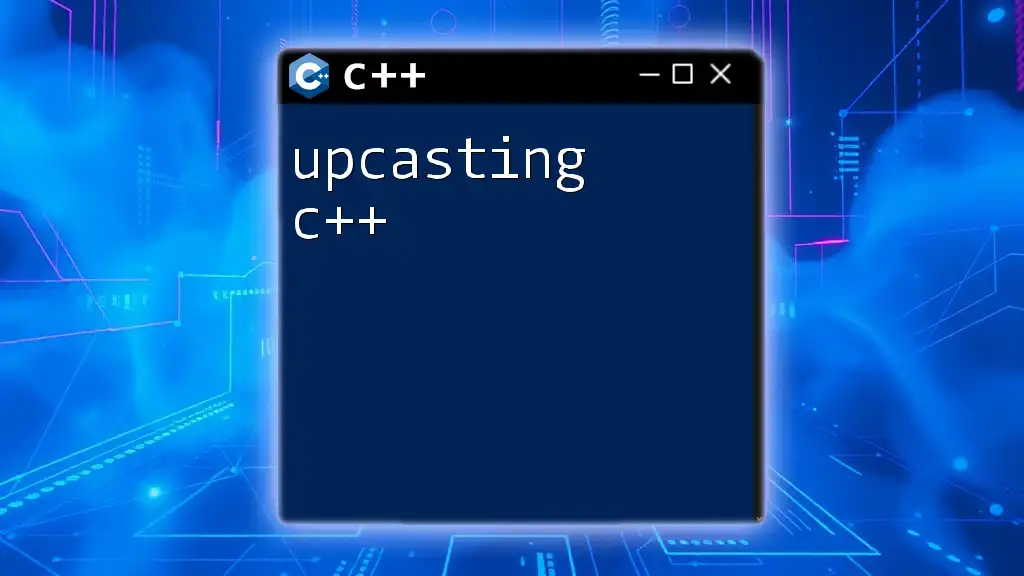
Conclusion
This guide has explored the intricacies of `cstring` in C++, emphasizing its functionalities, nuances in memory management, and contexts in which it excels. As you work with cstring, remember to prioritize safe programming practices, avoid common pitfalls, and recognize when to consider alternative string types. By mastering `cstring`, you'll enhance your programming capabilities and write more efficient, effective C++ code.
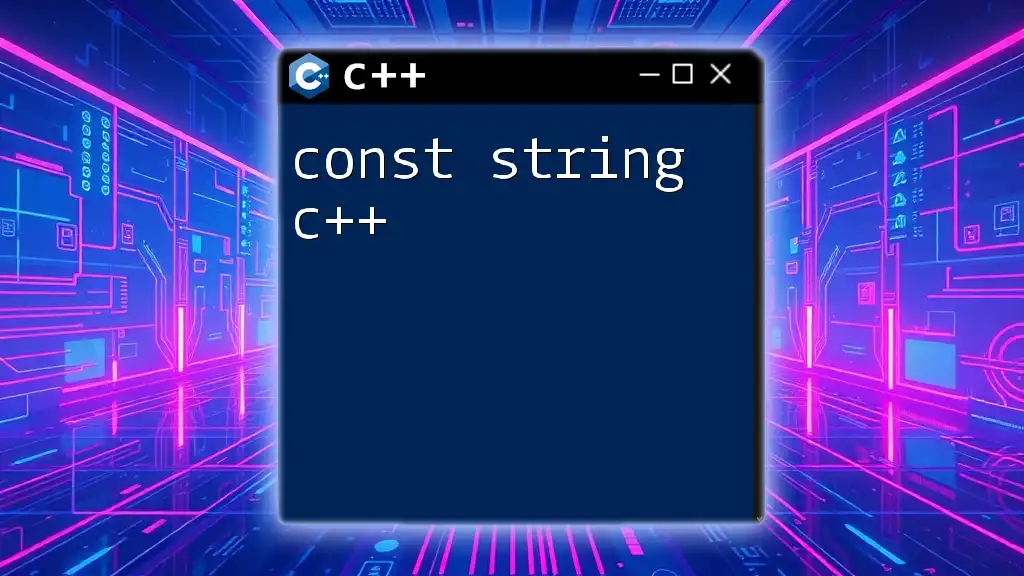
Further Reading and Resources
For those eager to deepen their understanding of `cstring c++`, consider exploring official C++ documentation or specific programming textbooks dedicated to the topic. Engaging with community forums and coding challenges can further cement your skills and knowledge in utilizing C-style strings effectively.
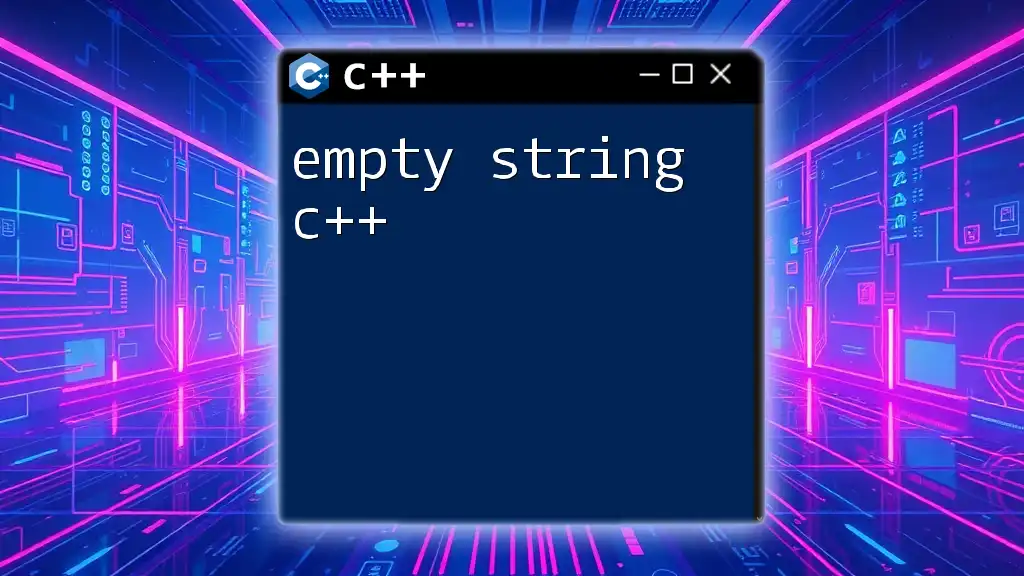
Call to Action
Now that you have acquired a comprehensive understanding of `cstring`, dive into your C++ environment and start experimenting! Implement the examples provided, explore variations, and solidify your grasp on these essential string manipulation techniques.